使用Spring MVC 4构建RESTful服务相对于其它框架来说,有很多优势。首先,Spring MVC 4作为Spring的框架之一,可以很好地与Spring进行集成。其次,Spring MVC 4的拦截器是在方法层级上的拦截,相对于其它MVC框架(如Struts2)的拦截器具有更高的效率。再者,Spring MVC 4采用基于注解的配置,入手容易,开发灵活。
Spring MVC 4采用的是jacson解析JSON。jacson一款非常高效强大的JSON工具类,可以轻松地在JAVA对象与json对象和XML文档之间进行序列化和反序列化。本文中的例子采用的是Spring 4.1.1.RELEASE和jacson 2.4.0版本,经测试无任何异常。接下来,上干货:
开发环境准备:
Eclipse Luna
Apache Tomcat 8.0
JDK 1.7
Spring 4.1.1.RELEASE
jackson 2.4.0
工程目录如下:
1. 新建Maven Project,选择在“Archetype类型”中,选择“maven-archetype-webapp”。
2. 在Pom.xml中添加
Maven 依赖,从而可以使用 Spring和jackson中的特性。如果读者使用的是Dynamic Web Project,可以从Maven仓库下载对的Spring Jar和 jacson jar。
Spring的Maven仓库地址:http://repo1.maven.org/maven2/org/springframework/
jackson的Maven仓库地址:http://repo1.maven.org/maven2/com/fasterxml/jackson/core/
3.在 web.xml中,配置Spring MVC 4 的DispatcherServlet转发和编码。
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.4" xmlns="http://java.sun.com/xml/ns/j2ee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee
http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd">
<display-name>favblog</display-name>
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value></param-value>
</context-param>
<filter>
<filter-name>encodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<servlet>
<servlet-name>springMVC</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath*:spring-context.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>springMVC</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app>
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/s ... ing-context-4.1.xsd
http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.1.xsd">
<context:component-scan base-package="com.favccxx.favsoft.favjson.controller"></context:component-scan>
<mvc:annotation-driven></mvc:annotation-driven>
<bean id="viewResolver" class="org.springframework.web.servlet.view.UrlBasedViewResolver">
<property name="viewClass"
value="org.springframework.web.servlet.view.JstlView" />
<property name="prefix" value="/WEB-INF/views" />
<property name="suffix" value=".jsp" />
</bean>
</beans>
4.新建 FavUser.java 类,测试JSON与Object之间的映射。需要注意的是,如果想对日期进行格式化,在其Getter方法上,使用 @JsonFormat 即可,这样就能输出符合pattern类型的日期,但是这同时也要求输入的日期参数也必须是pattern类型的。
package com.favccxx.favsoft.favjson.pojo;
import java.util.Date;
import com.fasterxml.jackson.annotation.JsonFormat;
public class FavUser {
private String userId;
private String userName;
private int userAge;
private Date createDate;
public String getUserId() {
return userId;
}
public void setUserId(String userId) {
this.userId = userId;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public int getUserAge() {
return userAge;
}
public void setUserAge(int userAge) {
this.userAge = userAge;
}
@JsonFormat(pattern="yyyy-MM-dd HH:mm:ss")
public Date getCreateDate() {
return createDate;
}
public void setCreateDate(Date createDate) {
this.createDate = createDate;
}
}
5. 新建FavRestfulController,通过不同的方法测试Spring MVC 4 返回String、Object类型的JSON。
package com.favccxx.favsoft.favjson.controller;
import java.io.IOException;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.favccxx.favsoft.favjson.pojo.FavUser;
@RestController
public class FavRestfulController {
@RequestMapping(value="/getUserName",method=RequestMethod.POST)
public String getUserName(@RequestParam(value="name") String name){
return name;
}
@RequestMapping("getFavUser")
public FavUser getFavUser(@RequestParam("userName") String userName,String userId,int userAge){
FavUser favUser = new FavUser();
favUser.setUserId(userId);
favUser.setUserName(userName);
favUser.setUserAge(userAge);
return favUser;
}
@RequestMapping("getFavUserBody")
public FavUser getFavUserBody(@RequestBody String body){
ObjectMapper mapper = new ObjectMapper();
FavUser favUser = null;
try {
favUser = mapper.readValue(body, FavUser.class);
} catch (JsonParseException e) {
e.printStackTrace();
} catch (JsonMappingException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return favUser;
}
}
6.运行结果如下:
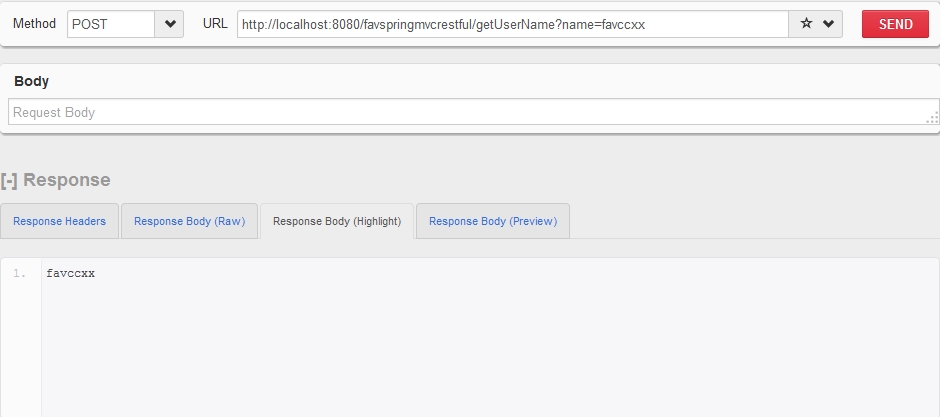
Spring MVC 4采用的是jacson解析JSON。jacson一款非常高效强大的JSON工具类,可以轻松地在JAVA对象与json对象和XML文档之间进行序列化和反序列化。本文中的例子采用的是Spring 4.1.1.RELEASE和jacson 2.4.0版本,经测试无任何异常。接下来,上干货:
开发环境准备:
Eclipse Luna
Apache Tomcat 8.0
JDK 1.7
Spring 4.1.1.RELEASE
jackson 2.4.0
工程目录如下:
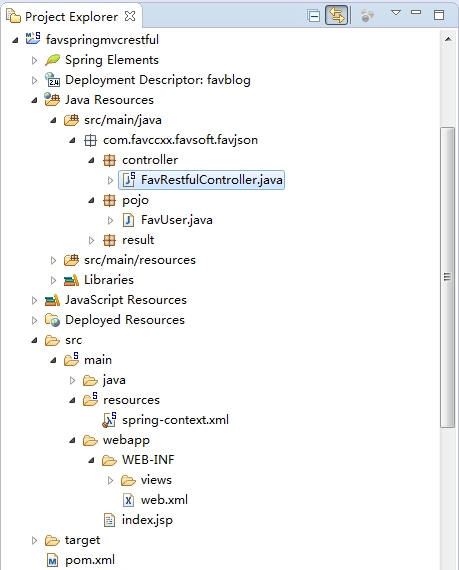
1. 新建Maven Project,选择在“Archetype类型”中,选择“maven-archetype-webapp”。
2. 在Pom.xml中添加
Maven 依赖,从而可以使用 Spring和jackson中的特性。如果读者使用的是Dynamic Web Project,可以从Maven仓库下载对的Spring Jar和 jacson jar。
Spring的Maven仓库地址:http://repo1.maven.org/maven2/org/springframework/
jackson的Maven仓库地址:http://repo1.maven.org/maven2/com/fasterxml/jackson/core/
3.在 web.xml中,配置Spring MVC 4 的DispatcherServlet转发和编码。
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.4" xmlns="http://java.sun.com/xml/ns/j2ee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee
http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd">
<display-name>favblog</display-name>
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value></param-value>
</context-param>
<filter>
<filter-name>encodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<servlet>
<servlet-name>springMVC</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath*:spring-context.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>springMVC</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app>
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/s ... ing-context-4.1.xsd
http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.1.xsd">
<context:component-scan base-package="com.favccxx.favsoft.favjson.controller"></context:component-scan>
<mvc:annotation-driven></mvc:annotation-driven>
<bean id="viewResolver" class="org.springframework.web.servlet.view.UrlBasedViewResolver">
<property name="viewClass"
value="org.springframework.web.servlet.view.JstlView" />
<property name="prefix" value="/WEB-INF/views" />
<property name="suffix" value=".jsp" />
</bean>
</beans>
4.新建 FavUser.java 类,测试JSON与Object之间的映射。需要注意的是,如果想对日期进行格式化,在其Getter方法上,使用 @JsonFormat 即可,这样就能输出符合pattern类型的日期,但是这同时也要求输入的日期参数也必须是pattern类型的。
package com.favccxx.favsoft.favjson.pojo;
import java.util.Date;
import com.fasterxml.jackson.annotation.JsonFormat;
public class FavUser {
private String userId;
private String userName;
private int userAge;
private Date createDate;
public String getUserId() {
return userId;
}
public void setUserId(String userId) {
this.userId = userId;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public int getUserAge() {
return userAge;
}
public void setUserAge(int userAge) {
this.userAge = userAge;
}
@JsonFormat(pattern="yyyy-MM-dd HH:mm:ss")
public Date getCreateDate() {
return createDate;
}
public void setCreateDate(Date createDate) {
this.createDate = createDate;
}
}
5. 新建FavRestfulController,通过不同的方法测试Spring MVC 4 返回String、Object类型的JSON。
package com.favccxx.favsoft.favjson.controller;
import java.io.IOException;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.favccxx.favsoft.favjson.pojo.FavUser;
@RestController
public class FavRestfulController {
@RequestMapping(value="/getUserName",method=RequestMethod.POST)
public String getUserName(@RequestParam(value="name") String name){
return name;
}
@RequestMapping("getFavUser")
public FavUser getFavUser(@RequestParam("userName") String userName,String userId,int userAge){
FavUser favUser = new FavUser();
favUser.setUserId(userId);
favUser.setUserName(userName);
favUser.setUserAge(userAge);
return favUser;
}
@RequestMapping("getFavUserBody")
public FavUser getFavUserBody(@RequestBody String body){
ObjectMapper mapper = new ObjectMapper();
FavUser favUser = null;
try {
favUser = mapper.readValue(body, FavUser.class);
} catch (JsonParseException e) {
e.printStackTrace();
} catch (JsonMappingException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return favUser;
}
}
6.运行结果如下:
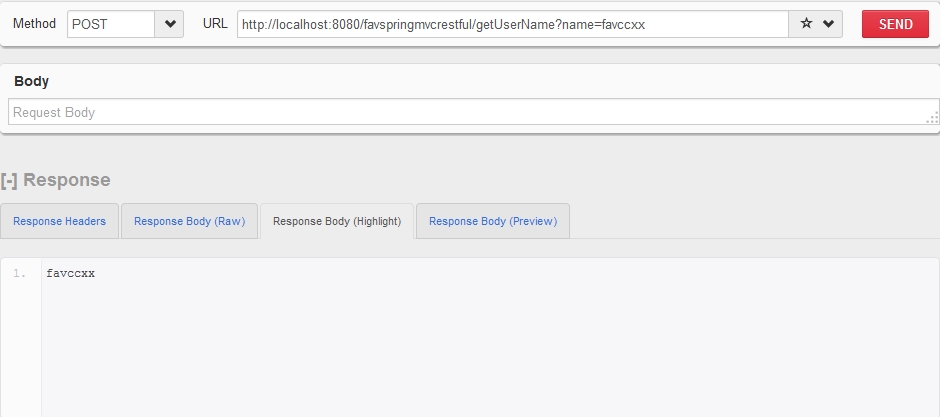
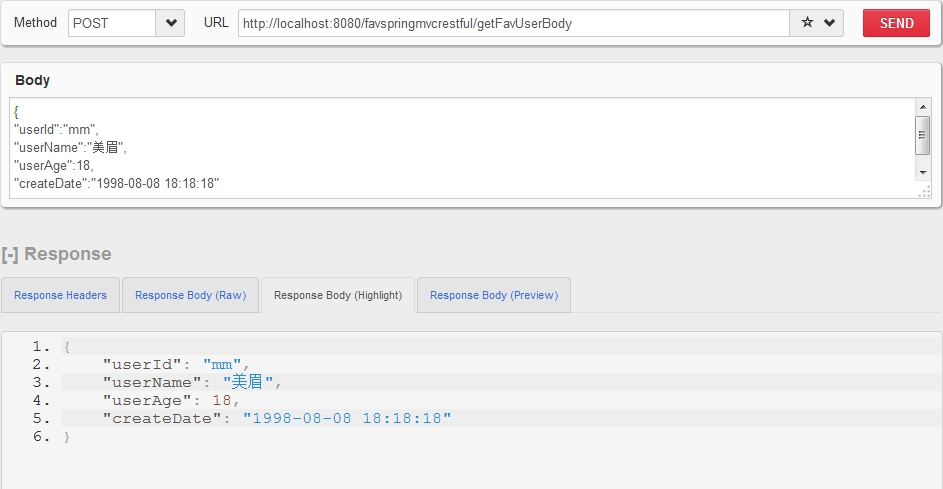