今天,为分享的第三个连连看游戏的开发与制作。今天分享第三个版本的连连看游戏一样功能完善,界面漂亮。希望大家可以喜欢,喜欢的帮忙点赞和关注,一起热爱编程、热爱进步。
开发环境
开发语言为Java,开发环境Eclipse或者IDEA都可以。运行主程序,或者执行打开JAR文件即可以运行本程序。运行程序可以对准Main.Java文件,点右键 run as application,也可以打包成jar包,双击JAR包即可以。
系统框架
利用JDK自带的SWING框架开发,不需要安装第三方JAR包。纯窗体模式,直接运行Main文件即可以。同时带有详细得设计文档
主要功能
游戏规则
玩家可以将2个相同图案的对子连接起来,连接线不多于3根直线,就可以成功将对子消除。
第一次使用鼠标点击棋盘中的棋子,该棋子此时为“被选中”,以特殊方式显示;再次以鼠标点击其他棋子,若该棋子与被选中的棋子图案相同,且把第一个棋子到第二个棋子连起来,中间的直线不超过3根,则消掉这一对棋子,否则第一颗棋子恢复成未被选中状态,而第二颗棋子变成被选中状态。
胜利条件
·将棋盘上面的对子全部消除掉。按消除的数量排列名次。
·其他玩家都输掉了。
失败条件
·每个玩家选择牌的时间为30秒,超过时间而未消除一对的玩家以判为输掉。
·有一个玩家将所有的牌都全部消掉。
功能要点
选择两个点,如果两点的图片完全一样,并且两点之间可以用不超过3条直线连接起来,则该两点可以消除。图框消除的要求:
两个方块不能是同一个坐标
两个方块必须是同种类型(图案)
两个方块中不能有任何一个已经消除过的(消除过后的值用 mark 表示)
实时显示连连看消除过后的分数,消除一个得一分,以此类推
运行效果
1 系统主界面
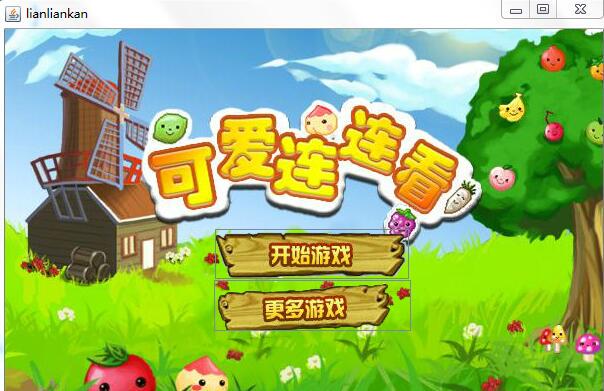
2 开始界面
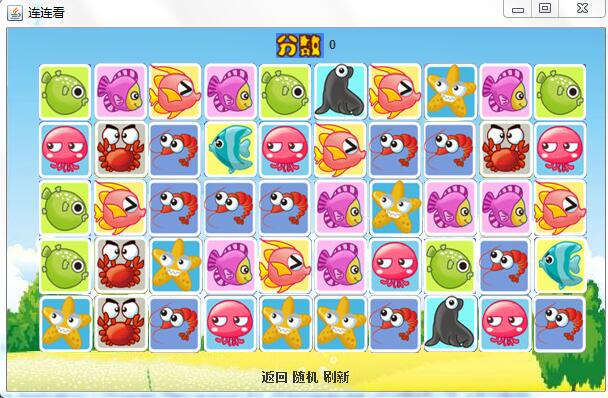
3 游戏中界面
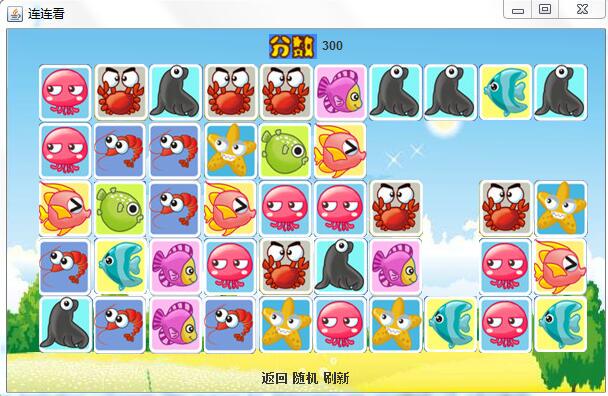
关键代码
public class MapUI
extends JPanel
implements ActionListener, Runnable {
private Map map;
private JButton[] dots;
private Point lastPoint = new Point(0, 0); ///上一个点的坐标
private boolean isSelected = false; //是否已经选择了一个点
private int score = 0; //记录用户的得分
private ClockAnimate clockAnimate; //同步显示时钟
//AnimateDelete animate;
JButton goTop10;
private ScoreAnimate scoreAnimate;
int stepScore = 0;
int limitTime = 0;
private boolean isPlaying = false;
public MapUI(Map map, JButton[] dots) {
this.map = map;
this.dots = dots;
//animate = new AnimateDelete(dots);
GridLayout gridLayout = new GridLayout();
this.setLayout(gridLayout);
gridLayout.setRows(Setting.ROW);
gridLayout.setColumns(Setting.COLUMN);
gridLayout.setHgap(2);
gridLayout.setVgap(2);
this.setLayout(gridLayout);
this.setBackground(Interface.DarkColor);
for (int row = 0; row < Setting.ROW; row++) {
for (int col = 0; col < Setting.COLUMN; col++) {
int index = row * Setting.COLUMN + col;
dots[index].addActionListener(this);
this.add(dots[index]);
}
}
}
public void setMap(Map map) {
this.map = map;
}
public void setTop10Button(JButton goTop10) {
this.goTop10 = goTop10;
}
private void paint() {
for (int row = 0; row < Setting.ROW; row++) {
for (int col = 0; col < Setting.COLUMN; col++) {
int index = row * Setting.COLUMN + col;
/*message("row = " + row + ", col = " + col + ", index = " + index
+ ", map[][] = " + map.getMap()[row][col]);*/
if (map.getMap()[row][col] > 0) {
dots[index].setIcon(Interface.BlocksIcon[map.getMap()[row][col] - 1]);
dots[index].setEnabled(true);
}
else {
dots[index].setIcon(null);
dots[index].setEnabled(false);
}
}
}
}
public void repaint(Graphics g) {
paint();
}
/**
*
* @param a
* @return
*/
private boolean validMap(Point a) {
if (map.getCount() == 0) {
return true;
}
Line line = new Line(0, new Point(), new Point());
map.setTest(true);
line = map.findNext(a);
int offset = 0;
if (line.direct == 1) {
return true;
}
else {
return false;
}
}
/**
*
* @param l
* @param c
*/
private void showScore(int l, int c) {
if (scoreAnimate == null) {
return;
}
scoreAnimate.setScore(l, c);
}
/**
* ˢ
*/
public void refresh() {
if (!isPlaying) {
return;
}
if (map.getCount() == 0) {
Interface.showHint("刷新结束");
}
if (Setting.Sound == 1) {
new Sound(Sound.REFRESH);
}
if (validMap(new Point( -1, -1))) {
score -= Setting.freshScore;
showScore(score - 1, score);
}
else {
showScore(score, score + Setting.freshScore);
score += Setting.freshScore;
}
score -= Setting.freshScore;
showScore(score - 1, score);
map.refresh();
paint();
}
/**
*
* @param a
* @param b
*/
void earse(Point a, Point b) {
//paint();
int offset;
offset = a.x * Setting.COLUMN + a.y;
dots[offset].setIcon(null);
dots[offset].setEnabled(false);
offset = b.x * Setting.COLUMN + b.y;
dots[offset].setIcon(null);
dots[offset].setEnabled(false);
//�
if (map.getCount() == 0) {
int remainTime = clockAnimate.getUsedTime();
message("remainTime = " + remainTime);
if (remainTime > 0) {
showScore(score, score + remainTime * Setting.timeScore);
score += remainTime * Setting.timeScore;
}
isPlaying = false;
stop();
Pass frame=new Pass();
frame.setTitle("过关啦");
frame.setSize(620,393);
Dimension screenSize=Toolkit.getDefaultToolkit().getScreenSize();
int screenWidth = screenSize.width;
int screenHeight =screenSize.height;
int x=(screenWidth-frame.getWidth())/2;
int y=(screenHeight-frame.getHeight())/2;
frame.setLocation(x+78,y+21);
frame.setVisible(true);
Interface.showHint("时间剩余" + remainTime + "秒,想看看你的排名吗?");
goTop10.setEnabled(true);
}
else {
//test1(map.getDeleteArray());
}
}
/**
*
* @param a
*/
public void findNext(Point a) {
if (!isPlaying) {
return;
}
if (map.getCount() == 0) {
Interface.showHint("还找?没了!");
return;
}
Line line = new Line(0, new Point(), new Point());
map.setTest(true);
line = map.findNext(a);
int offset = 0;
if (line.direct == 1) {
if (Setting.Sound == 1) {
new Sound(Sound.HINT);
}
offset = line.a.x * Setting.COLUMN + line.a.y;
dots[offset].setBorder(Interface.Hint);
offset = line.b.x * Setting.COLUMN + line.b.y;
dots[offset].setBorder(Interface.Hint);
score -= Setting.hintScore;
showScore(score - 1, score);
}
else {
Interface.showHint("找不到,请刷新");
}
}
/**
*
* @param a
* @param showMessage
* @return
*/
public boolean bomb(Point a, boolean showMessage) {
if (!isPlaying) {
return false;
}
if (map.getCount() == 0) {
Interface.showHint("你炸昏了头吧,没了!");
return false;
}
Line line = new Line(0, new Point(), new Point());
map.setTest(false);
line = map.findNext(a);
int offset = 0;
if (line.direct == 1) {
if (Setting.Sound == 1) {
new Sound(Sound.BOMB);
}
offset = line.a.x * Setting.COLUMN + line.a.y;
dots[offset].setBorder(Interface.unSelected);
offset = line.b.x * Setting.COLUMN + line.b.y;
dots[offset].setBorder(Interface.unSelected);
map.earse(line.a, line.b);
earse(line.a, line.b);
score -= Setting.bombScore;
showScore(score - 1, score);
return true;
}
else {
if (showMessage) {
Interface.showHint("炸弹用不了,请刷新");
}
return false;
}
}
private void message(String str) {
//System.out.println(str);
Interface.showHint(str);
}
/**
*
* @param score
*/
public void setScore(ScoreAnimate score) {
this.scoreAnimate = score;
}
/**
*
* @param clock
*/
public void setClock(ClockAnimate clock) {
this.clockAnimate = clock;
}
public void actionPerformed(ActionEvent e) {
JButton button = (JButton) e.getSource();
int offset = Integer.parseInt(button.getActionCommand());
int row, col;
row = Math.round(offset / Setting.COLUMN);
col = offset - row * Setting.COLUMN;
if (map.getMap()[row][col] < 1) {
return;
}
if (Setting.Sound == 1) {
new Sound(Sound.SELECT);
}
if (isSelected) {
message("上次已经选择了一个点");
message("上次选择点的坐标为: " + lastPoint.x + ", " + lastPoint.y +
"值为:" + map.getMap()[lastPoint.x][lastPoint.y] +
" 位移为: " + (lastPoint.x * Setting.COLUMN + lastPoint.y));
if (lastPoint.x == row && lastPoint.y == col) {
message("这次选择的点和上次的是同一点,取消选择状态̬");
button.setBorder(Interface.unSelected);
isSelected = false;
}
else {
message("这次选择的点和上次的点并不相同");
Point current = new Point(row, col);
message("这次选择的点的坐标为:" + row + ", " + col + " 值为:" +
map.getMap()[row][col] +
" 位移为:" + (row * Setting.COLUMN + col));
map.setTest(false);
if (map.test(lastPoint, current)) {
message("消除成功");
dots[row * Setting.COLUMN + col].setBorder(Interface.unSelected);
map.earse(current, lastPoint);
earse(current, lastPoint);
dots[lastPoint.x * Setting.COLUMN +
lastPoint.y].setBorder(Interface.unSelected);
lastPoint = new Point(0, 0);
isSelected = false;
showScore(score, score + Setting.correctScore + stepScore);
score += Setting.correctScore + stepScore;
if (Setting.Sound == 1) {
new Sound(Sound.EARSE);
}
}
else {
message("出错啦,重新再找和现在的点配对");
dots[lastPoint.x * Setting.COLUMN +
lastPoint.y].setBorder(Interface.unSelected);
button.setBorder(Interface.Selected);
lastPoint.x = row;
lastPoint.y = col;
isSelected = true;
score -= Setting.wrongScore;
showScore(score - 1, score);
}
}
}
else {
message("重新选择点");
message("当前点坐标为:" + row + ", " + col + "值为:" +
map.getMap()[row][col] +
"位移为:" + (row * Setting.COLUMN + col));
button.setBorder(Interface.Selected);
lastPoint.x = row;
lastPoint.y = col;
isSelected = true;
}
}
/**
*
* @param score
* @return
*/
public String encode() {
if (score < 0) {
Interface.showHint("负分啊,大哥!继续努力吧");
}
int level, f1, f2;
level = Setting.LevelIndex;
f1 = (score - 102) * (score - 102);
f2 = (1978 - score) * (1978 - score);
return "s=" + score + "&l=" + level + "&f1=" + f1 + "&f2=" + f2;
}
public void start() {
goTop10.setEnabled(false);
isPlaying = true;
limitTime = map.getCount() * Setting.limitScore;
message("欢迎使用JAVA连连看");
paint();
}
public void run() {
}
public void stop() {
clockAnimate.stop();
}
}
项目总结
开发一套系统,最重要的是细心,并不是一定要做到面面俱到,在准备工作中要正确分析社会需求了解现实应用,画出流程图,把大体框架做好,然后再逐一细化。我们不可能做到面面俱到,但一定要做到步步扎实,作为一个程序编程人员,要保持清醒的头脑,以现实为依据,让自己的每一行代码都能实现自己的意义。 通过这次课程设计,我收获的不仅仅是课程上的知识得到实际应用,还有编程的基本习惯和开发系统时应注意的流程。