import java.io.BufferedReader;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.net.HttpURLConnection;
import java.net.URL;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
public class DateUtil {
private final static SimpleDateFormat shortSdf = new SimpleDateFormat("yyyy-MM-dd");
private final static SimpleDateFormat longSdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.SSS");
/**
* 日期+1
* @param specifiedDay
* @return
*/
public static String getNowDate(String specifiedDay) {
Calendar c = Calendar.getInstance();
Date date = null;
try {
date = new SimpleDateFormat("yyyyMMdd").parse(specifiedDay);
} catch (ParseException e) {
e.printStackTrace();
}
c.setTime(date);
int day = c.get(Calendar.DATE);
c.set(Calendar.DATE, day + 1);
String dayBefore = new SimpleDateFormat("yyyyMMdd").format(c.getTime());
return dayBefore;
}
/**
* 当前时间是星期几
*
* @return
*/
public static int getWeekDay(String specifiedDay) {
Date date = null;
try {
date = new SimpleDateFormat("yyyyMMdd").parse(specifiedDay);
} catch (ParseException e) {
e.printStackTrace();
}
Calendar c = Calendar.getInstance();
c.setTime(date);
int week_of_year = c.get(Calendar.DAY_OF_WEEK);
week_of_year = week_of_year - 1;
if (week_of_year == 0) {
week_of_year = 7;
}
return week_of_year;
}
/**
* 当年的第几周
* @param specifiedDay
* @return
*/
public static int getWeekofYear(String specifiedDay) {
Calendar c = Calendar.getInstance();
Date date = null;
try {
date = new SimpleDateFormat("yyyyMMdd").parse(specifiedDay);
} catch (ParseException e) {
e.printStackTrace();
}
c.setTime(date);
c.setFirstDayOfWeek(Calendar.MONDAY);
int week = c.get(Calendar.WEEK_OF_YEAR);
return week;
}
/**
* 属于本年第几周
*
* @return
*/
public static int getYearWeekIndex(String specifiedDay) {
Date date = null;
try {
date = new SimpleDateFormat("yyyyMMdd").parse(specifiedDay);
} catch (ParseException e) {
e.printStackTrace();
}
Calendar calendar = Calendar.getInstance();
calendar.setFirstDayOfWeek(Calendar.MONDAY);
calendar.setTime(date);
return calendar.get(Calendar.WEEK_OF_YEAR);
}
/**
* 获取yyyymmdd
* @param start_day
* @return
* @throws ParseException
*/
public static String yyyymmdd(String start_day,String patten) throws ParseException{
Date date= new SimpleDateFormat("yyyyMMdd").parse(start_day);
return new SimpleDateFormat(patten).format(date);
}
/**
* 获取月多少天
*
* @param date
* @return
*/
public static int getMDaycnt(String start_day) {
Date date = null;
try {
date = new SimpleDateFormat("yyyyMMdd").parse(start_day);
} catch (ParseException e) {
e.printStackTrace();
}
Calendar c = Calendar.getInstance();
c.setTime(date);
int i = c.get(Calendar.DAY_OF_MONTH);
return i;
}
/**
* 获取月旬 三旬: 上旬1-10日 中旬11-20日 下旬21-31日
*
* @param date
* @return
*/
public static int getTenDay(String start_day) {
Date date = null;
try {
date = new SimpleDateFormat("yyyyMMdd").parse(start_day);
} catch (ParseException e) {
e.printStackTrace();
}
Calendar c = Calendar.getInstance();
c.setTime(date);
int i = c.get(Calendar.DAY_OF_MONTH);
if (i < 11)
return 1;
else if (i < 21)
return 2;
else
return 3;
}
/**
* 获取月旬 三旬: 上旬1-10日 中旬11-20日 下旬21-31日
*
* @param date
* @return
*/
public static String getTenDays(String start_day) {
Date date = null;
try {
date = new SimpleDateFormat("yyyyMMdd").parse(start_day);
} catch (ParseException e) {
e.printStackTrace();
}
Calendar c = Calendar.getInstance();
c.setTime(date);
int i = c.get(Calendar.DAY_OF_MONTH);
if (i < 11)
return "上旬";
else if (i < 21)
return "中旬";
else
return "下旬";
}
/**
* 获取月旬 三旬: 每旬多少天
*
* @param date
* @return
*/
public static int getTenDayscnt(String start_day) {
Date date = null;
try {
date = new SimpleDateFormat("yyyyMMdd").parse(start_day);
} catch (ParseException e) {
e.printStackTrace();
}
Calendar c = Calendar.getInstance();
c.setTime(date);
int i = c.get(Calendar.DAY_OF_MONTH);
if (i < 11){
return 10;
} else if (i < 21) {
return 10;
}else{
return Integer.valueOf(getMonthEndTime(start_day).substring(6,8))-20;
}
}
/**
* 当前时间季度
*
* @return
*/
public static int getQuarter(String start_day) {
Date date = null;
try {
date = new SimpleDateFormat("yyyyMMdd").parse(start_day);
} catch (ParseException e) {
e.printStackTrace();
}
Calendar c = Calendar.getInstance();
c.setTime(date);
int currentMonth = c.get(Calendar.MONTH) + 1;
int dt = 0;
try {
if (currentMonth >= 1 && currentMonth <= 3)
dt=1;
else if (currentMonth >= 4 && currentMonth <= 6)
dt=2;
else if (currentMonth >= 7 && currentMonth <= 9)
dt=3;
else if (currentMonth >= 10 && currentMonth <= 12)
dt=4;
} catch (Exception e) {
e.printStackTrace();
}
return dt;
}
/**
* 当前时间季度天数
*
* @return
*/
public static int getQuarterCntday(String start_day) {
int cnt_d=0;
int q= getQuarter(start_day);
if (q==1) {
start_day = start_day.substring(0,4);
start_day = getMonthEndTime(start_day+"0201");
cnt_d = getMDaycnt(start_day);
cnt_d=31+cnt_d+31;
}else if (q == 2) {
cnt_d=30+31+30;
}else if (q == 3) {
cnt_d=31+31+30;
}else if (q =
java生成日期维度表
最新推荐文章于 2023-03-11 23:19:44 发布
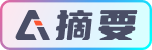