Spring + dubbo + testNg 环境搭建(一)
前言
本篇 介绍 2 种方式连接 dubbo 服务
- 通过zookeeper 调用 dubbo
- 点对点 直连,直接调用 dubbo
通过zookeeper 注册中心去调用 dubbo服务
粟子: 目标 服务 TestService addEdit()方法
工程 lib 文件下,引入 测试 服务TestService 需要的依赖资源 jar
- 新建 maven工程,在pom.xml配置 添加以下依赖:dubbo、spring、TestNG
- 还需要添加 对应 测试服务的依赖 test service
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.hank.demo</groupId>
<artifactId>AutoApi</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>AutoApi</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>dubbo</artifactId>
<version>2.6.2</version>
</dependency>
<dependency>
<groupId>org.apache.curator</groupId>
<artifactId>curator-framework</artifactId>
<version>2.9.1</version>
</dependency>
<dependency>
<groupId>org.apache.curator</groupId>
<artifactId>curator-client</artifactId>
<version>2.9.1</version>
</dependency>
<!-- Spring框架依赖:用于构建Spring容器 -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.1.5.RELEASE</version>
</dependency>
<!-- Spring单元测试依赖:用于测试类启动spring容器 -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-test</artifactId>
<version>5.1.5.RELEASE</version>
</dependency>
<!-- testng依赖:测试框架-->
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>6.14.3</version>
<scope>test</scope>
</dependency>
<!-- Test service -->
<dependency>
<groupId>com.user.test.service</groupId>
<artifactId>TestService</artifactId>
<!--<version>RELEASE</version>-->
</dependency>
</dependencies>
</project>
- Dubbo 服务消费者的spring 配置:resources目录下创建 consumer.xml 配置(消费者 )
消费者 consumer.xml 配置内容,可直接参考 对应服务 TestService 的 消费者配置文件
(可从测试环境 对应服务的 部署路径下查找对应的 消费者配置文件 如:dubbo_client.xml)
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:dubbo="http://code.alibabatech.com/schema/dubbo"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://code.alibabatech.com/schema/dubbo
http://code.alibabatech.com/schema/dubbo/dubbo.xsd">
<description>Dubbo Consumer 配置</description>
<!-- id为接口的名字在程序种应用时可以通过@Resource注解实例化。
interface为引用的接口的类的全路径。
registry 为仓库的地址的id
check 为spring初始化时是否检查服务是否可用
-->
<dubbo:application name="Test-service" owner="hank"/>
<dubbo:consumer timeout="5000" check="false" lazy="true" retries="0"/>
<dubbo:registry id="regTest" address="zookeeper://192.168.1.60:2020"/>
<dubbo:reference id="TestService" interface="com.user.test.service.TestService" registry="regTest" check="false" version="2.0.0"/>
</beans>
- 测试类编写
package com.hank.demo;
import com.user.test.service.criteria.TestServicePlayCriteria;
import com.user.common.service.params.Empty;
import com.user.common.service.params.Result;
import org.springframework.test.context.ContextConfiguration;
import com.user.test.service.service.TestService;
import org.springframework.test.context.testng.AbstractTestNGSpringContextTests;
import org.testng.annotations.Test;
import ort.testng.Repoter;
import javax.annotation.Resource;
/**
* Description
* dubbo 接口 通过 zookeeper注册中心 连接
* @Author Hank
* @Date Created in 2019-12-20 17:00
*/
@ContextConfiguration(locations = "classpath:applicationContext-dubbo-consumer.xml")
public class TestBase extends AbstractTestNGSpringContextTests {
@Resource
public TestService testService;
@Test
public void test1(){
long userId = 505009;
TestServicePlayCriteria testServicePlayCriteria = new TestServicePlayCriteria();
testServicePlayCriteria.setDrugName("test_dubbo");
testServicePlayCriteria.setDosage("50");
testServicePlayCriteria.setEffect("test");
testServicePlayCriteria.setUserId(userId);
// TestService.addOrEdit() return:Result<Empty>
Result<Empty> result = testService.addOrEdit(testServicePlayCriteria);
//testNg 打印
Repoter.log(result.getCode())
//控制台打印
System.out.println(result.getCode());
}
}
- 调用结果
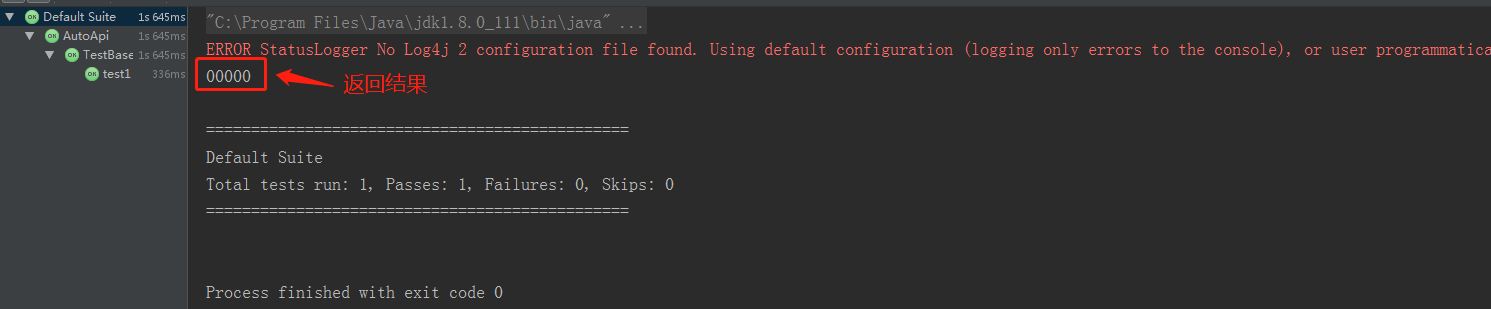
点对点 直连,不经过zk,直接调用 dubbo接口
直连 不需要consumer配置 ,TestService dubbo(生产者) 服务地址:192.168.1.80 1090
package com.hank.demo;
import com.alibaba.dubbo.config.ApplicationConfig;
import com.alibaba.dubbo.config.ReferenceConfig;
import com.user.test.service.criteria.TestServicePlayCriteria;
import com.user.test.service.service.TestService;
import com.user.common.service.params.Empty;
import com.user.common.service.params.Result;
/**
* Description
* dubbo 接口 直连dubbo 生产者 点对点
* @Author Hank
* @Date Created in 2019-12-24 10:37
*/
public class DubboInvoke {
public static void main(String args[]){
testInvoke();
}
public static void testInvoke(){
ApplicationConfig application = new ApplicationConfig();
application.setName("test");
ReferenceConfig<TestService> reference = new ReferenceConfig<TestService>();
reference.setApplication(application);
// 如果点对点直连,可以用reference.setUrl()指定目标地址,设置url后将绕过注册中心,
// 其中,协议对应provider.setProtocol()的值,端口对应provider.setPort()的值,
// 路径对应service.setPath()的值,如果未设置path,缺省path为接口名
reference.setUrl(String.format("dubbo://%s:%s/","192.168.1.80","1090"));
reference.setVersion("2.0.0");
reference.setTimeout(50000);
reference.setInterface("com.user.test.service.service.TestService");
TestService testService = reference.get();
long userId = 509005;
TestServicePlayCriteria testServicePlayCriteria = new TestServicePlayCriteria();
testServicePlayCriteria.setDrugName("test_dubbo");
testServicePlayCriteria.setDosage("50");
testServicePlayCriteria.setEffect("test");
testServicePlayCriteria.setUserId(userId);
Result<Empty> result = TestService.addOrEdit(testServicePlayCriteria);
System.out.println(result.getCode());
}
}
- 调用结果

基本环境搭建ok后,后续就可以进行相关的 接口功能测试了