/**************************************************************************************
* Function : 链表学习
* Create Date : 2014/05/15
* Author : NTSK13
* Email : beijiwei@qq.com
* Copyright : 欢迎大家和我一起交流学习,转载请保持源文件的完整性。
* 任何单位和个人不经本人允许不得用于商业用途
* Version : V0.1
***************************************************************************************
简单数据结构之链表(有头节点)
**************************************************************************************/
#include<stdio.h>
#include<stdlib.h>
#include<malloc.h>
typedef struct node //建立节点 数据结构
{
int data;
struct node *next;
}Lnode;
void link_init(Lnode **ph)//链表初始化
{
*ph=(Lnode *)malloc( sizeof(Lnode) );
if(NULL==*ph)
{
printf("malloc error !!!");
exit(0);
}
(*ph)->next=NULL;
}
int link_get_length(Lnode *ph)
{
int len=0;
Lnode *ptmp=ph;
if(ptmp->next==NULL)
return (0);
while(ptmp->next)
{
len++;
ptmp=ptmp->next;
}
return (len);
}
int link_search_element(Lnode ** ph,int val)//查找链表元素
{
Lnode *ptmp=(*ph)->next;
while( ptmp)
{
if(ptmp->data == val )
return (1);
ptmp=ptmp->next;
}
return (0);
}
void insert_head_list(Lnode ** ph,int val)//插入链表元素 插头
{
Lnode *node=NULL;
node=(Lnode *)malloc( sizeof(Lnode) );
if(node==NULL)
{
printf("Allocate memory fail !!! \n");
fflush(stdout);
}
node->data=val;
node->next=(*ph)->next;
(*ph)->next=node;
}
void insert_tail_list(Lnode **ph,int val)//插入链表元素 插尾
{
Lnode *node=NULL;
Lnode *ptmp=*ph;
node=(Lnode *)malloc( sizeof(Lnode) );
if(node==NULL)
{
printf("Allocate memory fail !!! \n");
fflush(stdout);
}
node->data=val;
node->next=NULL;
if( (*ph)->next == NULL)
{
(*ph)->next=node;
}else
{
while( (*ph)->next!=NULL)
*ph=(*ph)->next;
(*ph)->next=node;
*ph=ptmp;
}
}
void delete_nth_list(Lnode **ph,int n)//删除链表第n元素 n= 0,1,2,...
{
int i=0;
Lnode *pre=NULL;
Lnode *post=NULL;
pre=post=(*ph)->next;
if(n==0)
{
pre=(*ph)->next;
(*ph)->next=(*ph)->next->next;
free(pre);
}else
{
while(i != n)
{
pre=post;
post=post->next;
i++;
}
pre->next=post->next;
free(post);
}
}
void traverse_list(Lnode *ph)//遍历链表
{
int len=link_get_length(ph);//获取链表长度
int i=0;
Lnode *ptmp=ph->next;
for(i=0;i<len;i++)
{
printf("%d \n",ptmp->data);
fflush(stdout);
ptmp=ptmp->next;
}
}
void traverse_list2(Lnode *ph)//遍历链表
{
Lnode *ptmp=ph->next;
while(ptmp)
{
printf("%d \n",ptmp->data);
fflush(stdout);
ptmp=ptmp->next;
}
}
void destroy_list(Lnode **ph)
{
Lnode *ptmp=(*ph)->next;
Lnode *p=NULL;
while( ptmp )
{
p=ptmp;
ptmp=ptmp->next;
free(p);
}
}
int main()
{
Lnode * phead=NULL;//定义头指针
int array[5]={0,1,2,3,4};
int i=0;
link_init(&phead);//链表初始化
for(i=0;i<5;i++)
{
insert_tail_list(&phead,array[i]);
}
traverse_list(phead);
delete_nth_list(&phead,2);//删除链表第2个元素 0,1,2,...
printf("\n\n");
traverse_list(phead);
printf("\n\n");
for(i=0;i<5;i++)
{
insert_head_list(&phead,array[i]);
}
traverse_list2(phead);
printf("\n\n");
printf("%d \n",link_search_element(&phead,2) );//查找链表元素
destroy_list(&phead);
return (0);
}
简单数据结构之链表(有头节点)
最新推荐文章于 2023-01-23 23:22:36 发布
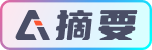