一、在 Visual Studio 2022 中创建 Blazor Web App 项目
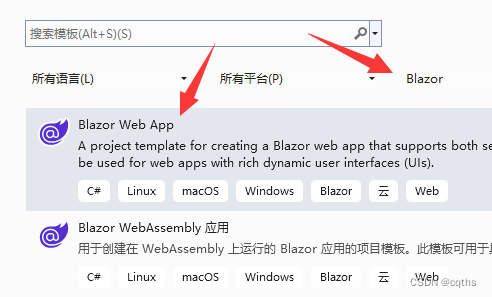
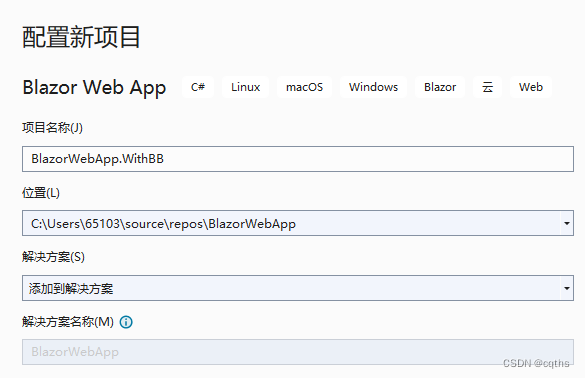
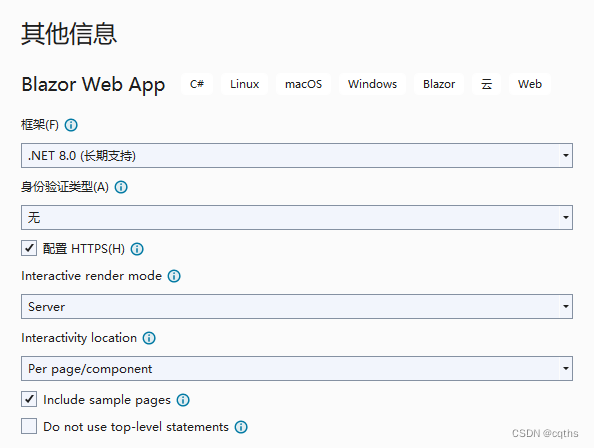
二、使用 NuGet 引用 BootstrapBlazor,尽量选择最新版本
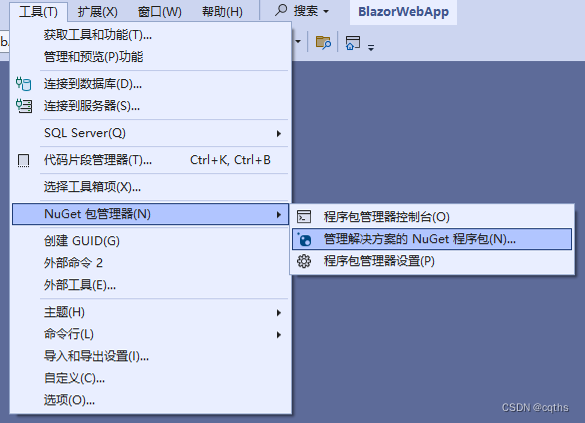
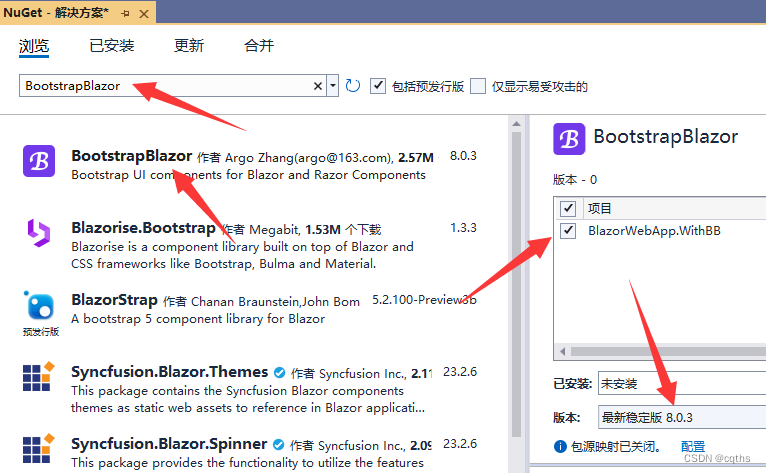
三、使用 NuGet 引用 BootstrapBlazor.FontAwesome
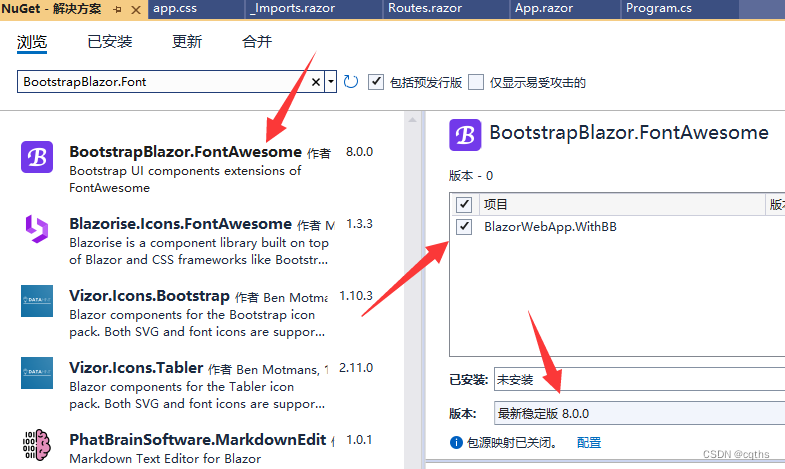
四 、在 Program.cs 中添加引用
注:Blazor Web App 不使用 <NotFound> 模板,需要自定义出错处理页面。
............
............
............
// Add services to the container.
builder.Services.AddRazorComponents()
.AddInteractiveServerComponents();
// BootstrapBlazor 引用
builder.Services.AddBootstrapBlazor();
var app = builder.Build();
............
............
............
// 指定错误处理页面:本例直接使用项目模板自带的 Error.razor
app.UseStatusCodePagesWithRedirects("/Error");
app.Run();
五、在 _Imports.razor 中添加引用
............
............
............
@using static Microsoft.AspNetCore.Components.Web.RenderMode
@using Microsoft.AspNetCore.Components.Web.Virtualization
@using Microsoft.JSInterop
@using BlazorWebApp.WithBB
@using BlazorWebApp.WithBB.Components
@* BootstrapBlazor 引用 *@
@using BootstrapBlazor.Components
六、在 App.razor 中添加引用,指定 rendermode(完整示例)
注1:如果不指定 @rendermode="InteractiveServer",则侧边栏折叠按钮无效。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<base href="/" />
@* 组件样式已集成 Bootstrap 最新版,项目模板自带的则不再需要
<link rel="stylesheet" href="bootstrap/bootstrap.min.css" /> *@
@* 组件样式已集成 Bootstrap 最新版 *@
<link rel="stylesheet" href="_content/BootstrapBlazor/css/bootstrap.blazor.bundle.min.css" />
@* FontAwesoem 字体样式 注意需要引用 BootstrapBlazor.FontAwesome 包 *@
<link rel="stylesheet" href="_content/BootstrapBlazor.FontAwesome/css/font-awesome.min.css" />
@* 如果不使用 Motronic 样式,则下句不需要 *@
<link rel="stylesheet" href="_content/BootstrapBlazor/css/motronic.min.css" />
<link rel="stylesheet" href="app.css" />
<link rel="stylesheet" href="BlazorWebApp.WithBB.styles.css" />
<link rel="icon" type="image/png" href="favicon.png" />
<HeadOutlet />
@* 当前项目的自定义样式 *@
<link rel="stylesheet" href="myapp.css" />
</head>
<body>
@* <Routes />
创建 Blazor Web App 项目时选择的是 Server 模式,此处需要匹配说明。
注1:此处指定 rendermode 后,则在其它 razor 组件中可以不再单独添加。
注2:例如,官方模板中自带的 Counter.razor,其第2行代码不再需要。*@
<Routes @rendermode="InteractiveServer" />
@* BootstrapBlazor 引用 *@
<script src="_content/BootstrapBlazor/js/bootstrap.blazor.bundle.min.js"></script>
<script src="_framework/blazor.web.js"></script>
</body>
</html>
注2:修改后的 Counter.razor
@page "/counter"
@* @rendermode InteractiveServer
注1:如果没有上面一句指定 rendermode,则运行时,单击按钮不会响应相应的事件。
注2:由于本示例已经在 App.razor 中的
<Routes @rendermode="InteractiveServer" />
语句中统一指定了 rendermode,此处就可以省略了。*@
@layout BlazorWebApp.WithBB.Components.Layout.BBLayout
<PageTitle>Counter</PageTitle>
<h1>Counter</h1>
<p role="status">Current count: @currentCount</p>
<button class="btn btn-primary" @onclick="IncrementCount">Click me</button>
@code {
private int currentCount = 0;
private void IncrementCount()
{
currentCount++;
}
}
myapp.css
/* ********** 以下 css 为 BootstrapBlazor 的 <Layout> 组件使用。 ********** */
.layout-header {
border-bottom: 1px solid var(--bs-border-color);
}
.layout-banner {
border-bottom: 1px solid var(--bs-border-color);
}
.layout-side {
border-right: 1px solid var(--bs-border-color);
}
.layout {
--bb-layout-user-height: 0px;
}
.layout-footer-gray {
color: gray;
cursor: pointer;
}
............
............
............
七、在 Layout 文件夹中创建 BootstrapBlazor 模板: BBLayout.razor(完整示例)
@inherits LayoutComponentBase
<Layout SideWidth="0" IsPage="true" IsFullSide="true" ShowCollapseBar="true"
IsFixedHeader="true" IsFixedFooter="true"
ShowFooter="true" ShowGotoTop="true"
Menus="@Menus"
UseTabSet="true" TabDefaultUrl="/">
<Header>
<span class="ms-3 flex-fill">Blazor Web App 模板引用 BootstrapBlazor</span>
<span class="mx-3 d-none d-sm-block">登录用户名</span>
</Header>
<Side>
<div class="layout-banner">
<img alt="logo" class="layout-logo" src="favicon.png" />
<div class="layout-title">
<span>项目名称</span>
</div>
</div>
</Side>
<Main>
@Body
</Main>
<Footer>
<div class="text-center flex-fill">
<a class="layout-footer-gray">版权说明、使用说明</a>
</div>
</Footer>
</Layout>
@code {
private IEnumerable<MenuItem>? Menus { get; set; }
protected override async Task OnInitializedAsync()
{
await base.OnInitializedAsync();
Menus = new List<MenuItem>
{
new () { Text = "Home", Icon = "fa-fw fa-solid fa-house", Url = "/" },
new () { Text = "counter", Icon = "fa-fw fa-solid fa-desktop", Url = "/counter" },
new() { Text = "weather", Icon = "fa-fw fa-solid fa-laptop", Url = "/weather" }
};
}
}
八、在 razor 页面中使用 Layout
@page "/"
@* 指定使用 BBLayout *@
@layout BlazorWebApp.WithBB.Components.Layout.BBLayout
<PageTitle>Home</PageTitle>
<h1>Hello, world!</h1>
Welcome to your new app.
九、运行程序,查看效果
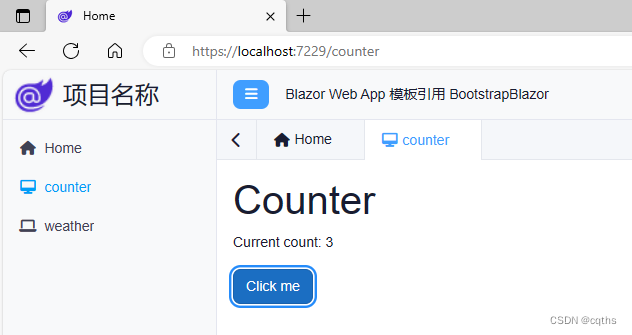