在WebService服务对接中,经常遇到这样的情况,对方服务的wsdl文件以及接口文档已经拿到,但由于这样或那样的原因导致网络一直不通,并且由于项目比较急,为方便边开发边调试,一般解决方法是在己方测试环境部署一个对方的模拟服务。
现有WSDL文件如下:
<?xml version="1.0" encoding="UTF-8" ?>
<wsdl:definitions xmlns:xsd="http://www.w3.org/2001/XMLSchema"
xmlns:wsdl="http://schemas.xmlsoap.org/wsdl/" xmlns:tns="http://www.lzg.test.com"
xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/" xmlns:ns1="http://schemas.xmlsoap.org/soap/http"
name="lzgWebService" targetNamespace="http://www.lzg.test.com">
<wsdl:types>
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema"
xmlns:tns="http://www.lzg.test.com" elementFormDefault="unqualified"
targetNamespace="http://www.lzg.test.com" version="1.0">
<xs:element name="doService" type="tns:doService" />
<xs:element name="doServiceResponse" type="tns:doServiceResponse" />
<xs:complexType name="doService">
<xs:sequence>
<xs:element minOccurs="0" name="serviceCode" type="xs:string" />
<xs:element minOccurs="0" name="reqXml" type="xs:string" />
</xs:sequence>
</xs:complexType>
<xs:complexType name="doServiceResponse">
<xs:sequence>
<xs:element minOccurs="0" name="return" type="xs:string" />
</xs:sequence>
</xs:complexType>
</xs:schema>
</wsdl:types>
<wsdl:message name="doServiceResponse">
<wsdl:part element="tns:doServiceResponse" name="parameters" />
</wsdl:message>
<wsdl:message name="doService">
<wsdl:part element="tns:doService" name="parameters" />
</wsdl:message>
<wsdl:portType name="ITestWebService">
<wsdl:operation name="doService">
<wsdl:input message="tns:doService" name="doService" />
<wsdl:output message="tns:doServiceResponse" name="doServiceResponse" />
</wsdl:operation>
</wsdl:portType>
<wsdl:binding name="testWebServiceSoapBinding" type="tns:ITestWebService">
<soap:binding style="document"
transport="http://schemas.xmlsoap.org/soap/http" />
<wsdl:operation name="doService">
<soap:operation soapAction="" style="document" />
<wsdl:input name="doService">
<soap:body use="literal" />
</wsdl:input>
<wsdl:output name="doServiceResponse">
<soap:body use="literal" />
</wsdl:output>
</wsdl:operation>
</wsdl:binding>
<wsdl:service name="testWebService">
<wsdl:port binding="tns:testWebServiceSoapBinding" name="TestWebServicePort">
<soap:address
location="https://192.168.4.188:8008/lzg/soap/testWebService" />
</wsdl:port>
</wsdl:service>
</wsdl:definitions>
以下已SpringBoot项目为例,一般有两种方法:
一:通过配置DefaultWsdl11Definition实现
1:配置Maven pom文件
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.lzg.test.ws</groupId>
<artifactId>SpringBootWSDemo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<java.version>1.8</java.version>
</properties>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.7.RELEASE</version>
<relativePath></relativePath>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<!-- 去掉springboot中内嵌的tomcat模块 -->
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
<!-- 在这里将scope属性设置为provided,这样在最终形成的WAR中不会包含这个JAR包 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
<scope>provided</scope>
</dependency>
<!--spring web Service的包 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web-services</artifactId>
</dependency>
<!--spring web service wsdl包 -->
<dependency>
<groupId>wsdl4j</groupId>
<artifactId>wsdl4j</artifactId>
</dependency>
<dependency>
<groupId>jaxen</groupId>
<artifactId>jaxen</artifactId>
</dependency>
<dependency>
<groupId>org.jdom</groupId>
<artifactId>jdom2</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<configuration>
<warName>ws</warName>
</configuration>
</plugin>
</plugins>
</build>
</project>
2:由WSDL文件写出其定义XSD文件
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema"
xmlns:tns="http://www.lzg.test.com" targetNamespace="http://www.lzg.test.com"
elementFormDefault="unqualified">
<xs:element name="doService">
<xs:complexType>
<xs:sequence>
<xs:element minOccurs="0" name="serviceCode" type="xs:string" />
<xs:element minOccurs="0" name="reqXml" type="xs:string" />
</xs:sequence>
</xs:complexType>
</xs:element>
<xs:element name="doServiceResponse">
<xs:complexType>
<xs:sequence>
<xs:element minOccurs="0" name="return" type="xs:string" />
</xs:sequence>
</xs:complexType>
</xs:element>
</xs:schema>
3:有XSD文件生成实体
右击XSD文件,依次如图:
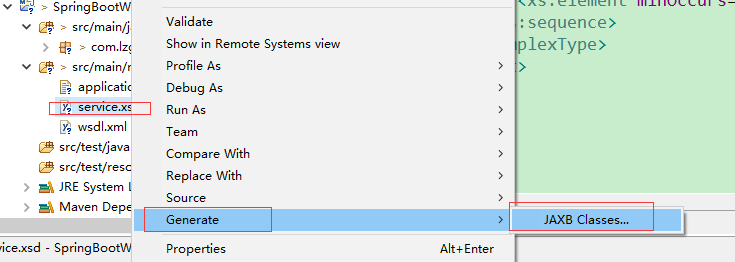
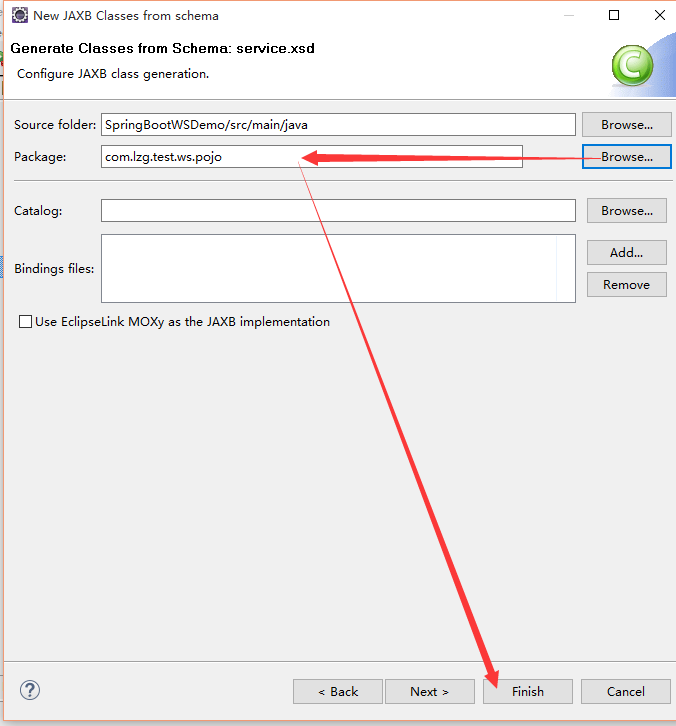
单击Yes:
查看,生成成功:
4:编写配置文件
配置WebServiceConfig让其继承WsConfigurerAdapter,依次配置消息分发Servlet MessageDispatcherServlet,默认WSDL定义类DefaultWsdl11Definition,加载XSD文件的Bean。
5:测试文件
编写模拟服务的接收消息后的处理逻辑LzgRepository。
6: Endpoint文件
编写WebService服务的调用入口LzgEndpoint。
7:SpringBoot启动文件
配置application.properties和Application.java8:比对WSDL文件
启动服务,在浏览器输入:http://localhost:8008/lzg/soap/testWebService.wsdl
查看文件和原WSDL文件比对:
发现丢失
<wsdl:message name="doService">
<wsdl:part element="tns:doService" name="parameters" />
</wsdl:message>
wsdl:portType和wsdl:binding节点也不一致。
9:测试
通过Postman调用:
URL:http://localhost:8008/lzg/soap/testWebService
参数:raw xml(text/xml)
请求报文:
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns:lzg="http://www.lzg.test.com">
<soapenv:Header/>
<soapenv:Body>
<lzg:doService>
<serviceCode>1111111</serviceCode><reqXml>22222222222</reqXml>
</lzg:doService>
</soapenv:Body>
</soapenv:Envelope>
结果:
测试可以通过。
二:通过配置SimpleWsdl11Definition实现
1:编写配置
把上边第4步中的配置的默认WSDL定义类DefaultWsdl11Definition的Bean和加载XSD文件的Bean的代码注释掉,重写一个SimpleWsdl11Definition Bean,在其内直接加载WSDL文件。
2:启动服务,比对WSDL文件
发现WSDL完全一致。
3:测试
测试可以通过。