今天为大家分享红绿灯游戏的开发与制作,目前是单机版游戏,后续时间空了,会进一步完善。整个系统界面漂亮,有完整得源码,希望大家可以喜欢。喜欢的帮忙点赞和关注。一起编程、一起进步
开发环境
开发语言为Java,开发环境Eclipse或者IDEA都可以。运行主程序,或者执行打开JAR文件即可以运行本程序。
系统框架
利用JDK自带的SWING框架开发,不需要安装第三方JAR包。纯窗体模式,直接运行Main文件即可以。同时带有详细得设计文档。
主要功能
本程序主要用来真实模仿路口的交通灯的运行机制,和现实版的交通灯运行原理是一样的。
启动方法
对TestFrame.java点右键,run as Application,启动红绿灯点游戏。
1 通过程序控制红灯、绿灯、黄灯这三种类型的灯交替变化
2 红灯60秒,绿灯60秒,黄灯 5秒
3 左边的面板以倒计时的方式显示当前所属于的交通灯
4 当前灯为绿灯的时候,所有车都可以畅通无阻
5 当前灯为红灯的时候,所有车不得超过斑马线。离斑马线还有一定距离的车可以形势到斑马线前面
6 当前灯为黄灯的时候,所有都可以继续前行驶
游戏运行效果
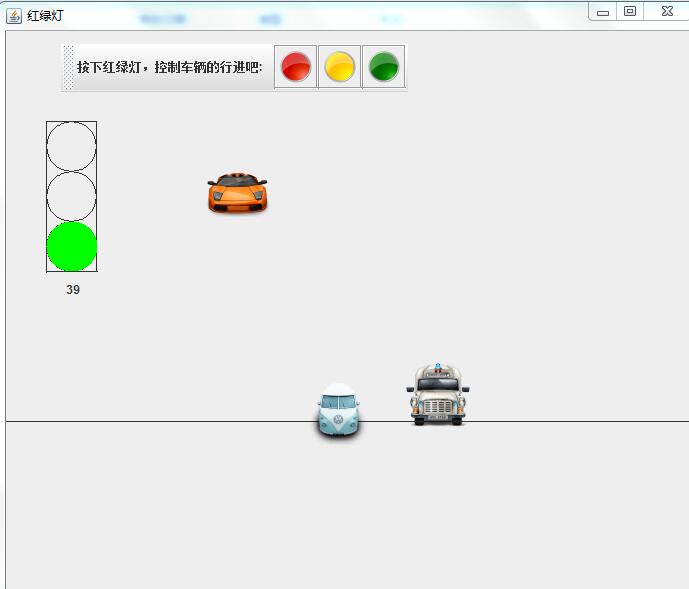
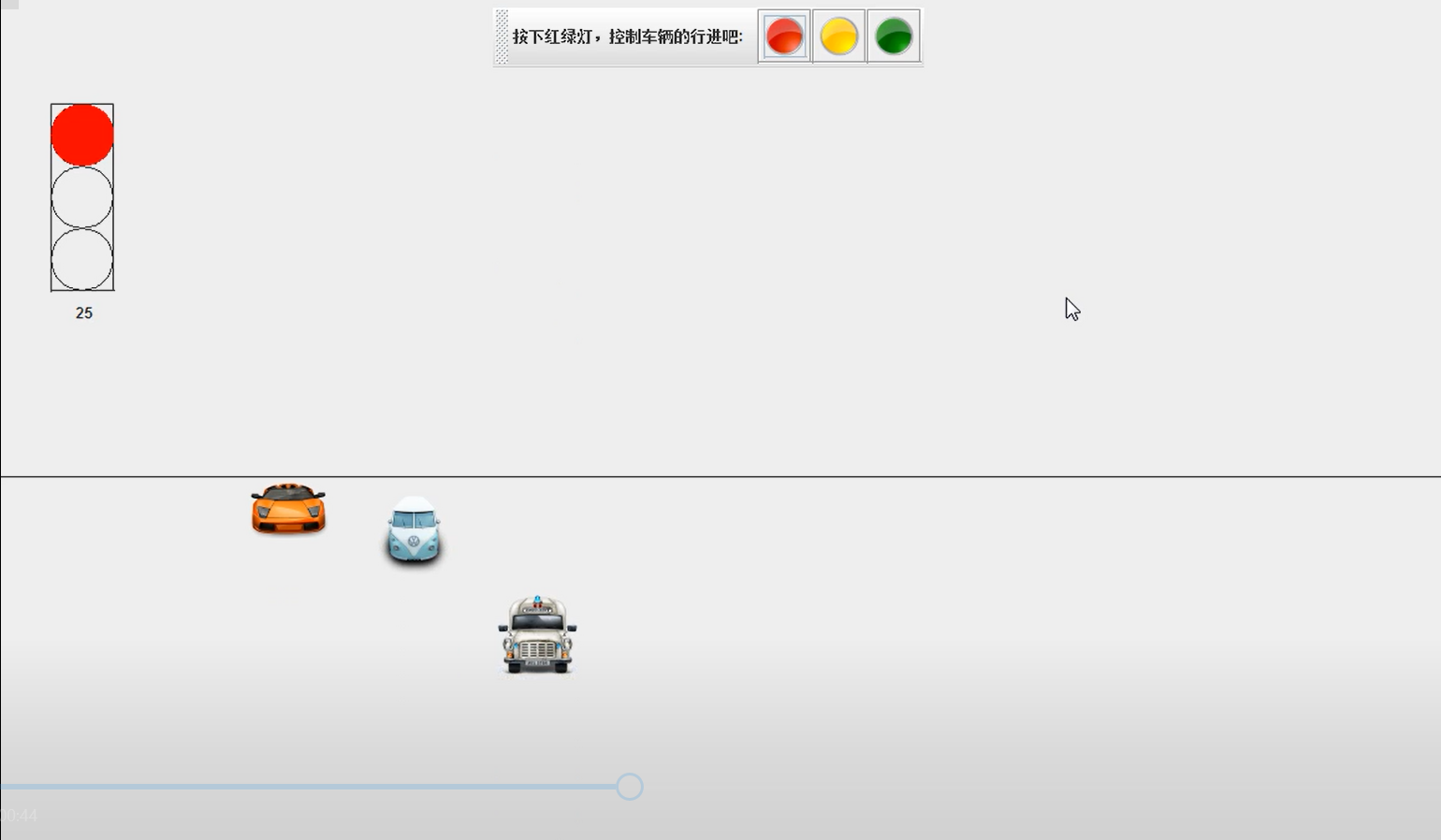
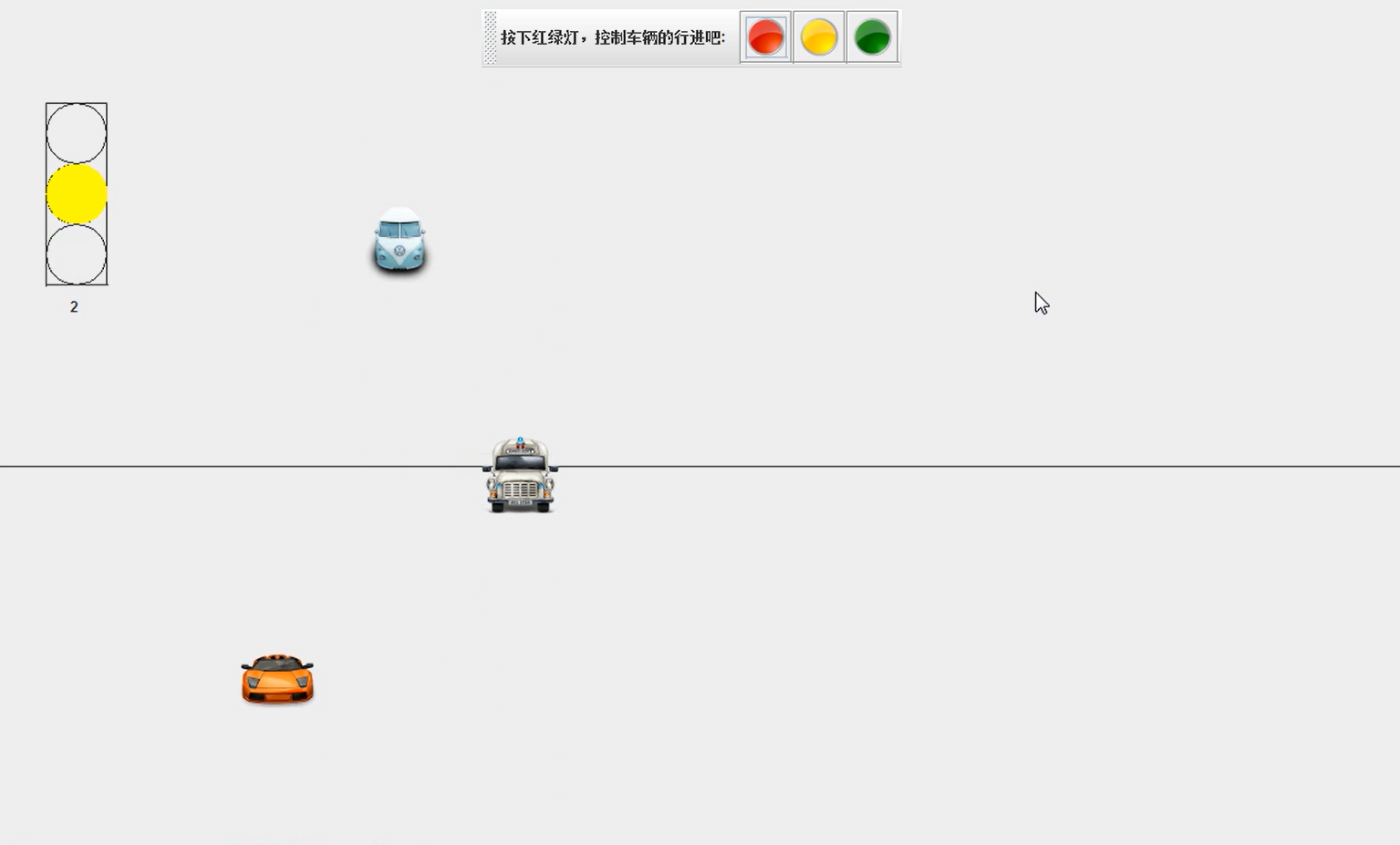
主要代码
package java2d;
import java.applet.*;
import java.awt.* ;
import java.awt.event.* ;
import javax.swing.*;
/**
* Draw sin function
* @author Args
*
*/
public class TestFrame {
static JFrame frame ;
public static void main(String[] args) {
frame = new JFrame("红绿灯") ;
final int WIDTH = 700 ;
final int HEIGHT = 600 ;
frame.setSize(WIDTH, HEIGHT) ;
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE) ;
Toolkit kit = Toolkit.getDefaultToolkit() ;
Dimension screen = kit.getScreenSize() ;
Dimension center = new Dimension((screen.width-WIDTH)/2,(screen.height-HEIGHT)/2) ;
frame.setLocation(center.width,center.height) ;
LightPane contentPane = new LightPane() ;
// contentPane.setLayout(new BorderLayout()) ;
frame.setContentPane(contentPane) ;
JToolBar toolbar = new JToolBar() ;
JButton red = new JButton(new ImageIcon("img\\icon\\red.png")) ;
JButton yellow = new JButton(new ImageIcon("img\\icon\\yellow.png"));
JButton green = new JButton(new ImageIcon("img\\icon\\green.png")) ;
red.setName("red") ;
yellow.setName("yellow") ;
green.setName("green") ;
LightActionListener lightListener = new LightActionListener() ;
red.addActionListener(lightListener) ;
yellow.addActionListener(lightListener) ;
green.addActionListener(lightListener) ;
JLabel info = new JLabel("按下红绿灯,控制车辆的行进吧: ") ;
toolbar.add(info) ;
toolbar.add(red) ;
toolbar.add(yellow) ;
toolbar.add(green) ;
contentPane.add(toolbar,"North") ;
frame.setVisible(true) ;
Thread thread = new Thread(contentPane) ;
thread.start() ;
}//end of main
}
/**
*
* 红绿灯面板
* @author Administrator
*
*/
class LightPane extends JPanel implements Runnable{
static String status = "green" ;//默认开始灯是绿色的
static int counter = 40;//红绿灯每次40秒,黄灯每次5秒
static JPanel road = new JPanel() ;
static JLabel car1 = new JLabel(new ImageIcon("img\\icon\\car1.png")) ;
static JLabel car2 = new JLabel(new ImageIcon("img\\icon\\car2.png")) ;
static JLabel car3 = new JLabel(new ImageIcon("img\\icon\\car3.png")) ;
static JLabel c = new JLabel() ;
static int car1X ;
static int car1Y ;
static int car2X ;
static int car2Y ;
static int car3X ;
static int car3Y ;
public LightPane(){
}
public void setStatus(String status){
LightPane.status = status ;
}
public void paintComponent(Graphics g){
super.paintComponent(g) ;
SwingUtilities.updateComponentTreeUI(TestFrame.frame);
g.drawRect(40, 90, 50, 150) ;//红绿灯外面的矩形
g.drawOval(40, 90, 50, 50) ;//红灯
g.drawOval(40, 140, 50, 50) ;//黄灯
g.drawOval(40, 190, 50, 50) ;//绿灯
g.drawLine(0, 390, this.getSize().width, 390) ;//停车线
if(status != null){
if(status.equals("red")){
g.setColor(Color.red) ;
g.fillOval(40, 90, 50, 50) ;//红灯涂上颜色
}else if(status.equals("yellow")){
g.setColor(Color.yellow) ;//黄灯涂上颜色
g.fillOval(40, 140, 50, 50) ;
}else if(status.equals("green")){
g.setColor(Color.green) ;
g.fillOval(40, 190, 50, 50) ;//绿灯涂上颜色
}
}
this.add(c) ;
c.setText(counter+"") ;
c.setLocation(60, 250) ;
this.add(car1) ;
this.add(car2) ;
this.add(car3) ;
car1.setLocation(car1X, car1Y) ;
car2.setLocation(car2X, car2Y) ;
car3.setLocation(car3X, car3Y) ;
}
public void run(){
car1X = 200 ;
car2X = 300 ;
car3X = 400 ;
car1Y = this.getSize().height-40 ;
car2Y = this.getSize().height-40 ;
car3Y = this.getSize().height-40 ;
while(true){
if(status.equals("yellow")){
car1Y = car1Y - 10 ;
car2Y = car2Y - 10 ;
car3Y = car3Y - 10 ;
if(counter == 1 ){
counter = 40 ;
setStatus("red") ;
}
}//黄灯减速
if(counter > 0){
counter-- ;
} else if(counter == 0){
counter = 40 ;
if(status.equals("red")){
setStatus("green") ;//红变绿
}else if(status.equals("green")){
setStatus("red") ;
}
}
if(status.equals("green") && counter ==1){
counter = 5 ;
setStatus("yellow") ;//绿变黄
}
if(status.equals("green") && counter != 1 ){
car1Y = car1Y - 10 ;
car2Y = car2Y - 20 ;
car3Y = car3Y - 30 ;
}//绿灯正常行驶
if(status.equals("red")){
if(car1Y != 386){
car1Y = car1Y - 10 ;
}else{
}
if(car2Y < 390 || (car2Y > 390 && car2Y - 20> 390)){
car2Y = car2Y - 20 ;
}else{
}
if(car3Y < 390 || (car3Y > 390 && car3Y -30 > 390)){
car3Y = car3Y - 30 ;
}else{
}
}
try {
Thread.sleep(300) ;
} catch (Exception e) {
e.printStackTrace();
}
if(car1Y <= 100){
car1Y = this.getSize().height-40 ;
continue ;
}
if(car2Y <= 100){
car2Y = this.getSize().height-40 ;
continue ;
}
if(car3Y <= 100){
car3Y = this.getSize().height-40 ;
continue ;
}//回归初始位置
}// end of while
}// end of run
}
/
class LightActionListener implements ActionListener{
public void actionPerformed(ActionEvent e){
JButton button = (JButton) e.getSource() ;
String name = button.getName() ;
LightPane light = new LightPane() ;
light.setStatus(name) ;
if(name.equals("yellow")){
light.counter = 5 ;
}else{
light.counter = 40 ;
}
light.repaint() ;
}
}