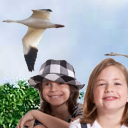
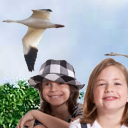
Multiple backgrounds with CSS3 and a little of animation. In CSS3 appear new possibility to use multiple backgrounds for the objects, in our new article I will demonstrate how to do it. And, I going to add a bit of JS code for simple animation (to make it not so boring). Commonly, it is very easy to apply multiple backgrounds – we just need to list them (separated by commas) in the object properties.
CSS3具有多个背景以及一些动画。 在CSS3中,出现了为对象使用多个背景的新可能性,在我们的新文章中,我将演示如何做到这一点。 而且,我将为简单的动画添加一些JS代码(使其不那么无聊)。 通常,应用多个背景非常容易-我们只需要在对象属性中列出它们(用逗号分隔)即可。
Here are samples and downloadable package:
以下是示例和可下载的软件包:
现场演示
[sociallocker]
[社交储物柜]
打包下载
[/sociallocker]
[/ sociallocker]
Ok, download the example files and lets start coding !
好的,下载示例文件并开始编码!
步骤1. HTML (Step 1. HTML)
As usual, we start with the HTML. This is source code of our sample:
和往常一样,我们从HTML开始。 这是我们示例的源代码:
index.html (index.html)
<html>
<head>
<title>Multiple backgrounds with CSS3 and a little of animation</title>
<link rel="stylesheet" type="text/css" href="css/main.css" />
<script type="text/javascript" src="js/main.js"></script>
</head>
<body>
<div class="example">
<div id="main_object"></div>
</div>
</body>
</html>
<html>
<head>
<title>Multiple backgrounds with CSS3 and a little of animation</title>
<link rel="stylesheet" type="text/css" href="css/main.css" />
<script type="text/javascript" src="js/main.js"></script>
</head>
<body>
<div class="example">
<div id="main_object"></div>
</div>
</body>
</html>
Nothing special, just <div id="main_object"></div> inside
没什么特别的,只是里面的<div id =“ main_object”> </ div>
步骤2. CSS (Step 2. CSS)
Here are single CSS file with all necessary styles:
这是具有所有必要样式的单个CSS文件:
css / main.css (css/main.css)
body{background:#eee;margin:0;padding:0}
.example{background:#FFF;width:950px;border:1px #000 solid;margin:20px auto;padding:15px;-moz-border-radius: 3px;-webkit-border-radius: 3px}
#main_object{
position:relative;width:950px;height:700px;overflow:hidden;cursor:pointer;
background-image: url(../images/layer1.png), url(../images/layer2.png), url(../images/layer3.png), url(../images/bg.jpg);
background-position: center bottom, right top, right bottom, left top;
background-repeat: no-repeat;
}
body{background:#eee;margin:0;padding:0}
.example{background:#FFF;width:950px;border:1px #000 solid;margin:20px auto;padding:15px;-moz-border-radius: 3px;-webkit-border-radius: 3px}
#main_object{
position:relative;width:950px;height:700px;overflow:hidden;cursor:pointer;
background-image: url(../images/layer1.png), url(../images/layer2.png), url(../images/layer3.png), url(../images/bg.jpg);
background-position: center bottom, right top, right bottom, left top;
background-repeat: no-repeat;
}
Here, you can see way how I pointing these several backgrounds to our main element – all comma separated. Same and for ‘background-position’ too. Of course, if you want – you always can use use ‘background’ property to set all necessary styles, in this case – you can enum your properties in same way, separating values with commas, example:
在这里,您可以看到如何将这几个背景指向我们的主要元素-所有逗号分隔。 相同,也适用于“背景位置”。 当然,如果需要–在这种情况下,您始终可以使用'background'属性设置所有必要的样式–您可以用相同的方式枚举属性,用逗号分隔值,例如:
background: url(../images/layer1.png) no-repeat center bottom, url(../images/layer2.png) no-repeat right top, url(../images/layer3.png) no-repeat right bottom, url(../images/bg.jpg) no-repeat left top;
}
background: url(../images/layer1.png) no-repeat center bottom, url(../images/layer2.png) no-repeat right top, url(../images/layer3.png) no-repeat right bottom, url(../images/bg.jpg) no-repeat left top;
}
步骤3. JS (Step 3. JS)
Here are a little of JS for our animation:
这是我们动画的一些JS:
js / main.js (js/main.js)
var ex76 = {
// variables
l1w : 358, // layer 1 width
l1h : 235, // layer 1 height
l2w : 441, // layer 2 width
l3w : 307, // layer 3 width
// inner variables
obj : 0,
xm : 0,
ym : 0,
x1 : 0,
y1 : 0,
x2 : 0,
x3 : 0,
// initialization
init : function() {
this.obj = document.getElementById('main_object');
this.x2 = this.obj.clientWidth;
this.x3 = -this.l3w;
this.run();
},
// refreshing mouse positions
mousemove : function(e) {
this.xm = e.clientX;
this.ym = e.clientY;
// recalculation new positions
this.x1 = this.xm - this.obj.offsetLeft - ex76.l1w/2;
this.y1 = this.ym - this.obj.offsetTop - ex76.l1h/2;
},
// loop function
run : function() {
// shifting second layer object to left
ex76.x2--;
if (ex76.x2 < -ex76.l2w) ex76.x2 = ex76.obj.clientWidth;
// shifting second layer object to right
ex76.x3++;
if (ex76.x3 > ex76.obj.clientWidth) ex76.x3 = -ex76.l3w;
// updating background-position value with new positions
ex76.obj.style.backgroundPosition = ex76.x1 + 'px ' + ex76.y1 + ', ' + ex76.x2 + 'px top, ' + ex76.x3 + 'px bottom, left top';
// loop
setTimeout(ex76.run, 20);
}
};
window.onload = function() {
ex76.init(); // initialization
// binding mouse move event
document.onmousemove = function(e) {
if (window.event)
e = window.event;
ex76.mousemove(e);
}
}
var ex76 = {
// variables
l1w : 358, // layer 1 width
l1h : 235, // layer 1 height
l2w : 441, // layer 2 width
l3w : 307, // layer 3 width
// inner variables
obj : 0,
xm : 0,
ym : 0,
x1 : 0,
y1 : 0,
x2 : 0,
x3 : 0,
// initialization
init : function() {
this.obj = document.getElementById('main_object');
this.x2 = this.obj.clientWidth;
this.x3 = -this.l3w;
this.run();
},
// refreshing mouse positions
mousemove : function(e) {
this.xm = e.clientX;
this.ym = e.clientY;
// recalculation new positions
this.x1 = this.xm - this.obj.offsetLeft - ex76.l1w/2;
this.y1 = this.ym - this.obj.offsetTop - ex76.l1h/2;
},
// loop function
run : function() {
// shifting second layer object to left
ex76.x2--;
if (ex76.x2 < -ex76.l2w) ex76.x2 = ex76.obj.clientWidth;
// shifting second layer object to right
ex76.x3++;
if (ex76.x3 > ex76.obj.clientWidth) ex76.x3 = -ex76.l3w;
// updating background-position value with new positions
ex76.obj.style.backgroundPosition = ex76.x1 + 'px ' + ex76.y1 + ', ' + ex76.x2 + 'px top, ' + ex76.x3 + 'px bottom, left top';
// loop
setTimeout(ex76.run, 20);
}
};
window.onload = function() {
ex76.init(); // initialization
// binding mouse move event
document.onmousemove = function(e) {
if (window.event)
e = window.event;
ex76.mousemove(e);
}
}
This is pretty easy – for second and third layer – I changing its X positions (by cycle), for first layer – I changing its position in place where located our mouse.
这很容易-对于第二层和第三层-我更改其X位置(按周期),对于第一层-我将其位置更改为鼠标所在的位置。
步骤4.图片 (Step 4. Images)
Also we need several images for our demo (for different layers):
另外,我们的演示需要一些图像(用于不同的层):
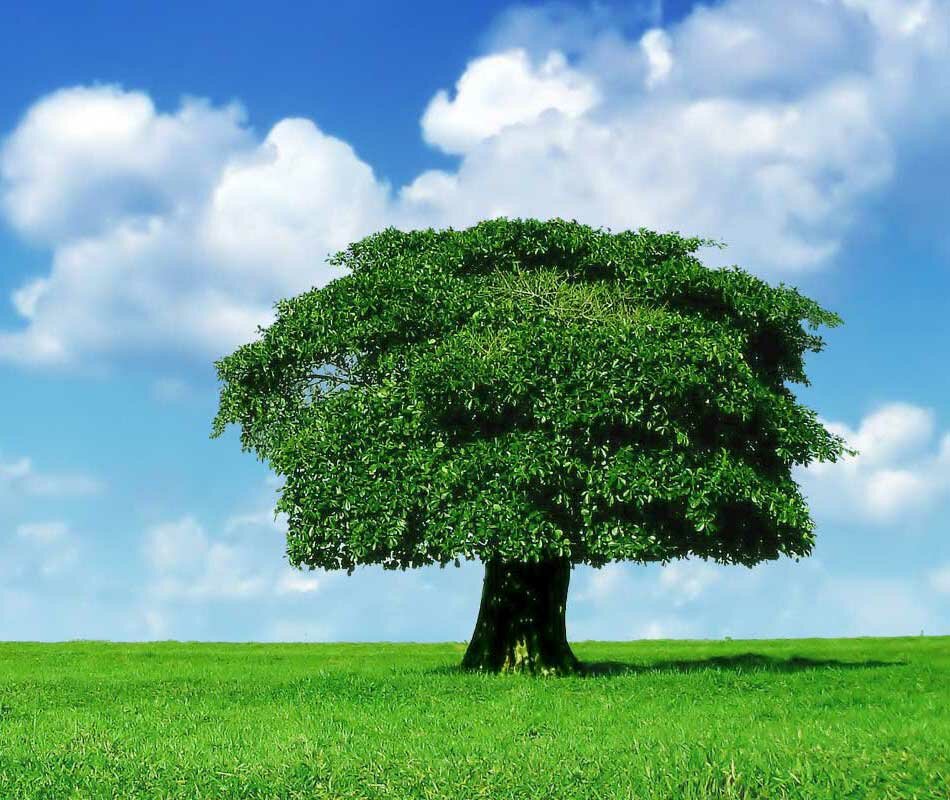
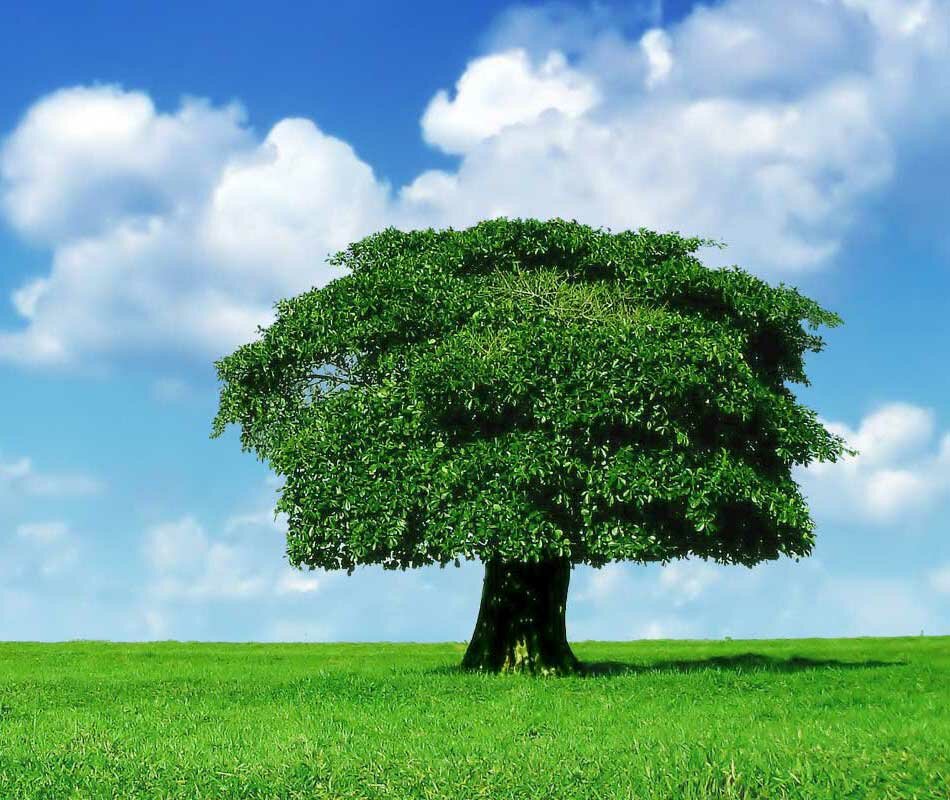
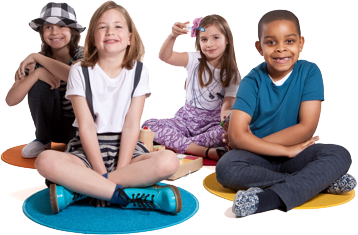
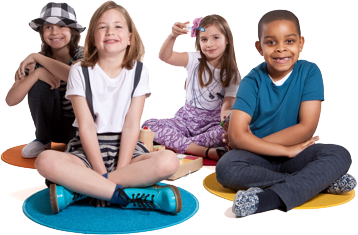
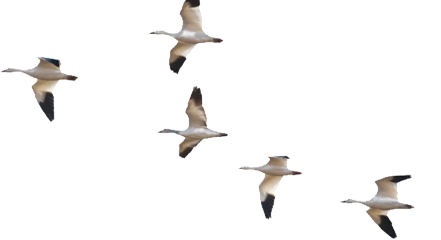
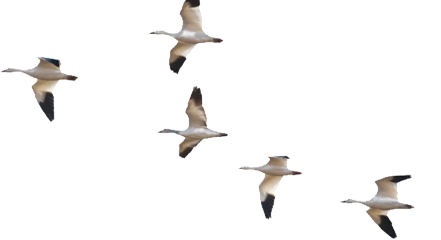
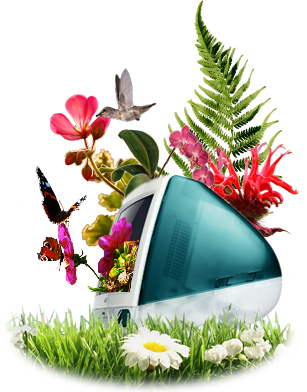
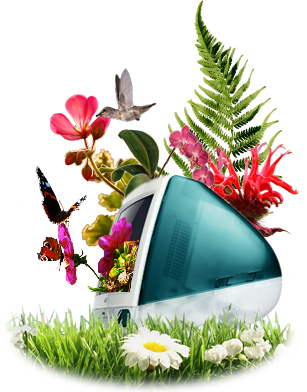
现场演示
结论 (Conclusion)
Today I told you how you can assign several images as background for single element. Hope that our demo gave you some ideas. Here are another application of this, you can create custom controls (buttons as example) with custom background, as example rounded buttons. You will need just set 2-3 layers (for beginning, center and end of button). Good luck!
今天,我告诉您如何分配多个图像作为单个元素的背景。 希望我们的演示给您一些想法。 这是此的另一个应用程序,您可以创建具有自定义背景的自定义控件(例如按钮),例如圆形按钮。 您只需要设置2-3层(用于按钮的开始,中心和结束)。 祝好运!
翻译自: https://www.script-tutorials.com/multiple-backgrounds-with-css3-and-a-little-of-animation/