Previously, we saw how to use Iron push queues with Laravel. All we needed to do was set up an Iron account, add a subscriber URL, push a message to queue, and receive the message.
之前 ,我们了解了如何在Laravel中使用Iron推入队列。 我们所需要做的就是设置一个Iron帐户,添加一个订户URL,将消息推送到队列中并接收消息。
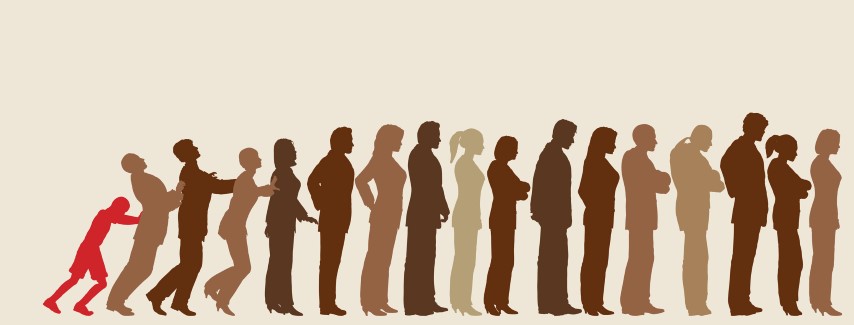
The way Laravel supports Iron push queues out-of-the-box is amazing, but there are always limitations. In this article we focus on those limitations and learn to tackle them.
Laravel开箱即用地支持Iron推送队列的方式令人惊叹,但始终存在局限性。 在本文中,我们将重点放在这些限制上,并学习解决这些限制。
情境 (Scenarios)
There are three possible scenarios:
有三种可能的方案:
You received the message and you finished the job successfully.
您收到了消息,并且您成功完成了作业。
- You need provision to tell Iron that the job was completed successfully and it needs to delete it from the queue. 您需要准备告知Iron,该作业已成功完成,并且需要将其从队列中删除。
You received the message and something went wrong. Basically, your job was not successful.
您收到了消息,出了点问题。 基本上,您的工作没有成功。
- In this case we should tell Iron that we failed and it should retry or if you couldn’t tell Iron anything, it should be clever enough to retry the job i.e., push the same message again to your subscriber after some time. 在这种情况下,我们应该告诉Iron我们失败了,并且应该重试,或者如果您什么也不能告诉Iron,它应该足够聪明以重试该作业,即在一段时间后再次向您的订户推送相同的消息。
You received the message, but your job is a long running process (something which takes more than a minute)
您收到了该消息,但是您的工作是一个漫长的过程(需要一分钟以上的时间)
- Iron should wait longer before it resends the message. Iron在重新发送消息之前应等待更长的时间。
These are all practical scenarios and you will face them on a daily basis. And yes, you need to cover these cases in your applications.
这些都是实际的方案,您将每天面对它们。 是的,您需要在应用程序中涵盖这些情况。
It’s possible to use Iron to its full power if we use the Iron MQ PHP Library. During setup we included "iron-io/iron_mq": "1.4.6"
in our composer file. The code for the same is hosted at https://github.com/iron-io/iron_mq_php
. Let’s take a peek into the documentation and try to understand how to use this library.
如果我们使用Iron MQ PHP库,则可以充分利用Iron。 在安装过程中,我们的作曲家文件中包含"iron-io/iron_mq": "1.4.6"
。 相同代码的托管在https://github.com/iron-io/iron_mq_php
。 让我们看一下文档并尝试了解如何使用该库。
We initiate a queue as follows:
我们启动队列,如下所示:
$ironmq = new IronMQ(array(
"token" => 'XXXXXXXXX',
"project_id" => 'XXXXXXXXX'
));
And this is how we post to a queue:
这就是我们发布到队列的方式:
$ironmq->postMessage($queue_name, "Test Message", array(
'timeout' => 120,
'delay' => 2,
'expires_in' => 2*24*3600 # 2 days
));
To turn a queue into a push queue (or create one) all we have to do is post to the queue with options like a list of subscribers, push_type etc. Using the library you can do the following:
要将队列变成推送队列(或创建一个队列),我们要做的就是将队列发布到队列中,并带有诸如订户列表,push_type等的选项。使用该库,您可以执行以下操作:
Note: If a queue doesn’t have any subscribers it is a pull queue.
注意:如果队列没有任何订阅者,则它是拉入队列。
$params = array(
"push_type" => "multicast",
"retries" => 5,
"subscribers" => array(
array("url" => "http://your.first.cool.endpoint.com/push"),
array("url" => "http://your.second.cool.endpoint.com/push")
)
);
$ironmq->updateQueue($queue_name, $params);
That’s it!
而已!
That’s exactly what Laravel does when you run the artisan queue:subscribe
command. To recap, after you do the necessary setup in the queue.php
file (filling it with project_id, token and queue_name), you run a command to register your subscribers:
当您运行artisan queue:subscribe
命令时,Laravel正是这样做的。 回顾一下,在queue.php
文件中进行了必要的设置(用project_id,token和queue_name填充之后)后 ,您运行一个命令来注册您的订户:
php artisan queue:subscribe queue_name http://foo.com/queue/receive
This command runs the following code:
此命令运行以下代码:
/vendor/laravel/framework/src/Illuminate/Queue/Console/SubscribeCommand.php
/vendor/laravel/framework/src/Illuminate/Queue/Console/SubscribeCommand.php
public function fire()
{
$iron->getIron()->updateQueue($this->argument('queue'), $this->getQueueOptions());
}
Some content is excluded from the file for brevity.
为简洁起见,一些内容已从文件中排除。
This is how Laravel converts our queue into a push queue. This is the same way it’s done when using the Iron MQ library.
Laravel就是这样将我们的队列转换为推送队列。 这与使用Iron MQ库时执行的方法相同。
使用Iron MQ PHP库 (Using the Iron MQ PHP Library)
Create a new controller IronController in app/controllers
在应用程序/控制器中创建一个新的控制器IronController
<?php class IronController extends Controller { protected $ironmq; public function __construct() { $this->ironmq = new IronMQ(array( "token" => 'XXXXXXXXXXXXXXXXXXX', //Your token from dashboard "project_id" => 'XXXXXXXXXXXXXXX' //Your project ID from dashboard )); } }
app/controller/IronController.php
app / controller / IronController.php
Before we create our methods, let’s create routes to understand what our controller will do:
在创建方法之前,让我们创建路由以了解控制器将执行的操作:
Route::get('iron/create','IronController@create'); //Create a Queue and add subscribers Route::get('iron/push','IronController@push'); //Push a message to Queue Route::get('iron/status/{id}','IronController@status'); //Know the status of the message we pushed Route::post('iron/receive','IronController@receive'); //Receive the message and process it
app/routes.php
app / routes.php
Now, let’s write a method to create a queue with a subscriber
现在,让我们编写一个与订户创建队列的方法
public function create() { $params = array( "subscribers" => array( array("url" => url('iron/receive')) ) ); $this->ironmq->updateQueue('testing', $params); return 'Push Queue Created'; }
app/controller/IronController.php
app / controller / IronController.php
You need to visit
http://953ffbb.ngrok.com/iron/create
i.e., use your ngrok url appended by/iron/create
. Note: Your ngrok url will be different.您需要访问
http://953ffbb.ngrok.com/iron/create
即,使用/iron/create
附加的ngrok网址。 注意:您的ngrok网址将不同。At this point you can go to Iron DashBoard -> MQ -> Queues -> Find a queue with name ‘testing’ -> Push Queues and make sure your subscriber is listed there similar to
http://953ffbb.ngrok.com/iron/receive
.此时,您可以转到Iron DashBoard-> MQ->队列->查找名称为“ testing”的队列-> Push Queues,并确保您的订户在此处列出,类似于
http://953ffbb.ngrok.com/iron/receive
。Let’s add methods to push a message onto a queue and check the status.
让我们添加一些方法来将消息推送到队列并检查状态。
Method to push a message:
推送消息的方法:
public function push() { //Just some data you want to pass $data = array( 'first_name' => 'Rajiv', 'last_name' => 'Seelam' ); //Convert data into a string so that we can pass it $data = serialize($data); //Post the message $job = $this->ironmq->postMessage('testing', $data); Log::info(__METHOD__.' - Message pushed with job_id : '.$job->id); return Redirect::to('iron/status/'.$job->id); }
Method to check the status of a message:
检查消息状态的方法:
public function status($id) { $status = $this->ironmq->getMessagePushStatuses('testing',$id); dd($status); }
app/controller/IronController.php
app / controller / IronController.php
We need a method to receive the message when we push.
我们需要一种在推送时接收消息的方法。
public function receive() { $req = Request::instance(); $jobId = $req->header('iron-message-id'); //Get job id $data = unserialize($req->getContent()); //Get content and unserialize it Log::info(__METHOD__.' - Job Received From Iron with Job ID : '.$jobId); return Response::json(array(),200); }
app/controller/IronController.php
app / controller / IronController.php
At this point you should understand that this is the method which will receive the message because in the create
method we listed url('iron/receive')
as the subscriber (check the routes file to confirm this url routes to this method).
此时,您应该理解这是将接收消息的方法,因为在create
方法中,我们将url('iron/receive')
列为订阅者(请检查路由文件以确认此url路由到此方法)。
Keep your app/storage/logs/laravel.log open to see what is happening.
保持您的应用程序/存储/日志/laravel.log打开,以查看正在发生的情况。
Now, if you visit http://953ffbb.ngrok.com/iron/push
, you should see a message similar to the following in your browser:
现在,如果您访问http://953ffbb.ngrok.com/iron/push
,则应该在浏览器中看到类似于以下内容的消息:
array (size=1)
0 =>
object(stdClass)[184]
public 'retries_delay' => int 60
public 'retries_remaining' => int 3
public 'retries_total' => int 3
public 'status_code' => int 200
public 'status' => string 'deleted' (length=7)
public 'url' => string 'http://953ffbb.ngrok.com/iron/receive' (length=38)
public 'id' => string '6019569765635787179' (length=19)
And your logs should be similar to :
并且您的日志应类似于:
[2014-05-31 12:45:04] production.INFO: IronController::push - Message pushed with job_id : 6019569765635787179 [] []
[2014-05-31 12:45:05] production.INFO: IronController::receive - Job Received From Iron with Job ID : 6019569765635787179 [] []
app/storage/logs/laravel.log
应用程序/存储/日志/laravel.log
These logs confirm our activity. Let’s summarize what we did here :
这些日志确认我们的活动。 让我们总结一下我们在这里所做的事情:
- We pushed a message to a queue called ‘testing’ 我们将消息推送到称为“测试”的队列
- Then we checked the status of the message on the queue 然后我们检查了队列中消息的状态
- We received the message and logged something. 我们收到了消息并记录了一些内容。
延迟和重试 (Delays and Retries)
Now, let’s go a bit deeper. Open your Iron dashboard and go to the queues page (Click on MQ) -> Open testing queue and check ‘Push Queues’, you will see the list of subscribers and under ‘Push Information’ you will see ‘Retries’ and ‘Retries Delay’.
现在,让我们更深入一些。 打开Iron仪表板,然后转到队列页面(单击MQ)->打开测试队列并检查“推送队列”,您将看到订户列表,在“推送信息”下将看到“重试”和“重试延迟” '。
What do these mean?
这些是什么意思?
Retries: The default value is 3, which means Iron will retry the message 3 times.
重试 :默认值为3,表示Iron将重试该消息3次。
Retries Delay: The default value is 60, which means Iron will by default push the message again to subscribers after 60 seconds if it thinks the message was not processed successfully.
重试延迟 :默认值为60,这意味着Iron如果认为未成功处理该消息,则默认在60秒后将消息再次推送给订阅者。
When does Iron retry a message?:
Iron何时重试消息? :
When it doesn’t get a response from your application:
如果它没有从您的应用程序得到响应:
- Your application failed to give a response because of some error. 您的应用程序由于某些错误而未能给出响应。
- Your application is still processing the job, a long running process. 您的应用程序仍在处理作业,这是一个长期运行的过程。
When it gets an error in response:
当收到错误消息时:
- Your app responded with 4xx or 5xx error. 您的应用回应了4xx或5xx错误。
When you send a 202 response:
当您发送202响应时:
- You are asking Iron to resend the message after a delay. 您要求Iron在延迟后重新发送该消息。
If you check the output of status (see above) you will notice the following line:
如果检查状态输出(请参见上文) ,则会注意到以下行:
public 'status' => string 'deleted'
This is how we will get to know the status of the message we pushed. The possible values for status (currently) are:
这是我们如何了解所推送消息的状态的方法。 状态的可能值(当前)为:
retrying – The job will be sent to endpoint i.e., subscriber (as many times as ‘Retries’ is set).
重试 –作业将发送到端点(即订户)( 与“重试”的设置次数相同 )。
deleted – The job is deleted.
已删除 –作业被删除。
reserved – The job will be retried after timeout.
保留 –超时后将重试该作业。
error – There was an error and nothing more will be done.
错误 –发生错误,将无法执行任何其他操作。
You have to send a 200 response to delete a message.
您必须发送200答复才能删除消息。
One has to remember that Iron will retry a message after timeout if it doesn’t get a response (it will retry after timeout as many times as you specify in ‘retries_total’).
必须记住,如果Iron没有得到响应,它将在超时后重试消息(超时后,它会重试“ retries_total”中指定的次数)。
Can we change these parameters? Of course!
我们可以更改这些参数吗? 当然!
While posting a message you can mention timeout (long running jobs may need more than a minute)
在发布消息时,您可以提及超时(长时间运行的工作可能需要一分钟以上)
$this->ironmq->postMessages('testing', "data", array(
"timeout" => 300 // Wait for 300 seconds = 5 minutes
));
If you want to decrease or increase the number of retries, you have to call the updateQueue method.
如果要减少或增加重试次数,则必须调用updateQueue方法。
$params = array(
"retries" => 5
);
$this->ironmq->updateQueue('testing', $params);
Can we change the status of a message which is in the queue? Of course! That is how you are supposed to use queues and in fact you are already doing that.
我们可以更改队列中消息的状态吗? 当然! 那就是您应该使用队列的方式,实际上您已经在使用队列了。
Let’s see how we can alter the status of a message:
让我们看看如何更改消息的状态:
retrying – This status is set when you push a message.
重试 -推送消息时设置此状态。
deleted – This status is set when the subscriber responds with a 200.
已删除 –当用户回复200时,将设置此状态。
reserved – This status is set when the subscriber responds with a 202.
保留 –当用户响应202时,将设置此状态。
error – If Iron exhausted number of retries and if it still doesn’t get a 200 response, the status is set to error.
错误 –如果Iron用尽了重试次数,但仍未收到200响应,则状态将设置为error。
Note: When your application responds with 4xx or 5xx errors (which usually means something went wrong with the server) Iron waits longer than the mentioned timeout.
注意:当您的应用程序以4xx或5xx错误响应(这通常意味着服务器出了点问题)时,Iron的等待时间超过了所提到的超时时间。
结论 (Conclusion)
Push queues aren’t rocket science when you look at them step by step. Try it yourself and tell us what you think in the comments below! I sincerely hope this helped you understand this often intimidating topic. I highly recommend you to read: http://dev.iron.io/mq/reference/push_queues/. Note that you can find the the source code for this article at: https://github.com/rajivseelam/laravel-queues.
逐步了解推式队列并不是火箭科学。 自己尝试一下,并在下面的评论中告诉我们您的想法! 我衷心希望这可以帮助您理解这个经常令人生畏的话题。 我强烈建议您阅读: http : //dev.iron.io/mq/reference/push_queues/ 。 请注意,您可以在以下位置找到本文的源代码: https : //github.com/rajivseelam/laravel-queues 。
Thanks for reading!
谢谢阅读!
翻译自: https://www.sitepoint.com/ironmq-laravel-delays-retries/