jQuery 对 Ajax 操作进行了封装:
第一层是在 jQuery 中最底层的方法时 $.ajax()第二层是 load(), $.get() 和 $.post()第三层是 $.getScript() 和 $.getJSON()
load()
load(url, [data], [callback])载入远程 HTML 文件代码并插入至 DOM 中
url:待装入 HTML 网页网址。data:发送至服务器的 key/value 数据。在jQuery 1.3中也可以接受一个字符串了。callback:载入成功时回调函数。函数有三个参数:
其值可能为: succuss, error, notmodify, timeout 4 种)data:返回内容textStatus: 代表请求状态的
XMLHttpRequest 对象
默认使用 GET 方式 - 传递附加参数时自动转换为 POST 方式
可以指定选择符,来筛选载入的 HTML 文档,DOM 中将仅插入筛选出的 HTML 代码
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
<!DOCTYPE html PUBLIC
"-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd"
>
<html>
<head>
<meta http-equiv=
"Content-Type"
content=
"text/html; charset=UTF-8"
>
<title>Insert title here</title>
<script type=
"text/javascript"
src=
"../js/jquery-1.8.3.js"
></script>
//===================================================================
<script type=
"text/javascript"
>
$(
function
(){
$(
"#ajaxbutton"
).click(
function
(){
var
url =
"/jQuery2/Demo"
;
var
parme = {
"username"
:
"张三"
,
"password"
:
"1234"
};
$(
this
).load(url,parme,
function
(data){
alert(data);
var
dataparme = eval(
"("
+data+
")"
);
alert(dataparme.message);
});
});
});
</script>
//==========================================================================
</head>
<body>
<input id=
"ajaxbutton"
type=
"button"
value=
"发送ajax"
/>
</body>
</html>
|
Servlet
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
public
void
doGet(HttpServletRequest request, HttpServletResponse response)
throws
ServletException, IOException {
String username = request.getParameter(
"username"
);
String password = request.getParameter(
"password"
);
System.out.println(username+
":"
+password);
//>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
//处理数据
response.setContentType(
"text/html;charset=UTF-8"
);
// 发送相应数据,JSON数据 ,
///* 注意: jQuery 不支持畸形的json格式,key必须使用双引号,否则畸形
//String json = "{'message':''}"; //此数据位畸形json格式
String json =
"{\"message\":\"操作成功\"}"
;
response.getWriter().print(json);
}
|
$.get()
jQuery.get(url, [data], [callback], [type]) 通过远程 HTTP GET 请求载入信息。
url:待载入页面的URL地址data:待发送 Key/value 参数。callback:载入成功时回调函数。type:返回内容格式 : xml, html, script, json, text, _default。
如果传递参数有中文,需要在服务器端接收时进行乱码处理
1
2
3
4
5
6
7
8
9
10
11
|
<script type=
"text/javascript"
>
$(
function
(){
$(
"#ajaxbutton"
).click(
function
(){
var
url =
"/jQuery2/Demo"
;
var
parme = {
"username"
:
"张三"
,
"password"
:
"1234"
};
$.get(url,parme,
function
(data){
alert(data.message);
},
"json"
);
});
});
</script>
|
Servlet
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
public
void
doGet(HttpServletRequest request, HttpServletResponse response)
throws
ServletException, IOException {
String username = request.getParameter(
"username"
);
String password = request.getParameter(
"password"
);
//get获取参数时需要处理中文乱码
String string =
new
String(username.getBytes(
"iso-8859-1"
),
"utf-8"
);
System.out.println(string+
":"
+password);
//>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
//处理数据
response.setContentType(
"text/html;charset=UTF-8"
);
// 发送相应数据,JSON数据 ,
///* 注意: jQuery 不支持畸形的json格式,key必须使用双引号,否则畸形
//String json = "{'message':''}"; //此数据位畸形json格式
String json =
"{\"message\":\"操作成功\"}"
;
response.getWriter().print(json);
}
|
$.post()
jQuery.post(url, [data], [callback], [type]),通过远程 HTTP POST 请求载入信息。
url:发送请求地址。data:待发送 Key/value 参数。callback:发送成功时回调函数。type:返回内容格式,xml, html, script, json, text, _default。
123456789101112<script type=
"text/javascript"
>
$(
function
(){
$(
"#ajaxbutton"
).click(
function
(){
var
url =
"/jQuery2/Demo"
;
var
parme = {
"username"
:
"张三"
,
"password"
:
"1234"
};
$.post(url,parme,
function
(data){
alert(data.message);
},
"json"
);
});
});
</script>
Servlet
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
public
void
doGet(HttpServletRequest request, HttpServletResponse response)
throws
ServletException, IOException {
String username = request.getParameter(
"username"
);
String password = request.getParameter(
"password"
);
System.out.println(username+
":"
+password);
//>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
//处理数据
response.setContentType(
"text/html;charset=UTF-8"
);
// 发送相应数据,JSON数据 ,
///* 注意: jQuery 不支持畸形的json格式,key必须使用双引号,否则畸形
//String json = "{'message':''}"; //此数据位畸形json格式
String json =
"{\"message\":\"操作成功\"}"
;
response.getWriter().print(json);
}
|
$.ajax()
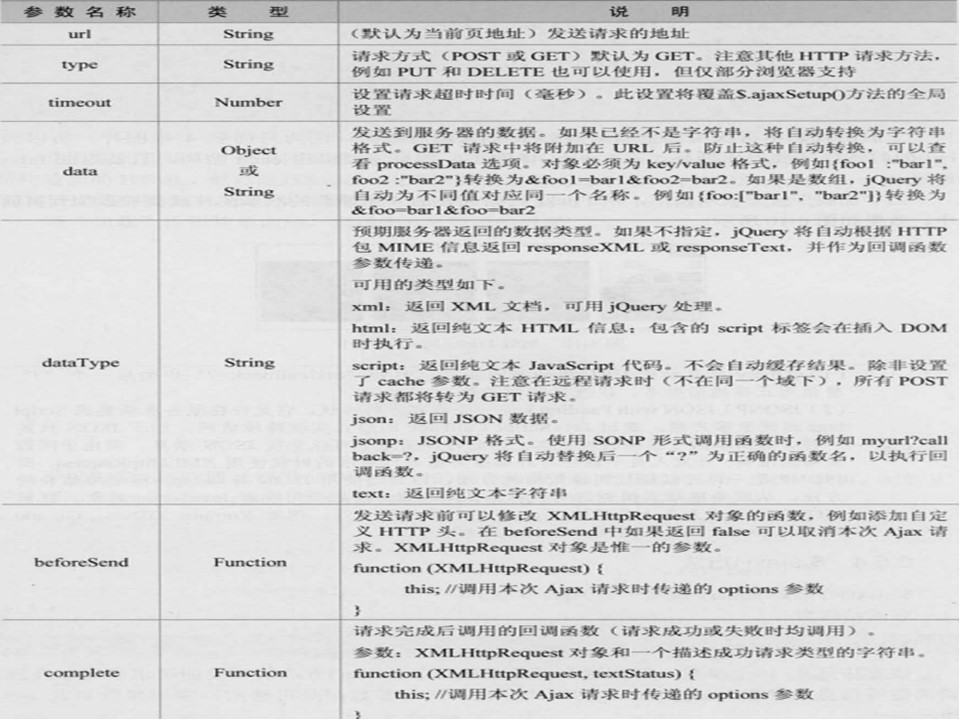
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
|
<script type=
"text/javascript"
>
$(
function
(){
$(
"#ajaxbutton"
).click(
function
(){
var
url =
"/jQuery2/Demo"
;
var
parme = {
"username"
:
"张三"
,
"password"
:
"1234"
};
/* 2.4 $.ajax 底层ajax请求
* $.ajax(url,[settings])
* 一般使用格式:$.ajax(settings)
* url :请求路径
* data :请求参数
* type :请求方式,get、post。默认: GET
* success :成功后回调函数
* error :异常时回调函数
* dataType :务器返回的数据类型。例如:json、html、xml 等
* "xml": 返回 XML 文档,可用 jQuery 处理。
* "html": 返回纯文本 HTML 信息;包含的script标签会在插入dom时执行。
* "script": 返回纯文本 JavaScript 代码。不会自动缓存结果。除非设置了"cache"参数。'''注意:'''在远程请求时(不在同一个域下),所有POST请求都将转为GET请求。(因为将使用DOM的script标签来加载)
* "json": 返回 JSON 数据 。
* "jsonp": JSONP 格式。使用 JSONP 形式调用函数时,如 "myurl?callback=?" jQuery 将自动替换 ? 为正确的函数名,以执行回调函数。
* "text": 返回纯文本字符串
*/
$.ajax({
"url"
:url,
"data"
:params,
"type"
:
"post"
,
"success"
:
function
(data){
alert(data);
},
"error"
:
function
(){
alert(
"服务器异常"
);
},
"dataType"
:
"json"
});
});
});
</script>
|