whois 和geoip
In a previous tutorial, we took a deep dive into WordPress HTTP API and we learned what APIs
are and how to use the HTTP API
to consume web services.
在上一教程中,我们深入研究了WordPress HTTP API,并了解了什么是APIs
以及如何使用HTTP API
来使用Web服务。
I promised to show some real-world examples of how to consume APIs in WordPress using the HTTP API
, this is the first in a series of upcoming articles.
我答应展示一些有关如何使用HTTP API
在WordPress中使用API的真实示例,这是一系列后续文章中的第一篇。
In this tutorial, we will develop a WordPress widget that display the WHOIS and social information of a domain name such as Google’s PageRank and +1 count, Alexa rank, the date a domain was created, when the domain expires, DNS name servers, Facebook shares and likes count, Twitter tweets and LinkedIn shares.
在本教程中,我们将开发一个WordPress小部件,该部件显示域名的WHOIS和社会信息,例如Google的PageRank和+1计数,Alexa排名,域的创建日期,域到期时,DNS名称服务器,Facebook分享和喜欢计数,Twitter推文和LinkedIn分享。
The domain information listed above will be obtained from JsonWhois API.
上面列出的域信息将从JsonWhois API获得。
To get this data, a GET
request will be sent to the endpoint http://jsonwhois.com/api/whois
with your API key and the domain name as the request parameters.
要获取此数据,将使用您的API密钥和域名作为请求参数,将GET
请求发送到端点http://jsonwhois.com/api/whois
。
Enter the URL below into your browser to reveal available information (in JSON format) about the domain sitepoint.com
:
在浏览器中输入以下URL,以显示有关域sitepoint.com
可用信息(JSON格式):
http://jsonwhois.com/api/whois/?apiKey=54183ad8c433fac10b6f5d7c&domain=sitepoint.com
It is from the JSON object the widget we develop will get its data from.
它是从JSON对象中获取的,我们开发的小部件将从中获取其数据。
If you want to jump ahead in this tutorial, you can view a demo of the widget and download the widget plugin.
如果您想继续学习本教程,可以查看该小部件的演示并下载小部件插件 。
编码小部件 (Coding the Widget)
First off, include the plugin header.
首先,包括插件头。
<?php
/*
Plugin Name: Domain Whois and Social Data
Plugin URI: https://www.sitepoint.com
Description: Display whois and social data of a Domain.
Version: 1.0
Author: Agbonghama Collins
Author URI: http://w3guy.com
License: GPL2
*/
To create a WordPress widget; first extend the standard WP_Widget
class, include the necessary class functions or methods and finally, register the widget.
创建一个WordPress小部件; 首先扩展标准的WP_Widget
类,包括必要的类函数或方法,最后注册该窗口小部件。
Create a child-class extending the WP_Widget
class.
创建扩展WP_Widget
类的子类。
class Domain_Whois_Social_Data extends WP_Widget {
// ...
Give the widget a name and description using the __construct()
magic method as follows.
如下使用__construct()
魔术方法为小部件命名和描述。
function __construct() {
parent::__construct(
'whois_social_widget', // Base ID
__( 'Domain Whois and Social Data', 'dwsd' ), // Name
array( 'description' => __( 'Display whois and social data of a Domain.', 'dwsd' ), ) // Description
);
}
We will create a method called json_whois_api
that will accept two arguments: the domain to query and your API key whose duty is to send a ‘GET’ request to the JsonWhois API, retrieve the response body and then convert the response to an object using json_decode() function.
我们将创建一个名为json_whois_api
的方法,该方法将接受两个参数:要查询的域和您的API密钥,其职责是向JsonWhois API发送“ GET”请求,检索响应主体,然后使用json_decode将响应转换为对象()功能。
/**
* Retrieve the response body of the API GET request and convert it to an object
*
* @param $domain
* @param $api_key
*
* @return object|mixed
*/
public function json_whois_api( $domain, $api_key ) {
$url = 'http://jsonwhois.com/api/whois/?apiKey=' . $api_key . '&domain=' . $domain;
$request = wp_remote_get( $url );
$response_body = wp_remote_retrieve_body( $request );
$decode_json_to_object = json_decode( $response_body );
return $decode_json_to_object;
}
For each piece of domain information the widget is going to display, a method that returns the individual data will also be created. That is, a method that returns the Alexa Rank, PageRank and so on will be created.
对于小部件将要显示的每个域信息,还将创建一种返回单个数据的方法。 也就是说,将创建一个返回Alexa Rank,PageRank等的方法。
值得一提 (Worthy of Note)
For those new to PHP programming and WordPress plugin development, you might find the something like this strange:
对于那些不熟悉PHP编程和WordPress插件开发的人,您可能会发现类似这样的奇怪现象:
return $response_data->social->facebook->data[0]->share_count;
The ->
is used to access an object property and []
for accessing an array.
->
用于访问对象属性, []
用于访问数组。
The reason for this is that the response return by JsonWhois after being decoded to an object is a multidimensional object with some property containing array as values.
原因是JsonWhois返回的响应在解码为对象后返回的是一个多维对象,具有包含数组作为值的某些属性。
The code below explains this $object->facebook->data[0]->share_count;
下面的代码解释了此$object->facebook->data[0]->share_count;
[facebook] => stdClass Object
(
[data] => Array
(
[0] => stdClass Object
(
https%3A%2F%2Feditor.sitepoint.com => https://www.sitepoint.com
[normalized_url] => https://www.sitepoint.com/
[share_count] => 1094
[like_count] => 448
[comment_count] => 161
[total_count] => 1703
[commentsbox_count] => 0
[comments_fbid] => 501562723433
[click_count] => 138
)
)
)
In no particular order, below are the class methods or functions that will return the various domain information the WordPress widget will display.
以下没有特殊顺序的类方法或函数将返回WordPress小部件将显示的各种域信息。
/**
* Get the domain Alexa Rank
*
* @param object $response_data JSON decoded response body
*
* @return integer
*/
public function alexa_rank( $response_data ) {
return $response_data->alexa->rank;
}
/**
* Number of times domain have been tweeted
*
* @param object $response_data JSON decoded response body
*
* @return integer
*/
public function twitter_tweets( $response_data ) {
return $response_data->social->twitter->count;
}
/**
* Number of times domain have been shared on Facebook
*
* @param object $response_data JSON decoded response body
*
* @return integer
*/
public function facebook_share_count( $response_data ) {
return $response_data->social->facebook->data[0]->share_count;
}
/**
* Number of times domain have been liked on Facebook
*
* @param object $response_data JSON decoded response body
*
* @return mixed
*/
public function facebook_like_count( $response_data ) {
return $response_data->social->facebook->data[0]->like_count;
}
/**
* Number of times domain have been shared to LinkedIn
*
* @param object $response_data JSON decoded response body
*
* @return integer
*/
public function linkedin_share( $response_data ) {
return $response_data->social->linkedIn;
}
/**
* Number of times domain have been shared on Google+
*
* @param object $response_data JSON decoded response body
*
* @return integer
*/
public function google_share( $response_data ) {
return $response_data->social->google;
}
/**
* Google PageRank of Domain
*
* @param object $response_data JSON decoded response body
*
* @return integer
*/
public function google_page_rank( $response_data ) {
return $response_data->google->rank;
}
/**
*Domain name servers
*
* @param object $response_data JSON decoded response body
*
* @return string
*/
public function domain_nameservers( $response_data ) {
$name_servers = $response_data->whois->domain->nserver;
return $name_servers->{0} . ' ' . $name_servers->{1};
}
/**
* Date domain was created
*
* @param object $response_data JSON decoded response body
*
* @return mixed
*/
public function date_created( $response_data ) {
return $response_data->whois->domain->created;
}
/**
* Domain expiration date
*
* @param object $response_data JSON decoded response body
*
* @return mixed
*/
public function expiration_date( $response_data ) {
return $response_data->whois->domain->expires;
}
The back-end widget settings form is created by the form()
method consisting of three form fields which house the widget title, domain and your API key.
后端窗口小部件设置表单是由form()
方法创建的,该方法由三个表单域组成,其中包含窗口小部件标题,域和您的API密钥。
/**
* Back-end widget form.
*
* @see WP_Widget::form()
*
* @param array $instance Previously saved values from database.
*
* @return string
*/
public function form( $instance ) {
if ( isset( $instance['title'] ) ) {
$title = $instance['title'];
} else {
$title = __( 'Domain Whois & Social Data', 'dwsd' );
}
$domain_name = isset( $instance['domain_name'] ) ? $instance['domain_name'] : '';
$api_key = isset( $instance['api_key'] ) ? $instance['api_key'] : '54183ad8c433fac10b6f5d7c';
?>
<p>
<label for="<?php echo $this->get_field_id( 'title' ); ?>"><?php _e( 'Title:' ); ?></label>
<input class="widefat" id="<?php echo $this->get_field_id( 'title' ); ?>"
name="<?php echo $this->get_field_name( 'title' ); ?>" type="text"
value="<?php echo esc_attr( $title ); ?>">
</p>
<p>
<label
for="<?php echo $this->get_field_id( 'domain_name' ); ?>"><?php _e( 'Domain name (without http://)' ); ?></label>
<input class="widefat" id="<?php echo $this->get_field_id( 'domain_name' ); ?>"
name="<?php echo $this->get_field_name( 'domain_name' ); ?>" type="text"
value="<?php echo esc_attr( $domain_name ); ?>">
</p>
<p>
<label for="<?php echo $this->get_field_id( 'api_key' ); ?>"><?php _e( 'API Key)' ); ?></label>
<input class="widefat" id="<?php echo $this->get_field_id( 'api_key' ); ?>"
name="<?php echo $this->get_field_name( 'api_key' ); ?>" type="text"
value="<?php echo esc_attr( $api_key ); ?>">
</p>
<?php
}
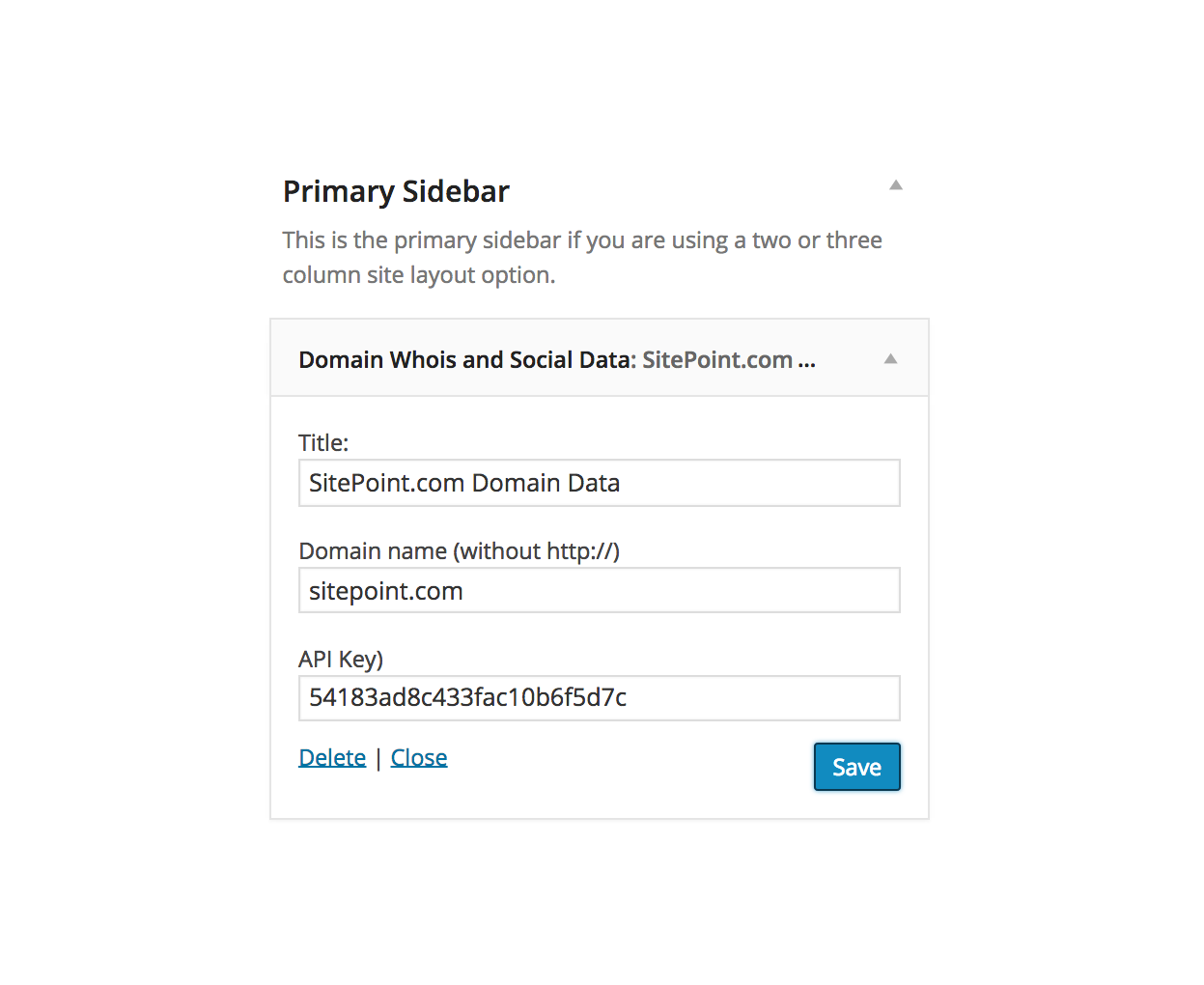
When the widget form is filled, the update()
method sanitizes and saves the entered values to the database for reuse.
填写窗口小部件表单时, update()
方法将清除输入的值并将其保存到数据库中以供重复使用。
/**
* Sanitize widget form values as they are saved.
*
* @see WP_Widget::update()
*
* @param array $new_instance Values just sent to be saved.
* @param array $old_instance Previously saved values from database.
*
* @return array Updated safe values to be saved.
*/
public function update( $new_instance, $old_instance ) {
$instance = array();
$instance['title'] = ( ! empty( $new_instance['title'] ) ) ? strip_tags( $new_instance['title'] ) : '';
$instance['domain_name'] = ( ! empty( $new_instance['domain_name'] ) ) ? strip_tags( $new_instance['domain_name'] ) : '';
return $instance;
}
The widget()
method displays the widget in the front-end of WordPress.
widget()
方法将插件显示在WordPress的前端。
/**
* Front-end display of widget.
*
* @see WP_Widget::widget()
*
* @param array $args Widget arguments.
* @param array $instance Saved values from database.
*/
public function widget( $args, $instance ) {
// Retrieve the saved widget API key
$api_key = ! empty( $instance['api_key'] ) ? $instance['api_key'] : '54183ad8c433fac10b6f5d7c';
// Get the Domain name saved in the widget
$domain_name = ! empty( $instance['domain_name'] ) ? $instance['domain_name'] : '';
// JsonWhois API response
$api_response = $this->json_whois_api( $domain_name, $api_key );
// Display the widget Title
echo $args['before_widget'];
if ( ! empty( $instance['title'] ) ) {
echo $args['before_title'] . apply_filters( 'widget_title', $instance['title'] ) . $args['after_title'];
}
echo '<ol>';
echo '<li> <strong>Alexa Rank:</strong> ', $this->alexa_rank( $api_response ), '</li>';
echo '<li> <strong>Google Page Rank:</strong> ', $this->google_page_rank( $api_response ), '</li>';
echo '<li> <strong>Facebook shares:</strong> ', $this->facebook_share_count( $api_response ), '</li>';
echo '<li> <strong>Facebook likes:</strong> ', $this->facebook_like_count( $api_response ), '</li>';
echo '<li> <strong>Twitter Tweets:</strong> ', $this->twitter_tweets( $api_response ), '</li>';
echo '<li> <strong>Google +1:</strong> ', $this->google_share( $api_response ), '</li>';
echo '<li> <strong>LinkedIn shares:</strong> ', $this->linkedin_share( $api_response ), '</li>';
echo '<li> <strong>Date created:</strong> ', $this->date_created( $api_response ), '</li>';
echo '<li> <strong>Expiration date:</strong> ', $this->expiration_date( $api_response ), '</li>';
echo '<li> <strong>Name servers:</strong> ', $this->domain_nameservers( $api_response ), '</li>';
echo '</ol>';
echo $args['after_widget'];
}
Code explanation: First, the saved widget form values (title, domain and API key) are retrieved from the database and saved to a variable.
代码说明:首先,从数据库中检索保存的窗口小部件表单值(标题,域和API密钥)并将其保存到变量中。
The domain and API key are passed to the json_whois_api
method with the resultant response body saved to $api_response
.
域和API密钥将传递到json_whois_api
方法,结果响应主体保存到$api_response
。
Calls to the various methods that return the domain data are made with the response body ($api_response
) as an argument.
以响应正文( $api_response
)作为参数,对返回域数据的各种方法进行调用。
Finally, we close the widget class.
最后,我们关闭小部件类。
} // class Domain_Whois_Social_Data
The widget class needs to be registered by being hooked to widgets_init
Action so it is recognized by WordPress internals.
需要通过挂接到widgets_init
Action来注册小部件类,以便WordPress内部人员可以识别它。
// register Domain_Whois_Social_Data widget
function register_whois_social_widget() {
register_widget( 'Domain_Whois_Social_Data' );
}
add_action( 'widgets_init', 'register_whois_social_widget' );
Below is a screenshot of the widget.
以下是小部件的屏幕截图。
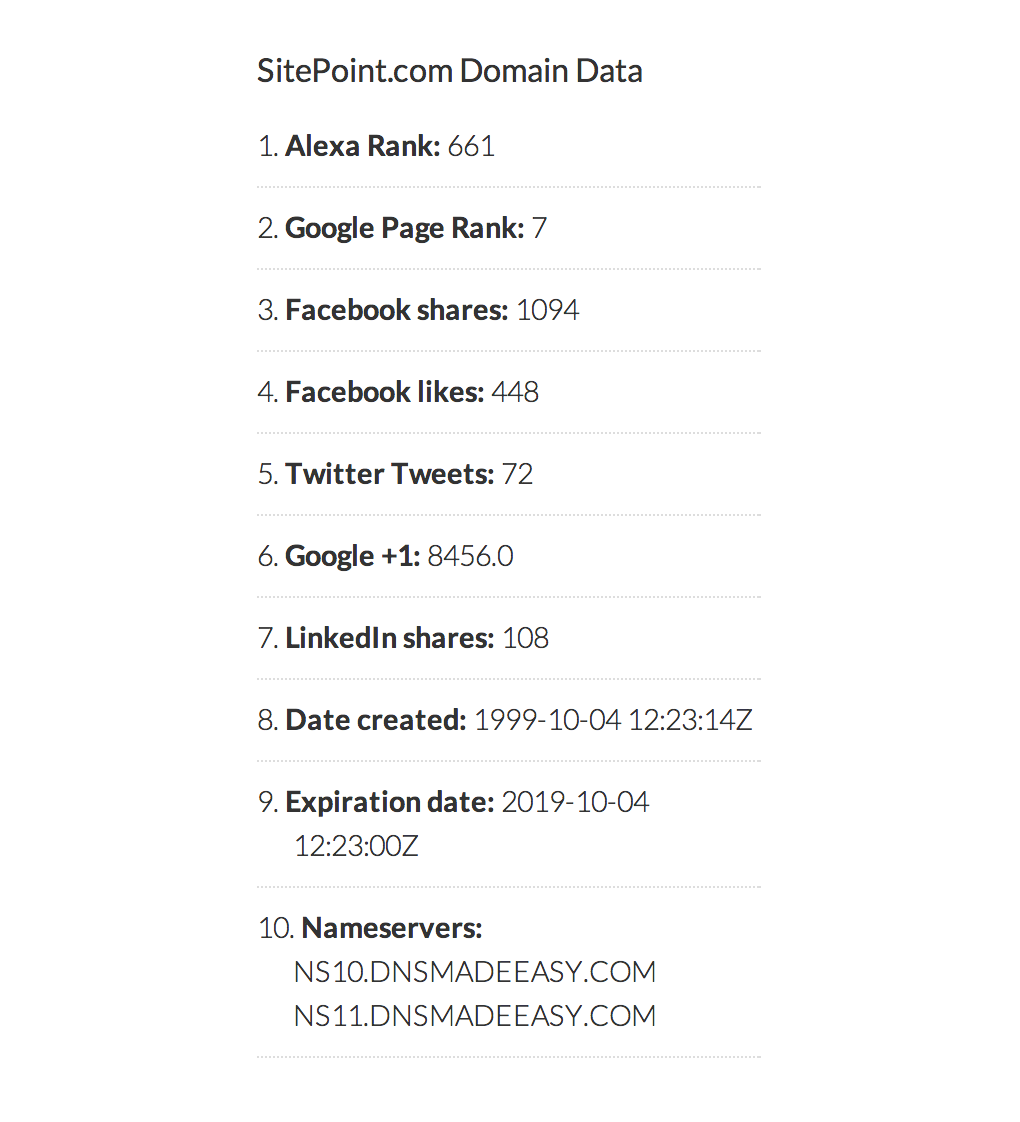
相关资源 (Related Resources)
If you’re interested in learning more about how WordPress widgets work, you might be interested in the following articles:
如果您想了解有关WordPress小部件如何工作的更多信息,则可能对以下文章感兴趣:
结语 (Wrap Up)
To further understand how the widget was built and how to implement it on your WordPress site, download the widget plugin from GitHub.
为了进一步了解该小部件的构建方式以及如何在您的WordPress网站上实现它,请从GitHub下载小部件插件。
As I mentioned, this article is the first in a series that will demonstrate how the WordPress HTTP API is used in a plugin.
正如我提到的,本文是系列文章的第一篇,它将演示如何在插件中使用WordPress HTTP API 。
Be sure to keep an eye on the WordPress channel for similar tutorials.
请务必关注WordPress频道,以获取类似的教程。
Until we meet again, happy coding!
直到我们再次见面,编码愉快!
翻译自: https://www.sitepoint.com/building-a-domain-whois-and-social-data-wordpress-widget/
whois 和geoip