This article was peer reviewed by Thomas Punt. Thanks to all of SitePoint’s peer reviewers for making SitePoint content the best it can be!
本文由Thomas Punt同行评审。 感谢所有SitePoint的同行评审人员使SitePoint内容达到最佳状态!
The PHP community has gone viral with the latest PHP 7 announcement and all the goodies it brings to the language. The PHP 7.1 release has more good news and features to offer. This article highlights the most important ones, and you should check PHP RFC for the full list.
最新PHP 7声明及其为语言带来的所有好处,使PHP社区Swift流行起来。 PHP 7.1发行版提供了更多的好消息和新功能。 本文重点介绍了最重要的内容,您应该查看PHP RFC的完整列表。
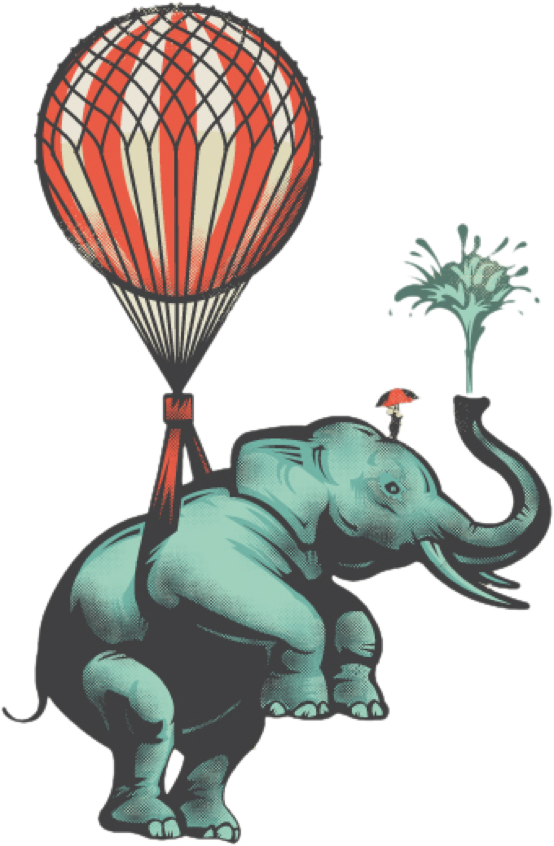
ArgumentCountError异常 (ArgumentCountError Exception)
Earlier versions of PHP allowed for function calls with fewer arguments than specified, emitting a warning when you have a missing argument.
PHP的早期版本允许使用比指定数量更少的参数的函数调用,当缺少参数时发出警告。
// PHP 5.6
function sum($a, $b)
{
return $a + $b;
}
sum();
// Warning: Missing argument 1 for sum()
// Warning: Missing argument 2 for sum()
sum(3);
// Warning: Missing argument 2 for sum()
sum(3, 4);
Warnings are not useful for this case, and the developer should check if all arguments are set correctly. In PHP 7.1, those warnings have been converted into an ArgumentCountError
exception.
警告在这种情况下没有用,开发人员应检查所有参数是否设置正确。 在PHP 7.1中,这些警告已转换为ArgumentCountError
异常。
// PHP 7.1
function sum($a, $b)
{
return $a + $b;
}
sum();
// Fatal error: Uncaught ArgumentCountError: Too few arguments to function sum(), 0 passed in /vagrant/index.php on line 18 and exactly 2 expected in /vagrant/index.php:13
sum(3); // skipped
sum(3, 4); // skipped
可空类型 (Nullable Types)
PHP 7 added type declarations for parameters and return types, but it seemed that something was missing! Nullable types enable for the specified type to take either that type or null. Here’s an example:
PHP 7为参数和返回类型添加了类型声明,但似乎缺少了一些东西! 可空类型使指定类型可以采用该类型或null。 这是一个例子:
function sum(int $a, int $b): ?int
{
return $a + $b;
}
The above function may return an integer or a null value, so if something wrong happens in the function’s logic you won’t be returning null as the wrong type. You can do the same for arguments, too!
上面的函数可能返回整数或null值,因此,如果函数逻辑中发生错误,您将不会以错误的类型返回null。 您也可以为参数做同样的事情!
function sum(?int $a, ?int $b): ?int
{
if ($a == null || $b == null) {
return null;
}
return $a + $b;
}
The unexpected thing is that calling the function without arguments will throw an exception!
出乎意料的是,不带参数调用该函数将引发异常!
var_dump(sum(null, null)); // NULL
var_dump(sum()); // throw ArgumentCountError exception
This means that you should explicitly specify arguments when they don’t have a default value.
这意味着您应该在没有默认值的情况下显式指定参数。
Another thing you should keep in mind is that when overriding or implementing methods, you can’t add the nullable type to the return type, but you’re allowed to remove it. And the reverse is true for arguments, you can’t remove the nullable type from arguments, but you’re allowed to add it!
您应该记住的另一件事是,在覆盖或实现方法时,不能将可为null的类型添加到返回类型中,但是可以将其删除。 对于参数而言,情况恰恰相反,您不能从参数中删除可为null的类型,但可以添加它!
interface Fooable {
function foo(): ?Fooable;
}
interface StrictFooable extends Fooable {
function foo(): Fooable; // valid
}
interface Fooable {
function foo(): Fooable;
}
interface LooseFooable extends Fooable {
function foo(): ?Fooable; // invalid
}
解构数组 (Destructuring Arrays)
We used to do the following when destructuring arrays.
解构数组时,我们通常执行以下操作。
list($a, $b, $c) = [1, 2, 3];
var_dump($a, $b, $c); // int(1) int(2) int(3)
But trying this would fail, because we couldn’t specify keys to extract and the function tried to use index keys.
但是尝试这样做将失败,因为我们无法指定要提取的键,并且该函数尝试使用索引键。
list($a, $b, $c) = ["a" => 1, "b" => 2, "c" => 3];
var_dump($a, $b, $c); // NULL NULL NULL
This RFC provides more control over array destructuring. The above code could be changed to this.
该RFC提供了对阵列解构的更多控制。 上面的代码可以更改为此。
list("a" => $a, "b" => $b, "c" => $c) = ["a" => 1, "b" => 2, "c" => 3];
var_dump($a, $b, $c); // int(1) int(2) int(3)
The list
function now accepts keys for array destructuring, and because this is used a lot, a new compact syntax has been introduced. Take this as an example.
list
函数现在接受用于数组解构的键,并且由于使用了很多键,因此引入了新的紧凑语法。 以这个为例。
["a" => $a, "b" => $b, "c" => $c] = ["a" => 1, "b" => 2, "c" => 3];
var_dump($a, $b, $c); // int(1) int(2) int(3)
Cool, isn’t it? And it also works on multidimensional arrays.
不错,不是吗? 它也适用于多维数组。
[[$a, $b], [$c, $d]] = [[1, 2], [3, 4]];
var_dump($a, $b, $c, $d); // int(1) int(2) int(3) int(4)
[["b" => $b], ["c" => $c]] = [["a" => 1, "b" => 2], ["c" => 3, "d" => 4]];
var_dump($b, $c); // int(2) int(3)
迭代类型 (Iterable Type)
The iterable
pseudo-type is added to join the array primitive type and the Traversable
interface which is used to make a value iterable. Take the below code as an example.
添加了iterable
伪类型,以将数组基本类型和Traversable
接口联接在一起,该接口用于使值可迭代。 以下面的代码为例。
// PHP 5.6
function dump(array $items)
{
var_dump($items);
}
dump([2, 3, 4]);
dump(new Collection());
array(3) {
[0]=>
int(2)
[1]=>
int(3)
[2]=>
int(4)
}
Catchable fatal error: Argument 1 passed to dump() must be of the type array, object given...
But in this case, the function won’t accept an iterable value and will throw an error. This new change lets you use iterable
to describe an iterable value instead of asserting it manually.
但是在这种情况下,该函数将不接受可迭代的值,并且将引发错误。 此新更改使您可以使用iterable
描述一个可迭代的值,而不用手动声明它。
// PHP 7.1
function dump(iterable $items)
{
var_dump($items);
}
dump([2, 3, 4]);
dump(new Collection());
array(3) {
[0]=>
int(2)
[1]=>
int(3)
[2]=>
int(4)
}
object(Collection)#2 (0) {
}
从可调用函数中关闭 (Closure From Callable Function)
The new fromCallable
method provides a performant and compact way of creating Closure objects from callables. Here’s an example:
新的fromCallable
方法提供了一种从可调用对象创建Closure对象的fromCallable
紧凑方式。 这是一个例子:
$callback = Closure::fromCallable([$this, 'fn']);
无效返回类型 (Void Return Type)
This is one of my favorite new features. It completes the return types feature introduced in PHP 7 where functions may not return anything and thus will be forced to return null!
这是我最喜欢的新功能之一。 它完成了PHP 7中引入的返回类型功能,该函数可能不返回任何内容,因此将被强制返回null!
function dump($object): void
{
var_dump($object);
}
A void function can omit the return statement or add an empty one (return;
).
void函数可以省略return语句或添加一个空语句( return;
)。
类常量可见性 (Class Constant Visibility)
This is a small change, but an essential part in OOP that enforces encapsulation. It adds visibility modifiers to class constants.
这是一个很小的变化,但是是OOP中强制封装的重要组成部分。 它将可见性修饰符添加到类常量。
class Post
{
protected const PUBLISHED = 1;
protected const DRAFT = 2;
protected const TRASHED = 3;
// ...
}
捕获多种异常类型 (Catching Multiple Exception Types)
Other languages like Java provide the ability to catch multiple exception types inside the same catch block, thus removing the need to duplicate code. Take the below code as an example:
其他语言(例如Java)提供了在同一个catch块中捕获多种异常类型的能力,从而消除了重复代码的需要。 以下面的代码为例:
// ...
try {
$user->payMonth($month);
} catch (UserSuspendedException $ex) {
DB::rollBack();
return redirect()
->back()
->withInput()
->withErrors([$ex->getMessage()]);
} catch (PaidMonthException $e) {
DB::rollBack();
return redirect()
->back()
->withInput()
->withErrors([$ex->getMessage()]);
}
// ...
You can remove the duplication by using the new catch block syntax:
您可以使用新的catch块语法删除重复项:
// ...
try {
$user->payMonth($month);
} catch (PaidMonthException | UserSuspendedException $ex) {
DB::rollBack();
return redirect()
->back()
->withInput()
->withErrors([$ex->getMessage()]);
}
// ...
无效的字符串算术 (Invalid String Arithmetics)
As you start learning PHP, you get an aha moment when doing arithmetics and you learn that strings are allowed! This is because PHP usually deals with the web and values are coming in as strings.
当你开始学习PHP的,你在做算术时,得到一个惊喜的时刻,你学到的字符串被允许! 这是因为PHP通常会处理Web,并且值以字符串形式出现。
// PHP 5.6
var_dump("1" + "2");
var_dump("1" + "a string");
var_dump("1" + "2 with a string");
int(3)
int(1)
int(3)
// PHP 7.1
var_dump("1" + "2");
var_dump("1" + "a string");
var_dump("1" + "2 with a string");
int(3)
Warning: A non-numeric value encountered in /vagrant/index.php on line 17
int(1)
Notice: A non well formed numeric value encountered in /vagrant/index.php on line 18
int(3)
Now we get a warning if a non numeric value is found, and a notice if the value is not well formatted (2 a string
= 2
).
现在,如果发现非数字值,则会收到警告,如果格式不正确( 2 a string
= 2
),则会发出通知。
结论 (Conclusion)
This list does not cover all the new changes, so be sure to check the RFC list for the full list. If you have a question or comment about the new PHP 7.1 features or PHP in general, please post them below!
此列表未涵盖所有新更改,因此请确保检查RFC列表以获取完整列表。 如果您对新PHP 7.1功能或一般PHP有疑问或意见,请在下面发布它们!
翻译自: https://www.sitepoint.com/whats-new-and-exciting-in-php-7-1/