public class ZipTest {
public static void main(String[] args) throws IOException {
zipFile("1.txt", "G:\\file\\");
}
/**
* 压缩单个文件
*
* @param fileName 需要压缩的文件名
* @param filePath 需要压缩的文件路径
*/
public static void zipFile(String fileName, String filePath) {
File srcfile = new File(filePath + fileName);
if (!srcfile.exists()) {
return;
}
byte[] buf = new byte[1024];
FileInputStream in = null;
ZipOutputStream out = null;
try {
File targetFile = new File(srcfile.getPath() + ".zip");
out = new ZipOutputStream(new FileOutputStream(targetFile));
int len;
in = new FileInputStream(srcfile);
out.putNextEntry(new ZipEntry(fileName));
while ((len = in.read(buf)) > 0) {
out.write(buf, 0, len);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (out != null) {
out.closeEntry();
}
if (in != null) {
in.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
JAVA 压缩单个文件
最新推荐文章于 2023-07-12 16:38:28 发布
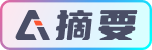