vue toast
toast-ui.vue编辑器 (toast-ui.vue-editor)
This is Vue component wrapping TOAST UI Editor.
这是包装TOAST UI Editor的Vue组件。
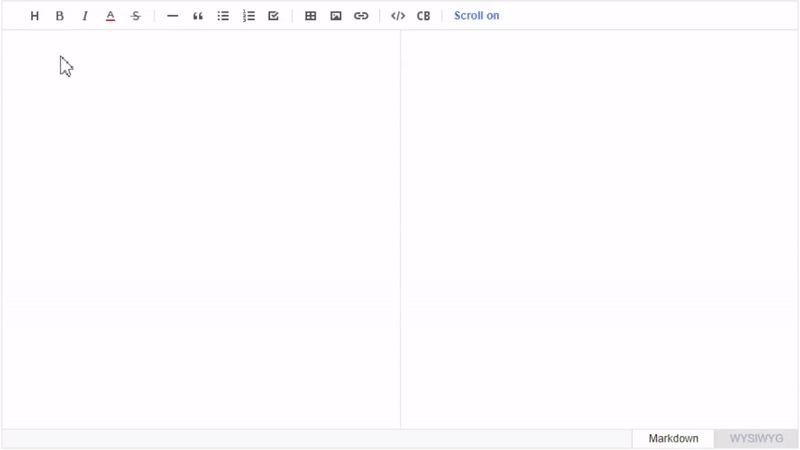
收集有关开源使用的统计信息 (Collect statistics on the use of open source)
Vue Wrapper of TOAST UI Editor applies Google Analytics (GA) to collect statistics on the use of open source, in order to identify how widely TOAST UI Editor is used throughout the world. It also serves as important index to determine the future course of projects. location.hostname (e.g. > “ui.toast.com") is to be collected and the sole purpose is nothing but to measure statistics on the usage. To disable GA, use the following usageStatistics
options when declare Vue Wrapper compoent.
TOAST UI Editor的Vue Wrapper应用Google Analytics(分析)(GA)来收集有关开源使用情况的统计信息,以便确定TOAST UI Editor在全世界的使用范围。 它也是确定项目未来走向的重要指标。 要收集location.hostname(例如>“ ui.toast.com”),其唯一目的只是测量使用情况统计信息。要禁用GA,请在声明Vue Wrapper usageStatistics
时使用以下usageStatistics
选项。
var options = {
...
usageStatistics: false
}
Or, include include tui-code-snippet.js
(v1.4.0 or later) and then immediately write the options as follows:
或者,包括include tui-code-snippet.js
( v1.4.0或更高版本 ),然后立即编写以下选项:
tui.usageStatistics = false;
💾安装 (💾 Install)
使用npm (Using npm)
npm install --save @toast-ui/vue-editor
🔨用法 (🔨 Usage)
If you want to more details, see Tutorials 👀
如果您想了解更多详细信息,请参见教程 👀
加载 (Load)
You can use Toast UI Editor for Vue as moudule format or namespace. Also you can use Single File Component (SFC of Vue). When using module format and SFC, you should load tui-editor.css
, tui-editor-contents.css
and codemirror.css
in the script.
您可以将Toast UI Editor for Vue用作模块格式或名称空间。 您也可以使用单一文件组件(Vue的SFC)。 使用模块格式和SFC时,应在脚本中加载tui-editor.css
, tui-editor-contents.css
和codemirror.css
。
-
Using Ecmascript module
使用Ecmascript模块
import 'tui-editor/dist/tui-editor.css'; import 'tui-editor/dist/tui-editor-contents.css'; import 'codemirror/lib/codemirror.css'; import { Editor } from '@toast-ui/vue-editor'
-
Using Commonjs module
使用Commonjs模块
require('tui-editor/dist/tui-editor.css'); require('tui-editor/dist/tui-editor-contents.css'); require('codemirror/lib/codemirror.css'); var toastui = require('@toast-ui/vue-editor'); var Editor = toastui.Editor;
-
Using Single File Component
使用单个文件组件
import 'tui-editor/dist/tui-editor.css'; import 'tui-editor/dist/tui-editor-contents.css'; import 'codemirror/lib/codemirror.css'; import Editor from '@toast-ui/vue-editor/src/editor.vue'
-
Using namespace
使用名称空间
var Editor = toastui.Editor;
实行 (Implement)
First implement <editor/>
in the template.
首先在模板中实现<editor/>
。
<template>
<editor/>
</template>
And then add Editor
to the components
in your component or Vue instance like this:
然后添加Editor
的components
在组件或VUE实例是这样的:
import { Editor } from '@toast-ui/vue-editor'
export default {
components: {
'editor': Editor
}
}
or
要么
import { Editor } from '@toast-ui/vue-editor'
new Vue({
el: '#app',
components: {
'editor': Editor
}
});
使用v模型 (Using v-model)
If you use v-model, you have to declare a data
for binding.
如果使用v-model,则必须声明要绑定的data
。
In the example below, editorText
is binding to the text of the editor.
在下面的示例中, editorText
绑定到编辑器的文本。
<template>
<editor v-model="editorText"/>
</template>
<script>
import { Editor } from '@toast-ui/vue-editor'
export default {
components: {
'editor': Editor
},
data() {
return {
editorText: ''
}
}
}
</script>
道具 (Props)
Name | Type | Default | Description |
---|---|---|---|
value | String | '' | This prop can change content of the editor. If you using v-model , don't use it. |
options | Object | following defaultOptions | Options of tui.editor. This is for initailize tui.editor. |
height | String | '300px' | This prop can control the height of the editor. |
previewStyle | String | 'tab' | This prop can change preview style of the editor. (tab or vertical ) |
mode | String | 'markdown' | This prop can change mode of the editor. (markdown or wysiwyg ) |
html | String | - | If you want change content of the editor using html format, use this prop. |
visible | Boolean | true | This prop can control visible of the eiditor. |
名称 | 类型 | 默认 | 描述 |
---|---|---|---|
值 | 串 | '' | 该道具可以改变编辑器的内容。 如果您使用v-model ,请不要使用它 。 |
选项 | 目的 | 以下defaultOptions | tui.editor的选项。 这是为了使tui.editor无效。 |
高度 | 串 | '300px' | 该道具可以控制编辑器的高度。 |
PreviewStyle | 串 | '标签' | 该道具可以改变编辑器的预览风格。 ( tab 或vertical ) |
模式 | 串 | '降价' | 该道具可以改变编辑器的模式。 ( markdown 或wysiwyg ) |
html | 串 | -- | 如果要使用html格式更改编辑器的内容,请使用此道具。 |
可见 | 布尔型 | 真正 | 该道具可以控制看守者。 |
const defaultOptions = {
minHeight: '200px',
language: 'en_US',
useCommandShortcut: true,
useDefaultHTMLSanitizer: true,
usageStatistics: true,
hideModeSwitch: false,
toolbarItems: [
'heading',
'bold',
'italic',
'strike',
'divider',
'hr',
'quote',
'divider',
'ul',
'ol',
'task',
'indent',
'outdent',
'divider',
'table',
'image',
'link',
'divider',
'code',
'codeblock'
]
};
事件 (Event)
load : It would be emitted when editor fully load
load:编辑器完全加载时会发出
change : It would be emitted when content changed
change:内容更改时会发出
stateChange : It would be emitted when format change by cursor position
stateChange:通过光标位置更改格式时将发出
focus : It would be emitted when editor get focus
focus:当编辑器获得焦点时将发出
blur : It would be emitted when editor loose focus
模糊:当编辑器失去焦点时会发出
方法 (Method)
If you want to more manipulate the Editor, you can use invoke
method to call the method of tui.editor. For more information of method, see method of tui.editor.
如果要更多地操纵编辑器,则可以使用invoke
方法来调用tui.editor方法。 有关method的更多信息,请参见tui.editor的method 。
First, you need to assign ref
attribute of <editor/>
and then you can use invoke
method through this.$refs
like this:
首先,您需要分配<editor/>
ref
属性,然后可以通过this.$refs
使用invoke
方法,如下所示:
<template>
<editor ref="tuiEditor"/>
</template>
<script>
import { Editor } from '@toast-ui/vue-editor'
export default {
components: {
'editor': Editor
},
methods: {
scroll() {
this.$refs.tuiEditor.invoke('scrollTop', 10);
},
moveTop() {
this.$refs.tuiEditor.invoke('moveCursorToStart');
},
getHtml() {
let html = this.$refs.tuiEditor.invoke('getHtml');
}
}
};
</script>
🔧拉取步骤 (🔧 Pull Request Steps)
TOAST UI products are open source, so you can create a pull request(PR) after you fix issues. Run npm scripts and develop yourself with the following process.
TOAST UI产品是开源的,因此您可以在解决问题后创建提取请求(PR)。 运行npm脚本并通过以下过程开发自己。
建立 (Setup)
Fork develop
branch into your personal repository. Clone it to local computer. Install node modules. Before starting development, you should check to haveany errors.
develop
分支develop
到您的个人存储库中。 克隆到本地计算机。 安装节点模块。 在开始开发之前,您应该检查是否有任何错误。
$ git clone https://github.com/{your-personal-repo}/[[repo name]].git
$ cd [[repo name]]
$ npm install
vue toast