文章目录
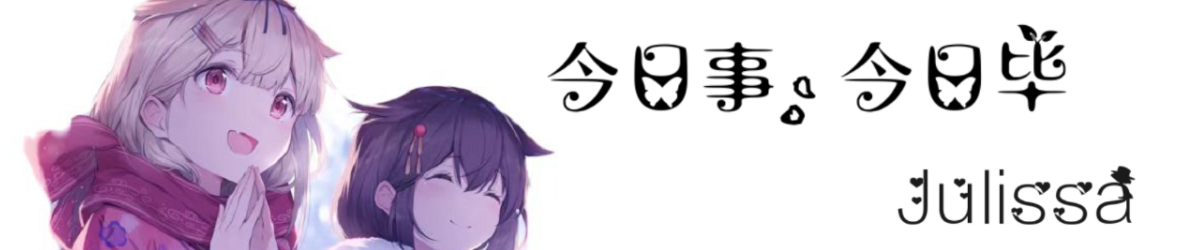
JDBC -(05)工具类封装
1. JDBC工具类封装 V1.0
封装一个工具类,内部包含连接池对象,对外提供获取连接和回收连接的方法
1.1 外部配置文件
位置:src/druid.properties
# Druid连接池配置参数,key固定命名
driverClassName=com.mysql.cj.jdbc.Driver
url=jdbc:mysql://127.0.0.1:3306/julissa
username=root
password=123456
1.2 工具类
package com.julissa.api.utils;
import com.alibaba.druid.pool.DruidDataSource;
import com.alibaba.druid.pool.DruidDataSourceFactory;
import javax.sql.DataSource;
import java.io.IOException;
import java.io.InputStream;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.Properties;
/**
*
* 工具类,内部包含连接池对象,对外提供获取连接和回收连接的方法
* 属性:
* 连接池对象
* 方法:
* 提供获取连接的方法
* 提供回收连接的方法
*/
public class JdbcUtils {
private static DataSource dataSource = null;//连接池对象
static {
//初始化连接池对象
Properties properties = new Properties();
InputStream resourceAsStream = JdbcUtils.class.getClassLoader().getResourceAsStream("druid.properties");
try {
properties.load(resourceAsStream);
} catch (IOException e) {
throw new RuntimeException(e);
}
try {
dataSource = DruidDataSourceFactory.createDataSource(properties);//获取连接池对象
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* 对外提供获取连接的方法
* @return 数据库连接对象
* @throws SQLException
*/
public static Connection getConnection() throws SQLException {
return dataSource.getConnection();
}
/**
* 对外提供回收连接的方法
* @param connection 数据库连接对象
* @throws SQLException
*/
public static void recycleConnection(Connection connection) throws SQLException {
connection.close();
}
}
1.3 测试类
@Test
public void testUtilsV1() throws Exception{
Connection connection = JdbcUtils.getConnection();
String sql = "select * from t_bank";
PreparedStatement preparedStatement = connection.prepareStatement(sql);
ResultSet resultSet = preparedStatement.executeQuery();
while (resultSet.next()){
System.out.println(resultSet.getString("account")+ "--" + resultSet.getInt("money"));
}
JdbcUtils.recycleConnection(connection);
}
1.4 执行结果
2. JDBC工具类封装 V2.0
优化工具类1.0版本,考虑事务的情况下,同一个线程不同方法获取同一个连接
2.1 ThreadLocal
ThreadLoacl,为解决多线程程序的并发问题提供一种新的思路,使用这个工具类可以很简洁地编写出多线程程序,通常用来再多线程中管理共享数据连接。
ThreadLoacl用于保存某个线程共享变量;在Java中,每一个线程对象都有一个ThreadLoaclMap<ThreadLoacl,Object>,其中key就是一个ThreadLoacl,而Object即为该线程的共享变量。而这个map是通过ThreadLoacl的set和get方法操作的。对于一个static ThreadLoacl,不同线程只能从中get,set,remove,而不会影响其他线程的变量。
- ThreadLoacl对象.get:获取ThreadLoacl中当前线程共享变量的值
- ThreadLoacl对象.set:设置ThreadLoacl中当前线程共享变量的值
- ThreadLoacl对象.remove:移除ThreadLoacl中当前线程共享变量的值
2.2 外部配置文件
位置:src/druid.properties
# Druid连接池配置参数,key固定命名
driverClassName=com.mysql.cj.jdbc.Driver
url=jdbc:mysql://127.0.0.1:3306/julissa
username=root
password=123456
2.3 工具类
package com.julissa.api.utils;
import com.alibaba.druid.pool.DruidDataSourceFactory;
import javax.sql.DataSource;
import java.io.IOException;
import java.io.InputStream;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.Properties;
/**
*
* 工具类2.0,内部包含连接池对象,对外提供获取连接和回收连接的方法
* 利用ThreadLocal,确保同一个线程的多个方法获取到同一个connection
* 属性:
* 连接池对象
* 方法:
* 提供获取连接的方法
* 提供回收连接的方法
*/
public class JdbcUtilsV2 {
private static DataSource dataSource = null;//连接池对象
private static ThreadLocal<Connection> threadLocal = new ThreadLocal<>();
static {
//初始化连接池对象
Properties properties = new Properties();
InputStream resourceAsStream = JdbcUtilsV2.class.getClassLoader().getResourceAsStream("druid.properties");
try {
properties.load(resourceAsStream);
} catch (IOException e) {
throw new RuntimeException(e);
}
try {
dataSource = DruidDataSourceFactory.createDataSource(properties);//获取连接池对象
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* 对外提供获取连接的方法
* @return 数据库连接对象
* @throws SQLException
*/
public static Connection getConnection() throws SQLException {
Connection connection = threadLocal.get();//从线程本地变量获取连接
if (connection == null) { //不存在
connection = dataSource.getConnection(); //线程本地变量没有,从连接池中获取
threadLocal.set(connection);
}
return connection;
}
/**
* 对外提供回收连接的方法
* @param
* @throws SQLException
*/
public static void recycleConnection() throws SQLException {
Connection connection = threadLocal.get();//从线程本地变量获取连接
if (connection!= null) {
threadLocal.remove(); //清空线程本地变量数据
connection.setAutoCommit(true);
connection.close();
}
}
}
1.3 测试类
@Test
public void testUtilsV1() throws Exception{
Connection connection = JdbcUtilsV2.getConnection();
String sql = "select * from t_bank";
PreparedStatement preparedStatement = connection.prepareStatement(sql);
ResultSet resultSet = preparedStatement.executeQuery();
while (resultSet.next()){
System.out.println(resultSet.getString("account")+ "--" + resultSet.getInt("money"));
}
JdbcUtilsV2.recycleConnection();
}