Strict Mode is an ES5 feature, and it’s a way to make JavaScript behave in a better way.
严格模式是ES5的功能,它是使JavaScript表现得更好的一种方式 。
And in a different way, as enabling Strict Mode changes the semantics of the JavaScript language.
并且以另一种方式 ,启用严格模式会更改JavaScript语言的语义。
It’s really important to know the main differences between JavaScript code in strict mode, and “normal” JavaScript, which is often referred as sloppy mode.
了解严格模式下JavaScript代码与通常称为“ 草率模式 ”的“正常” JavaScript之间的主要区别非常重要。
Strict Mode mostly removes functionality that was possible in ES3, and deprecated since ES5 (but not removed because of backwards compatibility requirements)
严格模式主要删除了ES3可能提供的功能,自ES5起不推荐使用(但由于向后兼容性要求而未删除)
如何启用严格模式 (How to enable Strict Mode)
Strict mode is optional. As with every breaking change in JavaScript, we can’t change how the language behaves by default, because that would break gazillions of JavaScript around, and JavaScript puts a lot of effort into making sure 1996 JavaScript code still works today. It’s a key of its success.
严格模式是可选的。 与JavaScript的每项重大更改一样,我们无法更改默认语言的行为,因为这将破坏成千上万JavaScript,并且JavaScript投入了大量精力来确保1996年JavaScript代码今天仍然有效。 这是其成功的关键。
So we have the 'use strict'
directive we need to use to enable Strict Mode.
因此,我们具有启用严格模式所需的'use strict'
指令。
You can put it at the beginning of a file, to apply it to all the code contained in the file:
您可以将其放在文件的开头,以将其应用于文件中包含的所有代码:
'use strict'
const name = 'Flavio'
const hello = () => 'hey'
//...
You can also enable Strict Mode for an individual function, by putting 'use strict'
at the beginning of the function body:
您还可以通过在函数主体的开头放置'use strict'
来为单个功能启用严格模式:
function hello() {
'use strict'
return 'hey'
}
This is useful when operating on legacy code, where you don’t have the time to test or the confidence to enable strict mode on the whole file.
当您在没有时间测试或没有信心在整个文件上启用严格模式的旧代码上运行时,这很有用。
严格模式下有什么变化 (What changes in Strict Mode)
偶然的全局变量 (Accidental global variables)
If you assign a value to an undeclared variable, JavaScript by default creates that variable on the global object:
如果为未声明的变量分配值,则JavaScript默认情况下会在全局对象上创建该变量:
;(function() {
variable = 'hey'
})()(() => {
name = 'Flavio'
})()
variable //'hey'
name //'Flavio'
Turning on Strict Mode, an error is raised if you try to do what we did above:
启用严格模式后,如果尝试执行上面的操作,则会引发错误:
;(function() {
'use strict'
variable = 'hey'
})()(() => {
'use strict'
myname = 'Flavio'
})()

分配错误 (Assignment errors)
JavaScript silently fails some conversion errors.
JavaScript默默地失败了一些转换错误。
In Strict Mode, those silent errors now raise issues:
在严格模式下,这些无提示错误现在引发问题:
const undefined = 1(() => {
'use strict'
undefined = 1
})()
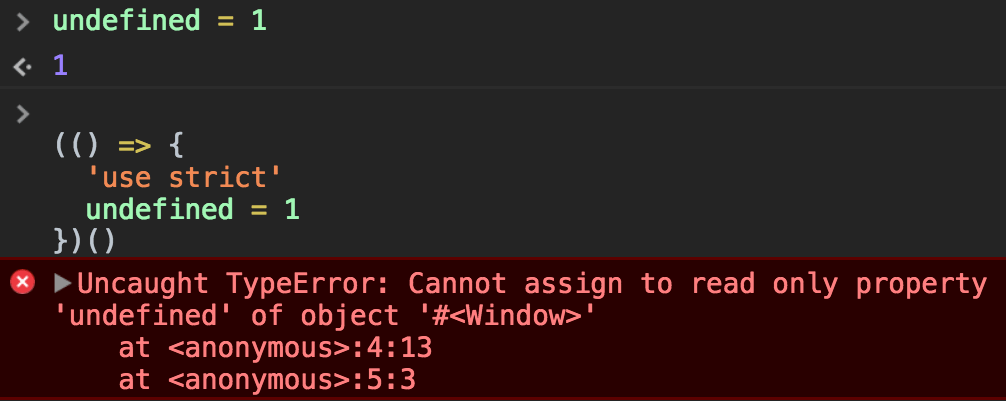
The same applies to Infinity, NaN, eval
, arguments
and more.
这同样适用于Infinity,NaN, eval
, arguments
等。
In JavaScript you can define a property of an object to be not writable, by using
在JavaScript中,您可以使用以下方法将对象的属性定义为不可写
const car = {}
Object.defineProperty(car, 'color', { value: 'blue', writable: false })
In strict mode, you can’t override this value, while in sloppy mode that’s possible:
在严格模式下,您不能覆盖此值,而在草率模式下,则可以:
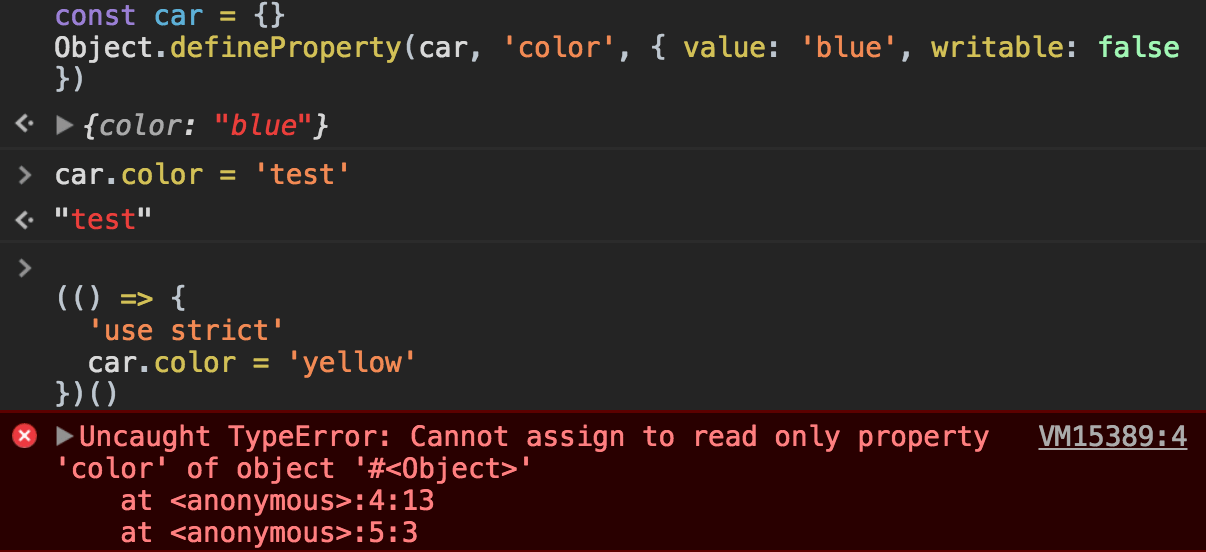
The same works for getters:
同样适用于吸气剂:
const car = {
get color() {
return 'blue'
}
}
car.color = 'red'(
//ok
() => {
'use strict'
car.color = 'yellow' //TypeError: Cannot set property color of #<Object> which has only a getter
}
)()
Sloppy mode allows to extend a non-extensible object:
马虎模式允许扩展一个不可扩展的对象:
const car = { color: 'blue' }
Object.preventExtensions(car)
car.model = 'Fiesta'(
//ok
() => {
'use strict'
car.owner = 'Flavio' //TypeError: Cannot add property owner, object is not extensible
}
)()
(see Object.preventExtensions()
)
(请参阅Object.preventExtensions()
)
Also, sloppy mode allows to set properties on primitive values, without failing, but also without doing nothing at all:
同样,草率模式允许在原始值上设置属性,而不会失败,也不会执行任何操作:
true.false = ''(
//''
1
).name =
'xxx' //'xxx'
var test = 'test' //undefined
test.testing = true //true
test.testing //undefined
Strict mode fails in all those cases:
在所有这些情况下,严格模式都会失败:
;(() => {
'use strict'
true.false = ''(
//TypeError: Cannot create property 'false' on boolean 'true'
1
).name =
'xxx' //TypeError: Cannot create property 'name' on number '1'
'test'.testing = true //TypeError: Cannot create property 'testing' on string 'test'
})()
删除错误 (Deletion errors)
In sloppy mode, if you try to delete a property that you cannot delete, JavaScript returns false, while in Strict Mode, it raises a TypeError:
在草率模式下,如果尝试删除无法删除的属性,则JavaScript返回false,而在严格模式下,它将引发TypeError:
delete Object.prototype(
//false
() => {
'use strict'
delete Object.prototype //TypeError: Cannot delete property 'prototype' of function Object() { [native code] }
}
)()
具有相同名称的函数参数 (Function arguments with the same name)
In normal functions, you can have duplicate parameter names:
在正常功能中,您可以具有重复的参数名称:
(function(a, a, b) {
console.log(a, b)
})(1, 2, 3)
//2 3
(function(a, a, b) {
'use strict'
console.log(a, b)
})(1, 2, 3)
//Uncaught SyntaxError: Duplicate parameter name not allowed in this context
Note that arrow functions always raise a SyntaxError
in this case:
请注意,在这种情况下,箭头函数始终会引发SyntaxError
:
((a, a, b) => {
console.log(a, b)
})(1, 2, 3)
//Uncaught SyntaxError: Duplicate parameter name not allowed in this context
八进制语法 (Octal syntax)
Octal syntax in Strict Mode is disabled. By default, prepending a 0
to a number compatible with the octal numeric format makes it (sometimes confusingly) interpreted as an octal number:
严格模式下的八进制语法已禁用。 默认情况下,在与八进制数字格式兼容的数字前加上0
会使它(有时会引起混淆)被解释为八进制数字:
(() => {
console.log(010)
})()
//8
(() => {
'use strict'
console.log(010)
})()
//Uncaught SyntaxError: Octal literals are not allowed in strict mode.
You can still enable octal numbers in Strict Mode using the 0oXX
syntax:
您仍然可以使用0oXX
语法在严格模式下启用八进制数字:
;(() => {
'use strict'
console.log(0o10)
})()
//8
删除with
(Removed with
)
Strict Mode disables the with
keyword, to remove some edge cases and allow more optimization at the compiler level.
严格模式禁用with
关键字,以删除一些边缘情况并允许在编译器级别进行更多优化。