- 长度不受限制的字符串函数
本章的库函数实现的模板都来自VS2022环境下,不同环境可能略有不同
1.1 strlen(求字符串长度)
size_t __cdecl strlen (const char * str)
{
const char *eos = str;
while( *eos++ ) ;
return( eos - str - 1 );
}
从上面的函数
字符串已经 '\0' 作为结束标志,strlen函数返回的是在字符串中 '\0' 前面出现的字符个数(不包
含 '\0' )。
参数指向的字符串必须要以 '\0' 结束。
注意函数的返回值为size_t,是unsigned int
1.2 strcpy(拷贝字符串)
char * strcpy(char * dst, const char * src)
{
char * cp = dst;
while( *cp++ = *src++ )
; /* Copy src over dst */
return( dst );
}
该函数的作用是将指针src处的字符串拷贝到指针dst处
这是cplusplus网站上对于strcpy的解释:
Copies the C string pointed by source into the array pointed by destination, including the terminating null character (and stopping at that point).
解析为以下几点:
源字符串必须以 '\0' 结束
会将源字符串中的 '\0' 拷贝到目标空间
目标空间必须足够大,以确保能存放源字符串
目标空间必须可变
另外:函数返回值为char* 类型的指针dst,指向拷贝的目标空间
1.3 strcat(追加/连接 字符串)
char * __cdecl strcat (char * dst,const char * src)
{
char * cp = dst;
while( *cp )
cp++; /* find end of dst */
while( *cp++ = *src++ ) ; /* Copy src to end of dst */
return( dst ); /* return dst */
}
该函数的作用是将指针src处的字符串追加到指针dst处
Appends a copy of the source string to the destination string. The terminating null character in destination is overwritten by the first character of source, and a null-character is included at the end of the new string formed by the concatenation of both in destination
destination and source shall not overlap.
源字符串必须以 '\0' 结束
目标空间必须有足够的大,能容纳下源字符串的内容
目标空间必须可修改
目标和来源不能重叠
1.4 strcmp(比较字符串)
int __cdecl strcmp (const char * src,const char * dst)
{
int ret = 0 ;
while( ! (ret = *(unsigned char *)src - *(unsigned char *)dst) && *dst)
++src, ++dst;
if ( ret < 0 )
ret = -1 ;
else if ( ret > 0 )
ret = 1 ;
return( ret );
}
该函数的作用是比较两个字符串的大小(非长度,而是ASCII码值)
This function starts comparing the first character of each string. If they are equal to each other, it continues with the following pairs until the characters differ or until a terminating null-character is reached.
This function performs a binary comparison of the characters.
比较每个字符串的第一个字符。如果它们彼此相等,则继续往下对,直到字符不同或达到终止空字符
此函数执行字符的二进制比较
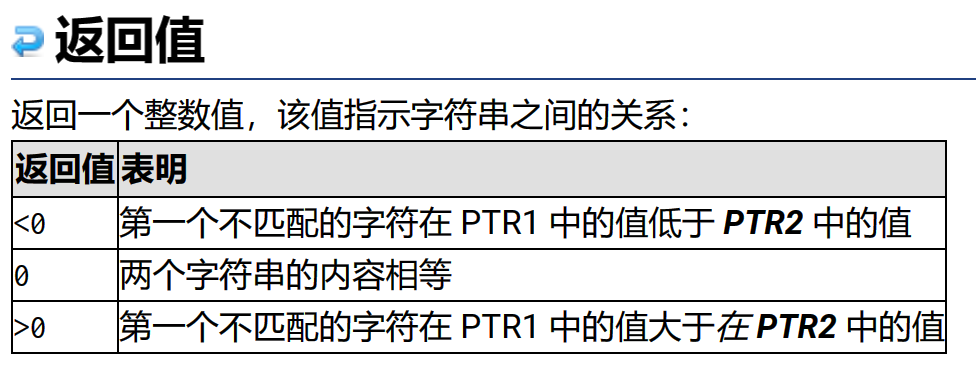
PS:这个库函数可以直接搭配另一个库函数qsort进行排升序
- 长度受限制的字符串函数
这部分函数和以上函数大致相同,只是附加了对字符串的处理长度,所以简化描述
2.1 strncpy(拷贝字符串)
char * strncpy ( char * destination, const char * source, unsigned int num );
Copies the first num characters of source to destination. If the end of the source C string
(which is signaled by a null-character) is found before num characters have been copied,
destination is padded with zeros until a total of num characters have been written to it.
拷贝num个字符从源字符串到目标空间
如果源字符串的长度小于num,则拷贝完源字符串之后,在目标的后边追加0,直到num个
2.2 strncat(追加字符串)
char * strncat ( char * destination, const char * source, unsigned int num );
Appends the first num characters of source to destination, plus a terminating null-character.
If the length of the C string in source is less than num, only the content up to the terminating null-character is copied.
将源的第一个数字字符追加到目标,外加一个终止空字符
如果源中 C 字符串的长度小于 num,则仅复制终止空字符之前的内容
2.3 strncmp(比较字符串)
int strncmp ( const char * str1, const char * str2, unsigned int num );
Compares up to num characters of the C string str1 to those of the C string str2.
This function starts comparing the first character of each string. If they are equal to each other, it continues with the following pairs until the characters differ, until a terminating null-character is reached, or until num characters match in both strings, whichever happens first.
将 字符串 str1 的字符数与 字符串 str2 的字符数进行比较。
此函数开始比较每个字符串的第一个字符。如果它们彼此相等,则继续使用往下对,直到字符不同,或者直到达到终止的空字符,或者直到两个字符串中的 num 个字符全部匹配,以先发生者为准
- 字符串查找
3.1 strstr (在一个字符串中找另一个字符串)
char * __cdecl strstr (const char * str1,const char * str2)
{
char *cp = (char *) str1;
char *s1, *s2;
if ( !*str2 )
return((char *)str1);
while (*cp)
{
s1 = cp;
s2 = (char *) str2;
while ( *s1 && *s2 && !(*s1-*s2) )
s1++, s2++;
if (!*s2)
return(cp);
cp++;
}
return(NULL);
}
该函数的功能是在字符串str1中寻找字符串str2
Returns a pointer to the first occurrence of str2 in str1, or a null pointer if str2 is not part of str1.
The matching process does not include the terminating null-characters, but it stops there.
如果字符串str1中能够匹配字符串str2,则返回字符串str1中对应str2的地址,若不匹配则返回NULL
匹配过程中 ' \0 '不参与匹配,但是它是结束标志
可以观察下面的例子,思考返回值的作用
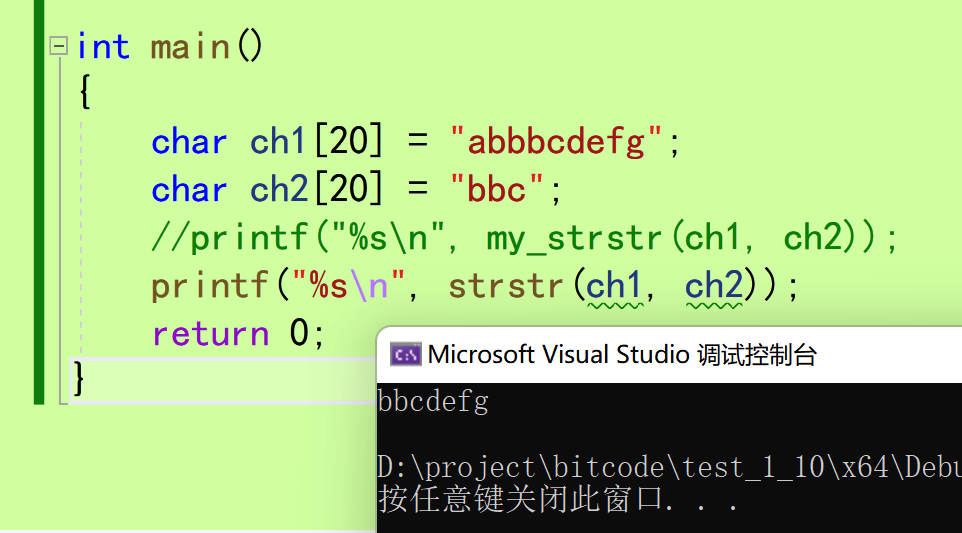