这个是对初学者实用的,全部都是利用线性布局实现的相对比较复杂
先上效果图
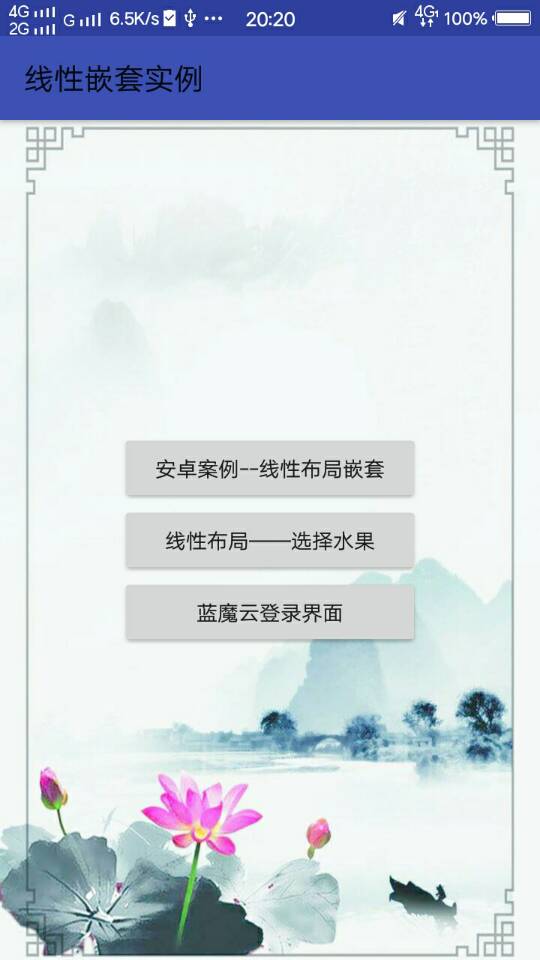 {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
public void doWeight(View view){
Intent intent = new Intent(MainActivity.this,WeightActivity.class);
startActivity(intent);
}
public void doChoose(View view){
Intent intent = new Intent(MainActivity.this,ChooseActivity.class);
startActivity(intent);
}
public void doMy(View view){
Intent intent = new Intent(MainActivity.this,MyActivity.class);
startActivity(intent);
}
}
编写AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.administrator.nest">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity
android:name=".WeightActivity"
android:label="@string/weight_name" />
<activity
android:name=".ChooseActivity"
android:label="@string/choose_name" />
<activity
android:name=".MyActivity"
android:label="@string/my_name" />
</application>
</manifest>
上面的代码,就简单的实现了窗口的切换。
好。。基本工作我们已经完成了
现在写第一个图线性嵌套
1.在布局文件中编写activity_weight.xml文件,代码如下
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@mipmap/bg"
android:orientation="vertical"
tools:context="com.example.administrator.nest.WeightActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="2">
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ff0000" />
</LinearLayout>
<!--中间LinearLayout-->
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="5"
android:gravity="center"
android:background="#ffff00" >
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:gravity="center">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/btn_name" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/btn_name" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/btn_name"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/btn_name" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:gravity="center">
<TextView
android:layout_width="300dp"
android:layout_height="150dp"
android:text="@string/tv_content"
android:inputType="textMultiLine"
android:maxLines="6"
android:background="#999999"
android:textSize="20sp"
android:padding="15dp"
/>
</LinearLayout>
</LinearLayout>
</LinearLayout>
<!--第三个-->
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="3"
android:orientation="horizontal" >
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:background="#ff00ff"
android:layout_weight="3"
android:orientation="vertical"
android:gravity="center">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/btn_name"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/btn_name"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/btn_name"/>
</LinearLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_weight="4"
android:layout_height="match_parent"
android:background="#0fffff"
android:orientation="horizontal"
android:gravity="center">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/btn_name"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/btn_name"/>
</LinearLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_weight="3"
android:layout_height="match_parent"
android:background="#ff0000"
android:orientation="vertical"
android:gravity="center">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/btn_name"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/btn_name"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/btn_name"/>
</LinearLayout>
</LinearLayout>
</LinearLayout>
编写WeightActivity.java文件
package com.example.administrator.nest;
import android.app.Activity;
import android.os.Bundle;
public class WeightActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_weight);
}
}
第二线性嵌套的九公格效果
编写 activity_choose.xml文件
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:background="@mipmap/bg"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal"
android:gravity="center"
tools:context="com.example.administrator.nest.ChooseActivity">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:orientation="vertical"
android:layout_marginLeft="20dp" >
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center_horizontal"
android:src="@mipmap/k1" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="0dp"
android:lines="1"
android:text="@string/apple"
android:textSize="18sp" />
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center_horizontal"
android:src="@mipmap/k1" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="0dp"
android:lines="1"
android:text="@string/apple"
android:textSize="18sp" />
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center_horizontal"
android:src="@mipmap/k1" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="0dp"
android:lines="1"
android:text="@string/apple"
android:textSize="18sp" />
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center_horizontal"
android:src="@mipmap/k1" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="0dp"
android:lines="1"
android:text="@string/apple"
android:textSize="18sp" />
</LinearLayout>
<!--2-->
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="left"
android:layout_marginLeft="20dp"
android:orientation="vertical">
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center_horizontal"
android:src="@mipmap/k1" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="0dp"
android:lines="1"
android:text="@string/apple"
android:textSize="18sp" />
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center_horizontal"
android:src="@mipmap/k1" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="0dp"
android:lines="1"
android:text="@string/apple"
android:textSize="18sp" />
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center_horizontal"
android:src="@mipmap/k1" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="0dp"
android:lines="1"
android:text="@string/apple"
android:textSize="18sp" />
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center_horizontal"
android:src="@mipmap/k1" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="0dp"
android:lines="1"
android:text="@string/apple"
android:textSize="18sp" />
</LinearLayout>
<!--3-->
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="left"
android:layout_marginLeft="20dp"
android:orientation="vertical">
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center_horizontal"
android:src="@mipmap/k1" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="0dp"
android:lines="1"
android:text="@string/apple"
android:textSize="18sp" />
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center_horizontal"
android:src="@mipmap/k1" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="0dp"
android:lines="1"
android:text="@string/apple"
android:textSize="18sp" />
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center_horizontal"
android:src="@mipmap/k1" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="0dp"
android:lines="1"
android:text="@string/apple"
android:textSize="18sp" />
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center_horizontal"
android:src="@mipmap/k1" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="0dp"
android:lines="1"
android:text="@string/apple"
android:textSize="18sp" />
</LinearLayout>
<!--4-->
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="left"
android:orientation="vertical"
android:layout_marginLeft="20dp"
>
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center_horizontal"
android:src="@mipmap/k1" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="0dp"
android:lines="1"
android:text="@string/apple"
android:textSize="18sp" />
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center_horizontal"
android:src="@mipmap/k1" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="0dp"
android:lines="1"
android:text="@string/apple"
android:textSize="18sp" />
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center_horizontal"
android:src="@mipmap/k1" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="0dp"
android:lines="1"
android:text="@string/apple"
android:textSize="18sp" />
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center_horizontal"
android:src="@mipmap/k1" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="0dp"
android:lines="1"
android:text="@string/apple"
android:textSize="18sp" />
</LinearLayout>
</LinearLayout>
编写ChooseActivity文件
package com.example.administrator.nest;
import android.app.Activity;
import android.os.Bundle;
public class ChooseActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_choose);
}
}
编写第三个 小实例 蓝墨云界面
编写activity_my.xml文件
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#EDEDED"
android:orientation="vertical"
tools:context="com.example.administrator.nest.MyActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:background="#ffffff">
<ImageView
android:layout_width="80dp"
android:layout_height="60dp"
android:src="@mipmap/timg" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="@string/name"
android:textSize="20sp" />
</LinearLayout>
<!--kaishi-->
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<View
android:layout_width="wrap_content"
android:layout_height="1dp"
android:background="#aaaaaa" />
<!--1开始-->
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal">
<LinearLayout
android:layout_width="95dp"
android:layout_height="wrap_content"
android:orientation="vertical"
android:background="#ffffff">
<TextView
android:layout_width="95dp"
android:layout_height="20dp"
android:layout_gravity="bottom"
android:layout_marginLeft="28dp"
android:layout_marginTop="10dp"
android:text="@string/tv_number01"
android:textColor="#00EE00"
android:textSize="14sp" />
<TextView
android:layout_width="95dp"
android:layout_height="30dp"
android:layout_gravity="top"
android:layout_marginLeft="18dp"
android:text="@string/tv_values"
android:textColor="#A6A6A6"
android:textSize="18sp" />
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal">
<View
android:layout_width="1dp"
android:layout_height="60dp"
android:background="#000000" />
</LinearLayout>
<!--第二次开始-->
<LinearLayout
android:layout_width="95dp"
android:layout_height="wrap_content"
android:orientation="vertical"
android:background="#ffffff">
<TextView
android:layout_width="95dp"
android:layout_height="20dp"
android:layout_gravity="bottom"
android:layout_marginLeft="28dp"
android:layout_marginTop="10dp"
android:text="@string/tv_number01"
android:textColor="#00EE00"
android:textSize="14sp" />
<TextView
android:layout_width="95dp"
android:layout_height="30dp"
android:layout_gravity="top"
android:layout_marginLeft="18dp"
android:text="@string/tv_values"
android:textColor="#A6A6A6"
android:textSize="18sp" />
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal">
<View
android:layout_width="1dp"
android:layout_height="60dp"
android:background="#000000" />
</LinearLayout>
<!--2次end 3次begin-->
<LinearLayout
android:layout_width="95dp"
android:layout_height="wrap_content"
android:orientation="vertical"
android:background="#ffffff">
<TextView
android:layout_width="95dp"
android:layout_height="20dp"
android:layout_gravity="bottom"
android:layout_marginLeft="28dp"
android:layout_marginTop="10dp"
android:text="@string/tv_number01"
android:textColor="#00EE00"
android:textSize="14sp" />
<TextView
android:layout_width="95dp"
android:layout_height="30dp"
android:layout_gravity="top"
android:layout_marginLeft="18dp"
android:text="@string/tv_values"
android:textColor="#A6A6A6"
android:textSize="18sp" />
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal">
<View
android:layout_width="1dp"
android:layout_height="60dp"
android:background="#000000" />
</LinearLayout>
<!--3end 4begin-->
<LinearLayout
android:layout_width="95dp"
android:layout_height="wrap_content"
android:orientation="vertical"
android:background="#ffffff">
<TextView
android:layout_width="95dp"
android:layout_height="20dp"
android:layout_gravity="bottom"
android:layout_marginLeft="28dp"
android:layout_marginTop="10dp"
android:text="@string/tv_number01"
android:textColor="#00EE00"
android:textSize="14sp" />
<TextView
android:layout_width="95dp"
android:layout_height="30dp"
android:layout_gravity="top"
android:layout_marginLeft="18dp"
android:text="@string/tv_values"
android:textColor="#A6A6A6"
android:textSize="18sp" />
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal">
<View
android:layout_width="1dp"
android:layout_height="60dp"
android:background="#000000" />
</LinearLayout>
<!--end-->
</LinearLayout>
<View
android:layout_width="wrap_content"
android:layout_height="1dp"
android:background="#aaaaaa" />
</LinearLayout>
<View
android:layout_width="wrap_content"
android:layout_height="1dp"
android:background="#aaaaaa"
android:layout_marginTop="20dp"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="50dp"
android:layout_marginTop="0dp"
android:background="#ffffff">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="10dp"
android:layout_marginTop="10dp"
android:text="@string/myset"
android:textSize="16sp" />
</LinearLayout>
<View
android:layout_width="wrap_content"
android:layout_height="1dp"
android:background="#aaaaaa" />
<!--中间4格结束-->
<!--我的空间-->
<View
android:layout_width="wrap_content"
android:layout_height="1dp"
android:background="#aaaaaa"
android:layout_marginTop="15dp"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="50dp"
android:layout_marginTop="0dp"
android:background="#ffffff">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="@string/mykongjian"
android:layout_marginLeft="10dp"
android:layout_marginTop="15dp"
android:textSize="16sp"/>
</LinearLayout>
<View
android:layout_width="wrap_content"
android:layout_height="1dp"
android:background="#aaaaaa" />
<!--我的收藏-->
<LinearLayout
android:layout_width="match_parent"
android:layout_height="50dp"
android:background="#ffffff">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="@string/myshouchang"
android:layout_marginLeft="10dp"
android:layout_marginTop="15dp"
android:textSize="16sp"/>
</LinearLayout>
<View
android:layout_width="wrap_content"
android:layout_height="1dp"
android:background="#aaaaaa" />
<!--收到的卡片,心意-->
<!--我的收藏-->
<LinearLayout
android:layout_width="match_parent"
android:layout_height="50dp"
android:background="#ffffff">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="@string/mycard"
android:layout_marginLeft="10dp"
android:layout_marginTop="15dp"
android:textSize="16sp"/>
</LinearLayout>
<View
android:layout_width="wrap_content"
android:layout_height="1dp"
android:background="#aaaaaa" />
<!--分享给朋友-->
<LinearLayout
android:layout_width="match_parent"
android:layout_height="50dp"
android:layout_marginTop="20dp"
android:background="#ffffff"
android:orientation="horizontal">
<TextView
android:layout_width="200dp"
android:layout_height="wrap_content"
android:text="@string/myfriend"
android:layout_marginLeft="10dp"
android:layout_marginTop="15dp"
android:textSize="16sp"/>
<TextView
android:layout_width="200dp"
android:layout_height="wrap_content"
android:text="@string/myfriend2"
android:layout_marginLeft="10dp"
android:layout_marginTop="10dp"
android:textSize="16sp"/>
</LinearLayout>
<View
android:layout_width="wrap_content"
android:layout_height="1dp"
android:background="#aaaaaa" />
<!--设置-->
<View
android:layout_width="wrap_content"
android:layout_height="1dp"
android:background="#aaaaaa" />
<!--最后一栏-->
<LinearLayout
android:layout_width="match_parent"
android:layout_height="100dp"
android:orientation="vertical"
android:background="#ffffff"
android:layout_marginTop="50dp"
android:layout_gravity="bottom">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="50dp">
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:src="@mipmap/one"
android:layout_marginLeft="60dp"/>
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:src="@mipmap/two"
android:layout_marginLeft="60dp"/>
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:src="@mipmap/three"
android:layout_marginLeft="60dp"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="20dp">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/mybanke"
android:layout_marginLeft="70dp"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/myfaxian"
android:layout_marginLeft="80dp"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/my"
android:layout_marginLeft="85dp"/>
</LinearLayout>
</LinearLayout>
</LinearLayout>
编写MyActivity.java文件
package com.example.administrator.nest;
import android.app.Activity;
import android.os.Bundle;
public class MyActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_my);
}
}
公共的Strings文件
<resources>
<string name="app_name">线性嵌套实例</string>
<string name="weight_name">安卓案例--线性布局嵌套</string>
<string name="choose_name">线性布局——选择水果</string>
<string name="my_name">我的</string>
<string name="str_weigtht">安卓案例--线性布局嵌套</string>
<string name="str_my">蓝魔云登录界面</string>
<string name="str_choose">线性布局——选择水果</string>
<string name="btn_name">按钮1</string>
<string name="tv_content">演示布局的嵌套:横向的 纵向的布局可以相互嵌套,形成比较复杂的布局</string>
<string name="apple">苹果</string>
<string name="name">曾佐艳</string>
<string name="tv_number01">1000</string>
<string name="tv_values">经验值</string>
<string name="mykongjian">我的空间</string>
<string name="myshouchang">我的收藏</string>
<string name="mycard">收到的卡片、心意</string>
<string name="myfriend">分享给朋友</string>
<string name="myfriend2">累计分享成功100次</string>
<string name="myset">设置</string>
<string name="mybanke">班课</string>
<string name="myfaxian">发现</string>
<string name="my">我的</string>
</resources>
所有案例 都是线性布局来编写 代码量比较多,看起来可能 有点晕
建议使用其他布局来写,如果你想看看自己的线性布局学得昨样,可参考
后面我会持续更新的。