symfony 2
Symfony has a very powerful authentication and authorization system, which can cater to a lot of our needs out of the box. FOSUserBundle is a library built on top of Symfony’s security system, which provides functionality such as registration, login, password resets, etc. It has built in support for MongoDB, as well as ORMs such as Doctrine, Propel, etc.
Symfony具有非常强大的身份验证和授权系统,可以立即满足我们的许多需求。 FOSUserBundle是一个基于Symfony安全系统构建的库,它提供诸如注册,登录,密码重置等功能。它内置了对MongoDB以及诸如Doctrine,Propel等ORM的支持。
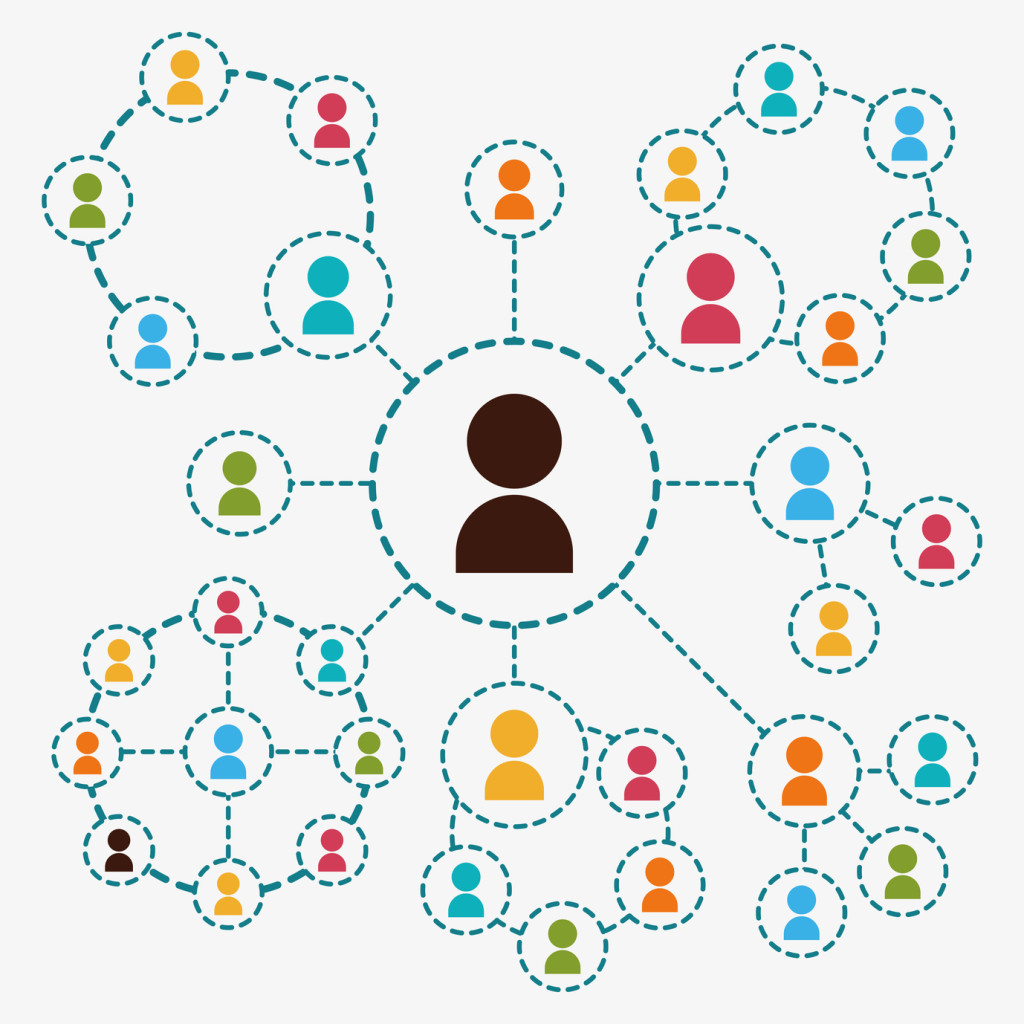
We will use Homestead Improved for our demo.
我们将使用Homestead改良版进行演示。
Let’s add a new site in the Homestead.yml
file.
让我们在Homestead.yml
文件中添加一个新站点。
sites:
- map: symfonylogin.app
to: /home/vagrant/Code/SymfonyLogin/web
type: symfony
databases:
- symfony
This will set up a new site in the VM that points to the web
folder of the Symfony application. When type: symfony
is specified, it will pick an Nginx configuration that is specifically created for Symfony applications.
这将在VM中设置一个新站点,该站点指向Symfony应用程序的web
文件夹。 当指定type: symfony
,它将选择专门为Symfony应用程序创建的Nginx配置。
We should also add 192.168.10.10 symfonylogin.app
into the main operating system’s /etc/hosts
file. Now we can boot the VM with vagrant up
.
我们还应该将192.168.10.10 symfonylogin.app
添加到主操作系统的/etc/hosts
文件中。 现在我们可以启动虚拟机vagrant up
。
Before we begin, let’s quickly set up the Symfony application using Symfony’s installer, a command line tool for installing Symfony projects. To install the installer, we enter the VM using vagrant ssh
and run the following commands.
在开始之前,让我们使用Symfony的安装程序(用于安装Symfony项目的命令行工具)快速设置Symfony应用程序。 要安装安装程序,我们使用vagrant ssh
输入VM并运行以下命令。
curl -LsS http://symfony.com/installer > symfony
sudo mv symfony /usr/local/bin/symfony
chmod a+x /usr/local/bin/symfony
The first command downloads the installer from the Symfony website to the current directory. Then, we move it to the bin directory and make it executable.
第一个命令将安装程序从Symfony网站下载到当前目录。 然后,将其移至bin目录并使其可执行。
Now let’s create a Symfony application inside the VM.
现在,让我们在VM内创建一个Symfony应用程序。
cd Code
symfony new SymfonyLogin
This will create a Symfony skeleton application and install all the dependencies from Packagist. Once it’s finished, we can go to the app/config
folder and update parameters.yml
with our database and email configuration values.
这将创建一个Symfony框架应用程序并安装Packagist的所有依赖项。 完成后,我们可以转到app/config
文件夹并使用数据库和电子邮件配置值更新parameters.yml
。
We can now open http://symfonylogin.app
in the browser to see the skeleton application running.
现在,我们可以在浏览器中打开http://symfonylogin.app
来查看框架应用程序正在运行。
Symfony restricts the development environment’s access to the IP 127.0.0.1
. When using Vagrant, you might need to update the app_dev.php
file to add your host IP to the list.
Symfony限制了开发环境对IP 127.0.0.1
的访问。 使用Vagrant时,您可能需要更新app_dev.php
文件以将主机IP添加到列表中。
基本用法 (Basic Usage)
FOSUserBundle can be easily plugged into Symfony projects with minimal configuration and code changes. Let’s see how we can do that in ours.
FOSUserBundle可以轻松地插入到Symfony项目中,而只需进行最少的配置和代码更改。 让我们看看如何在我们的网站上做到这一点。
安装捆绑包 (Installing the bundle)
First, we will install the package:
首先,我们将安装该软件包:
composer require friendsofsymfony/user-bundle "~2.0@dev"
组态 (Configuration)
Before we can use FOSUserBundle, we should register the bundle in AppKernel.
在使用FOSUserBundle之前,我们应该在AppKernel中注册该包。
class AppKernel extends Kernel
{
public function registerBundles()
{
$bundles = array(
...
new FOS\UserBundle\FOSUserBundle(),
);
...
return $bundles;
}
FOSUserBundle uses the Symfony translation component for displaying form labels and errors. By default, it is disabled in the configuration, and we need to make the following modification in config.yml
.
FOSUserBundle使用Symfony转换组件显示表单标签和错误。 默认情况下,它在配置中处于禁用状态,我们需要在config.yml
进行以下修改。
translator: { fallbacks: ["%locale%"] }
Now let us configure the security in security.yml
.
现在让我们在security.yml中配置安全security.yml
。
security:
encoders:
AppBundle\Entity\User: bcrypt
role_hierarchy:
ROLE_ADMIN: ROLE_USER
ROLE_SUPER_ADMIN: ROLE_ADMIN
providers:
fos_userbundle:
id: fos_user.user_provider.username
firewalls:
main:
pattern: ^/
form_login:
provider: fos_userbundle
csrf_provider: security.csrf.token_manager
logout: true
anonymous: true
access_control:
- { path: ^/login$, role: IS_AUTHENTICATED_ANONYMOUSLY }
- { path: ^/register, role: IS_AUTHENTICATED_ANONYMOUSLY }
- { path: ^/resetting, role: IS_AUTHENTICATED_ANONYMOUSLY }
- { path: ^/admin/, role: ROLE_ADMIN }
Symfony’s security component provides a number of configuration options which will let us configure pretty much every aspect. Here we have specified the only options that we need to change and left everything else at the default value. Let’s see what we have changed.
Symfony的安全组件提供了许多配置选项,这使我们几乎可以在各个方面进行配置。 在这里,我们指定了唯一需要更改的选项,并将所有其他选项保留为默认值。 让我们看看我们已经改变了。
encoders:
AppBundle\Entity\User: bcrypt
encoders
will tell the application which algorithm to use to encode passwords for the User object. This is our User entity class which we will create in the steps below.
encoders
将告诉应用程序使用哪种算法来编码User对象的密码。 这是我们的用户实体类,将在以下步骤中创建。
providers:
fos_userbundle:
id: fos_user.user_provider.username
This will register the user provider where fos_user.user_provider.username
is the ID of the service FOS\UserBundle\Security\UserProvider
registered in FOS User Bundle.
这将注册用户提供程序,其中fos_user.user_provider.username
是在FOS用户捆绑包中注册的服务FOS\UserBundle\Security\UserProvider
的ID。
firewalls
in Symfony is analogous to the firewalls that we are familiar with. We have created one firewall named main
. The pattern
key specifies the url patterns to which this firewall should match. The key form_login
specifies that we will be using a login form to authenticate users and we want to use our fos_userbundle
as the user provider.
firewalls
在Symfony的是类似于我们熟悉的防火墙。 我们创建了一个名为main
防火墙。 pattern
密钥指定此防火墙应匹配的URL模式。 密钥form_login
指定我们将使用登录表单来验证用户身份,并且我们希望将fos_userbundle
用作用户提供者。
access_control
limits access to URLs based on user roles. Login, Registration and Resetting pages should be accessible publicly, so they require the IS_AUTHENTICATED_ANONYMOUSLY
role, which is given by default to anyone accessing any page.
access_control
根据用户角色限制对URL的访问。 登录,注册和重置页面应该可以公开访问,因此它们需要IS_AUTHENTICATED_ANONYMOUSLY
角色,默认情况下,访问任何页面的任何人都将获得该角色。
用户类 (The User class)
FOSUserBundle requires us to create a User entity that needs to be persisted into the database. The bundle already provides an abstract base class with most of the fields that we need. We just need to create the entity that extends this base class.
FOSUserBundle要求我们创建一个需要持久存储到数据库中的用户实体。 该捆绑包已经提供了一个抽象基类,其中包含我们需要的大多数字段。 我们只需要创建扩展此基类的实体即可。
<?php
// src/AppBundle/Entity/User.php
namespace AppBundle\Entity;
use FOS\UserBundle\Model\User as BaseUser;
use Doctrine\ORM\Mapping as ORM;
/**
* User
*
* @ORM\Table("fos_user")
* @ORM\Entity
*/
class User extends BaseUser
{
/**
* @var integer
*
* @ORM\Column(name="id", type="integer")
* @ORM\Id
* @ORM\GeneratedValue(strategy="AUTO")
*/
protected $id;
/**
* Get id
*
* @return integer
*/
public function getId()
{
return $this->id;
}
}
The base class doesn’t have an ID field, so we should have that in our Entity along with any other fields that we need for our application.
基类没有ID字段,因此我们应该将其与我们应用程序所需的任何其他字段一起放在Entity中。
After creating the Entity, we need to tell the application to use this as the user class. There are three fields required by FOSUserBundle that we should specify in config.yml
. We should add the following settings to the end of config.yml
.
创建实体后,我们需要告诉应用程序将其用作用户类。 FOSUserBundle需要三个字段,我们应该在config.yml
指定。 我们应该在config.yml
的末尾添加以下设置。
fos_user:
db_driver: orm
firewall_name: main
user_class: AppBundle\Entity\User
db_driver : orm
will tell the bundle to use Doctrine.
db_driver : orm
会告诉捆绑软件使用Doctrine。
firewall_name : main
specifies the name of the firewall to use.
firewall_name : main
指定要使用的防火墙的名称。
user_class: AppBundle\Entity\User
tells the application that we want to use AppBundle\Entity\User
as the user class.
user_class: AppBundle\Entity\User
告诉应用程序我们要使用AppBundle\Entity\User
作为用户类。
更新架构 (Update Schema)
Once we create the Entity, we can also generate the user table using Symfony’s CLI.
创建实体后,我们还可以使用Symfony的CLI生成用户表。
php app/console doctrine:schema:update --force
This will create a table named fos_user in our database, with the fields available from FOSUserBundle. If you need any additional fields, you can specify them in the User entity before you generate the schema.
这将在我们的数据库中创建一个名为fos_user的表,其中的字段可从FOSUserBundle获取。 如果需要任何其他字段,则可以在生成架构之前在User实体中指定它们。
进口路线 (Import routes)
Symfony doesn’t import vendor routes by itself. Instead, we need to manually add them in app/config/routing.yml
.
Symfony不会自行导入供应商路由。 相反,我们需要在app/config/routing.yml
手动添加它们。
fos_user:
resource: "@FOSUserBundle/Resources/config/routing/all.xml"
This will import all the routes available in FOSUserBundle
.
这将导入FOSUserBundle
可用的所有路由。
Now that we have set up the bundle and configured everything, we can check if it is working. If we go to http://symfonylogin.app/register/
, we should see a registration form like this one.
现在我们已经设置了捆绑包并配置了所有内容,我们可以检查捆绑包是否正常工作。 如果转到http://symfonylogin.app/register/
,我们应该看到这样的注册表格。
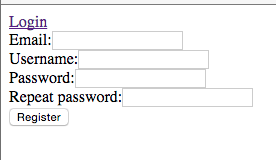
自定义模板 (Customizing Templates)
We now have a working but basic user registration and login system. The form looks too naive for use in any real projects. Next, we will see how we can customize the templates to look better.
现在,我们有了一个有效但基本的用户注册和登录系统。 该表格看起来太幼稚,无法在任何实际项目中使用。 接下来,我们将看到如何自定义模板以使其看起来更好。
Symfony’s powerful templating system allows us to easily extend and override templates that come with bundles. In order to override the bundle templates, we must create a directory under app/Resources
and give it the name of the bundle. Then, we create a views
directory and place any templates that do overriding in there.
Symfony强大的模板系统使我们能够轻松扩展和覆盖捆绑软件随附的模板。 为了覆盖包模板,我们必须在app/Resources
下创建一个目录,并为其指定包的名称。 然后,我们创建一个views
目录,并在其中放置所有要覆盖的模板。
If we check the location vendor/friendsofsymfony/user-bundle/Resources/views
, we will find all the templates used by FOSUserBundle. We need to create the directory structure FOSUserBundle/views/
under app/Resources/
and place our template files there. Let’s copy the Registration
directory from FOSUserBundle
to this location. Now, our app/Resources
directory should look something like this.
如果我们检查位置vendor/friendsofsymfony/user-bundle/Resources/views
,则会找到FOSUserBundle使用的所有模板。 我们需要在app/Resources/
下创建目录结构FOSUserBundle/views/
并将模板文件放置在此处。 让我们将Registration
目录从FOSUserBundle
复制到此位置。 现在,我们的app/Resources
目录应如下所示。
// ProjectRoot/app/Resources
├── FOSUserBundle
│ └── views
│ └── Registration
│ ├── checkEmail.html.twig
│ ├── confirmed.html.twig
│ ├── email.txt.twig
│ ├── register.html.twig
│ └── register_content.html.twig
└── views
├── base.html.twig
└── default
└── index.html.twig
Now we can update register.html.twig
based on our requirements.
现在,我们可以根据需求更新register.html.twig
。
{% extends "base.html.twig" %}
{% block body %}
<div class="row">
<div class="col s12 m8 offset-m2">
<h4 class="card-panel teal lighten-2 white-head">Signup</h4>
</div>
</div>
<div class="row">
<div class="col s12 m8 offset-m2">
{% include "FOSUserBundle:Registration:register_content.html.twig" %}
</div>
</div>
{% endblock body %}
Here I assume you already have a layout file base.html.twig for your application, with body content rendered into the body
block.
在这里,我假设您已经为您的应用程序提供了布局文件base.html.twig ,并将正文内容呈现到body
块中。
We will also change register_content.html.twig
so that we can display the form elements as we wish.
我们还将更改register_content.html.twig
以便我们可以根据需要显示表单元素。
{% trans_default_domain 'FOSUserBundle' %}
{{form_start(form, {'method': 'POST', 'attr':{'class': 'register'}})}}
<div class="row">
<div class="input-field col s12">
{{ form_label(form.username) }}
{{ form_widget(form.username) }}
</div>
{{ form_errors(form.username) }}
</div>
<div class="row no-padding">
<div class="input-field col s12">
{{ form_label(form.email) }}
{{ form_widget(form.email) }}
</div>
{{ form_errors(form.email) }}
</div>
<div class="row no-padding">
<div class="input-field col s12">
{{ form_label(form.plainPassword.first) }}
{{ form_widget(form.plainPassword.first) }}
</div>
{{ form_errors(form.plainPassword.first) }}
</div>
<div class="row no-padding">
<div class="input-field col s12">
{{ form_label(form.plainPassword.second) }}
{{ form_widget(form.plainPassword.second) }}
</div>
{{ form_errors(form.plainPassword.second) }}
</div>
<div align="center" class="button-wrap">
<button class="btn-large waves-effect waves-light" type="submit" name="action">
{{ 'registration.submit'|trans }}
<i class="material-icons">send</i>
</button>
</div>
{{ form_end(form) }}
form_start
will open a form tag with the given attributes and form_end
will close that form. If we check the actual template, we will see {{ form_widget(form) }}
only, which will render the entire form. But since we need our own styles for form elements, we are rendering individual form elements using form_label
and form_widget
. If any element fails the validation when the form is submitted, form_errors
will render the error message for the corresponding field.
form_start
将打开具有给定属性的表单标签,而form_end
将关闭该表单。 如果我们检查实际模板,则只会看到{{ form_widget(form) }}
,它将呈现整个表单。 但是由于表单元素需要自己的样式,因此我们使用form_label
和form_widget
渲染单个表单元素。 如果在提交表单时任何元素未能通过验证,则form_errors
将呈现相应字段的错误消息。
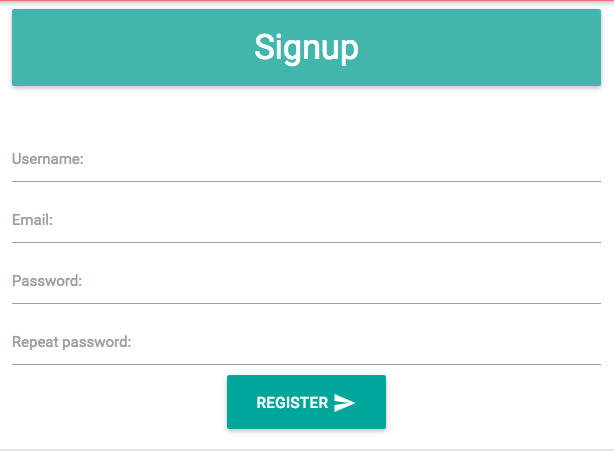
We can customize all the other templates to match rest of the application. Likewise, if we want to customize the labels and other messages, we can copy the translations
directory from FOSUserBundle and place it in our app/Resources/FOSUserBundle
directory. Then, we edit FOSUserBundle.en.yml
and change any values we need.
我们可以自定义所有其他模板以匹配应用程序的其余部分。 同样,如果要自定义标签和其他消息,则可以从FOSUserBundle复制translations
目录并将其放置在我们的app/Resources/FOSUserBundle
目录中。 然后,我们编辑FOSUserBundle.en.yml
并更改我们需要的任何值。
注册确认 (Registration Confirmation)
In most cases, we need the user to confirm their email address before completing the registration process. This can be easily done by updating the fos_user
configuration in config.yml
. Now it should look something like this.
在大多数情况下,我们需要用户在完成注册过程之前确认其电子邮件地址。 这可以通过更新来轻松完成fos_user
在配置config.yml
。 现在看起来应该是这样。
fos_user:
db_driver: orm
firewall_name: main
user_class: AppBundle\Entity\User
from_email:
address: admin@example.com
sender_name: Example.com
registration:
confirmation:
enabled: true
template: FOSUserBundle:Registration:email.txt.twig
Notice the new registration
key we have added here. enabled: true
for confirmation
will enable the confirmation process and we are using FOSUserBundle:Registration:email.txt.twig
template for the email content.
请注意我们在此处添加的新registration
密钥。 enabled: true
为confirmation
将启用确认过程,我们正在使用FOSUserBundle:Registration:email.txt.twig
模板作为电子邮件内容。
Also note the from_email
key that we have added. It will tell the bundle to use this email as the from address for all emails we are sending.
还要注意我们添加的from_email
键。 它将告诉分发包将此电子邮件用作我们发送的所有电子邮件的发件人地址。
Now, users trying to register will get a confirmation email with a link to confirm their email address.
现在,尝试注册的用户将收到带有链接的确认电子邮件,以确认其电子邮件地址。
For sending emails from Symfony, make sure you have a working smtp configuration in the parameters.yml
file.
要从Symfony发送电子邮件,请确保您在parameters.yml
文件中具有有效的smtp配置。
登录 (Login)
So far, we have seen how to set up the registration form and customize the templates. Logging in doesn’t need any additional setup, the built in functionality is enough for any application. If we navigate to http://symfonylogin.app/login
, we will see the login form. If we are already logged in, we will also see a logout link in the default template.
到目前为止,我们已经了解了如何设置注册表单和自定义模板。 登录不需要任何其他设置,内置功能足以满足任何应用程序的需要。 如果导航到http://symfonylogin.app/login
,则会看到登录表单。 如果我们已经登录,我们还将在默认模板中看到一个注销链接。
Symfony’s security system intercepts the login form submission and tries to authenticate the user using the authentication provider that we have specified in the security configuration. Most of this is done by Symfony’s security component itself, documented here.
Symfony的安全系统拦截登录表单提交,并尝试使用我们在安全配置中指定的身份验证提供程序对用户进行身份验证。 大部分操作是由Symfony的安全组件本身完成的, 在此处进行了说明 。
忘记密码 (Forgot Password)
FOSUserBundle also provides the ability to reset a password. Users can navigate to http://symfonylogin.app/resetting/request
and provide their username or email to reset their password. If that user exists, the application will send an email to the registered email address.
FOSUserBundle还提供了重置密码的功能。 用户可以导航至http://symfonylogin.app/resetting/request
并提供其用户名或电子邮件以重置其密码。 如果该用户存在,则应用程序将向已注册的电子邮件地址发送电子邮件。
用户资料 (User Profile)
FOSUserBundle comes with a basic profile page. Once logged in, if we navigate to http://symfonylogin.app/profile/
, we will see the default profile page. Of course, this page also needs to be customized for use in any non-default projects.
FOSUserBundle带有一个基本的配置文件页面。 登录后,如果我们导航到http://symfonylogin.app/profile/
,我们将看到默认的配置文件页面。 当然,还需要自定义此页面以用于任何非默认项目。
{% trans_default_domain 'FOSUserBundle' %}
{% extends "base.html.twig" %}
{% block body %}
<div class="fos_user_user_show">
<p>{{ 'profile.show.username'|trans }}: {{ user.username }}</p>
<p>{{ 'profile.show.email'|trans }}: {{ user.email }}</p>
</div>
<a href="{{ path('fos_user_profile_edit') }}">Edit Profile</a>
{% endblock body %}
The user
object holds the details of the currently logged in user. Right now, we have only the username and email available in the database.
user
对象保存当前登录用户的详细信息。 现在,数据库中只有可用的用户名和电子邮件。
We can also update the profile from the profile edit page (http://symfonylogin.app/profile/edit
). For added security, the user should provide the current password to update the profile.
我们还可以从个人资料编辑页面( http://symfonylogin.app/profile/edit
)更新个人资料。 为了提高安全性,用户应提供当前密码来更新配置文件。
摘要 (Summary)
FOSUserBundle is a plug-in for user registration and management in Symfony applications. Built on top of Symfony’s security system, this bundle takes care of most if not all the complexity of Symfony’s security when implementing user registration and login.
FOSUserBundle是用于Symfony应用程序中的用户注册和管理的插件。 构建在Symfony安全系统之上,该捆绑包在实施用户注册和登录时可以处理Symfony安全的大部分(即使不是全部)复杂性。
For more information, check out the extensive documentation to learn more about this Bundle.
有关更多信息,请查看大量文档以了解有关此捆绑软件的更多信息。
The code for this tutorial is available on Github. Let us know if you have any questions or comments!
Github上提供了本教程的代码。 让我们知道您是否有任何疑问或意见!
翻译自: https://www.sitepoint.com/basic-user-management-in-symfony2-with-fosuserbundle/
symfony 2