layout: post
title: Struts2
subtitle: Struts基础
date: 2018-05-28
author: ZL
header-img: img/20180528.jpg
catalog: true
tags:
- Struts
搭建运行实例
- 导包
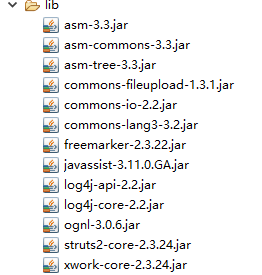
image
- 书写Action类
package zl.hello;
public class TestAction {
public String test() {
// TODO Auto-generated method stub
System.out.println("1111111111111111111");
return "success";
}
}
- 书写src/struts.xml配置文件
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.3//EN"
"http://struts.apache.org/dtds/struts-2.3.dtd">
<struts>
<package name="test" namespace="/test" extends="struts-default">
<action name="TestAction" class="zl.hello.TestAction" method="test">
<result name="success">/hello.jsp</result>
</action>
</package>
</struts>
- web.xml
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
- 运行
image
配置文件详解
struts.xml配置
- package:将Action配置封装.就是可以在Package中配置很多action.
- package的name:package的名字,不能与其他package name重复。
- package的namespace:给action的访问路径中定义一个命名空间。就是在浏览器里面输入的地址。
- package的extends:继承一个指定包。
- package的abstract(一般用不到):包是否为抽象的,标识性属性,标识该包不能独立运行,专门用来被继承。
- action的name:浏览器中的地址名称。
- action的class:Action的全类名。
- action的method:Action类的方法名称。指定调用Action的哪个方法来处理请求。
- result的name:action中方法的返回值。标签体的内容是页面的路径。
- result的type:指定调用哪个result类来处理结果。默认type="dispatcher"。
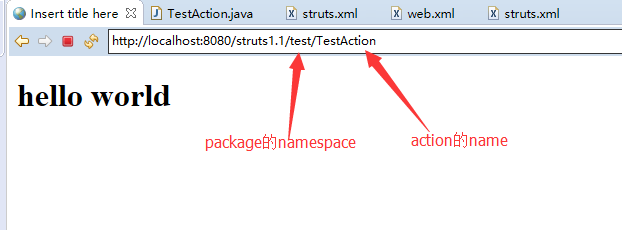
image
result的type有下面几种。
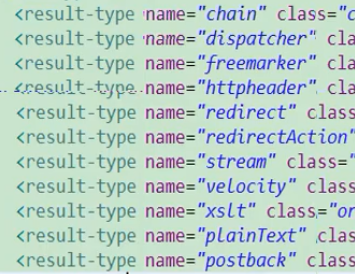
image
struts2常量配置
不去配置的话,有默认的配置。
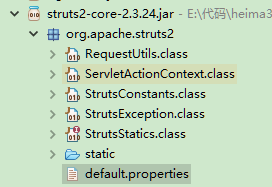
image
修改常量配置。(一般就使用第一种)
- 方式一:
在src/struts.xml中:
image - 方式二:
在src下创建struts.properties
image - 方式三:
在项目的web.xml中
image
加载顺序(后加载的配置覆盖先加载的配置)
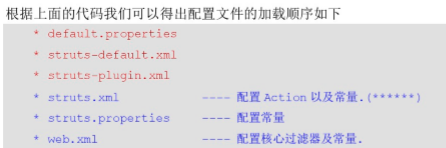
image
常量有哪些?
<!-- i18n:国际化. 解决post提交乱码 -->
<constant name="struts.i18n.encoding" value="UTF-8"></constant>
<!-- 指定反问action时的后缀名(默认的后缀名是.action或者空) -->
<constant name="struts.action.extension" value="action"></constant>
上面的value值如果是action的话,浏览器的地址最后.action 或者为空。http://localhost:8080/struts2_day01/hello/HelloAction.action
但是如果是别的,比如value = “do”,那么浏览器的地址必须要.do。http://localhost:8080/struts2_day01/hello/HelloAction.do
<!-- 指定struts2是否以开发模式运行,默认false
1.热加载主配置.(不需要重启即可生效)
2.提供更多错误信息输出,方便开发时的调试
-->
<constant name="struts.devMode" value="true"></constant>
开启以后,更改struts的配置内容,不需要重新启动服务器就可以生效。
提供了更多的错误输出,方便开发调试。
建议开发的时候打开,开发结束后关闭。
进阶配置
动态方法调用
问题引入
还是上面的例子,现在TestAction里面有多个方法。
package zl.hello;
public class TestAction {
public String fun1() {
System.out.println("fun1");
return "success";
}
public String fun2() {
System.out.println("fun2");
return "success";
}
public String fun3() {
System.out.println("fun3");
return "success";
}
}
可是一个package只能配置一个method。想要调用三个方法,还得配置三个package。
<package name="test" namespace="/test" extends="struts-default">
<action name="TestAction" class="zl.hello.TestAction" method="fun1">
<result name="success">/hello.jsp</result>
</action>
</package>
<package name="test2" namespace="/test2" extends="struts-default">
<action name="TestAction" class="zl.hello.TestAction" method="fun2">
<result name="success">/hello.jsp</result>
</action>
</package>
<package name="test3" namespace="/test3" extends="struts-default">
<action name="TestAction" class="zl.hello.TestAction" method="fun3">
<result name="success">/hello.jsp</result>
</action>
</package>
这就比较麻烦了,动态方法调用就可以解决这个问题,一个package就可以访问到Action里面的多个方法。
方式一(不常用)
strutsx.xml配置文件。
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.3//EN"
"http://struts.apache.org/dtds/struts-2.3.dtd">
<struts>
<!-- 配置动态方法调用是否开启常量
默认是关闭的,需要开启
-->
<constant name="struts.enable.DynamicMethodInvocation" value="true"></constant>
<package name="test" namespace="/test" extends="struts-default">
<action name="TestAction" class="zl.hello.TestAction">
<result name="success">/hello.jsp</result>
</action>
</package>
</struts>
使用:
调用fun1
http://localhost:8080/struts1.1/test/TestAction!fun1
调用fun2
http://localhost:8080/struts1.1/test/TestAction!fun2
调用fun3
http://localhost:8080/struts1.1/test/TestAction!fun3
方式二
strutsx.xml配置文件.注意action的name和method。
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.3//EN"
"http://struts.apache.org/dtds/struts-2.3.dtd">
<struts>
<package name="test" namespace="/test" extends="struts-default">
<action name="TestAction_*" class="zl.hello.TestAction" method="{1}">
<result name="success">/hello.jsp</result>
</action>
</package>
</struts>
使用:
调用fun1
http://localhost:8080/struts1.1/test/TestAction_fun1
调用fun2
http://localhost:8080/struts1.1/test/TestAction_fun2
调用fun3
http://localhost:8080/struts1.1/test/TestAction_fun3
include引入xml
与Android的xml里面的include引入功能一样。

image
Action类的创建方式。(方式三最常用)
方式一
//方式1: 创建一个类.可以是POJO
//POJO:不用继承任何父类.也不需要实现任何接口.
//使struts2框架的代码侵入性更低.
public class Demo3Action {
}
方式二
//方式2: 实现一个接口Action
// 里面有execute方法,提供action方法的规范.
// Action接口预置了一些字符串.可以在返回结果时使用.为了方便
//Action.SUCCESS;
//Action.ERROR;
//Action.NONE;
//Action.INPUT;
//Action.LOGIN
import com.opensymphony.xwork2.Action;
public class Demo4Action implements Action {
@Override
public String execute() throws Exception {
return Action.SUCCESS;
}
}
方式三
//方式3: 继承一个类.ActionSupport
// 帮我们实现了 Validateable, ValidationAware, TextProvider, LocaleProvider .
//如果我们需要用到这些接口的实现时,不需要自己来实现了.
import com.opensymphony.xwork2.ActionSupport;
public class Demo5Action extends ActionSupport{
}
result的type的四种类型
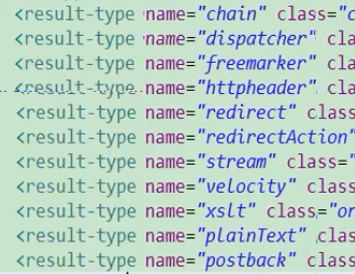
image
type有很多种类型,这里介绍四个,他们是dispatcher、redirect、chain、redirectAction。
<package name="result" namespace="/" extends="struts-default" >
<!-- 转发 -->
<action name="Demo1Action" class="cn.itheima.a_result.Demo1Action" method="execute" >
<result name="success" type="dispatcher" >/hello.jsp</result>
</action>
<!-- 重定向 -->
<action name="Demo2Action" class="cn.itheima.a_result.Demo2Action" method="execute" >
<result name="success" type="redirect" >/hello.jsp</result>
</action>
<!-- 转发到Action -->
<action name="Demo3Action" class="cn.itheima.a_result.Demo3Action" method="execute" >
<result name="success" type="chain">
<!-- action的名字 -->
<param name="actionName">Demo1Action</param>
<!-- action所在的命名空间 -->
<param name="namespace">/</param>
</result>
</action>
<!-- 重定向到Action -->
<action name="Demo4Action" class="cn.itheima.a_result.Demo4Action" method="execute" >
<result name="success" type="redirectAction">
<!-- action的名字 -->
<param name="actionName">Demo1Action</param>
<!-- action所在的命名空间 -->
<param name="namespace">/</param>
</result>
</action>
</package>