The Hypertext Transfer Protocol (HTTP) is inarguably the most significant protocol used on the Internet today.
超文本传输协议(HTTP)无疑是当今Internet上使用的最重要的协议。
Web services, network enabled appliances and the growth of network computing continues to expand the role of the HTTP protocol beyond user driven web browsers, while increasing the number of applications that require HTTP support.
Web服务,具有网络功能的设备以及网络计算的增长继续将HTTP协议的作用扩展到用户驱动的Web浏览器之外,同时增加了需要HTTP支持的应用程序的数量。
In recent times, companies offering products or services to end-users are ‘APIfying’ their websites and/or applications.
近年来,向最终用户提供产品或服务的公司正在“ APIfying”他们的网站和/或应用程序。
An API – Application Programming Interface – at its most basic level allows product or service to talk to other products or services.
API(应用程序编程接口)在其最基本的级别上允许产品或服务与其他产品或服务对话。
In this way, an API allows you to open up data and functionality to other developers, to other businesses or even between departments and locations within your company.
通过这种方式,API允许您向其他开发人员,其他业务甚至公司内部的部门和位置之间开放数据和功能。
It is increasingly the way in which companies exchange data, services and complex resources, both internally, externally with partners, and openly with the public.
公司越来越在内部,外部与合作伙伴以及与公众公开地交换数据,服务和复杂资源。
Credit: API Evangelist
图片来源: API传播者
Within PHP, there are many possible ways for sending HTTP requests. Examples include file_get_contents, fsockopen, and cURL.
在PHP中,有许多发送HTTP请求的可能方法。 示例包括file_get_contents , fsockopen和cURL 。
Prior to WordPress 2.7, plugin developers all had their own implementation for sending HTTP requests and receiving the response which was an added burden to them because they had to support it afterwards to keep them working.
在WordPress 2.7之前,插件开发人员都有自己的实现,用于发送HTTP请求和接收响应,这给他们增加了负担,因为他们后来必须支持它才能使它们正常工作。
The WordPress HTTP API was created to standardize a single API that handled everything with regards to HTTP as simply as possible. The HTTP API supports various PHP HTTP transports or implementations to cater for different hosting environments and configurations.
创建WordPress HTTP API是为了标准化单个API,该API尽可能简单地处理与HTTP有关的所有问题。 HTTP API支持各种PHP HTTP传输或实现,以适应不同的托管环境和配置。
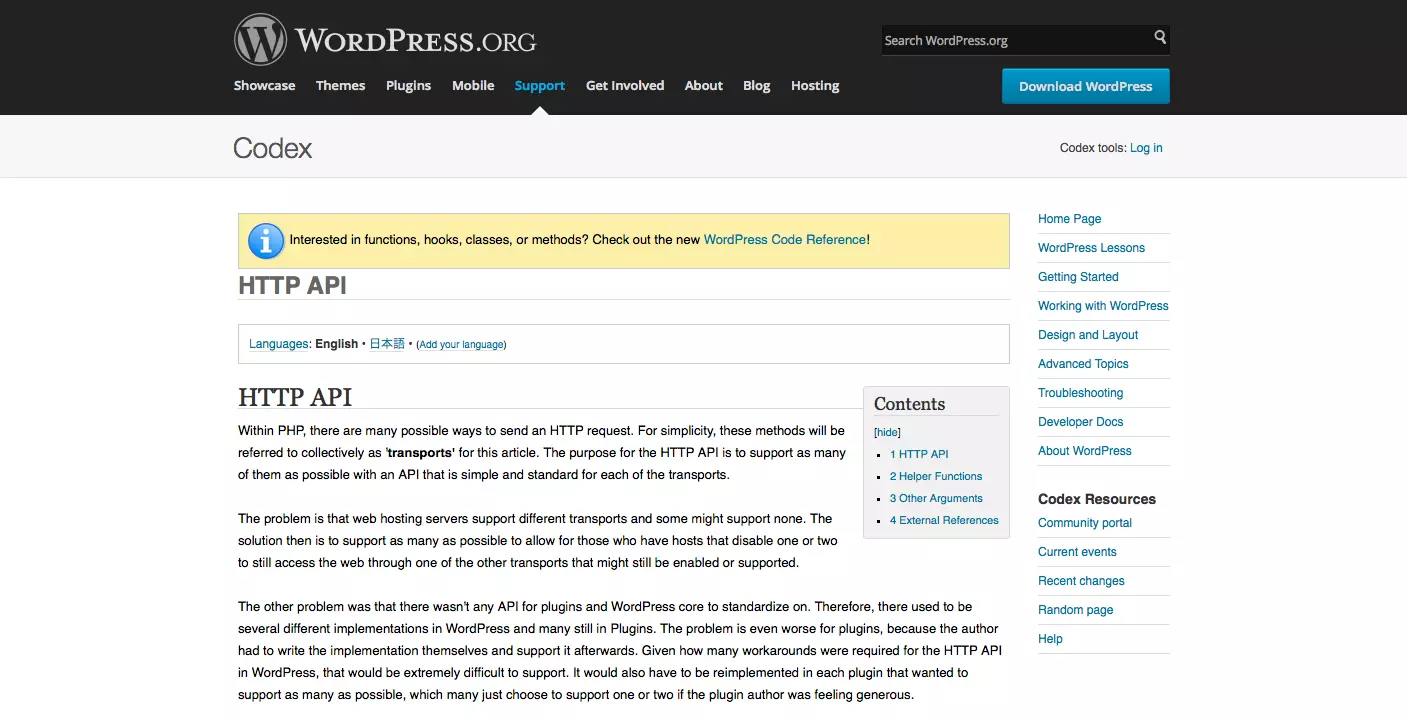
使用HTTP API发送请求 (Sending Requests with the HTTP API)
To send or make a request to a web service or API using the WordPress HTTP API, the following helper functions come in handy.
要使用WordPress HTTP API向Web服务或API发送请求或向Web服务或API发出请求,以下辅助功能将派上用场。
wp_remote_get() – send HTTP GET method requests.
wp_remote_get() –发送HTTP GET方法请求。
wp_remote_post() – send HTTP POST requests.
wp_remote_post() –发送HTTP POST请求。
wp_remote_head() – send HTTP HEAD requests.
wp_remote_head() –发送HTTP HEAD请求。
wp_remote_request() – send requests using any custom HTTP method, be it GET, POST, HEAD, PUT or DELETE.
wp_remote_request() –使用任何自定义HTTP方法发送请求,无论是GET,POST,HEAD,PUT还是DELETE。
Soon, we’ll go over the above functions and see how they work.
很快,我们将介绍上述功能,并查看它们如何工作。
For the purpose of this tutorial, all requests will be made to httpbin.org – an HTTP Request & Response Service.
就本教程而言,所有请求都将发送到httpbin.org (一个HTTP请求和响应服务)。
基本范例 (Basic Examples)
wp_remote_get( $url, $args )
The function above is used for sending a GET request. It has two parameters: the URL to be acted upon or endpoint ( $url
) and an array of argument details ( $args
).
上面的函数用于发送GET请求。 它有两个参数:要作用的URL或端点( $url
)和参数详细信息数组( $args
)。
Let’s see some examples to have an understanding of these functions.
让我们看一些例子来理解这些功能。
$url = 'http://httpbin.org/get?a=b&c=d';
$response = wp_remote_get( $url );
All the code does is send a GET request to the endpoint http://httpbin.org/get
where the query string are the GET parameters ?a=b&c=d
所有代码所做的就是将GET请求发送到端点http://httpbin.org/get
,其中查询字符串是GET参数?a=b&c=d
Using PHP print_r function to print the HTTP data ($response
) in a human-readable format reveals the following:
使用PHP print_r函数以人类可读的格式打印HTTP数据( $response
)会显示以下内容:
Array
(
[headers] => Array
(
[access-control-allow-credentials] => true
[access-control-allow-origin] => *
[content-type] => application/json
[date] => Mon, 22 Sep 2014 15:46:40 GMT
[server] => gunicorn/18.0
[content-length] => 407
[connection] => Close
)
[body] => {
"args": {
"a": "b",
"c": "d"
},
"headers": {
"Accept": "*/*",
"Accept-Encoding": "deflate;q=1.0, compress;q=0.5, gzip;q=0.5",
"Connection": "close",
"Host": "httpbin.org",
"User-Agent": "WordPress/4.0; http://localhost/blog",
"X-Request-Id": "bb98282e-f428-48c8-a7d4-07660a70cf37"
},
"origin": "41.203.67.131",
"url": "http://httpbin.org/get?a=b&c=d"
}
[response] => Array
(
[[code]] => 200
[message] => OK
)
[cookies] => Array
(
)
[filename] =>
)
Taking a closer look at the human readable information, you will see the response is a multi-dimensional array broken into five parts: headers, body, response, cookies and filename.
仔细研究人类可读的信息,您将看到响应是一个多维数组,分为五个部分:标头,正文,响应,cookie和文件名。
The
headers
contain the HTTP header fields of the request and response.headers
包含请求和响应的HTTP标头字段。body
is the response message sent by the API server or web service.body
是API服务器或Web服务发送的响应消息。response
contains the request HTTP status code.response
包含请求的HTTP状态代码 。cookies
contains the cookies set by the web service or endpoint server if present.cookies
包含由Web服务或端点服务器(如果存在)设置的cookie。filename
contains the location or path of a file being sent to an API endpoint. This can be done for example by a POST request.filename
包含要发送到API端点的文件的位置或路径。 例如,这可以通过POST请求来完成。
Let’s assume you want to inform the API you are sending a request so that the content body being sent is a JSON object, including the Content-type
header in the array of argument function parameter as follows does the trick.
假设您想通知API您正在发送请求,以使正在发送的内容主体为JSON对象,包括在参数函数parameter数组中的Content-type
标头,如下所示。
$url = 'http://httpbin.org/get?a=b&c=d';
$args = array(
'headers' => array( "Content-type" => "application/json" )
);
$response = wp_remote_get( $url, $args );
To conveniently retrieve the different parts of the response and also test for any resultant error, the WordPress HTTP API provides the following helper functions:
为了方便地检索响应的不同部分并测试任何结果错误,WordPress HTTP API提供了以下帮助器功能:
wp_remote_retrieve_body() – Retrieves just the body from the response.
wp_remote_retrieve_body() –仅从响应中检索正文。
wp_remote_retrieve_headers() – Returns an array of all the response HTTP headers.
wp_remote_retrieve_headers() –返回所有响应HTTP标头的数组。
wp_remote_retrieve_header() – Returns the value of an HTTP header based on the supplied name.
wp_remote_retrieve_header() –根据提供的名称返回HTTP标头的值。
wp_remote_retrieve_response_code() – Returns the response status codes of the HTTP request.
wp_remote_retrieve_response_code() –返回HTTP请求的响应状态代码。
To retrieve only the response body of the GET request to http://httpbin.org/get
, the wp_remote_retrieve_body()
(which accepts the response as an argument) comes in handy.
要仅检索对http://httpbin.org/get
的GET请求的响应正文, wp_remote_retrieve_body()
(接受响应作为参数)非常方便。
$url = 'http://httpbin.org/get?a=b&c=d';
$args = array(
'headers' => array( "Content-type" => "application/json" )
);
$response = wp_remote_get( $url, $args );
$body = wp_remote_retrieve_body( $response );
To retrieve only the response header, use the wp_remote_retrieve_headers()
as follows.
要仅检索响应头,请按如下所示使用wp_remote_retrieve_headers()
。
$response_headers = wp_remote_retrieve_headers( $response );
print_r( $response_headers );
Result:
结果:
Array
(
[access-control-allow-credentials] => true
[access-control-allow-origin] => *
[content-type] => application/json
[date] => Mon, 22 Sep 2014 19:25:57 GMT
[server] => gunicorn/18.0
[content-length] => 448
[connection] => Close
)
To return the value of a single header say content-type
, the function wp_remote_retrieve_header()
is used.
要返回单个标头(例如content-type
wp_remote_retrieve_header()
值,请使用函数wp_remote_retrieve_header()
。
$response_header = wp_remote_retrieve_header( $response, 'content-type' );
print_r( $response_header );
Result:
结果:
application/json
To retrieve only the response status code, use the wp_remote_retrieve_response_code()
function.
要仅检索响应状态代码,请使用wp_remote_retrieve_response_code()
函数。
$response_code = wp_remote_retrieve_response_code( $response );
print_r( $response_code );
While wp_remote_retrieve_response_code()
retrieves the response status code, wp_remote_retrieve_response_message
retrieves the status message.
wp_remote_retrieve_response_code()
检索响应状态代码时, wp_remote_retrieve_response_message
检索状态消息。
Below is a header response returned by an HTTP request.
以下是HTTP请求返回的标头响应。
[response] => Array
(
[[code]] => 200
[message] => OK
)
The former will returns 200
(the status code) and the latter OK
(the status message).
前者将返回200
(状态代码),而后者将返回OK
(状态消息)。
So far, we’ve overviewed the various helper functions provided by the WordPress HTTP API, how to make GET request via wp_remote_get()
and retrieving the response headers and body using the response helper functions.
到目前为止,我们已经概述了WordPress HTTP API提供的各种帮助程序功能,如何通过wp_remote_get()
发出GET请求以及如何使用响应帮助程序功能检索响应标头和正文。
POST请求 (POST Requests)
The function wp_remote_post()
is use for sending POST requests.
函数wp_remote_post()
用于发送POST请求。
$url = 'http://httpbin.org/post';
$args = array(
'body' => array(
"name" => "collins",
"url" => 'http://w3guy.com'
)
);
$response = wp_remote_post( $url, $args );
The code above is a basic POST request to the endpoint http://httpbin.org/post
with an array of parameter or body sent alongside.
上面的代码是对端点http://httpbin.org/post
的基本POST请求,同时发送了一组参数或正文。
To retrieve the response body, headers, status code and message, use the appropriate response helper function provided by the HTTP API.
要检索响应主体,标头,状态代码和消息,请使用HTTP API提供的适当的响应帮助器功能。
头要求 (Head Requests)
To send an HTTP request using the HEAD method, the function wp_remote_head()
is used.
要使用HEAD方法发送HTTP请求,请使用函数wp_remote_head()
。
$url = 'http://httpbin.org';
$args = array(
'body' => array(
"name" => "collins",
"url" => 'http://w3guy.com'
)
);
$response = wp_remote_head( $url, $args );
$response_code = wp_remote_retrieve_response_message( $response );
使用其他HTTP方法的请求 (Requests Using Other HTTP Methods)
To send a PUT, DELETE or any other custom HTTP method request, use the wp_remote_request()
function.
要发送PUT,DELETE或任何其他自定义HTTP方法请求,请使用wp_remote_request()
函数。
An example of a PUT request:
PUT请求的示例:
$url = 'http://httpbin.org';
$args = array(
'method' => 'PUT'
);
$response = wp_remote_request( $url, $args );
An example of a DELETE request:
DELETE请求的示例:
$url = 'http://httpbin.org';
$args = array(
'method' => 'DELETE'
);
$response = wp_remote_request( $url, $args );
The function wp_remote_request()
can be use also to make GET and POST requests.
函数wp_remote_request()
也可以用于发出GET和POST请求。
GET request
GET请求
$url = 'http://httpbin.org/get';
$args = array(
'method' => 'GET'
);
$response = wp_remote_request( $url, $args );
POST request
POST请求
$url = 'http://httpbin.org/post';
$args = array(
'method' => 'POST'
);
$response = wp_remote_request( $url, $args );
进阶设定 (Advanced Configuration)
The argument
timeout
allows for setting the time in seconds before the connection is dropped and an error returned.参数
timeout
允许以秒为单位设置断开连接并返回错误之前的时间。Note: the default for this value is
注意:此值的默认值为
5 seconds
.5 seconds
。The timeout in the GET request below has been incremented to
45 seconds
.下面的GET请求中的超时已增加到
45 seconds
。
$url = 'http://httpbin.org/get';
$args = array(
'method' => 'GET',
'timeout' => 45,
);
$response = wp_remote_request( $url, $args );
The
user-agent
argument is used to set the user-agent.user-agent
参数用于设置用户代理。The default is,
WordPress/4.0; http://w3guy.com
where 4.0 is the WordPress version number and w3guy.com, the blog URL.默认值为
WordPress/4.0; http://w3guy.com
WordPress/4.0; http://w3guy.com
,其中4.0是WordPress版本号,而w3guy.com是博客URL。
$args = array(
'user-agent' => 'Crawler by w3guy',
);
The
sslverify
argument checks to see if the SSL certificate is valid (not self-signed) and will deny the response if it isn’t.sslverify
参数检查SSL证书是否有效(非自签名),如果无效,则将拒绝响应。If you know the site is self-signed but can be trusted, then set to
false
.如果您知道该站点是自签名的但可以信任,则将其设置为
false
。The default value is always
默认值为始终
true
true
$args = array(
'sslverify' => true
);
结语 (Wrap Up)
Whew! We’ve come to the end of this tutorial. By now, you should know what APIs are, have a basic understanding of the WordPress HTTP API and how it works.
ew! 我们到了本教程的结尾。 到现在为止,您应该知道什么是API,对WordPress HTTP API及其工作原理有基本的了解。
In subsequent tutorials, we’ll see more of the HTTP API in action. Be sure to keep an eye on the WordPress channel.
在后续教程中,我们将看到更多的HTTP API实际应用。 确保关注WordPress频道 。