Most Python newcomers don’t know how to set up a development environment that follows the latest standards used by professional programmers, so this tutorial will teach you how to properly create a fully working Python development environment using industry accepted best practices.
大多数Python新手都不知道如何建立遵循专业程序员所使用的最新标准的开发环境,因此本教程将教您如何使用业界公认的最佳实践来正确创建一个可以正常工作的Python开发环境。
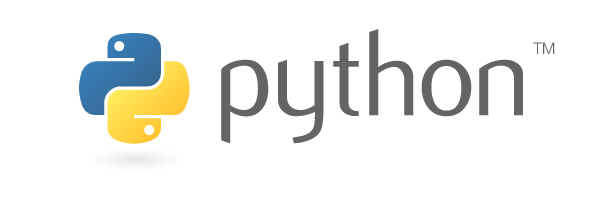
虚拟环境 (Virtual Environments)
There are so many libraries I use for my own personal projects, three web application development frameworks I use and many others I would love to explore in the near future. It is very clear that a serious project in Python depends on other packages written by other developers. If you are a Django developer, I am very sure you make use of South for performing automatic database migrations, Django Debug Toolbar for gathering various debug information about the current request/response, Celery for taking care of real-time operations and scheduling as well, and so on.
我用于自己的个人项目的库太多了,我使用了三个Web应用程序开发框架,在不久的将来我也想探索许多其他的库。 很明显,Python中的一个认真的项目取决于其他开发人员编写的其他程序包。 如果您是Django开发人员,我非常确定您可以利用South来执行自动数据库迁移,使用Django Debug Toolbar来收集有关当前请求/响应的各种调试信息,以及使用Celery来处理实时操作和调度, 等等。
I, for example, use the package requests
a lot for my projects, and a Django web application I am currently working on depends on version 2.3.0. According to the official documentation, the latest version of this package is version 2.5.1. Say I go ahead and install the latest version of the library on my Ubuntu machine because I need it for another project, and everything seems to work fine until I try to make use of my older project which worked fine with 2.3.0. Suddenly, everything is broken. What happened? Maybe the API of the latest version of requests has changed since version 2.3.0? The reason does not matter at this point, as my older project is broken and no longer works.
例如,我对我的项目经常使用该程序包requests
,而我当前正在使用的Django Web应用程序则取决于版本2.3.0。 根据官方文档,此软件包的最新版本为2.5.1版。 说我继续在我的Ubuntu机器上安装该库的最新版本,因为我需要将其用于另一个项目,在我尝试使用在2.3.0之前可以正常工作的旧项目之前,一切似乎都可以正常工作。 突然,一切都坏了。 发生了什么? 自版本2.3.0起,最新版本请求的API可能已更改? 此时的原因无关紧要,因为我的旧项目已损坏并且不再起作用。
A conflict between two projects has been created. They make use of the same library, but they require different versions of it.
两个项目之间已创建冲突。 它们使用相同的库,但是需要不同的版本。
The tool virtualenv is the solution to this problem.
工具virtualenv是解决此问题的方法。
虚拟环境 (virtualenv)
Virtualenv helps solve project dependency conflicts by creating isolated environments which can contain all the goodies Python programmers need to develop their projects. A virtual environment created using this tool includes a fresh copy of the Python binary itself as well as a copy of the entire Python standard library.
Virtualenv通过创建隔离的环境来解决项目依赖冲突,该环境可以包含Python程序员开发项目所需的所有好处。 使用此工具创建的虚拟环境包括Python二进制本身的新副本以及整个Python标准库的副本。
To create a virtual environment for your Python project, you type the command virtualenv
followed by the name you want to give to the virtual environment like shown below.
要为您的Python项目创建虚拟环境,请键入命令virtualenv
然后输入您想要赋予虚拟环境的名称,如下所示。
virtualenv virt1
It is very important to know that each time you want to use a created virtual environment, you need to activate it. The following command does this:
知道每次要使用创建的虚拟环境时都需要激活它,这一点非常重要。 下面的命令执行此操作:
source virt1/bin/activate
Everything installed in the virt1
directory will not affect the global packages or the system wide installations, thus avoiding dependency conflicts.
安装在virt1
目录中的所有内容都不会影响全局软件包或系统范围的安装,从而避免了依赖性冲突。
If I now install the newest version of requests, the old one does not get uninstalled. The new one is installed in the virtual environment exclusively.
如果我现在安装最新版本的请求,则旧版本不会被卸载。 新的虚拟机专门安装在虚拟环境中。
pip -I install requests
The above command produces the following output:
上面的命令产生以下输出:
Downloading/unpacking requests
Downloading requests-2.5.1-py2.py3-none-any.whl (464kB): 464kB downloaded
Installing collected packages: requests
Successfully installed requests
Cleaning up...
点子 (pip)
A word on the pip
command above – it’s a package manager that is very useful when you want to distribute your project to others, as it allows the developer to install all the required packages with a requirements.txt
file.
上面pip
命令中的一个词–它是一个包管理器,当您要将您的项目分发给其他人时非常有用,因为它允许开发人员安装所有要求的包以及requirements.txt
文件。
For example, a fellow developer can activate a virtual environment and then run the following command to install the dependencies of the project:
例如,同一个开发人员可以激活虚拟环境,然后运行以下命令来安装项目的依赖项:
pip install -r requirements.txt
To generate the dependencies file of your project, you run the following command.
要生成项目的依赖项文件,请运行以下命令。
pip freeze > requirements.txt
The pip tool can also be used to uninstall a package from your machine:
pip工具还可用于从计算机上卸载软件包:
pip uninstall some-package-name
One can install pip system-wide with the default Python package manager:
可以使用默认的Python软件包管理器在全系统范围内安装pip:
sudo easy_install pip
And then use pip to install virtualenv
:
然后使用pip安装virtualenv
:
sudo pip install virtualenv
创建环境后 (After the environment is created)
Once the virtual environment gets activated, your terminal prompt changes to show the user in which directory they are working:
激活虚拟环境后,终端提示将更改为向用户显示他们正在使用的目录:
(virt1)oltjano@baby:~/Desktop/myproject$
The following command will let you deactivate the virtual environment:
以下命令将使您停用虚拟环境:
deactivate
You can use the which
command to check the Python binary that is used in the current virtual environment.
您可以使用which
命令来检查当前虚拟环境中使用的Python二进制文件。
which python
If everything is working well, you should get something similar to the following output.
如果一切正常,您应该获得类似于以下输出的内容。
/home/oltjano/Desktop/myproject/virt1/bin/python
If you deactivate
and which
again, you should get a different output.
如果你deactivate
和which
再次,你应该得到一个不同的输出。
/usr/bin/python
This is because when working inside a virtual environment, the binary copy placed inside that environment is being used. The same applies to packages.
这是因为在虚拟环境中工作时,正在使用放置在该环境中的二进制副本。 包也是如此。
We can use the option -p
while working with virtualenv in order to use a specific version of Python which is globally installed on the machine.
我们可以在使用virtualenv时使用-p
选项,以使用特定版本的Python,该特定版本的Python已全局安装在计算机上。
For example, the following command can be used to create the virtual environment virt2
with Python3 in it, if you have Python3 installed on your machine.
例如,如果在计算机上安装了Python3,则以下命令可用于创建其中包含Python3的虚拟环境virt2
。
virtualenv -p /usr/bin/python3 virt2
And to delete a virtual environment you use the rm -r
command like you do with any other directory you want to delete.
要删除虚拟环境,请使用rm -r
命令,就像对要删除的任何其他目录一样。
rm -r virt2
虚拟环境包装器 (virtualenvwrapper)
Virtualenvwrapper provides very useful commands that make working with virtual environments even easier. For example, the workon
command can be used to activate and work on a virtual environment:
Virtualenvwrapper提供了非常有用的命令,使使用虚拟环境更加容易。 例如, workon
命令可用于激活虚拟环境并在其上工作:
workon virt1
It, too, is installed easily with pip.
它也可以通过pip轻松安装。
pip install virtualenvwrapper
I like to keep all my virtual environments in a single place. To accomplish this, run the following command to create a new directory for your virtual environments.
我喜欢将所有虚拟环境放在一个地方。 为此,请运行以下命令为您的虚拟环境创建一个新目录。
mkdir ~/.virtualenvs
Then set WORKON_HOME
to ~/.virtualenvs
like shown below.
然后将WORKON_HOME
设置为~/.virtualenvs
如下所示。
export WORKON_HOME=~/.virtualenvs
Once this is done, we can open the .bashrc
file inside the home directory using a text editor and add the following line to the end of it.
完成此操作后,我们可以使用文本编辑器打开主目录中的.bashrc
文件,并在其末尾添加以下行。
. /usr/local/bin/virtualenvwrapper.sh
Then, we reload the .bashrc
file with the following command.
然后,我们使用以下命令重新加载.bashrc
文件。
source ~/.bashrc
Now, the mkvirtualenv
command can be used to easily make new environments placed by default inside this folder.
现在,可以使用mkvirtualenv
命令轻松创建默认情况下放置在此文件夹中的新环境。
mkvirtualenv sitepoint
We can easily activate the virtual environment with the help of the workon
command:
我们可以在workon
命令的帮助下轻松激活虚拟环境:
workon sitepoint
The command to deactivate the virtual environment is the same as the one we used before.
停用虚拟环境的命令与我们之前使用的命令相同。
deactivate
It’s very easy to switch between different virtual environments. For example, to workon
another virtual environment:
在不同的虚拟环境之间切换非常容易。 例如,要workon
另一个虚拟环境上工作:
workon another_virtualenv
To delete a virtual environment, the command rmvirtualenv
should be used.
要删除虚拟环境,应使用命令rmvirtualenv
。
rmvirtualenv sitepoint
汽车环境 (Autoenv)
Another very useful tip is the one that helps automatically activate the virtual environments when the one cd’s into their project.
另一个非常有用的技巧是,当一张CD进入其项目时,该技巧有助于自动激活虚拟环境。
For this, a .env
file should be placed inside the project directory.
为此,应在项目目录中放置一个.env
文件。
cd sitepointproject
vim .env
Inside the .env
file, we insert the following line.
在.env
文件中,插入以下行。
workon name_of_virtualenv_here
The autoenv tool will help us automagically activate the environment when navigating into a directory containing a .env
file. To install it, we clone it.
当导航到包含.env
文件的目录时, autoenv工具将帮助我们自动激活环境。 要安装它,我们将其克隆。
git clone git://github.com/kennethreitz/autoenv.git ~/.autoenv
Then we use echo
to place another line inside the .bashrc
file. You can also use a text editor to do it.
然后,我们使用echo
在.bashrc
文件中放置另一行。 您也可以使用文本编辑器来执行此操作。
echo 'source ~/.autoenv/activate.sh' >> ~/.bashrc
Now, every time you cd
into your project, the virtual environment will get activated automatically.
现在,每次cd
到你的项目,在虚拟环境中会得到自动激活。
结论 (Conclusion)
In this tutorial, we learned some interesting hacks for efficiency when working with Python virtual environments. The tools are out there – embrace them and improve your workflow. Do you use any other interesting approaches in your Python development workflow? Let us know!
在本教程中,我们学习了一些有趣的技巧,可在使用Python虚拟环境时提高效率。 工具无处不在-拥抱它们并改善您的工作流程。 您是否在Python开发工作流程中使用了其他有趣的方法? 让我们知道!
翻译自: https://www.sitepoint.com/virtual-environments-python-made-easy/