开发环境
开发语言为Java,开发环境Eclipse或者IDEA都可以。数据库采用:MySQL。运行主程序,或者执行打开JAR文件即可以运行本程序。
系统框架
利用JDK自带的SWING框架开发,需要导入MySMyQL的JDBC JAR包。MySQL数据库,纯窗体模式,直接运行Main文件即可以。同时带有详细得设计文档
系统主要功能
二手房在线租房系统 系统用Java语言编写,是一个典型的窗体程序,采用swing框架编写,系统涉及到文件、UI、数据库操作、事件等操作。通过运行Main.java,或者直接运行解压文件假下的JAR文件
程序运行方法
二手房系统系统用Java语言编写,是一个简单的窗体程序,采用swing框架编写,系统涉及到文件、UI、数据库操作、事件等操作。通过运行Main.java,或者直接运行解压文件假下的JAR文件
主要功能点
整个二手房系统采用的图形化界面方式进行设计,左边是菜单栏,右边是对应的table信息
1 系统有三种角色:系统管理员、卖家、买家。系统管理员主要主要进行基础信息的日常维护,买家主要查询房源信息。卖家主要是发布房源和查找买家信息。
2 系统管理员主要主要进行基础信息的日常维护,主要的功能包括:修改密码、公告管理、用户管理
二手房管理、交易管理
3 买家:查询房源信息,查看订单进度、修改密码
4 卖家:发布房源信息、查询房源、查询买家信息。
5 房源信息包含以下一些字段:房源编号、卖家注册号、房源名称、所属地址、建筑单元
朝向、户型、面积、楼层、单元号、车库面积、装修状况、物业管理费、房源状态、房屋售价。
实现效果
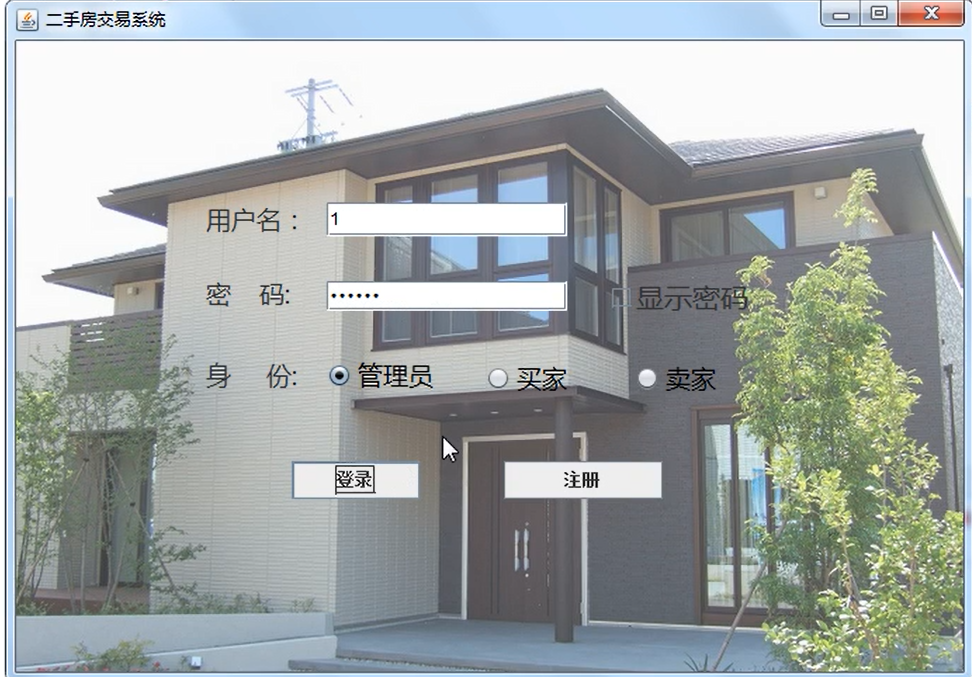
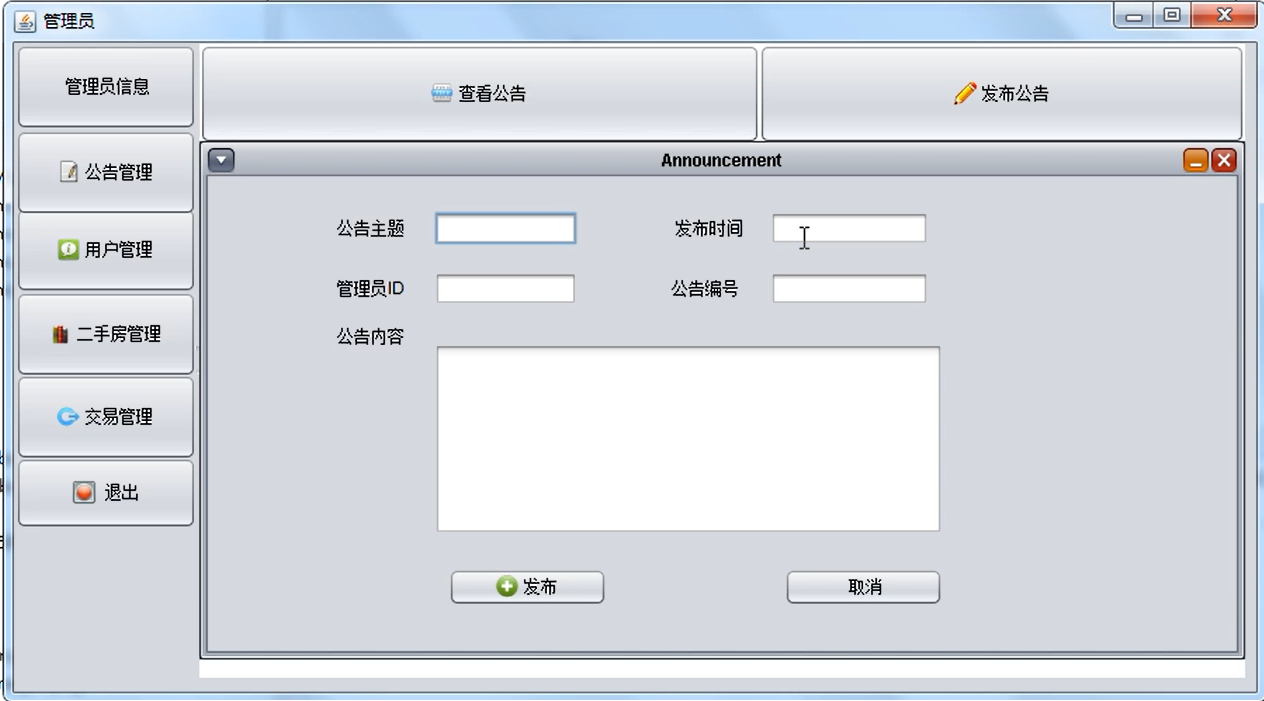
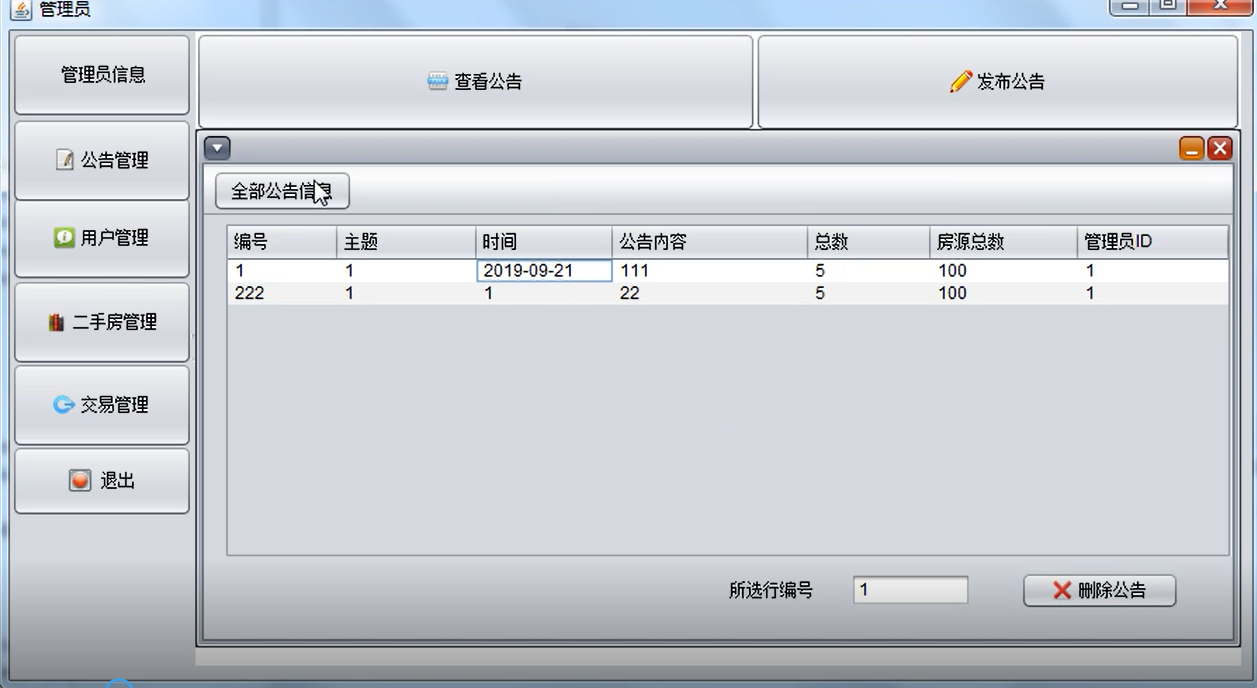
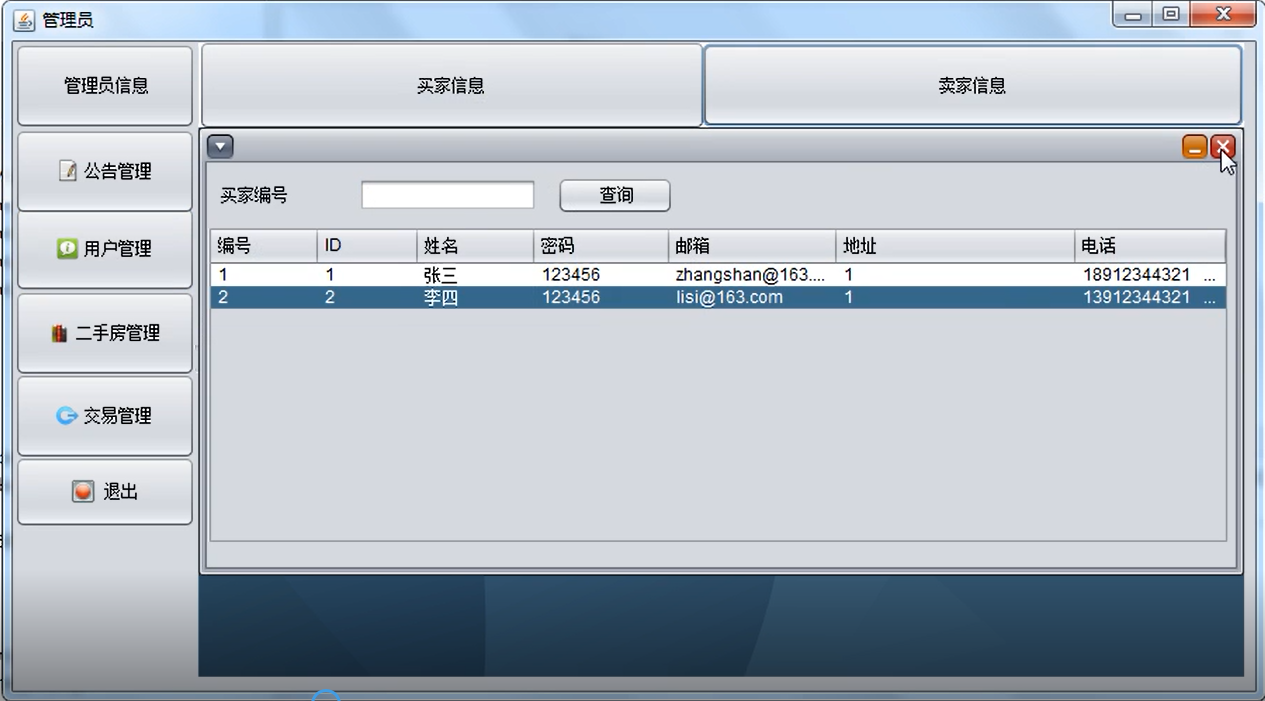
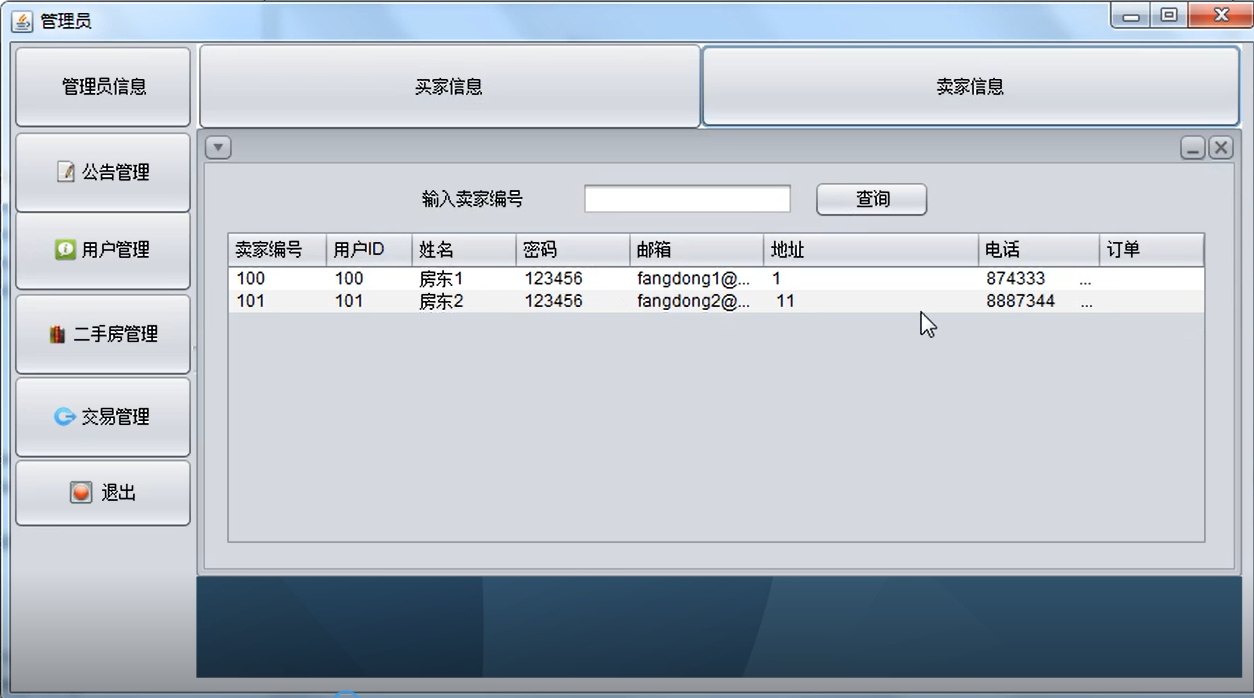
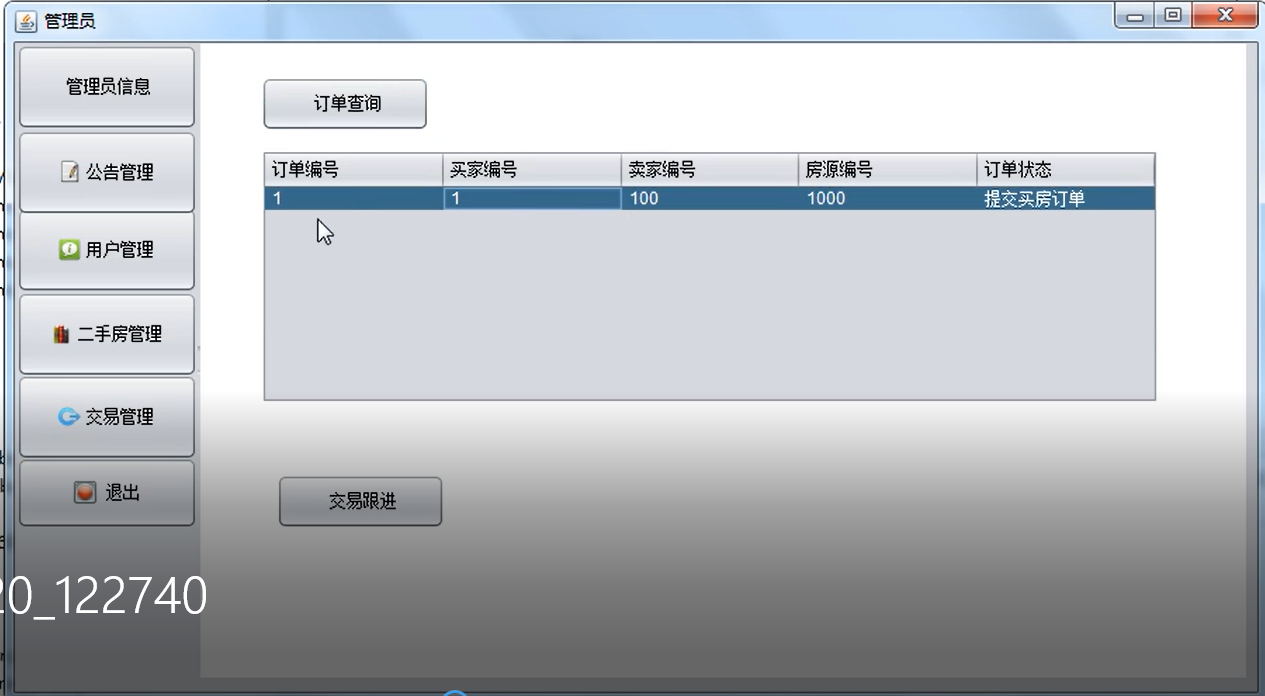
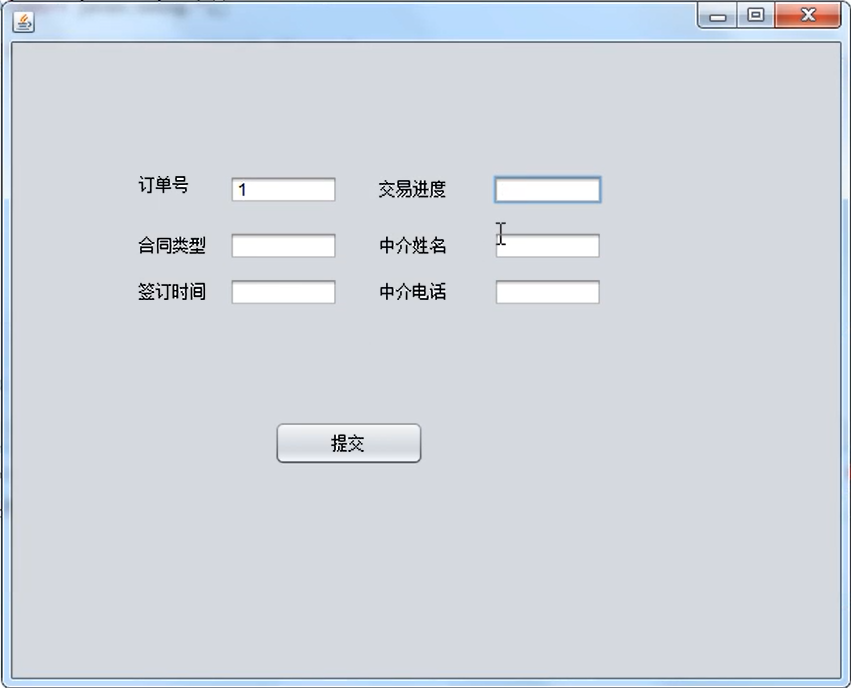
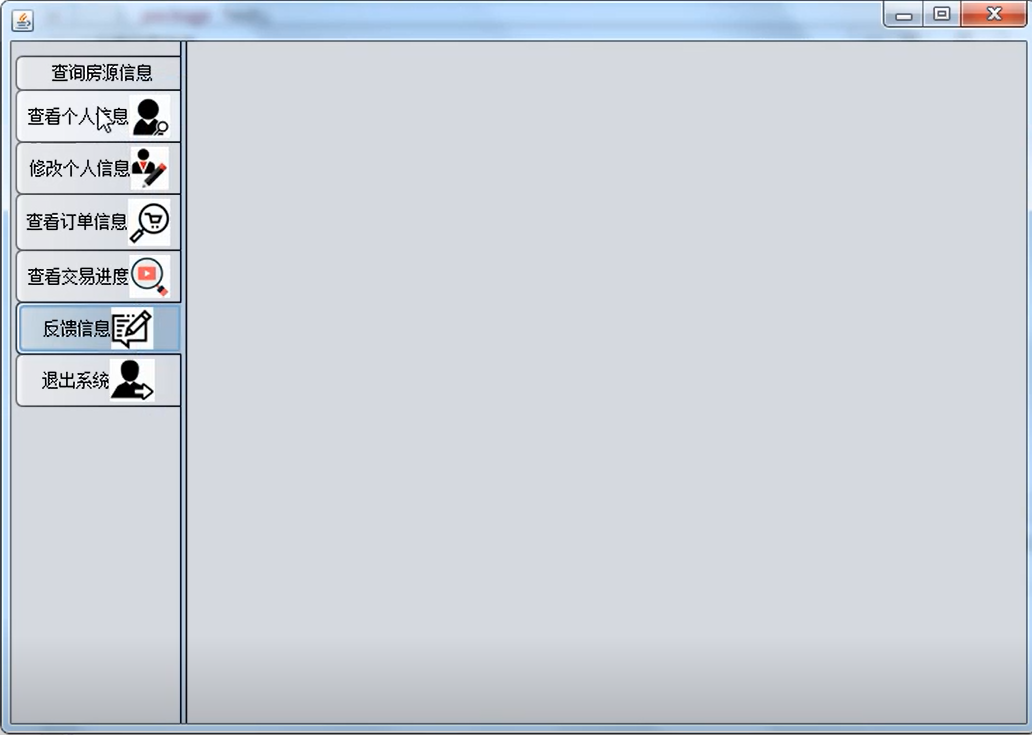
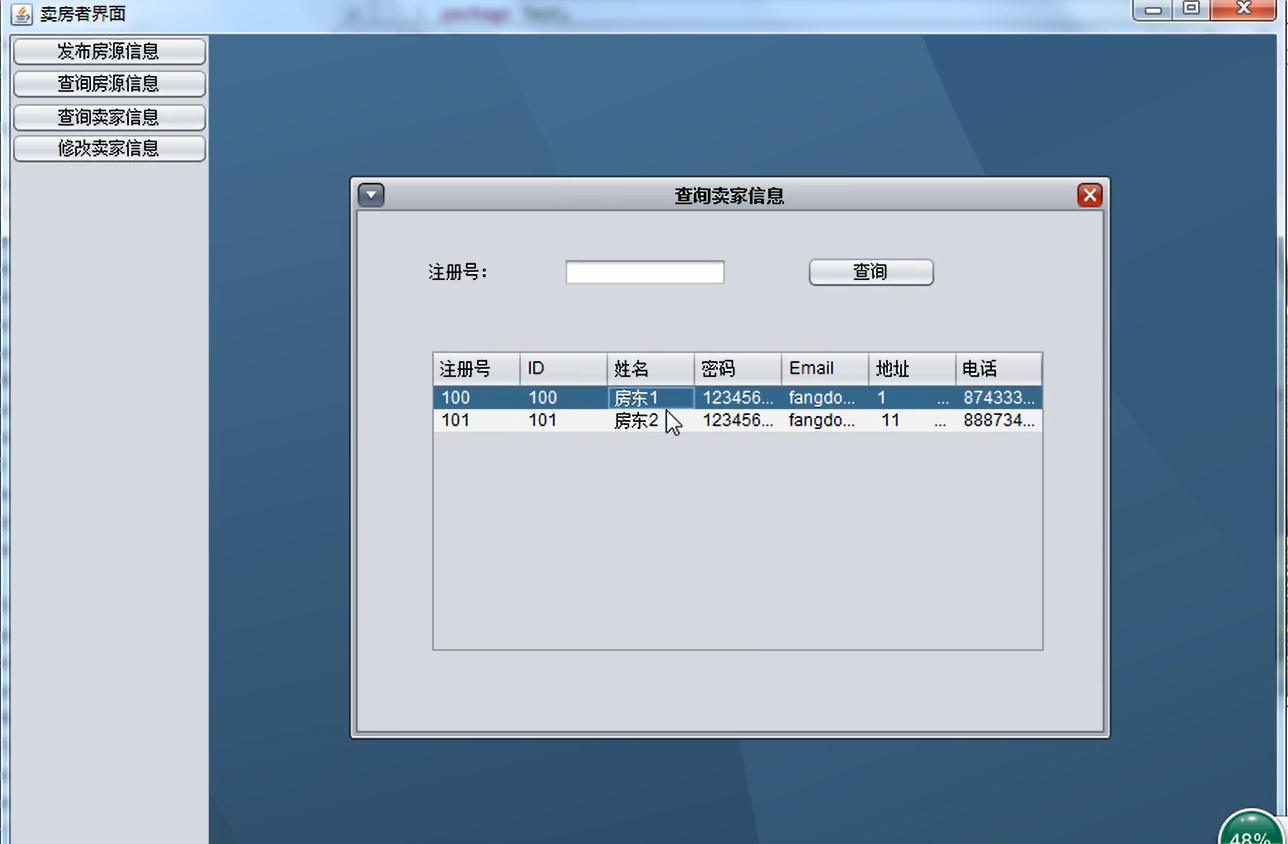
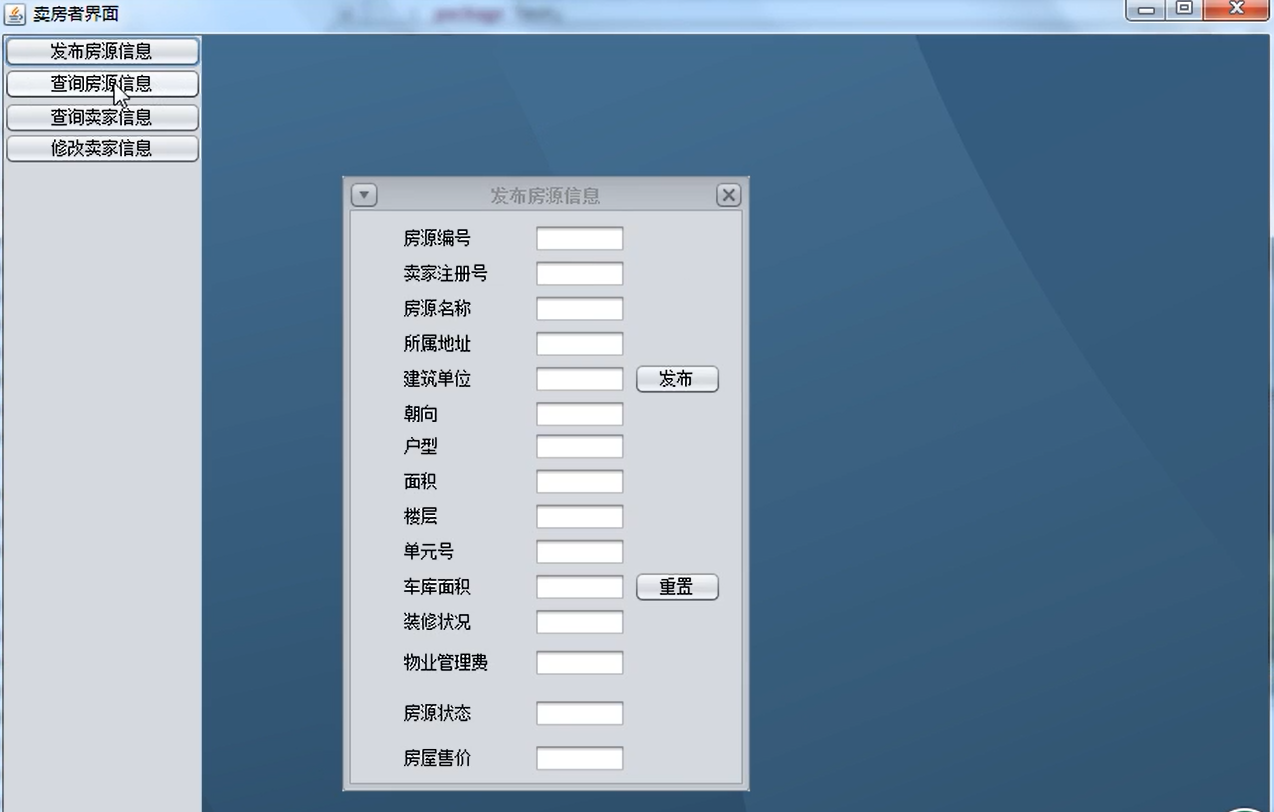
关键代码
package com.system.view;
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
import javax.swing.JDesktopPane;
import java.awt.Window.Type;
import javax.swing.border.EtchedBorder;
public class SellerFrm extends JFrame {
private JPanel contentPane;
private JDesktopPane desktopPane;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
SellerFrm frame = new SellerFrm();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public SellerFrm() {
setType(Type.POPUP);
setTitle("\u5356\u623F\u8005\u754C\u9762");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 916, 618);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(null);
JButton button = new JButton("\u53D1\u5E03\u623F\u6E90\u4FE1\u606F");
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
HouseinfoInterFrm houseinfoInterFrm=new HouseinfoInterFrm();
houseinfoInterFrm.setVisible(true);
desktopPane.add(houseinfoInterFrm);
}
});
button.setBounds(0, 0, 141, 23);
contentPane.add(button);
JButton btnNewButton = new JButton("\u67E5\u8BE2\u623F\u6E90\u4FE1\u606F");
btnNewButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
SearchInterFrm searchInterFrm=new SearchInterFrm();
searchInterFrm.setVisible(true);
desktopPane.add(searchInterFrm);
}
});
btnNewButton.setBounds(0, 23, 141, 23);
contentPane.add(btnNewButton);
JButton button_1 = new JButton("\u67E5\u8BE2\u5356\u5BB6\u4FE1\u606F");
button_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
SearchSellerInterFrm searchSellerInterFrm=new SearchSellerInterFrm();
searchSellerInterFrm.setVisible(true);
desktopPane.add(searchSellerInterFrm);
}
});
button_1.setBounds(0, 47, 141, 23);
contentPane.add(button_1);
JButton btnNewButton_1 = new JButton("\u4FEE\u6539\u5356\u5BB6\u4FE1\u606F");
btnNewButton_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
AlterInterFrm alterInterFrm=new AlterInterFrm();
alterInterFrm.setVisible(true);
desktopPane.add(alterInterFrm);
}
});
btnNewButton_1.setBounds(0, 69, 141, 23);
contentPane.add(btnNewButton_1);
desktopPane = new JDesktopPane();
desktopPane.setBounds(141, 0, 1500, 900);
contentPane.add(desktopPane);
}
}
package com.system.util;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DbUtil {
String dbUrl="jdbc:sqlserver://127.0.0.1:1433;DatabaseName=Second_handHouse";//数据库地址
String userName="sa";
String userPwd="124421";
String jdbcName="com.microsoft.sqlserver.jdbc.SQLServerDriver";//驱动
public Connection getCon() throws Exception {
Class.forName(jdbcName);
Connection con=DriverManager.getConnection(dbUrl, userName, userPwd);
return con;
}
public void closeCon(Connection con) throws Exception {
if(con!=null) {
con.close();
}
}
public static void main(String[] args) {
DbUtil dbUtil=new DbUtil();
try {
dbUtil.getCon();
System.out.println("数据库连接成功");
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}