#ifndef MYOBJECT_H
#define MYOBJECT_H
#include <QObject>
#include <QtQml>
class MyObject : public QObject
{
Q_OBJECT
Q_PROPERTY(QString name READ getValue WRITE setValue NOTIFY nameChange)
public:
static MyObject *Get()
{
static MyObject obj;
return &obj;
}
explicit MyObject(QObject *parent = nullptr);
void setValue(const QString &);
QString getValue();
Q_INVOKABLE void func();
private:
QString name;
signals:
void nameChange(const QString &);
void mySignal(QVariant);
public slots:
void test(QString name);
};
#endif
#include "myobject.h"
MyObject::MyObject(QObject *parent) : QObject(parent)
{
}
void MyObject::setValue(const QString &_context)
{
name = _context;
emit nameChange(name);
}
QString MyObject::getValue()
{
return name;
}
void MyObject::func()
{
qDebug() << __FUNCTION__;
emit mySignal(100);
}
void MyObject::test(QString name)
{
qDebug() << name;
}
import QtQuick 2.9
import QtQuick.Window 2.2
import QtQuick.Controls 2.4
Window {
objectName: "root"
id : root
visible: true
width: 480
height: 360
title: qsTr("Hello World")
signal sigTest(string name)
function func(v){
console.log("func : ", v)
}
Button{
objectName: "btn"
width: 100
height: 100
onClicked: {
sigTest("hello")
}
}
}
#include <QGuiApplication>
#include <QQmlApplicationEngine>
#include <QQmlContext>
#include <QtQml>
#include "myobject.h"
int main(int argc, char *argv[])
{
QCoreApplication::setAttribute(Qt::AA_EnableHighDpiScaling);
QGuiApplication app(argc, argv);
QQmlApplicationEngine engine;
engine.load(QUrl(QStringLiteral("qrc:/main.qml")));
if (engine.rootObjects().isEmpty())
return -1;
QList<QObject*> list = engine.rootObjects();
QObject *obj = list.first();
if(obj)
{
qDebug() << obj->objectName();
QObject::connect(obj, SIGNAL(sigTest(QString)), MyObject::Get(), SLOT(test(QString)));
QObject::connect(MyObject::Get(), SIGNAL(mySignal(QVariant)), obj, SLOT(func(QVariant)));
emit MyObject::Get()->mySignal(100);
}
return app.exec();
}
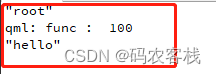
import QtQuick 2.9
import QtQuick.Window 2.2
import QtQuick.Controls 2.4
import MyObj 1.0
Window {
objectName: "root"
id : root
visible: true
width: 480
height: 360
title: qsTr("Hello World")
signal sigTest(string name)
function func(v){
console.log("func : ", v)
}
MyObject{
id : obj
Component.onCompleted: {
console.log("MyObject Completed")
obj.func()
}
}
Component.onCompleted: {
sigTest.connect(obj.test)
obj.mySignal.connect(func)
obj.test("i am cpp")
sigTest("sigTest")
}
}
#include <QGuiApplication>
#include <QQmlApplicationEngine>
#include <QQmlContext>
#include <QtQml>
#include "myobject.h"
int main(int argc, char *argv[])
{
QCoreApplication::setAttribute(Qt::AA_EnableHighDpiScaling);
QGuiApplication app(argc, argv);
qmlRegisterType<MyObject>("MyObj", 1, 0, "MyObject");
QQmlApplicationEngine engine;
engine.load(QUrl(QStringLiteral("qrc:/main.qml")));
if (engine.rootObjects().isEmpty())
return -1;
return app.exec();
}
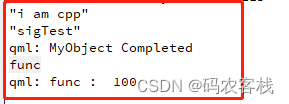
#include <QGuiApplication>
#include <QQmlApplicationEngine>
#include <QQmlContext>
#include <QtQml>
#include "myobject.h"
int main(int argc, char *argv[])
{
QCoreApplication::setAttribute(Qt::AA_EnableHighDpiScaling);
QGuiApplication app(argc, argv);
QQmlApplicationEngine engine;
engine.load(QUrl(QStringLiteral("qrc:/main.qml")));
if (engine.rootObjects().isEmpty())
return -1;
QList<QObject*> list = engine.rootObjects();
QObject *obj = list.first();
if(obj)
{
qDebug() << obj->objectName();
QVariant res;
QVariant arg = "hello, qml";
QMetaObject::invokeMethod(obj, "func", Q_RETURN_ARG(QVariant,res),
Q_ARG(QVariant, arg));
}
return app.exec();
}
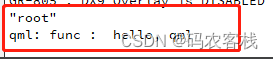
#ifndef OBJECT_H
#define OBJECT_H
#include <QObject>
#include <QtQml>
#include <QLabel>
class Object : public QObject
{
Q_OBJECT
public:
Q_PROPERTY(int index MEMBER index NOTIFY cppSigIndexChanged);
static Object *Get()
{
static Object o;
return &o;
}
explicit Object(QObject *parent = nullptr);
int index = 0;
Q_INVOKABLE void func()
{
qDebug() << "hello, qml";
}
signals:
void cppSigIndexChanged();
};
#endif
import QtQuick 2.2
import QtQuick.Controls 1.2
import QtQuick.Controls.Styles 1.2
import QtQuick 2.14
import Qt.labs.qmlmodels 1.0
import QtMultimedia 5.12
import QtQuick.Controls 2.12
ApplicationWindow {
visible: true
width: 480
height: 360
title: qsTr("Test Custom TableView")
Component.onCompleted: {
obj.func()
obj.index = 100
console.log("index : " + obj.index)
}
}
Object obj;
engine.rootContext()->setContextProperty("obj", &obj);