A friend of mine operates a multi-author blog powered by WordPress.
我的一个朋友经营着一个由WordPress驱动的多作者博客。
To prevent any legal trouble, he often adds a ‘Disclaimer’ in every post made by guest authors which he did by editing and including the disclaimer text before publication.
为了防止任何法律麻烦,他经常在来宾作者的每篇帖子中添加“免责声明”,他在发布前通过编辑并包括免责声明文本来做到这一点。
I’m sure you will agree, that editing and adding disclaimers in every post made by guest authors seems a daunting task.
我相信您会同意,在来宾作者的每篇文章中编辑和添加免责声明似乎是一项艰巨的任务。
In this article, we will build a simple Disclaimer Notice plugin that will have an option page where a site administrator can add the disclaimer text
which automatically gets appended immediately before or after the post content.
在本文中,我们将构建一个简单的“免责声明通知”插件,该插件将具有一个选项页,站点管理员可以在其中添加disclaimer text
,该disclaimer text
会在帖子内容之前或之后立即自动添加。
插件开发 (Plugin Development)
To begin the plugin development, we need to include the plugin header in the plugin PHP file.
要开始插件开发,我们需要在插件PHP文件中包含插件头。
Without the header, WordPress will not recognize the plugin.
没有标题,WordPress将无法识别该插件。
<?php
/*
Plugin Name: Disclaimer Manager
Plugin URI: https://www.sitepoint.com
Description: Easy Disclaimer Manager for Multi-author blogs.
Version: 1.0
Author: Agbonghama Collins
Author URI: http://w3guy.com
License: GPL2
*/
To get started building the settings page for the plugin; first, we will add the sub menu page to the ‘Settings’ menu using the function add_options_page placed in a function registered with the admin_menu
.
开始构建插件的设置页面; 首先,我们将使用在admin_menu
注册的函数中放置的add_options_page函数将子菜单页面添加到“设置”菜单。
// Add the admin options page
add_action( 'admin_menu', 'dm_settings_page' );
function dm_settings_page() {
add_options_page( 'Disclaimer Manager', 'Disclaimer Manager', 'manage_options', 'disclaimer-manager', 'dm_options_page' );
}
The argument passed to add_options_page()
are as follows:
传递给add_options_page()
的参数如下:
Disclaimer Manager
: The text to be displayed in the title tags of the page when the menu is selected.Disclaimer Manager
:选择菜单时在页面标题标签中显示的文本。Disclaimer Manager
: The text to be used for the menu.Disclaimer Manager
:用于菜单的文本。manage_options
: The capability required for this menu to be displayed to the user.manage_options
:向用户显示此菜单所需的功能 。disclaimer-manager
: The slug name to refer to this menu.disclaimer-manager
:引用此菜单的子名称 。dm_options_page
: The function to be called to output the plugin settings page.dm_options_page
:要输出插件设置页面的函数。
Below, is the code for the callback function dm_options_page
that will display the settings page.
下面是用于显示设置页面的回调函数dm_options_page
的代码。
// Draw the options page
function dm_options_page() {
?>
<div class="wrap">
<?php screen_icon(); ?>
<h2> Disclaimer Manager for Authors </h2>
<form action="options.php" method="post">
<?php settings_fields( 'disclaimer_manager_options' ); ?>
<?php do_settings_sections( 'disclaimer-manager' ); ?>
<?php submit_button(); ?>
</form>
</div>
<?php
}
The WordPress Settings API is being used to build and manage the settings form.
WordPress 设置API用于构建和管理设置表单。
The settings_fields function in dm_options_page()
above output the nonce, action, and form fields for the settings page while the do_settings_sections()
prints out all settings sections added to a particular settings page.
上面dm_options_page()
的settings_fields函数输出设置页面的现时,动作和表单字段,而do_settings_sections()
打印出添加到特定设置页面的所有设置部分。
Below is the full Settings API code for the settings page.
以下是设置页面的完整设置API代码。
// Register and define the settings
add_action( 'admin_init', 'dm_admin_init' );
function dm_admin_init() {
register_setting( 'disclaimer_manager_options', 'disclaimer_manager_options',
'' );
add_settings_section( 'dm_main', 'Plugin Settings',
'', 'disclaimer-manager' );
add_settings_field( 'dm_textarea-id', 'Enter Disclaimer Text',
'disclaimer_text_textarea', 'disclaimer-manager', 'dm_main' );
add_settings_field( 'dm_select-id', 'Disclaimer Position',
'disclaimer_text_position', 'disclaimer-manager', 'dm_main' );
}
// Display and fill the form field
function disclaimer_text_textarea() {
// get option 'disclaimer_text' value from the database
$options = get_option( 'disclaimer_manager_options' );
$disclaimer_text = $options['disclaimer_text'];
// echo the field
echo "<textarea rows='8' cols='50' id='disclaimer_text' name='disclaimer_manager_options[disclaimer_text]' >$disclaimer_text</textarea>";
}
function disclaimer_text_position() {
// get option 'disclaimer_position' value from the database
$options = get_option( 'disclaimer_manager_options' );
$disclaimer_position = $options['disclaimer_position'];
echo '<select name="disclaimer_manager_options[disclaimer_position]">';
echo '<option value="top"' . selected( $disclaimer_position, 'top' ) . '>Top</option>';
echo '<option value="bottom"' . selected( $disclaimer_position, 'bottom' ) . '>Bottom</option>';
echo '</select>';
}
Take Note: The register setting()
registers the setting.
注意: register setting()
注册设置。
The add_settings_section()
creates settings sections – groups of settings you see on WordPress settings pages with a shared heading.
add_settings_section()
创建设置部分 -您在WordPress设置页面上看到的带有共享标题的设置组。
The add_settings_field()
registers a settings field to a settings page and section.
add_settings_field()
将设置字段注册到设置页面和部分。
The get_option()
retrieves the values of the settings form from the database and the update_option()
saves the form values to the database.
get_option()
从数据库中检索设置表单的值,而update_option()
将表单值保存到数据库。
We are done building the settings page for the plugin.
我们已经完成了插件的设置页面的构建。
Below is a screenshot of the plugin settings page.
以下是插件设置页面的屏幕截图。
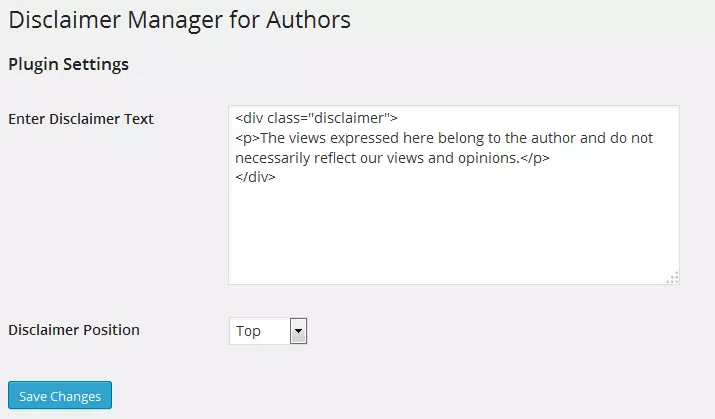
The function add_disclaimer_to_post
as its name implies, appends the ‘Disclaimer’ text to the top or bottom of every post as defined in the plugin settings page.
顾名思义,功能add_disclaimer_to_post
将“免责声明”文本附加到插件设置页面中定义的每个帖子的顶部或底部。
function add_disclaimer_to_post( $content ) {
$options = get_option( 'disclaimer_manager_options' );
// get disclaimer text form DB
$disclaimer_text = $options['disclaimer_text'];
// get disclaimer position from DB
$disclaimer_position = $options['disclaimer_position'];
// ensure we are in a post and not a page
if ( is_single() ) : // if disclaimer position is set to top
{
if ( $disclaimer_position == 'top' ) {
$content = $disclaimer_text . $content;
}
// if disclaimer position is set to bottom
if ( $disclaimer_position == 'bottom' ) {
$content .= $disclaimer_text;
}
}
endif;
return $content;
}
Allow me to explain what the code above does.
请允许我解释以上代码的作用。
The ‘Disclaimer Text’ and its position are retrieved from the database and saved to the variables $disclaimer_text
and $disclaimer_position
.
从数据库中检索“免责声明文本”及其位置,并将其保存到变量$disclaimer_text
和$disclaimer_position
。
Next, we use the Boolean WordPress function is_single()
to ensure we are dealing with a post and not an attachment or page.
接下来,我们使用布尔值WordPress函数is_single()
来确保我们处理的是帖子而不是附件或页面。
The next two if
conditional statements append the disclaimer to the top or bottom of the post content depending on the outcome of $disclaimer_position
.
接下来的两个if
条件语句取决于$disclaimer_position
的结果,将免责声明附加到帖子内容的顶部或底部。
To put the function to work, we need to hook it to the content
filter (used to filter the content of a post after it is retrieved from the database and before it is printed to the screen).
为了使该功能正常工作,我们需要将其挂接到the content
过滤器(用于从数据库中检索帖子的内容之后,再将其打印到屏幕上,以过滤该帖子的内容)。
add_filter( 'the_content', 'add_disclaimer_to_post' );
Voila! We are done coding the ‘Disclaimer Plugin’.
瞧! 我们已经完成了“免责声明插件”的编码。
Here is a screenshot of the plugin in action:
这是运行中的插件的屏幕截图:
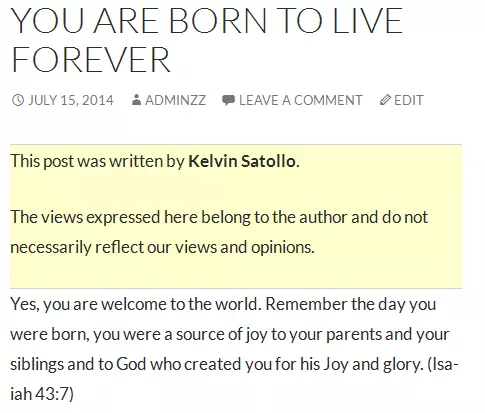
结论 (Conclusion)
To further understand how the plugin was built and how you can implement it in your WordPress site, download the plugin.
要进一步了解插件的构建方式以及如何在WordPress网站中实现该插件 ,请下载 。
If you are looking for an advanced disclaimer plugin with features such as:
如果您正在寻找具有以下功能的高级免责声明插件:
- Ability to choose the authors that will have a disclaimer or notification displayed in their post. 能够选择将在其帖子中显示免责声明或通知的作者。
- Built-in editor for adding CSS Styles for the ‘Disclaimer’ text / notification and lots more. 内置的编辑器,用于为“免责声明”文本/通知添加CSS样式等。
Grab the improved version from the WordPress Plugin Directory.
从WordPress插件目录中获取改进的版本。
Let me know your thoughts in the comments.
在评论中让我知道您的想法。
翻译自: https://www.sitepoint.com/building-a-disclaimer-notice-plugin/