Maven依赖
<dependency>
<groupId>com.deepoove</groupId>
<artifactId>poi-tl</artifactId>
<version>1.9.0</version>
</dependency>
页面
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Excel下载</title>
</head>
<body>
<button id="downloadBtn">Excel下载</button>
<script>
function downloadFile(blob, fileName) {
const link = document.createElement('a');
link.href = URL.createObjectURL(blob);
link.download = fileName;
document.body.appendChild(link);
link.click();
document.body.removeChild(link);
URL.revokeObjectURL(link.href);
}
const downloadBtn = document.getElementById('downloadBtn');
downloadBtn.addEventListener('click', function () {
//请求路径
fetch('/download/excel')
.then(response => response.blob())
.then(blob => {
//文件名称和文件后缀
downloadFile(blob, 'people.xlsx');
});
});
</script>
</body>
</html>
Controller
@RestController
public class IndexController {
@Autowired
ExcelService excelService;
@RequestMapping("/download/excel")
public void downloadExcel(HttpServletResponse response) throws IOException {
//查询数据
List<People> peopleList = excelService.getPeoples();
//输出流
ByteArrayOutputStream outputStream = null;
//Excel文件
XSSFWorkbook workBook = new XSSFWorkbook();
//Excel页脚
XSSFSheet sheet = workBook.createSheet("数据导出");
//设置列的宽度
sheet.setDefaultColumnWidth(16);
//创建标题行
XSSFRow titleRow = sheet.createRow(0);
String[] title = new String[]{"id", "name", "status", "content"};
//设置标题字体样式
XSSFCellStyle cellStyle = workBook.createCellStyle();
XSSFFont font = workBook.createFont();
font.setBold(true);//加粗
font.setFontHeightInPoints((short) 14);//设置字体大小
cellStyle.setFont(font);
//设置标题列
for (int i = 0; i < title.length; i++) {
//创建标题的单元格
XSSFCell titleCell = titleRow.createCell(i);
//填充标题数值
titleCell.setCellValue(title[i]);
//设置样式
titleCell.setCellStyle(cellStyle);
}
//填充数据
//第一行是标题所以要从第二行开始
for (int i = 0; i < peopleList.size(); i++) {
People people = peopleList.get(i);
XSSFRow row = sheet.createRow(i + 1);
for (int j = 0; j < title.length; j++) {
XSSFCell titleCell = row.createCell(j);
String exportKey = title[j];
switch (exportKey) {
case "id":
titleCell.setCellValue(people.getId());
break;
case "name":
titleCell.setCellValue(people.getName());
break;
case "status":
titleCell.setCellValue(people.getStatus());
break;
case "content":
titleCell.setCellValue(people.getContent());
break;
}
}
}
outputStream = new ByteArrayOutputStream();
workBook.write(outputStream);
workBook.close();
// 设置响应头信息
response.setContentType("application/vnd.openxmlformats-officedocument.spreadsheetml.sheet");
response.setHeader("Content-disposition", "attachment; filename=people.xlsx");
// 将字节数组写入响应输出流
response.getOutputStream().write(outputStream.toByteArray());
}
}
Service
@Service
public class ExcelService {
public List<People> getPeoples() {
ArrayList<People> list = new ArrayList<>();
list.add(new People("1","zhangsan","1","hello"));
list.add(new People("2","lisi","1","world"));
list.add(new People("3","wangwu","1","java"));
return list;
}
}
People
public class People {
private String id;
private String name;
private String status;
private String content;
public People(String id, String name, String status, String content) {
this.id = id;
this.name = name;
this.status = status;
this.content = content;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
}
效果
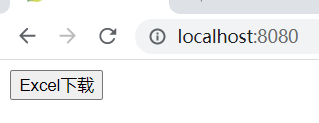
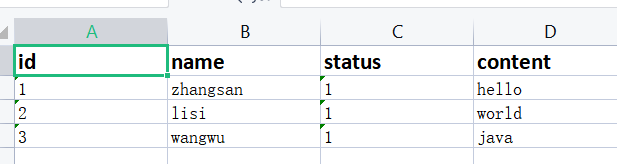