drupal模块
In this article, we are going to look at how we can create a Drupal module which will allow your users to like your posts. The implementation will use jQuery to make AJAX calls and save this data asynchronously.
在本文中,我们将研究如何创建一个Drupal模块,该模块将使您的用户喜欢您的帖子。 该实现将使用jQuery进行AJAX调用并异步保存此数据。
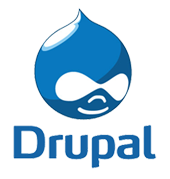
创建类似Drupal的模块 (Creating your Drupal like module)
Let’s start by creating the new Drupal module. To do that we should first create a folder called likepost
in the sites\all\modules\custom
directory of your Drupal installation as shown below:
让我们从创建新的Drupal模块开始。 为此,我们应该首先在Drupal安装的sites\all\modules\custom
目录中创建一个名为likepost
的文件夹,如下所示:
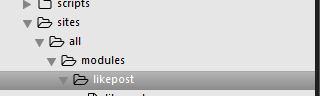
Inside this folder, you should create a file called likepost.info
with the following contents:
在此文件夹中,您应该创建一个名为likepost.info
的文件, likepost.info
包含以下内容:
name = likepost
description = This module allows the user to like posts in Drupal.
core = 7.x
This file is responsible for providing metadata about your module. This allows Drupal to detect and load its contents.
该文件负责提供有关模块的元数据。 这允许Drupal检测并加载其内容。
Next, you should create a file called as likepost.module
in the same directory. After creating the file, add the following code to it:
接下来,您应该在同一目录中创建一个名为likepost.module
的文件。 创建文件后,向其中添加以下代码:
/**
* @file
* This is the main module file.
*/
/**
* Implements hook_help().
*/
function likepost_help($path, $arg) {
if ($path == 'admin/help#likepost') {
$output = '<h3>' . t('About') . '</h3>';
$output .= '<p>' . t('This module allows the user to like posts in Drupal.') . '</p>';
return $output;
}
}
Once you have completed this you can go to the modules section in your Drupal administration and should be able to see the new module. Do not enable the module yet, as we will do so after adding some more functionality.
完成此操作后,您可以转到Drupal管理中的“模块”部分,并且应该能够看到新模块。 尚不启用该模块,因为我们将在添加更多功能后启用该模块。
创建模式 (Creating the schema)
Once you have created the module file, you can create a likepost.install
file inside the module root folder. Inside, you will define a table schema which is needed to store the likes on each post for each user. Add the following code to the file:
创建模块文件后,可以在模块根文件夹中创建一个likepost.install
文件。 在内部,您将定义一个表架构,该架构用于在每个用户的每个帖子上存储喜欢的内容。 将以下代码添加到文件中:
<?php
/**
* Implements hook_schema().
*/
function likepost_schema() {
$schema['likepost_table_for_likes'] = array(
'description' => t('Add the likes of the user for a post.'),
'fields' => array(
'userid' => array(
'type' => 'int',
'not null' => TRUE,
'default' => 0,
'description' => t('The user id.'),
),
'nodeid' => array(
'type' => 'int',
'unsigned' => TRUE,
'not null' => TRUE,
'default' => 0,
'description' => t('The id of the node.'),
),
),
'primary key' => array('userid', 'nodeid'),
);
return $schema;
}
In the above code we are are implementing the hook_schema(), in order to define the schema for our table. The tables which are defined within this hook are created during the installation of the module and are removed during the uninstallation.
在上面的代码中,我们正在实现hook_schema() ,以便为我们的表定义模式。 该挂钩中定义的表是在模块安装期间创建的,在卸载过程中将被删除。
We defined a table called likepost_table_for_likes
with two fields: userid
and nodeid
. They are both integers and will store one entry per userid – nodeid combination when the user likes a post.
我们定义了一个名为likepost_table_for_likes
的表, likepost_table_for_likes
包含两个字段: userid
和nodeid
。 它们都是整数,当用户喜欢帖子时,将为每个userid – nodeid组合存储一个条目。
Once you have added this file, you can install the module. If everything has gone correctly, your module should be enabled without any errors and the table likepost_table_for_likes
should be created in your database. You should also see the help link enabled in the module list next to your likepost
module. If you click on that you should be able to see the help message you defined in the hook_help()
implementation.
添加此文件后,即可安装该模块。 如果一切正常,应该启用您的模块而不会出现任何错误,并且应该在数据库中创建likepost_table_for_likes
表。 您还应该在likepost
模块旁边的模块列表中看到启用的帮助链接。 如果单击该按钮,则应该能够看到您在hook_help()
实现中定义的帮助消息。
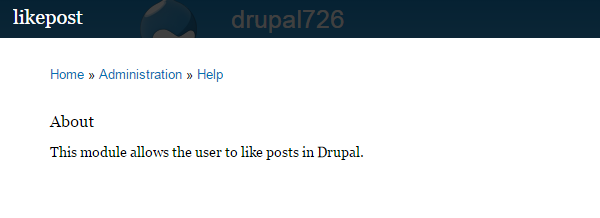
创建一个菜单回调来处理喜欢 (Creating a menu callback to handle likes)
Once we have enabled the module, we can add a menu callback which will handle the AJAX request to add or delete the like. To do that, add the following code to your likepost.module
file
启用模块后,我们可以添加菜单回调,该回调将处理AJAX请求以添加或删除like 。 为此,将以下代码添加到likepost.module
文件中
/**
* Implements hook_menu().
*/
function likepost_menu() {
$items['likepost/like/%'] = array(
'title' => 'Like',
'page callback' => 'likepost_like',
'page arguments' => array(2),
'access arguments' => array('access content'),
'type' => MENU_SUGGESTED_ITEM,
);
return $items;
}
function likepost_like($nodeid) {
$nodeid = (int)$nodeid;
global $user;
$like = likepost_get_like($nodeid, $user->uid);
if ($like !== 0) {
db_delete('likepost_table_for_likes')
->condition('userid', $user->uid)
->condition('nodeid', $nodeid)
->execute();
//Update the like value , which will be sent as response
$like = 0;
} else {
db_insert('likepost_table_for_likes')
->fields(array(
'userid' => $user->uid,
'nodeid' => $nodeid
))
->execute();
//Update the like value , which will be sent as response
$like = 1;
}
$total_count = likepost_get_total_like($nodeid);
drupal_json_output(array(
'like_status' => $like,
'total_count' => $total_count
)
);
}
/**
* Return the total like count for a node.
*/
function likepost_get_total_like($nid) {
$total_count = db_query('SELECT count(*) from {likepost_table_for_likes} where nodeid = :nodeid',
array(':nodeid' => $nid))->fetchField();
return (int)$total_count;
}
/**
* Return whether the current user has liked the node.
*/
function likepost_get_like($nodeid, $userid) {
$like = db_query('SELECT count(*) FROM {likepost_table_for_likes} WHERE
nodeid = :nodeid AND userid = :userid', array(':nodeid' => $nodeid, ':userid' => $userid))->fetchField();
return (int)$like;
}
In the above code, we are implementing hook_menu() so that whenever the path likepost/like
is accessed with the node ID, it will call the function likepost_like()
.
在上面的代码中,我们正在实现hook_menu(),以便每当使用节点ID访问likepost/like
路径时,它将调用函数likepost_like()
。
Inside of likepost_like()
we get the node ID and the logged in user’s ID and pass them to the function likepost_get_like()
. In the function likepost_get_like()
we check our table likepost_table_for_likes
to see if this user has already liked this post. In case he has, we will delete that like, otherwise we will insert an entry. Once that is done, we call likepost_get_total_like()
with the node ID as a parameter, which calculates the total number of likes from all users on this post. These values are then returned as JSON using the drupal_json_output()
API function.
在likepost_like()
内部,我们获取节点ID和已登录用户的ID,并将它们传递给函数likepost_get_like()
。 在函数likepost_get_like()
我们检查表likepost_table_for_likes
以查看该用户是否已经喜欢此帖子。 如果他有,我们将删除它,否则,我们将插入一个条目。 完成此操作后,我们likepost_get_total_like()
节点ID作为参数调用likepost_get_total_like()
,该操作将计算来自该帖子的所有用户的点likepost_get_total_like()
总数。 然后,使用drupal_json_output()
API函数将这些值作为JSON返回。
This menu callback will be called from our JQuery AJAX call and will update the UI with the JSON it receives.
此菜单回调将从我们的JQuery AJAX调用中调用,并将使用接收到的JSON更新UI。
在节点上显示“赞”按钮 (Displaying the Like button on the node )
Once we have created the callback, we need to show the like link on each of the posts. We can do so by implementing hook_node_view()
as below:
创建回调之后,我们需要在每个帖子上显示like链接。 我们可以通过如下实现hook_node_view()
来做到这一点:
/**
* Implementation of hook_node_view
*/
function likepost_node_view($node, $view_mode) {
if ($view_mode == 'full'){
$node->content['likepost_display'] = array('#markup' => display_like_post_details($node->nid),'#weight' => 100);
$node->content['#attached']['js'][] = array('data' => drupal_get_path('module', 'likepost') .'/likepost.js');
$node->content['#attached']['css'][] = array('data' => drupal_get_path('module', 'likepost') .'/likepost.css');
}
}
/**
* Displays the Like post details.
*/
function display_like_post_details($nid) {
global $user;
$totalLike = likepost_get_total_like($nid);
$hasCurrentUserLiked = likepost_get_like($nid , $user->uid);
return theme('like_post',array('nid' =>$nid, 'totalLike' =>$totalLike, 'hasCurrentUserLiked' => $hasCurrentUserLiked));
}
/**
* Implements hook_theme().
*/
function likepost_theme() {
$themes = array (
'like_post' => array(
'arguments' => array('nid','totalLike','hasCurrentUserLiked'),
),
);
return $themes;
}
function theme_like_post($arguments) {
$nid = $arguments['nid'];
$totalLike = $arguments['totalLike'];
$hasCurrentUserLiked = $arguments['hasCurrentUserLiked'];
global $base_url;
$output = '<div class="likepost">';
$output .= 'Total number of likes on the post are ';
$output .= '<div class="total_count">'.$totalLike.'</div>';
if($hasCurrentUserLiked == 0) {
$linkText = 'Like';
} else {
$linkText = 'Delete Like';
}
$output .= l($linkText, $base_url.'/likepost/like/'.$nid, array('attributes' => array('class' => 'like-link')));
$output .= '</div>';
return $output;
}
Inside likepost_node_view()
we check for when the node is in the full
view mode and we add the markup returned by the function display_like_post_details()
. We also attached our custom JS and CSS file when the view is rendered using the attached property on the node content. In function display_like_post_details()
we get the total number of likes for the post and whether or not the current user has liked the post. Then we call the theme function which will call the function theme_like_post()
which we have declared in the implementation of ‘hook_theme’ but will allow the designers to override if required. In theme_like_post()
, we create the HTML output accordingly. The href
on the link is the $base_url
and the path to our callback appended to it. The node ID is also attached to the URL which will be passed as a parameter to the callback.
在likepost_node_view()
内部,我们检查节点何时处于full
视图模式,并添加由display_like_post_details()
函数返回的标记。 当使用节点内容上的附加属性呈现视图时,我们还附加了自定义JS和CSS文件。 在函数display_like_post_details()
我们获得帖子的喜欢总数,以及当前用户是否喜欢该帖子。 然后,我们调用主题函数,该函数将调用我们在“ hook_theme”的实现中声明的函数theme_like_post()
,但允许设计者在需要时重写。 在theme_like_post()
,我们相应地创建HTML输出。 链接上的href
是$base_url
并在$base_url
附加了回调的路径。 节点ID也附加到URL,URL将作为参数传递给回调。
Once this is done, add a file likepost.css
to the module root folder with the following contents:
完成此操作后,将具有以下内容的文件(如likepost.css
添加到模块根文件夹:
.likepost {
border-style: dotted;
border-color: #98bf21;
padding: 10px;
}
.total_count {
font-weight: bold;
}
.like-link {
color:red;
}
.like-link:hover {
color: red;
}
Now if you go to the complete page of a post you will see the Like post count as shown below.
现在,如果您转到帖子的整个页面,您将看到“喜欢”帖子计数,如下所示。
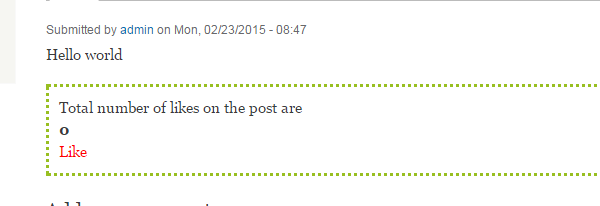
添加jQuery逻辑 (Adding the jQuery logic)
Now that we see the like link displayed, we will just have to create the likepost.js
file with the following contents:
现在我们看到显示了like链接,我们只需要创建具有以下内容的likepost.js
文件:
jQuery(document).ready(function () {
jQuery('a.like-link').click(function () {
jQuery.ajax({
type: 'POST',
url: this.href,
dataType: 'json',
success: function (data) {
if(data.like_status == 0) {
jQuery('a.like-link').html('Like');
}
else {
jQuery('a.like-link').html('Delete Like');
}
jQuery('.total_count').html(data.total_count);
},
data: 'js=1'
});
return false;
});
});
The above code binds the click event to the like link and makes an AJAX request to the URL of our callback menu function. The latter will update the like post count accordingly and then return the new total count and like status, which is used in the success function of the AJAX call to update the UI.
上面的代码将click事件绑定到like链接,并向我们的回调菜单函数的URL发出AJAX请求。 后者将相应地更新“赞”帖子计数,然后返回新的总数和“赞”状态,该状态在AJAX调用的成功功能中用于更新UI。
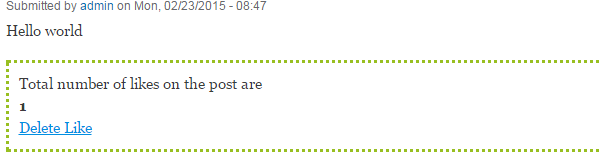
结论 (Conclusion)
jQuery and AJAX are powerful tools to create dynamic and responsive websites. You can easily use them in your Drupal modules to add functionality to your Drupal site, since Drupal already leverages jQuery for its interface.
jQuery和AJAX是创建动态和响应式网站的强大工具。 您可以在Drupal模块中轻松使用它们,以便为Drupal网站添加功能,因为Drupal已经利用jQuery作为其界面。
Have feedback? Let us know in the comments!
有意见吗? 让我们在评论中知道!
翻译自: https://www.sitepoint.com/drupal-goes-social-building-liking-module-drupal/
drupal模块