带倍速播放的播放器
MP3 Player with HTML5. Lesson 2 – Controlling Play, Pause and Stop with Javascript In the previous section we created an audio element using HTML5 and then controlled it using the built-in controls available through the browser. In most applications, however, you will want the user to have more control over the audio (or video) playback. The Javascript API associated with the audio (and video) element provides a robust set of commands for controlling and monitoring the media stream.
带有HTML5的MP3播放器。 第2课–使用Javascript控制播放,暂停和停止在上一节中,我们使用HTML5创建了音频元素,然后使用浏览器提供的内置控件对其进行了控制。 但是,在大多数应用程序中,您将希望用户对音频(或视频)播放有更多的控制。 与音频(和视频)元素关联的Javascript API提供了一组可靠的命令,用于控制和监视媒体流。
In this chapter, we’re going to move beyond the provided HTML5 controls and start using Javascript to control the audio playback.
在本章中,我们将超越提供HTML5控件,并开始使用Javascript来控制音频播放。
If you haven’t used much Javascript before, let me talk a little bit about the language and its usage. First, you will often hear Javascript referred to as a scripting language — not a programming language. While the difference between scripting and programming languages is becoming much less distinct, it is worth mentioning. Javascript is considered a scripting language because it is processed line-by-line in the browser from top to bottom. This is unlike a true programming language, which is interpreted as machine language or (in the case of Java or .Net languages) as a byte code.
如果您以前没有使用过太多的Javascript,那么让我再谈一下该语言及其用法。 首先,您经常会听到称为脚本语言的Javascript,而不是编程语言。 尽管脚本语言和编程语言之间的差异变得越来越少,但值得一提。 Javascript被视为一种脚本语言,因为它是在浏览器中从上到下逐行处理的。 这不同于真正的编程语言,后者被解释为机器语言或(对于Java或.Net语言而言)被解释为字节码。
While the distinction referenced above may seem like minutiae, it has important ramifications for the scope and performance of the language itself. The first, and perhaps most obvious ramification of the scripting environment, is that the language is dependent on the browser environment to run. Scripting languages also run more slowly than more traditional programming languages.
尽管上面提到的区别看起来像是细节,但是它对语言本身的范围和性能也有重要的影响。 脚本环境的第一个,也是最明显的后果是,该语言取决于运行的浏览器环境。 与更传统的编程语言相比,脚本语言的运行速度也更慢。
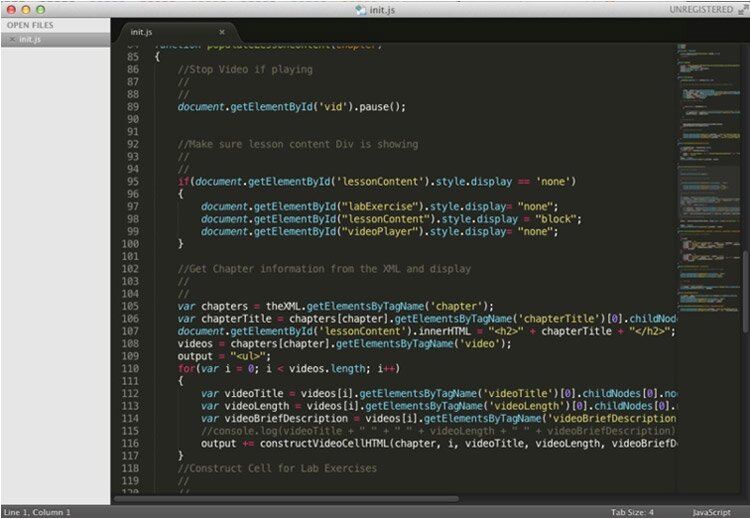
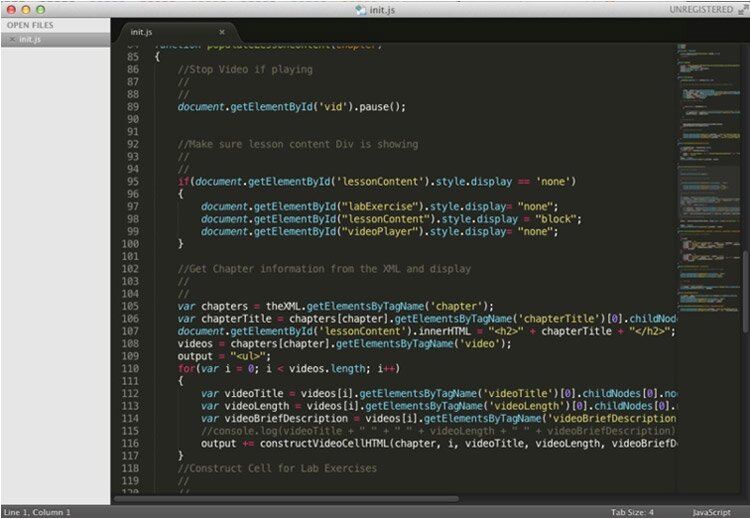
Javascript code viewed in a text editor
在文本编辑器中查看的Java代码
Despite what you might perceive as drawbacks of using a scripting language, Javascript is becoming an increasingly important tool in the programmer’s toolkit. Due to the growth of mobile applications, and the need to offload heavy processing from a user’s environment on to a server, the service-oriented architecture became important. Javascript is used to facilitate a service-oriented architecture, as it allows direct communication with a web server.
尽管您可能会觉得使用脚本语言有很多弊端,但是Javascript在程序员工具箱中正变得越来越重要。 由于移动应用程序的增长,以及需要将繁重的处理任务从用户环境转移到服务器上, 面向服务的体系结构变得非常重要。 Javascript用于促进面向服务的体系结构,因为它允许与Web服务器直接通信。
In our example, we’re going to use Javascript to go beyond the HTML5 embedded player and control the media ourselves.
在我们的示例中,我们将使用Javascript超越HTML5嵌入式播放器并自己控制媒体。
To get started, we’re going to need to load the example that we created in the previous chapter.
首先,我们需要加载上一章中创建的示例。
1. Open your text editor.
1.打开您的文本编辑器。
2. Open the file that you created in the first lesson. If you don’t have the file any longer, type the code as it appears below. Remember to save your music file(s) to your drive in the same folder as your HTML code.
2.打开您在第一课中创建的文件。 如果您再也没有该文件,请输入下面显示的代码。 请记住,将音乐文件保存到驱动器中与HTML代码相同的文件夹中。
<!DOCTYPE html>
<html>
<head>
<title>Audio Player</title>
</head>
<body>
<audio controls="controls">
<source src="music.mp3" type="audio/mpeg">
<source src="music.ogg" type="audio/ogg">
</audio>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title>Audio Player</title>
</head>
<body>
<audio controls="controls">
<source src="music.mp3" type="audio/mpeg">
<source src="music.ogg" type="audio/ogg">
</audio>
</body>
</html>
3. In the <audio> tag, delete the controls="controls" attribute and value. We’re going to be making these controls ourselves using Javascript in this and subsequent lessons.
3.在<audio>标记中,删除controls =“ controls”属性和值。 在本课程和后续课程中,我们将使用Javascript自己制作这些控件。
4. Since the controls will not be visible, we’re going to have to provide some controls to the user. We’ll do this by providing buttons that the user can click. Place your cursor after the closing audio tag and before the closing body tag. You’ll recall that closing tags begin with a forward slash.
4.由于控件将不可见,因此我们将不得不向用户提供一些控件。 我们将通过提供用户可以单击的按钮来完成此操作。 将光标放在结束音频标签之后和结束正文标签之前。 您会记得关闭标签以正斜杠开头。
5. Add the button code as it appears below:
5.添加按钮代码,如下所示:
<button onclick="playMusic()">Play</button><br />
<button onclick="pauseMusic()">Pause</button><br />
<button onclick="stopMusic()">Stop</button><br />
<button onclick="playMusic()">Play</button><br />
<button onclick="pauseMusic()">Pause</button><br />
<button onclick="stopMusic()">Stop</button><br />
The tag button, as you might guess, creates a visible button on the screen. The text written on the button appears as the content between the opening and closing button tags. The onclick attribute delegates control to a function with the name contained in the value when the button is clicked. When the ‘Play’ button is clicked, control will be delegated to a function called ‘playMusic()’.
您可能会猜到,标记按钮会在屏幕上创建一个可见按钮。 写在按钮上的文本显示为打开和关闭按钮标签之间的内容。 当单击按钮时,onclick属性将控件委派给具有包含在值中的名称的函数。 单击“播放”按钮时,控件将委派给名为“ playMusic()”的函数。
I’m sure you’ve also noticed the <br /> tag. This tag creates a line break in the browser display. The forward slash follows that tag name because in this case our <br> tags serve as both opening and closing tags.
我确定您也注意到了<br />标签。 此标记在浏览器显示中创建换行符。 该标记名后跟正斜杠,因为在这种情况下,我们的<br>标记同时用作开始和结束标记。
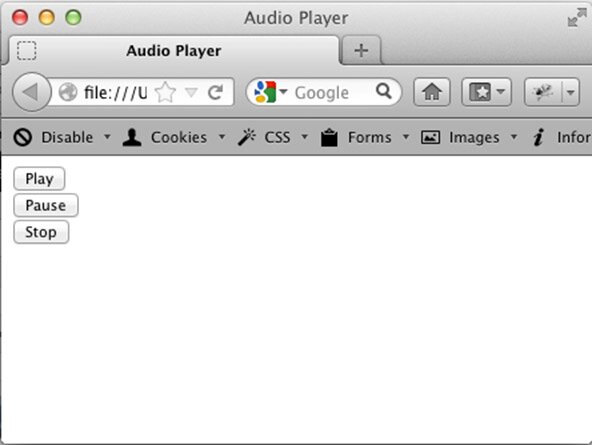
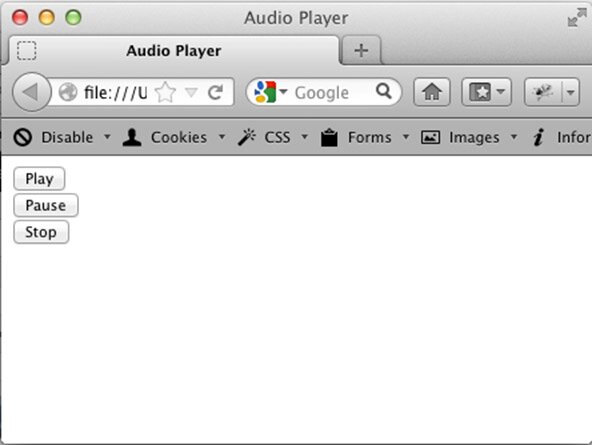
The Play, Pause and Stop buttons as they appear in the Firefox browser
出现在Firefox浏览器中的“播放”,“暂停”和“停止”按钮
6. Next we need to make a space for our Javascript functions. Generally, I put these either in the head of the file or in a separate, attached document. In this tutorial, I’ll be putting the Javascript in the head section of our document. In actual production work, I would attach a separate file; however, for the purposes of this tutorial, it’s simply more convenient to place the functions in the document head. Next, you’re going to put <script> tags after the <title> element in your HTML. After you have done that, your <head> section should appear like this:
6.接下来,我们需要为Javascript函数留出空间。 通常,我将它们放在文件的开头或单独的附加文档中。 在本教程中,我将把Javascript放在文档的开头。 在实际的生产工作中,我将附加一个单独的文件。 但是,出于本教程的目的,将功能放置在文档头中更加方便。 接下来,将在HTML中的<title>元素之后放置<script>标记。 完成之后,您的<head>部分应如下所示:
<head>
<title>Audio Player</title>
<script>
</script>
</head>
<head>
<title>Audio Player</title>
<script>
</script>
</head>
7. Now we’re going to be working inside the <script> element. Inside the script element, the browser knows to interpret the code as Javascript (not HTML). Inside the script tags, create the signature of the playMusic function. The signature is the skeleton of the function. Write code as follows:
7.现在,我们将在<script>元素中进行操作。 在script元素内部,浏览器知道将代码解释为Javascript(不是HTML)。 在脚本标签内,创建playMusic函数的签名。 签名是功能的框架。 编写代码如下:
<head>
<title>Audio Player</title>
<script>
function playMusic()
{
}
</script>
</head>
<head>
<title>Audio Player</title>
<script>
function playMusic()
{
}
</script>
</head>
The keyword function indicates that we are declaring the function called playMusic. The curly brackets contain the function code itself—often called the implementation. Now we’re going to add three more functions and declare a reference.
关键字function表示我们正在声明一个名为playMusic的函数。 大括号包含功能代码本身-通常称为实现。 现在,我们将添加三个功能并声明一个引用。
8. Modify the head one more time. Modify the code as follows:
8.再修改一次头部。 修改代码,如下所示:
<script>
var player;
window.onload = function()
{
}
function playMusic()
{
}
function pauseMusic()
{
}
function stopMusic()
{
}
</script>
<script>
var player;
window.onload = function()
{
}
function playMusic()
{
}
function pauseMusic()
{
}
function stopMusic()
{
}
</script>
Double-check your code to make sure you keyed it in correctly.
仔细检查您的代码,以确保正确键入。
There are a couple of new elements in this code block. The line that reads ‘var player’ declares a reference to a variable called player. In the next step we’re going to make that refer to the audio element in the HTML. The ‘window.οnlοad=function()’ declares an anonymous function that will be called when the browser loads. This will allow us to run some initialization code. Finally, we added the necessary function signatures for the pauseMusic and stopMusic functions.
此代码块中有几个新元素。 读取“ var player”的行声明了对称为player的变量的引用。 在下一步中,我们将引用HTML中的音频元素。 “ window.onload = function()”声明了一个匿名函数,该匿名函数将在浏览器加载时被调用。 这将使我们能够运行一些初始化代码。 最后,我们为pauseMusic和stopMusic函数添加了必要的函数签名。
9. Now we’re going to implement the functions. Edit your code in the <script> element so it appears as follows:
9.现在,我们将实现这些功能。 在<script>元素中编辑代码,使其显示如下:
<script>
var player;
window.onload = function()
{
player= document.getElementById('player');
}
function playMusic()
{
player.play();
}
function pauseMusic()
{
player.pause();
}
function stopMusic()
{
player.pause();
}
</script>
<script>
var player;
window.onload = function()
{
player= document.getElementById('player');
}
function playMusic()
{
player.play();
}
function pauseMusic()
{
player.pause();
}
function stopMusic()
{
player.pause();
}
</script>
We’re also going to need to make a change to the <audio> element itself in the HTML. Where it currently reads <audio>, make the following change:
我们还需要对HTML中的<audio>元素本身进行更改。 当前读取<audio>的位置,请进行以下更改:
<audio id="player">
<audio id="player">
We’ve actually done quite a bit of work here. Let’s first turn our attention to the anonymous function that runs after the ‘window.onload’ event. Where the variable player is declared in the first line of the script, the anonymous function assigns the audio element to this variable. Since the audio element now has the id player, we’re able to ‘get’ it with the getElementById(‘player’) function. If you’re coming from another programming language, it may be helpful to think of the Javascript variable player as a pointer to the audio element object.
实际上,我们在这里做了很多工作。 首先,我们将注意力转向在window.onload事件之后运行的匿名函数。 如果在脚本的第一行中声明了变量player,则匿名函数将audio元素分配给该变量。 由于音频元素现在具有id播放器,因此我们可以使用getElementById('player')函数“获取”它。 如果您来自另一种编程语言,那么将Javascript变量播放器视为指向音频元素对象的指针可能会有所帮助。
In the playMusic, pauseMusic and stopMusic functions, we used the player object to start and pause the music. You may be wondering why we didn’t use the stop command inside the stopMusic function. The explanation is simple—there is no stop command. More on this in a moment.
在playMusic,pauseMusic和stopMusic函数中,我们使用播放器对象开始和暂停音乐。 您可能想知道为什么我们没有在stopMusic函数中使用stop命令。 解释很简单-没有停止命令。 稍后对此进行更多讨论。
10. This is an excellent time to test your player. If you don’t have any typos or other errors, when you press Play, your music should play. Both the Pause button and the Stop button will stop the player in its current position. Pressing Play again will cause the song to continue. Now we’ll make a small change, which will allow the Stop button to function as expected.
10.这是测试玩家的绝佳时机。 如果没有任何错别字或其他错误,请按“播放”,然后播放音乐。 暂停按钮和停止按钮都可以将播放器停止在当前位置。 再按一次播放将使歌曲继续播放。 现在,我们将做一个小的更改,这将使“停止”按钮能够按预期的方式工作。
11. Edit your stopMusic function to appear as follows:
11.编辑stopMusic函数,使其显示如下:
function stopMusic()
{
player.pause();
player.currentTime = 0;
}
function stopMusic()
{
player.pause();
player.currentTime = 0;
}
Test your player again, and you will now notice that the Stop button will cause the song to start over if played again.
再次测试您的播放器,您现在会注意到,如果再次播放,“停止”按钮将使歌曲重新开始。
The currentTime property allows you to set or read the time elapsed in milliseconds. When we set that to zero, as we do here, we are essentially moving back to the beginning of the audio.
currentTime属性使您可以设置或读取经过的时间(以毫秒为单位)。 当我们将其设置为零时,就像我们在此处所做的那样,我们实际上是在移回到音频的开头。
12. Make sure you save and admire your hard work!
12.确保保存并欣赏您的辛勤工作!
翻译自: https://www.script-tutorials.com/mp3-player-with-html5-lesson-2/
带倍速播放的播放器