github组织存储库使用
In this post I’m going to ask to the GitHub API information about public repositories, using just the Go net/http
stdlib package, without any additional library.
在本文中,我将仅使用Go net/http
stdlib包,不带任何其他库的情况下,向GitHub API询问有关公共存储库的信息。
GitHub has a nice public API called Search, which you can inspect at https://developer.github.com/v3/search/#search-repositories.
GitHub有一个不错的公共API,称为Search,您可以在https://developer.github.com/v3/search/#search-repositories进行检查。
In particular, I’m interested in getting the Go repos with more than 10k stars at this point in time.
特别是,我有兴趣在此时获得超过1万颗星的Go存储库 。
We can pass it a query to get exactly what we want. Looking at the Search API docs we’ll have to make an HTTP GET request to https://api.github.com/search/repositories
passing the query ?q=stars:>=10000+language:go&sort=stars&order=desc
: give me the repositories with more than 10k stars, with language Go, sort them by number of stars.
我们可以向其传递查询以获取所需的确切信息。 查看Search API文档,我们必须通过查询?q=stars:>=10000+language:go&sort=stars&order=desc
向https://api.github.com/search/repositories
发出HTTP GET请求:给我超过1万颗星的存储库,使用Go语言,按星数对它们进行排序。
Simplest snippet: we use http.Get
from net/http
and we read everything that’s returned using ioutil.ReadAll
:
最简单的代码段:我们使用来自net/http
http.Get
,并使用ioutil.ReadAll
读取返回的所有内容:
package main
import (
"io/ioutil"
"log"
"net/http"
)
func main() {
res, err := http.Get("https://api.github.com/search/repositories?q=stars:>=10000+language:go&sort=stars&order=desc")
if err != nil {
log.Fatal(err)
}
body, err := ioutil.ReadAll(res.Body)
res.Body.Close()
if err != nil {
log.Fatal(err)
}
if res.StatusCode != 200 {
log.Fatal("Unexpected status code", res.StatusCode)
}
log.Printf("Body: %s\n", body)
}

It’s not much readable, as you can see. You can paste what you get using an online formatter to understand the actual structure.
如您所见,它的可读性不高 。 您可以使用在线格式化程序粘贴获得的内容,以了解实际结构。
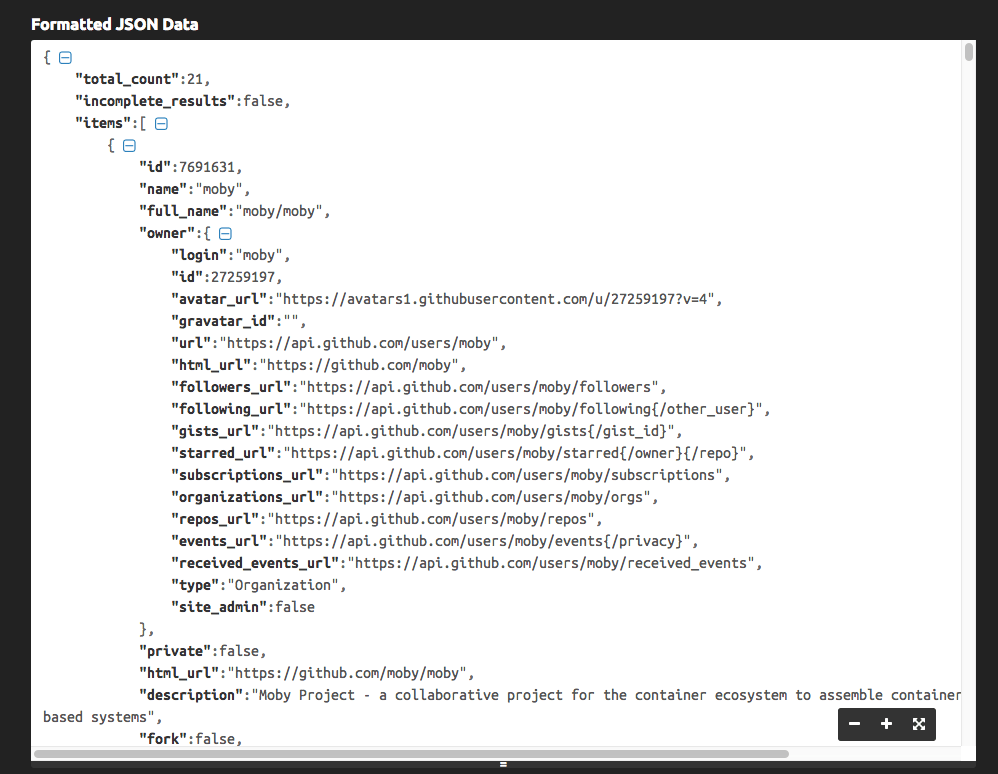
Let’s now process the JSON and generate a nice table from it.
现在让我们处理JSON并从中生成一个漂亮的表。
If you’re new to the JSON topic, check out my preliminary guide on how to process JSON with Go.
如果您不熟悉 JSON主题,请查看我的初步指南, 了解如何使用Go处理JSON 。
package main
import (
"encoding/json"
"fmt"
"io/ioutil"
"log"
"net/http"
"os"
"text/tabwriter"
"time"
)
// Owner is the repository owner
type Owner struct {
Login string
}
// Item is the single repository data structure
type Item struct {
ID int
Name string
FullName string `json:"full_name"`
Owner Owner
Description string
CreatedAt string `json:"created_at"`
StargazersCount int `json:"stargazers_count"`
}
// JSONData contains the GitHub API response
type JSONData struct {
Count int `json:"total_count"`
Items []Item
}
func main() {
res, err := http.Get("https://api.github.com/search/repositories?q=stars:>=10000+language:go&sort=stars&order=desc")
if err != nil {
log.Fatal(err)
}
body, err := ioutil.ReadAll(res.Body)
res.Body.Close()
if err != nil {
log.Fatal(err)
}
if res.StatusCode != http.StatusOK {
log.Fatal("Unexpected status code", res.StatusCode)
}
data := JSONData{}
err = json.Unmarshal(body, &data)
if err != nil {
log.Fatal(err)
}
printData(data)
}
func printData(data JSONData) {
log.Printf("Repositories found: %d", data.Count)
const format = "%v\t%v\t%v\t%v\t\n"
tw := new(tabwriter.Writer).Init(os.Stdout, 0, 8, 2, ' ', 0)
fmt.Fprintf(tw, format, "Repository", "Stars", "Created at", "Description")
fmt.Fprintf(tw, format, "----------", "-----", "----------", "----------")
for _, i := range data.Items {
desc := i.Description
if len(desc) > 50 {
desc = string(desc[:50]) + "..."
}
t, err := time.Parse(time.RFC3339, i.CreatedAt)
if err != nil {
log.Fatal(err)
}
fmt.Fprintf(tw, format, i.FullName, i.StargazersCount, t.Year(), desc)
}
tw.Flush()
}
The above code creates 3 structs to unmarshal the JSON provided by GitHub. JSONData
is the main container, Items
is a slice of Item
, the repository struct, and inside item, Owner
contains the repository owner data.
上面的代码创建了3个结构以解组GitHub提供的JSON。 JSONData
是主要容器, Items
是Item
,是存储库结构,而在item内部, Owner
包含存储库所有者数据。
Given the HTTP response res
returned by http.Get
we extract the body using res.Body
and we read all of it in body
with ioutil.ReadAll
.
由于HTTP响应res
通过返回http.Get
我们使用提取体res.Body
和我们读到这一切在body
与ioutil.ReadAll
。
After checking the res.StatusCode
matches the http.StatusOK
constant (which corresponds to 200
), we unmarshal the JSON using json.Unmarshal
into our JSONData
struct data
, and we print it by passing it to our printData
struct.
检查res.StatusCode
与http.StatusOK
常量(对应于200
)匹配后,我们使用json.Unmarshal
将JSON解json.Unmarshal
到JSONData
结构data
,并将其传递给printData
结构进行打印。
Inside printData
I instantiate a tabwriter.Writer
, then process and format the JSON data accordingly to output a nice table layout:
在printData
内部,我实例化了一个tabwriter.Writer
,然后相应地处理和格式化JSON数据以输出漂亮的表布局:

github组织存储库使用