一、概念:
SpEL(Spring Expression Language)既Spring表达式语言,它是一种强大、简洁的装配Bean的方式。它通过运行期执行的表达式将值装配到Bean的属性或构造参数中。它拥有很多特征,包括使用bean的id来引用bean;调用方法和访问对象属性;对值进行算术、关系和逻辑运算;集合操作等等。
二、SpEL的用法(以一个歌手唱歌,表演吉他为例)
- SpEL的首要目标是通过计算获取某个值,使用#{}作为定界符
1、使用bean的id来引用bean
- 定义乐器接口
public interface MusicalInstruments {
public void perform();
}
- 定义吉他实现类
public class Guitar implements MusicalInstruments{
@Override
public void perform() {
System.out.println("吉他表演...");
}
}
- 定义歌手接口
public interface Person {
public void perform();
}
- 歌手实现类
public class Singer implements Person {
private String singerName; //歌手名称
private int age; //歌手年纪
private String songName; //歌曲名称
private MusicalInstruments musicalInstrument; //表演乐器
public void perform(){
System.out.println("我是歌手"+singerName+",今年"+age+"岁,即将演唱的歌曲‘"+ songName+"’");
if(musicalInstrument!=null){
musicalInstrument.perform();
}
}
public String getSingerName() {
return singerName;
}
public void setSingerName(String singerName) {
this.singerName = singerName;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getSongName() {
return songName;
}
public void setSongName(String songName) {
this.songName = songName;
}
public MusicalInstruments getMusicalInstrument() {
return musicalInstrument;
}
public void setMusicalInstrument(MusicalInstruments musicalInstrument) {
this.musicalInstrument = musicalInstrument;
}
}
- 配置文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd">
<bean id="guitar" class="com.chensr.test.Guitar"/>
<!-- 歌手 -->
<bean id="singer" class="com.chensr.test.Singer">
<property name="singerName" value="#{'chensr'}"></property>
<property name="age" value="#{18}"></property>
<property name="musicalInstrument" value="#{guitar}"></property>
<property name="songName" value="#{'英雄泪'}"></property>
</bean>
</beans>
注意:如果注入的值是字符串需要在#{}添加单引号’’;如果注入的是对象只需要在#{}中添加对象的id,spring就会去配置文件中找相应的bean进行注入。
- 编程测试类
public class Test {
@org.junit.Test
public void test(){
ClassPathXmlApplicationContext ac = new ClassPathXmlApplicationContext("spring-context.xml");
Person singer = (Person)ac.getBean("singer");
singer.perform();
}
}
- 运行结果:
我是歌手chensr,今年18岁,即将演唱的歌曲有‘[英雄泪]’
吉他表演...
2、调用方法和访问对象属性
- 定义一个‘模仿者’,该模仿者演唱歌手(歌手名字chensr),并且模仿者的名称就叫做CHENSR COPIER(原歌手名字大写+COPIER)
- 配置文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd">
<bean id="guitar" class="com.chensr.test.Guitar"/>
<!-- 歌手 -->
<bean id="singer" class="com.chensr.test.Singer">
<property name="singerName" value="#{'chensr'}"></property>
<property name="age" value="#{18}"></property>
<property name="musicalInstrument" value="#{guitar}"></property>
<property name="songName" value="#{'英雄泪'}"></property>
</bean>
<!-- 模仿者 -->
<bean id="copier" class="com.chensr.test.Singer">
<property name="singerName" value="#{singer.singerName.toUpperCase()+' COPIER'}"></property>
<property name="age" value="#{18}"></property>
<property name="musicalInstrument" value="#{guitar}"></property>
<property name="songName" value="#{singer.getSongName()}"></property>
</bean>
</beans>
注:配置文件中,模仿者的singerName直接引用singer.singerName,songsName直接调用singer的getSongsName()方法
- 编写测试类
public class Test {
@org.junit.Test
public void test(){
ClassPathXmlApplicationContext ac = new ClassPathXmlApplicationContext("spring-context.xml");
Person copier = (Person)ac.getBean("copier");
copier.perform();
}
}
- 运行结果:
我是歌手CHENSR COPIER,今年18岁,即将演唱的歌曲‘英雄泪’
吉他表演...
3、对值进行算术、关系和逻辑运算
- SpEL支持多种运算符
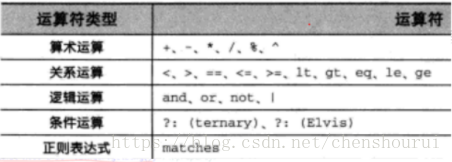
这些运算符跟java并没啥区别,比如模仿者比歌手大一岁,这些如下配置
<property name="age" value="#{singer.age+1}"></property>
4、集合操作(SpEL中筛选集合)
- 通过util空间创建list集合bean存放歌曲,然后随机获取集合bean的值注入Singer
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:util="http://www.springframework.org/schema/util"
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-4.0.xsd">
<util:list id="songs">
<value>英雄泪</value>
<value>封锁我一生</value>
<value>白桦树</value>
</util:list>
<bean id="guitar" class="com.chensr.test.Guitar"/>
<!-- 歌手 -->
<bean id="singer" class="com.chensr.test.Singer">
<property name="singerName" value="#{'chensr'}"></property>
<property name="age" value="#{18}"></property>
<property name="musicalInstrument" value="#{guitar}"></property>
<property name="songName" value="#{songs[T(java.lang.Math).random()*3]}"></property>
</bean>
</beans>
注:[T(java.lang.Math).random()*3用于获取0-2的值
- 编写测试类
public class Test {
@org.junit.Test
public void test(){
ClassPathXmlApplicationContext ac = new ClassPathXmlApplicationContext("spring-context.xml");
Person singer = (Person)ac.getBean("singer");
singer.perform();
}
}
运行结果可以看到歌手随机唱一首歌
注: 除此之外,SpEL还提供了(.?[“查询条件”])用于查找集合成员,同时用(.^[“查询条件”])表示查询第一个符合条件的元素,用(.?[“查询条件”])表示查询最后一个符合条件的元素。而已,SpEL还提供了投影.![“字段名”]来从集合中每个成员选择特定字段放到新的集合中。工作几年也没用过,所以不做详细研究