文档对象模型dom
The DOM is the browser’s internal representation of a web page. When the browser retrieves your HTML from your server, the parser analyzes the structure of your code and creates a model of it. Based on this model, the browser then renders the page on the screen.
DOM是浏览器对网页的内部表示。 当浏览器从服务器检索HTML时,解析器将分析代码的结构并为其创建模型。 然后,基于此模型,浏览器将页面呈现在屏幕上。
Browsers expose an API that you can use to interact with the DOM. This is how modern JavaScript frameworks work - they use the DOM API to tell the browser what to display on the page.
浏览器公开了可用于与DOM交互的API。 这就是现代JavaScript框架的工作方式-它们使用DOM API来告诉浏览器在页面上显示什么。
In Single Page Applications, the DOM continuously changes to reflect what appears on the screen, and as a developer you can inspect it using the Browser Developer Tools.
在单页应用程序中,DOM不断变化以反映屏幕上显示的内容,作为开发人员,您可以使用浏览器开发人员工具对其进行检查。
The DOM is language-agnostic, and the de-facto standard to access the DOM is by using JavaScript, since it’s the only language that browsers can run.
DOM与语言无关,事实上,访问DOM的标准是使用JavaScript ,因为它是浏览器可以运行的唯一语言。
The DOM is standardized by WHATWG in the DOM Living Standard Spec.
WHATWG在DOM Living Standard Spec中对DOM进行了标准化 。
With JavaScript you can interact with the DOM to:
使用JavaScript,您可以与DOM交互以:
- inspect the page structure 检查页面结构
- access the page metadata and headers 访问页面元数据和标题
- edit the CSS styling 编辑CSS样式
- attach or remove event listeners 附加或删除事件监听器
- edit any node in the page 编辑页面中的任何节点
- change any node attribute 更改任何节点属性
.. and much more.
.. 以及更多。
The main 2 objects provided by the DOM API that you you will interact the most with are document
and window
.
您将与DOM API进行最多互动的2个主要对象是document
和window
。
窗口对象 (The Window object)
The window
object represents the window that contains the DOM document.
window
对象代表包含DOM文档的窗口。
window.document
points to the document
object loaded in the window.
window.document
指向窗口中加载的document
对象。
Properties and methods of this object can be called without referencing window
explicitly, because it represents the global object. So, the previous property window.document
is usually called just document
.
无需显式引用window
即可调用此对象的属性和方法,因为它表示全局对象。 因此,以前的属性window.document
通常称为document
。
物产 (Properties)
Here is a list of useful properties you will likely reference a lot:
这是您可能会大量引用的有用属性的列表:
console
points to the browser debugging console. Useful to print error messages or logging, usingconsole.log
,console.error
and other tools (see the Browser DevTools article)console
指向浏览器调试控制台。 使用console.log
,console.error
和其他工具来打印错误消息或记录日志时很有用(请参阅Browser DevTools文章)document
as already said, points to thedocument
object, key to the DOM interactions you will performdocument
指向document
对象,是您将执行的DOM交互的关键history
gives access to the History APIhistory
允许访问历史APIlocation
gives access to the Location interface, from which you can determine the URL, the protocol, the hash and other useful information.location
可以访问Location界面 ,从中可以确定URL,协议,哈希和其他有用信息。localStorage
is a reference to the Web Storage API localStorage objectlocalStorage
是对Web Storage API localStorage对象的引用sessionStorage
is a reference to the Web Storage API sessionStorage objectsessionStorage
是对Web Storage API sessionStorage对象的引用
方法 (Methods)
The window
object also exposes useful methods:
window
对象还公开了有用的方法:
alert()
: which you can use to display alert dialogsalert()
:可用于显示警报对话框postMessage()
: used by the Channel Messaging APIpostMessage()
:由Channel Messaging API使用requestAnimationFrame()
: used to perform animations in a way that’s both performant and easy on the CPUrequestAnimationFrame()
:用于以在CPU上既高效又容易的方式执行动画setInterval()
: call a function every n milliseconds, until the interval is cleared withclearInterval()
setInterval()
:每n毫秒调用一次函数,直到使用clearInterval()
清除间隔为止clearInterval()
: clears an interval created withsetInterval()
clearInterval()
:清除使用setInterval()
创建的间隔setTimeout()
: execute a function after ‘n’ millisecondssetTimeout()
:在“ n”毫秒后执行函数setImmediate()
: execute a function as soon as the browser is readysetImmediate()
:浏览器准备好后立即执行一个函数addEventListener()
: add an event listener to the documentaddEventListener()
:将事件侦听器添加到文档中removeEventListener()
: remove an event listener from the documentremoveEventListener()
:从文档中删除事件侦听器
See the full reference of all the properties and methods of the window
object at https://developer.mozilla.org/en-US/docs/Web/API/Window
在https://developer.mozilla.org/zh-CN/docs/Web/API/Window上查看window
对象的所有属性和方法的完整参考。
Document对象 (The Document object)
The document
object represents the DOM tree loaded in a window.
document
对象表示加载在窗口中的DOM树。
Here is a representation of a portion of the DOM pointing to the head and body tags:
这是DOM指向head和body标签的一部分的表示:
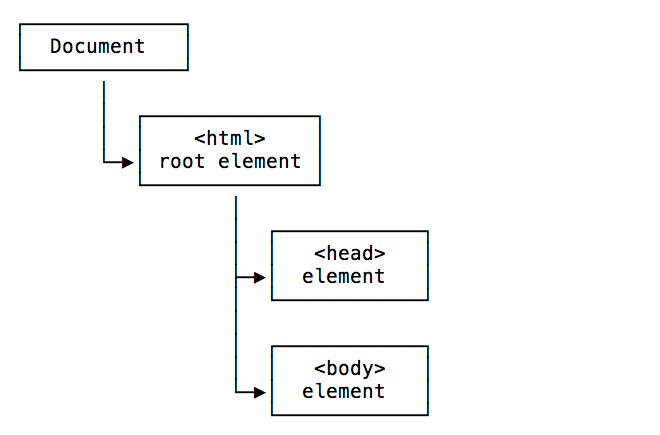
Here is a representation of a portion of the DOM showing the head tag, containing a title tag with its value:
这是DOM的一部分表示,显示了head标签,其中包含带有其值的title标签:

Here is a representation of a portion of the DOM showing the body tag, containing a link, with a value and the href attribute with its value:
这是DOM的一部分的表示形式,显示了body标签,其中包含带有值的链接和带有其值的href属性:
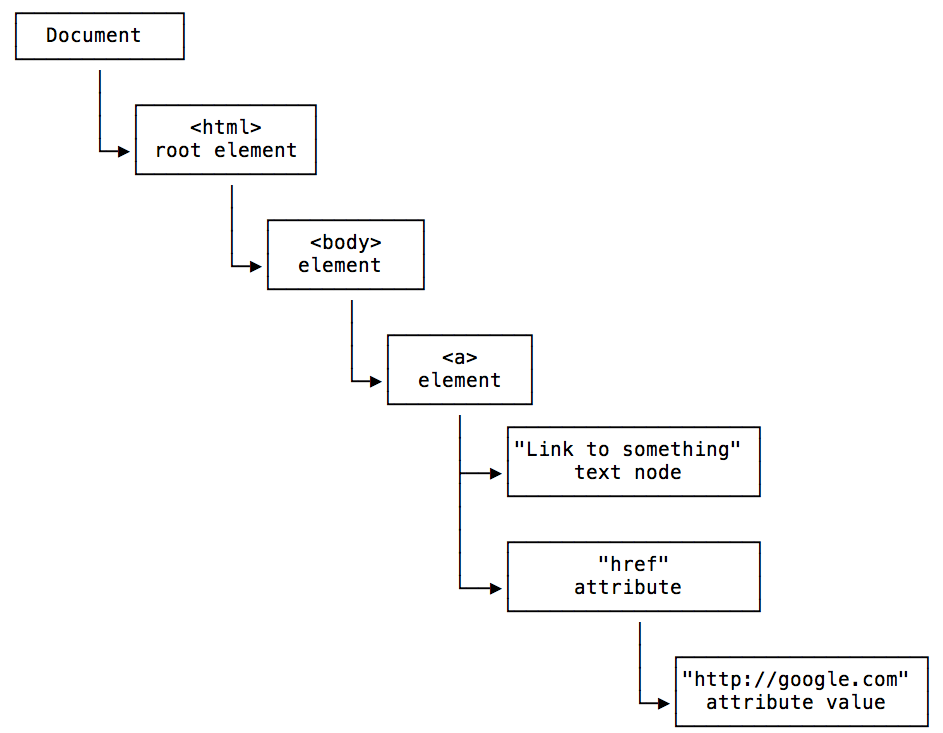
The Document object can be accessed from window.document
, and since window
is the global object, you can use the shortcut document
object directly from the browser console, or in your JavaScript code.
可以从window.document
访问Document对象,并且由于window
是全局对象,因此可以直接从浏览器控制台或在JavaScript代码中使用快捷方式document
对象。
This Document object has a ton of properties and methods. The Selectors API methods are the ones you’ll likely use the most:
该Document对象具有大量的属性和方法。 Selectors API方法是您最常使用的方法:
document.getElementById()
document.getElementById()
document.querySelector()
document.querySelector()
document.querySelectorAll()
document.querySelectorAll()
document.getElementsByTagName()
document.getElementsByTagName()
document.getElementsByClassName()
document.getElementsByClassName()
You can get the document title using document.title
, and the URL using document.URL
. The referrer is available in document.referrer
, the domain in document.domain
.
您可以使用document.title
获得文档标题,并使用document.URL
获得URL。 引荐是提供document.referrer
,在域document.domain
。
From the document
object you can get the body and head Element nodes:
从document
对象中,您可以获取body和head 元素节点 :
document.documentElement
: the Document nodedocument.documentElement
:“文档”节点document.body
: thebody
Element nodedocument.body
:body
元素节点document.head
: thehead
Element nodedocument.head
:head
元素节点
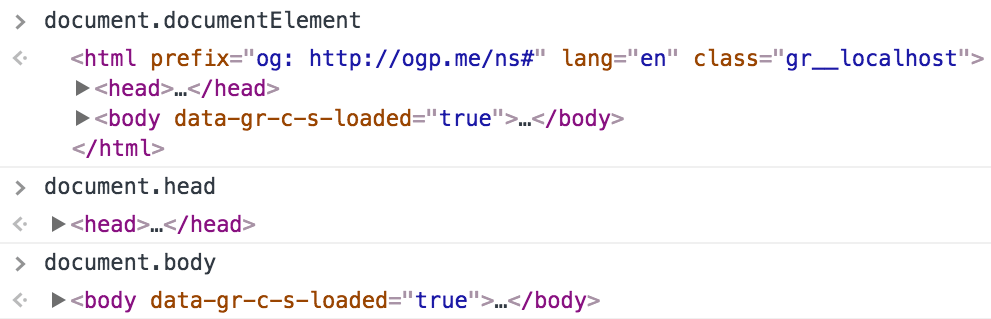
You can also get a list of all the element nodes of a particular type, like an HTMLCollection of all the links using document.links
, all the images using document.images
, all the forms using document.forms
.
您还可以得到一个特定类型的所有元素的节点列表,像一个HTMLCollection全部使用环节的document.links
,全部采用图像document.images
,全部采用的形式document.forms
。
The document cookies are accessible in document.cookie
. The last modified date in document.lastModified
.
可在document.cookie
中访问文档cookie 。 document.lastModified
的最后修改日期。
You can do much more, even get old school and fill your scripts with document.write()
, a method that was used a lot back in the early days of JavaScript to interact with the pages.
您可以做更多的事情,甚至学到老,并用document.write()
填充脚本,该方法早在JavaScript早期就用于与页面进行交互。
See the full reference of all the properties and methods of the document
object at https://developer.mozilla.org/en-US/docs/Web/API/Document
请参阅https://developer.mozilla.org/zh-CN/docs/Web/API/Document上的document
对象的所有属性和方法的完整参考。
节点类型 (Types of Nodes)
There are different types of nodes, some of which you have already seen in the example images above. The main ones you will encounter are:
有不同类型的节点,您已经在上面的示例图像中看到了其中的一些。 您将遇到的主要问题是:
Document: the document Node, the start of the tree
Document :文档节点,树的开始
Element: an HTML tag
元素 :HTML标签
Attr: an attribute of a tag
Attr :标签的属性
Text: the text content of an Element or Attr Node
文本 :元素或属性节点的文本内容
Comment: an HTML comment
注释 :HTML注释
DocumentType: the Doctype declaration
DocumentType : Doctype声明
遍历DOM (Traversing the DOM)
The DOM is a tree of elements, with the Document node at the root, which points to the html
Element node, which in turn points to its child element nodes head
and body
, and so on.
DOM是一棵元素树,其Document节点位于根,它指向html
Element节点,而html
元素节点又指向其子元素节点head
和body
,依此类推。
From each of those elements, you can navigate the DOM structure and move to different nodes.
从这些元素的每一个中,您都可以导航DOM结构并移至其他节点。
得到父母 (Getting the parent)
Every element has just one parent.
每个元素只有一个父对象。
To get it, you can use Node.parentNode
or Node.parentElement
(where Node means a node in the DOM).
要获取它,可以使用Node.parentNode
或Node.parentElement
(其中Node表示DOM中的一个节点)。
They are almost the same, except when ran on the html
element: parentNode
returns the parent of the specified node in the DOM tree, while parentElement
returns the DOM node’s parent Element, or null if the node either has no parent, or its parent isn’t a DOM Element.
它们几乎相同,除了在html
元素上运行时: parentNode
返回DOM树中指定节点的父级,而parentElement
返回DOM节点的父元素,如果该节点没有父级,或者其父级不是不是DOM元素。
People usually use parentNode
.
人们通常使用parentNode
。
让孩子们 (Getting the children)
To check if a Node has child nodes, use Node.hasChildNodes()
which returns a boolean value.
要检查Node是否有子节点,请使用Node.hasChildNodes()
,它返回一个布尔值。
To access all the Children Element Nodes of a node, use Node.childNodes
.
要访问节点的所有子元素节点,请使用Node.childNodes
。
The DOM also exposes a Node.children
method. However, it does not just include Element nodes, but also the white space between elements as Text nodes. This is not something you generally want.
DOM还公开了Node.children
方法。 但是,它不仅包含Element节点,而且还包含Text元素之间的空白。 这不是您通常想要的东西。
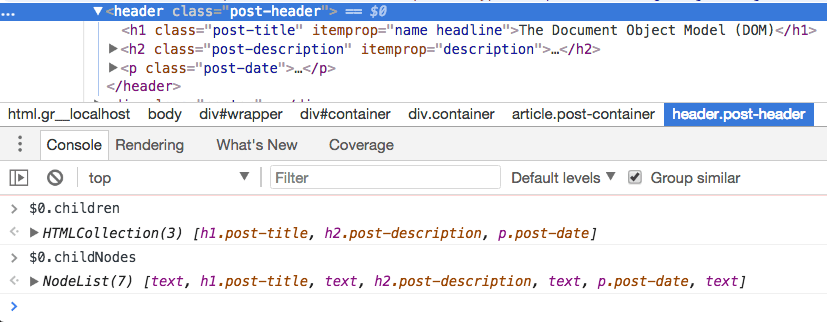
To get the first child Element Node, use Node.firstElementChild
. To get the last child Element Node, use Node.lastElementChild
:
要获取第一个子元素节点,请使用Node.firstElementChild
。 要获取最后一个子元素Node,请使用Node.lastElementChild
:

The DOM also exposes Node.firstChild
and Node.lastChild
, with the difference that they do not “filter” the tree for Element nodes only. They will also show empty Text nodes that indicate white space.
DOM还公开了Node.firstChild
和Node.lastChild
,不同之处在于它们不“过滤”仅用于Element节点的树。 它们还将显示空的Text节点,指示空白。
In short, to navigate children Element Nodes use
简而言之,要导航子元素节点使用
Node.childNodes
Node.childNodes
Node.firstElementChild
Node.firstElementChild
Node.lastElementChild
Node.lastElementChild
得到兄弟姐妹 (Getting the siblings)
In addition to getting the parent and the children, since the DOM is a tree you can also get the siblings of any Element Node.
除了获取父级和子级之外,由于DOM是一棵树,因此您还可以获取任何元素节点的同级。
You can do so using
您可以使用
Node.previousElementSibling
Node.previousElementSibling
Node.nextElementSibling
Node.nextElementSibling
The DOM also exposes previousSibling
and nextSibling
, but as their counterparts described above, they include white spaces as Text nodes, so you generally avoid them.
DOM还公开了previousSibling
和nextSibling
,但正如上文所述,它们包括空白作为Text节点,因此通常避免使用它们。
编辑DOM (Editing the DOM)
The DOM offers various methods to edit the nodes of the page and alter the document tree.
DOM提供了多种方法来编辑页面的节点并更改文档树。
With
用
document.createElement()
: creates a new Element Nodedocument.createElement()
:创建一个新的Element节点document.createTextNode()
: creates a new Text Nodedocument.createTextNode()
:创建一个新的文本节点
you can create new elements, and add them to the DOM elements you want as children, by using document.appendChild()
:
您可以使用document.appendChild()
创建新元素,并将其添加到想要作为子元素的DOM元素中:
const div = document.createElement('div')
div.appendChild(document.createTextNode('Hello world!'))
first.removeChild(second)
removes the child node “second” from the node “first”.first.removeChild(second)
从节点“ first”中删除子节点“ second”。document.insertBefore(newNode, existingNode)
inserts “newNode” as a sibling of “existingNode”, placing it before that in the DOM tree structure.document.insertBefore(newNode, existingNode)
将“ newNode”作为“ existingNode”的同级插入,并将其放置在DOM树结构中。element.appendChild(newChild)
alters the tree under “element”, adding a new child Node “newChild” to it, after all the other children.element.appendChild(newChild)
更改“ element”下的树,并在所有其他子节点之后向其添加新的子节点“ newChild”。element.prepend(newChild)
alters the tree under “element”, adding a new child Node “newChild” to it, before other child elements. You can pass one or more child Nodes, or even a string which will be interpreted as a Text node.element.prepend(newChild)
更改“ element”下的树,并在其他子元素之前向其添加新的子节点“ newChild”。 您可以传递一个或多个子节点,甚至可以传递将被解释为文本节点的字符串。element.replaceChild(newChild, existingChild)
alters the tree under “element”, replacing “existingChild” with a new Node “newChild”.element.replaceChild(newChild, existingChild)
更改“ element”下的树,将“ existingChild”替换为新的节点“ newChild”。element.insertAdjacentElement(position, newElement)
inserts “newElement” in the DOM, positioned relatively to “element” depending on “position” parameter value. See the possible values.element.insertAdjacentElement(position, newElement)
在DOM中插入“ newElement”,其位置取决于“ position”参数值,相对于“ element”。 请参阅可能的值 。element.textContent = 'something'
changes the content of a Text node to “something”.element.textContent = 'something'
将文本节点的内容更改为“ something”。
文档对象模型dom