#!/bin/bash
API_URL="https://ssss"
REQUEST_COUNT=$(( RANDOM % 23 + 38))
calculate_window() {
local current_ts=$(date +%s)
local today_start=$(date -d "today 09:00:00" +%s)
local today_end=$(date -d "today 21:00:00" +%s)
if [ $current_ts -gt $today_end ]; then
start=$(date -d "tomorrow 09:00:00" +%s)
end=$(date -d "tomorrow 21:00:00" +%s)
else
start=$today_start
end=$today_end
fi
effective_start=$(( current_ts > start ? current_ts : start ))
}
generate_times() {
calculate_window
local window_duration=$(( end - effective_start ))
if [ $window_duration -le 0 ]; then
echo "错误:当前不在有效时间窗口(09:00-21:00)"
exit 1
fi
timestamps=()
for ((i=0; i<REQUEST_COUNT; i++)); do
random_offset=$(( RANDOM % window_duration ))
timestamps+=( $(( effective_start + random_offset )) )
done
timestamps=( $(printf "%s\n" "${timestamps[@]}" | sort -n) )
}
execute_requests() {
echo "今日计划执行 ${REQUEST_COUNT} 次,时间分布如下:"
printf "%s\n" "${timestamps[@]}" | xargs -I{} date -d @{} "+%H:%M:%S"
for ts in "${timestamps[@]}"; do
local current_ts=$(date +%s)
local remaining=$(( ts - current_ts ))
if [ $remaining -gt 0 ]; then
echo "下一次执行:$(date -d "@$ts" '+%H:%M:%S'),等待 ${remaining} 秒..."
sleep $remaining
fi
if curl -s -o /dev/null -w "状态码: %{http_code} 时间: %{time_total}s" "$API_URL"; then
echo " - 请求成功 @ $(date '+%H:%M:%S')"
else
echo " - 请求失败 @ $(date '+%H:%M:%S')"
fi
done
}
generate_times
execute_requests
echo "今日随机 ${REQUEST_COUNT} 次请求全部完成"
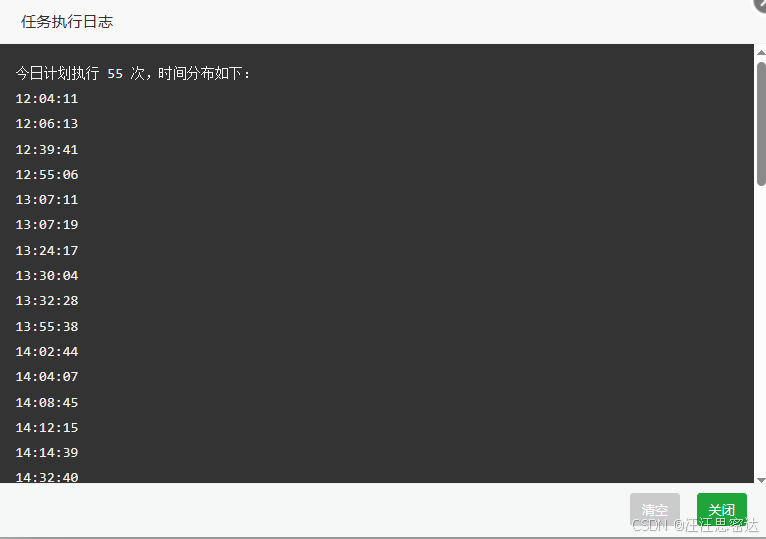