1函数
1.1向邻接表中插入一个数据,并保证邻接表的有序性
void insertIntoAdjVertics(struct vNode** adjVertics, int start, int end)//向邻接表中插入一个数据,并保证邻接表的有序性
{
struct vNode* node = (struct vNode*)malloc(sizeof(struct vNode));
struct vNode* head = adjVertics[start];
node->value = end;
node->next = NULL;
if (head == NULL) {
adjVertics[start] = node;
return;
}
if (head->next == NULL && head->value > end) {
node->next = head;
adjVertics[start] = node;
return;
}
while (head->next != NULL && head->next->value < end) {
head = head->next;
}
if (head->next == NULL) {
head->next = node;
return;
}
node->next = head->next;
head->next = node;
}
1.2输出邻接矩阵
void displayedges(MGraph g, int n) //打印邻接矩阵
{
int i, j;
printf("\n");
for (i = 1; i <= n; i++) {
for (j = 1; j <= n; j++) {
printf("%d ", g.edges[i][j]);
}
printf("\n");
}
}
1.3输出邻接表
void displayAdjVertice(struct vNode** adjVertics, int n) //打印邻接表
{
int i;
for (i = 1; i <= n; i++) {
struct vNode* head = adjVertics[i];
printf("%d: ", i);
while (head != NULL) {
printf("->%d ", head->value);
head = head->next;
}
printf("\n");
}
}
1.4主函数
int main() {
MGraph g;
int n, e, i, j;
int start, end;
printf("input vertex and edge number: ");
scanf_s("%d%d", &n, &e);
for (i = 1; i <= n; i++) { //初始化邻接矩阵
for (j = 1; j <= n; j++) {
g.edges[i][j] = 0;
}
}
struct vNode** adjVertics;
adjVertics = (struct vNode**)malloc(sizeof(struct vNode*) * n);
for (i = 1; i <= n; i++) {
adjVertics[i] = NULL;
}
i = 0;
//输入定点,格式为 a b
printf("input start and end vertex, format a b \n");
while (1) {
scanf_s("%d", &start);
scanf_s("%d", &end);
g.edges[start][end] = 1; //对称矩阵
g.edges[end][start] = 1;
insertIntoAdjVertics(adjVertics, start, end);
insertIntoAdjVertics(adjVertics, end, start);
i++;
if (i == e) {
break;
}
}
displayedges(g, n);
printf("-------------\n-------------\n-------------\n");
displayAdjVertice(adjVertics, n);
system("pause");
return EXIT_SUCCESS;
}
2可执行代码
#define MAXV 20
#include <stdio.h>
#include <stdlib.h>
#include <iostream>
using namespace std;
//图的邻接矩阵
typedef struct //图的定义
{
int edges[MAXV][MAXV]; //邻接矩阵
int n, e; //顶点数,弧数
} MGraph; //图的邻接矩阵表示类型
//邻接表中的链表节点
struct vNode {
int value;
struct vNode* next;
};
void insertIntoAdjVertics(struct vNode** adjVertics, int start, int end);//向邻接表中插入一个数据,并保证邻接表的有序性
void displayedges(MGraph g, int n);//打印邻接矩阵
void displayAdjVertice(struct vNode** adjVertics, int n);//打印邻接表
int main() {
MGraph g;
int n, e, i, j;
int start, end;
printf("input vertex and edge number: ");
scanf_s("%d%d", &n, &e);
for (i = 1; i <= n; i++) { //初始化邻接矩阵
for (j = 1; j <= n; j++) {
g.edges[i][j] = 0;
}
}
struct vNode** adjVertics;
adjVertics = (struct vNode**)malloc(sizeof(struct vNode*) * n);
for (i = 1; i <= n; i++) {
adjVertics[i] = NULL;
}
i = 0;
//输入定点,格式为 a b
printf("input start and end vertex, format a b \n");
while (1) {
scanf_s("%d", &start);
scanf_s("%d", &end);
g.edges[start][end] = 1; //对称矩阵
g.edges[end][start] = 1;
insertIntoAdjVertics(adjVertics, start, end);
insertIntoAdjVertics(adjVertics, end, start);
i++;
if (i == e) {
break;
}
}
displayedges(g, n);
printf("-------------\n-------------\n-------------\n");
displayAdjVertice(adjVertics, n);
system("pause");
return EXIT_SUCCESS;
}
void insertIntoAdjVertics(struct vNode** adjVertics, int start, int end)//向邻接表中插入一个数据,并保证邻接表的有序性
{
struct vNode* node = (struct vNode*)malloc(sizeof(struct vNode));
struct vNode* head = adjVertics[start];
node->value = end;
node->next = NULL;
if (head == NULL) {
adjVertics[start] = node;
return;
}
if (head->next == NULL && head->value > end) {
node->next = head;
adjVertics[start] = node;
return;
}
while (head->next != NULL && head->next->value < end) {
head = head->next;
}
if (head->next == NULL) {
head->next = node;
return;
}
node->next = head->next;
head->next = node;
}
void displayedges(MGraph g, int n) //打印邻接矩阵
{
int i, j;
printf("\n");
for (i = 1; i <= n; i++) {
for (j = 1; j <= n; j++) {
printf("%d ", g.edges[i][j]);
}
printf("\n");
}
}
void displayAdjVertice(struct vNode** adjVertics, int n) //打印邻接表
{
int i;
for (i = 1; i <= n; i++) {
struct vNode* head = adjVertics[i];
printf("%d: ", i);
while (head != NULL) {
printf("->%d ", head->value);
head = head->next;
}
printf("\n");
}
}
3运行结果展示
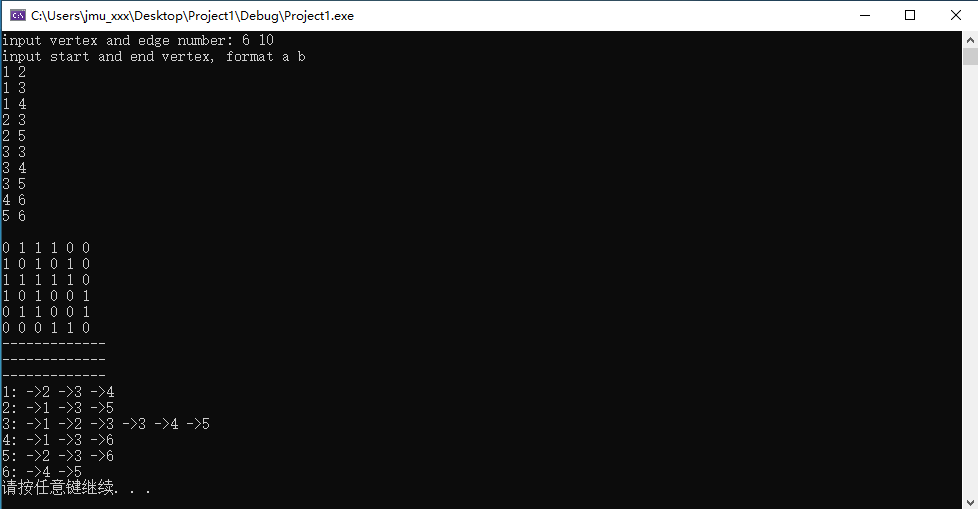