React AntDesign组件库
AntDesign的安装
npm install antd –save
或
yarn add antd
- 我们需要在index.js中引入全局的Antd样式:
import "antd/dist/antd.css";
npm install --save @ant-design/icons
- 考虑一个问题:Antd是否会将一些没有用的代码(组件或者逻辑代码)引入,造成包很大呢?
- antd 官网有提到:antd 的 JS 代码默认支持基于 ES modules 的 tree shaking,对于 js 部分,直接引入 import { Button } from 'antd' 就会有按需加载的效果
认识craco
- 上面的使用过程是无法对主题进行配置的,好像对主题等相关的高级特性进行配置,需要修改create-react-app 的默认配置。
- 如何修改create-react-app 的默认配置呢?
- 前面我们讲过,可以通过yarn run eject来暴露出来对应的配置信息进行修改;
- 但是对于webpack并不熟悉的人来说,直接修改 CRA 的配置是否会给你的项目带来负担,甚至会增加项目的隐患和不稳定性呢?
- 所以,在项目开发中是不建议大家直接去修改 CRA 的配置信息的;
- 那么如何来进行修改默认配置呢?社区目前有两个比较常见的方案:
- react-app-rewired + customize-cra;(这个是antd早期推荐的方案)
- craco;(目前antd推荐的方案)
Craco的使用步骤
yarn add @craco/craco
- 第二步:修改package.json文件
- 原本启动时,我们是通过react-scripts来管理的;
- 现在启动时,我们通过craco来管理
/* package.json */
"scripts": {
- "start": "react-scripts start",
- "build": "react-scripts build",
- "test": "react-scripts test",
+ "start": "craco start",
+ "build": "craco build",
+ "test": "craco test",
}
- 第三步:在根目录下创建craco.config.js文件用于修改默认配置
/* craco.config.js */
module.exports = {
// ...
};
配置主题
- 按照 配置主题 的要求,自定义主题需要用到类似 less-loader 提供的 less 变量覆盖功能:
- 我们可以引入 craco-less 来帮助加载 less 样式和修改变量;
- 安装 craco-less:
yarn add craco-less
- 修改craco.config.js中的plugins:
- 使用modifyVars可以在运行时修改LESS变量;
const CracoLessPlugin = require('craco-less');
module.exports = {
plugins: [
{
plugin: CracoLessPlugin,
options: {
lessLoaderOptions: {
lessOptions: {
modifyVars: { '@primary-color': '#1DA57A' },
javascriptEnabled: true,
},
},
},
},
],
};
- 引入antd的样式时,引入antd.less文件:
import 'antd/dist/antd.less';
- 修改后重启 yarn start,如果看到一个绿色的按钮就说明配置成功了。
配置别名
- 在项目开发中,某些组件或者文件的层级会较深,
- 如果我们通过上层目录去引入就会出现这样的情况:../../../../components/button;
- 如果我们可以配置别名,就可以直接从根目录下面开始查找文件:@/components/button,甚至是:components/button;
- 配置别名也需要修改webpack的配置,当然我们也可以借助于 craco 来完成:
- craco.config.js:
const CracoLessPlugin = require('craco-less');
const path = require("path");
const resolve = dir => path.resolve(__dirname, dir);
module.exports = {
plugins: [
{
plugin: CracoLessPlugin,
options: {
lessLoaderOptions: {
lessOptions: {
modifyVars: { '@primary-color': '#1DA57A' },
javascriptEnabled: true,
},
},
},
}
],
webpack: {
alias: {
"@": resolve("src"),
"components": resolve("src/components")
}
}
}
AntDesign案例
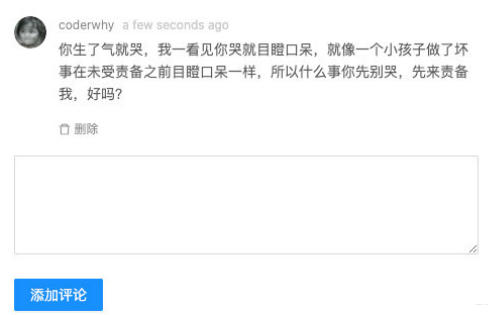
import React, { PureComponent } from 'react';
import moment from 'moment';
import { Input, Button } from "antd";
export default class CommentInput extends PureComponent {
constructor(props) {
super(props);
this.state = {
content: ""
}
}
render() {
return (
<div>
<Input.TextArea rows={4}
value={this.state.content}
onChange={e => this.handleChange(e)}/>
<Button type="primary" onClick={e => this.addComment()}>添加评论</Button>
</div>
)
}
handleChange(event) {
this.setState({
content: event.target.value
})
}
addComment() {
const commentInfo = {
id: moment().valueOf(),
avatar: "https://upload.jianshu.io/users/upload_avatars/1102036/c3628b478f06.jpeg?imageMogr2/auto-orient/strip|imageView2/1/w/240/h/240",
nickname: "coderwhy",
datetime: moment(),
content: this.state.content,
comments: [
]
}
this.props.submitComment(commentInfo);
this.setState({
content: ""
})
}
}
import React, { PureComponent } from 'react';
import {
Comment,
Avatar,
Tooltip
} from "antd";
import { DeleteOutlined } from "@ant-design/icons";
export default class CommentItem extends PureComponent {
render() {
const { nickname, avatar, content, datetime } = this.props.comment;
return (
<Comment
author={<a href="/#">{nickname}</a>}
avatar={<Avatar src={avatar} alt={nickname} />}
content={<p>{content}</p>}
datetime={
<Tooltip title={datetime.format("YYYY-MM-DD")}>
<span>{datetime.fromNow()}</span>
</Tooltip>
}
actions={[
<span onClick={e => this.removeItem()}><DeleteOutlined />删除</span>
]}
/>
)
}
removeItem() {
this.props.removeItem();
}
}
import React, { PureComponent } from 'react';
import CommentInput from './components/CommentInput';
import CommentItem from './components/CommentItem';
export default class App extends PureComponent {
constructor(props) {
super(props);
this.state = {
commentList: []
}
}
render() {
return (
<div style={{width: "500px", padding: "20px"}}>
{
this.state.commentList.map((item, index) => {
return <CommentItem key={item.id}
comment={item}
removeItem={e => this.removeComment(index)}/>
})
}
<CommentInput submitComment={this.submitComment.bind(this)}/>
</div>
)
}
submitComment(info) {
this.setState({
commentList: [...this.state.commentList, info]
})
}
removeComment(index) {
const newCommentList = [...this.state.commentList];
newCommentList.splice(index, 1);
this.setState({
commentList: newCommentList
})
}
}