介绍 (Introduction)
If you want to create a blog using MongoDB and PHP, this article will teach you to:
如果您想使用MongoDB和PHP创建博客,那么本文将教您:
- Connect to a MongoDB database 连接到MongoDB数据库
- Save documents in a collection 将文档保存在集合中
- Query documents in a collection 查询集合中的文档
- Perform range queries 执行范围查询
- Sort documents, update a document, delete one or more documents from a collection 对文档进行排序,更新文档,从集合中删除一个或多个文档
The reason I chose to build a blog application is because it is a basic CRUD application and it is very suitable for easing into PHP and MongoDB web development. We will build a plain user interface using Bootstrap with simple textboxes and buttons. A MongoDB database will store all the content. You can download full source from github, see a demo frontend here and try the demo app’s backend with the user name and password being duythien.
我选择构建博客应用程序的原因是因为它是基本的CRUD应用程序,非常适合简化PHP和MongoDB Web开发。 我们将使用带有简单文本框和按钮的Bootstrap构建简单的用户界面。 MongoDB数据库将存储所有内容。 您可以从github下载完整的源代码, 在此处查看演示前端,并尝试使用用户名和密码为duythien的演示应用程序的后端 。
什么是MongoDB (What is MongoDB)
According to the official website MongoDB is a document database that provides high performance, high availability, and easy scalability. MongoDB falls into the group of documents-oriented NoSQL databases. For other subtypes of NoSQL databases, see here.
根据官方网站, MongoDB是一个文档数据库,可提供高性能,高可用性和易扩展性。 MongoDB属于面向文档的NoSQL数据库。 有关NoSQL数据库的其他子类型,请参见此处 。
MongoDB概念:数据库,集合和文档 (MongoDB Concepts: Databases, Collections, and Documents)
Database: MongoDB groups data into databases in the very same way as most relational databases do. If you have any experience with relational databases, you should think of these the same way. In an RDBMS, a database is a set of tables, stored procedures, views, and so on. In MongoDB, a database is a set of collections. A MongoDB database contains one or more collections. For example, a database for a blogging application named blog may typically have the collections articles, authors, comments, categories, and so on.
数据库:MongoDB与大多数关系数据库一样,将数据分组到数据库中。 如果您对关系数据库有任何经验,则应该以相同的方式思考这些问题。 在RDBMS中,数据库是一组表,存储过程,视图等。 在MongoDB中,数据库是一组集合。 MongoDB数据库包含一个或多个集合。 例如,用于名为blog的blogging应用程序的数据库通常可能具有馆藏文章,作者,评论,类别等。
Collection: A collection is the equivalent of an RDBMS table. A collection exists within a single database. Collections do not enforce a schema. Documents within a collection can have different fields. Typically, all documents in a collection have a similar or related purpose.
集合:集合等效于RDBMS表。 集合存在于单个数据库中。 集合不强制执行架构。 集合中的文档可以具有不同的字段。 通常,集合中的所有文档都具有相似或相关的目的。
Documents: A record in a MongoDB collection and the basic unit of data in MongoDB. Documents are analogous to JSON objects but exist in the database in a more type-rich format known as BSON. A document contains a set of fields or key-value pairs. The best way to think of a document is as a multidimensional array. In an array, you have a set of keys that map to values (Document == Array). See Documents.
文档:MongoDB集合中的记录和MongoDB中的基本数据单元。 文档类似于JSON对象,但是以类型更丰富的格式(称为BSON)存在于数据库中。 文档包含一组字段或键值对。 认为文档的最佳方法是多维数组。 在数组中,您有一组映射到值的键(文档==数组) 。 请参阅文档 。
安装MongoDB (Installing MongoDB)
MongoDB runs on most platforms and supports 32-bit and 64-bit architectures. MongoDB is available as a binary, or as a package. In production environments, use 64-bit MongoDB binaries. This section will cover installation on Ubuntu Linux and Windows. For other operating systems, please see their documentation.
MongoDB在大多数平台上运行,并支持32位和64位架构。 MongoDB可作为二进制文件或作为软件包使用。 在生产环境中,请使用64位MongoDB二进制文件。 本节将介绍在Ubuntu Linux和Windows上的安装。 对于其他操作系统,请参阅其文档。
This is how Mongo is installed on Ubuntu Linux. Open terminal and execute the following:
这就是Mongo在Ubuntu Linux上的安装方式。 打开终端并执行以下操作:
sudo apt-key adv --keyserver hkp://keyserver.ubuntu.com:80 --recv 7F0CEB10
#
echo 'deb http://downloads-distro.mongodb.org/repo/ubuntu-upstart dist 10gen' | sudo tee /etc/apt/sources.list.d/mongodb.list
Now issue the following command to update your repository and install the latest stable version of MongoDB:
现在发出以下命令来更新您的存储库并安装最新稳定版本的MongoDB:
sudo apt-get update
sudo apt-get install mongodb-10gen
Done, you have successfully installed MongoDB. Now start and stop service MongoDB via command line below.
完成,您已经成功安装了MongoDB。 现在,通过下面的命令行启动和停止服务MongoDB。
sudo service mongodb start
sudo service mongodb stop
In case of start error to try run the following command:
如果出现启动错误,请尝试运行以下命令:
sudo mongod --fork --logpath /var/log/mongodb/mongodb.log
#or
sudo mongod -f /etc/mongodb.conf
The following describes how to install it on Windows:
下面介绍如何在Windows上安装它:
Head on over to the downloads page on the MongoDB official website. Click on the download link for the latest stable release under Windows.
转到MongoDB官方网站上的下载页面。 单击下载链接以获取Windows下最新的稳定版本。
After the download is finished, extract and move it into C:\
. MongoDB requires a data folder in which to store its files. The default location for the MongoDB data directory is C:\data\db. If it doesn’t exist, create it.
下载完成后,解压缩并将其移至C:\
。 MongoDB需要一个数据文件夹来存储其文件。 MongoDB数据目录的默认位置是C:\ data \ db。 如果不存在,请创建它。
To start MongoDB, execute from the Command Prompt
要启动MongoDB,请从命令提示符处执行
C:\> cd \mongodb\bin
C:\mongodb\bin> mongod
Done, you have successfully installed MongoDB. Now start and stop service MongoDB via command line below.
完成,您已经成功安装了MongoDB。 现在,通过下面的命令行启动和停止服务MongoDB。
net start MongoDB
net stop MongoDB
为MongoDB安装PHP驱动程序 (Installing the PHP driver for MongoDB)
The MongoDB server is built to already work with your current web server, but not PHP. To make PHP talk to the MongoDB server, we are going to need the PHP-MongoDB driver. It is a PHP extension library.
MongoDB服务器已构建为可以与当前的Web服务器一起使用,但不适用于PHP。 为了使PHP与MongoDB服务器对话,我们将需要PHP-MongoDB驱动程序。 这是一个PHP扩展库。
If you use Linux install it easily via:
如果您使用Linux,则可以通过以下方式轻松安装:
sudo pecl install mongo
Add the line extension=mongo.so
to your php.ini configuration and you’re good to go:
将line extension=mongo.so
添加到您的php.ini配置中,您可以开始:
sudo -i
echo 'extension=mongo.so' >> /etc/php5/apache2/php.ini
Restart your web server and verify via command line:
重新启动您的Web服务器并通过命令行进行验证:
php -i |grep "mongo"
php --re mongo
在Windows上安装驱动程序 (Installing the Driver on Windows)
Let us try installing the driver on a Windows machine running PHP 5.4 on Apache (XAMPP):
让我们尝试在Apache(XAMPP)上运行PHP 5.4的Windows计算机上安装驱动程序:
Download the ZIP archive https://github.com/mongodb/mongo-php-driver/downloads on your machine and extract it.
在您的计算机上下载ZIP存档https://github.com/mongodb/mongo-php-driver/downloads并解压缩。
- Copy the php_mongo.dll file from the extracted folder to the PHP extension directory(C:\xampp\php\ext). 将php_mongo.dll文件从提取的文件夹复制到PHP扩展目录(C:\ xampp \ php \ ext)。
- Open the php.ini file inside your PHP installation and add the following line: extension=php_mongo.dll 在您PHP安装中打开php.ini文件,并添加以下行:extension = php_mongo.dll
- Save the file and close it. Restart the XAMP. 保存文件并关闭它。 重新启动XAMP。
Open up your text editor and add the following code to a new file:
<?php phpinfo();?>
, save the file as phpinfo.php inside the DocumentRoot of the Apache web server (htdocs) and open the PHP script in the browser. If you see mongo in the PHP info, the installation was successful.打开您的文本编辑器,然后将以下代码添加到新文件中:
<?php phpinfo();?>
,将文件另存为apache Web服务器(htdocs)的DocumentRoot中的phpinfo.php,然后在浏览器。 如果您在PHP信息中看到mongo,则说明安装成功。
用PHP进行Mongo Web开发 (Mongo Web Development with PHP)
连接到MongoDB数据库服务器 (Connecting to a MongoDB Database Server)
Connecting to MongoDB from PHP is very similar to connecting to any other database. The default host is localhost, and the default port is 27017.
从PHP连接到MongoDB与连接到任何其他数据库非常相似。 默认主机为localhost,默认端口为27017。
$connection = new Mongo();
Connecting to a remote host with optional custom port and auth:
使用可选的自定义端口和身份验证连接到远程主机:
$connecting_string = sprintf('mongodb://%s:%d/%s', $hosts, $port,$database),
$connection= new Mongo($connecting_string,array('username'=>$username,'password'=>$password));
选择数据库 (Selecting a Database)
Once the database server connection is established, we will use it to access a database. The defined way to do this is:
建立数据库服务器连接后,我们将使用它来访问数据库。 定义的方法是:
$dbname = $connection->selectDB('dbname');
基础知识(CRUD操作) (The Basics (CRUD Operations))
MongoDB provides rich semantics for reading and manipulating data. CRUD stands for create, read, update, and delete. These terms are the foundation for all interactions with the database.
MongoDB为读取和处理数据提供了丰富的语义。 CRUD代表创建,读取,更新和删除。 这些术语是与数据库进行所有交互的基础。
创建/选择集合 (Creating/Selecting a Collection)
Selecting and creating a collection is very similar to accessing and creating a database. If a collection does not exist, it is created:
选择和创建集合与访问和创建数据库非常相似。 如果不存在集合,则会创建它:
$collection = $dbname->collection;
//or
$collection = $dbname->selectCollection('collection');
For example, this creates the collection “posts” in my blog:
例如,这将在我的博客中创建集合“ posts”:
$posts = $dbname->posts
建立文件 (Creating a Document)
Creating a document in MongoDB could not be easier. Create an array. Pass it into the insert method on the collection object
在MongoDB中创建文档再简单不过了。 创建一个数组。 将其传递到集合对象的insert方法中
$post = array(
'title' => 'What is MongoDB',
'content' => 'MongoDB is a document database that provides high performance...',
'saved_at' => new MongoDate()
);
$posts->insert($post);
The insert()
method stores the data in the collection. The $post
array automatically receives a field named _id
, which is the autogenerated unique ObjectId of the inserted BSON document. You could also use the save()
method, which upserts – updates an existing record, or creates a new one if it doesn’t exist.
insert()
方法将数据存储在集合中。 $post
数组自动接收一个名为_id
的字段,该字段是插入的BSON文档的自动生成的唯一ObjectId。 您还可以使用save()
方法,该方法将更新-更新现有记录,或者创建一个不存在的新记录。
阅读文件 (Reading a Document)
To get data from a collection, I use the find()
method, which gets all the data in a collection. findOne()
returns only one document that satisfies the specified query criteria. The following examples will show you how to query one or more records.
为了从集合中获取数据,我使用find()
方法,该方法获取集合中的所有数据。 findOne()
仅返回一个满足指定查询条件的文档。 以下示例将向您展示如何查询一个或多个记录。
// all records
$result = $posts::find();
// one record
$id = '52d68c93cf5dc944128b4567';
$results = $posts::findOne(array('_id' => new MongoId($id)));
更新文件 (Updating a Document)
Modifies an existing document or documents in a collection. By default, the update()
method updates a single document. If the multi option is set to true, the method updates all documents that match the query criteria.
修改现有文档或集合中的文档。 默认情况下, update()
方法更新单个文档。 如果multi选项设置为true,则该方法将更新所有符合查询条件的文档。
$id = '52d68c93cf5dc944128b4567';
$posts->update(
array('_id' => new MongoId($id)),
array('$set' => array(title' => 'What is phalcon'))
);
The update() method takes two parameters. The first is criteria to describe the objects to update and the second the object with which to update the matching records. There is also a third optional parameter whereby you can pass in an array of options.
update()方法采用两个参数。 第一个是描述要更新的对象的条件,第二个是用来更新匹配记录的对象的条件。 还有第三个可选参数,您可以在其中传递一组选项。
迷你博客 (Mini blog)
The structure of the project we’ll be building:
我们将要构建的项目的结构:
|-------admin
|------index.php # This is dashboard
|------auth.php
|-------view
|------admin
|-------create.view.php #form create article
|-------dashbroad.view.php
|------index.view.php
|------layout.php #This is main layout it will be shown for every action executed within the app
|------single.view.php # This is view for a single article
|-------config.php # Define paramater database, url
|-------app.php # Include multi-php files
|-------db.php # Container class db
|-------index.php
|-------single.php
|-------static # Container css,js...
|-----css
|-----js
|-------vendor
|----markdown # Library converts markdown into html
Before we start with our actual PHP code we need to create our files and folders like above.
在开始实际PHP代码之前,我们需要创建上述文件和文件夹。
config.php (config.php)
This is your configuration file that tells our app how to connect to the database. This is where you have defined the database name, username and password of the user to access that database:
这是您的配置文件,它告诉我们的应用程序如何连接到数据库。 在此定义了用于访问该数据库的用户的数据库名称,用户名和密码:
<?php
define('URL', 'http://duythien.dev/sitepoint/blog-mongodb');
define('UserAuth', 'duythien');
define('PasswordAuth', 'duythien');
$config = array(
'username' => 'duythien',
'password' => 'qazwsx2013@',
'dbname' => 'blog',
'connection_string'=> sprintf('mongodb://%s:%d/%s','127.0.0.1','27017','blog')
);
where we define paramaters UserAuth and PasswordAuth to protect the admin folder via HTTP authentication. We’re using HTTP auth for simplicity here, seeing as the central theme of the article is connecting to MongoDB – you would usually use some sort of decent framework with ACL to build in access control.
我们定义参数UserAuth和PasswordAuth来通过HTTP身份验证保护admin文件夹。 在这里,为了简化起见,我们使用HTTP auth,因为本文的中心主题是连接到MongoDB –您通常会使用带有ACL的某种体面的框架来构建访问控制。
app.php:
app.php:
<?php
include 'config.php';
include 'layout.php';
include 'db.php';
use Blog\DB,
Blog\Layout;
// Constructor to the db
$db = new DB\DB($config);
// Constructor to layout view
$layout = new Layout\Layout();
管理员 (admin)
This is the folder that contains the CRUD code.
这是包含CRUD代码的文件夹。
<?php
require_once 'auth.php';
require_once '../app.php';
require_once '../vendor/markdown/Markdown.inc.php';
use \Michelf\MarkdownExtra,
\Michelf\Markdown;
if ((is_array($_SESSION) && $_SESSION['username'] == UserAuth)) {
$data = array();
$status = (empty($_GET['status'])) ? 'dashboard' : $_GET['status'];
switch ($status) {
//create post
case 'create':
break;
//edit post
case 'edit':
break;
//delete post
case 'delete':
//display all post in dashboard
default:
$currentPage = (isset($_GET['page'])) ? (int)$_GET['page'] : 1;
$data = $db->get($currentPage, 'posts');
$layout->view('admin/dashboard', array(
'currentPage' => $data[0],
'totalPages' => $data[1],
'cursor' => $data[2]
));
break;
}
}
For the full file index.php see here. Above I used the view function in the class layout.php
which will automatically load dashboard.view.php
.
有关完整文件index.php的信息,请参见此处 。 上面我在class layout.php
类中使用了view函数,它将自动加载dashboard.view.php
。
<?php namespace Blog\Layout;
Class Layout
{
/**
* @var array
*/
public $data;
public function view($path, $data)
{
if (isset($data)) {
extract($data);
}
$path .= '.view.php';
include "views/layout.php";
}
}
The GET parameter status
corresponds to a CRUD action. For example, when status is “create”:
GET参数status
对应于CRUD操作。 例如,当状态为“创建”时:
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$article = array();
$article['title'] = $_POST['title'];
$article['content'] = Markdown::defaultTransform($_POST['content']);
$article['saved_at'] = new MongoDate();
if (empty($article['title']) || empty($article['content'])) {
$data['status'] = 'Please fill out both inputs.';
} else {
// then create a new row in the collection posts
$db->create('posts', $article);
$data['status'] = 'Row has successfully been inserted.';
}
}
$layout->view('admin/create', $data);
Function view(‘admin/create’, $data)
shows an HTML form where the user can write the title/content of a new blog post, or it saves the user-submitted data to MongoDB. By default the script displays the following HTML form:
函数view('admin/create', $data)
显示一个HTML表单,用户可以在其中编写新博客文章的标题/内容,或者将用户提交的数据保存到MongoDB。 默认情况下,脚本显示以下HTML形式:
<form action="" method="post">
<div><label for="Title">Title</label>
<input type="text" name="title" id="title" required="required"/>
</div>
<label for="content">Content</label>
<p><textarea name="content" id="content" cols="40" rows="8" class="span10"></textarea></p>
<div class="submit"><input type="submit" name="btn_submit" value="Save"/></div>
</form>
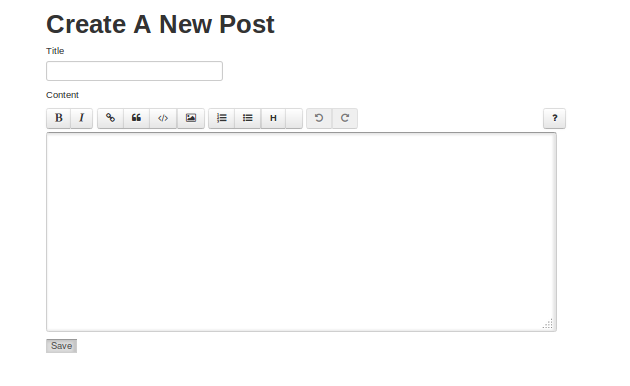
Next let’s look at db.php
, which can be found in full here
接下来让我们看一下db.php
,可以在这里找到完整内容
<?php
namespace Blog\DB;
class DB
{
/**
* Return db
* @var object
*/
private $db;
/**
* Results limit.
* @var integer
*/
public $limit = 5;
public function __construct($config)
{
$this->connect($config);
}
//connecting mongodb
private function connect($config)
{
}
//get all data
public function get($page, $collection)
{
}
//get one data by id
public function getById($id, $collection)
{
}
//create article
public function create($collection, $article)
{
}
//delete article by id
public function delete($id, $collection)
{
}
//update article
public function update($id, $collection, $acticle)
{
}
//create and update comment
public function commentId($id, $collection, $comment)
{
}
}
The MongoDB cursor makes pagination easy. These cursor methods can be chained off of the cursor object that find returns and each other. Combining limit with skip makes pagination easy. These can also be combined with order. For example.
MongoDB光标使分页变得容易。 这些游标方法可以与查找返回的游标对象相互链接。 将限制与跳过结合使用可使分页变得容易。 这些也可以与订单组合。 例如。
public function get($page,$collection){
$currentPage = $page;
$articlesPerPage = $this->limit;
//number of article to skip from beginning
$skip = ($currentPage - 1) * $articlesPerPage;
$table = $this->db->selectCollection($collection);
$cursor = $table->find();
//total number of articles in database
$totalArticles = $cursor->count();
//total number of pages to display
$totalPages = (int) ceil($totalArticles / $articlesPerPage);
$cursor->sort(array('saved_at' => -1))->skip($skip)->limit($articlesPerPage);
$data=array($currentPage,$totalPages,$cursor);
return $data;
}
index.php
: template files can be found in the view folder; such as index.view.php
. Here is an example of index.php
:
index.php
:模板文件可以在view文件夹中找到; 例如index.view.php
。 这是index.php
的示例:
<?php
require 'app.php';
try {
$currentPage = (isset($_GET['page'])) ? (int)$_GET['page'] : 1; //current page number
$data = $db->get($currentPage, 'posts');
$layout->view('index', array(
'currentPage' => $data[0],
'totalPages' => $data[1],
'cursor' => $data[2],
'name' => 'Duy Thien'
));
} catch (Exception $e) {
echo 'Caught exception: ', $e->getMessage(), "\n";
}
Open your browser and navigate to http://duythien.dev/sitepoint/blog-mongodb
. It lists all the current articles in the blog:
打开浏览器,然后浏览至http://duythien.dev/sitepoint/blog-mongodb
。 它列出了博客中的所有当前文章:
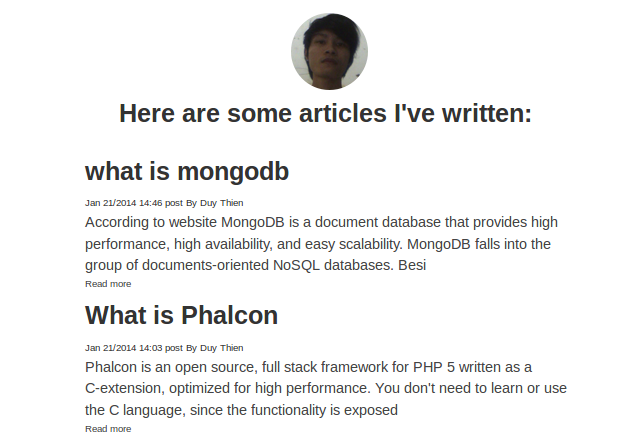
single.php
: When you view a single post page (click Read more on a post), you are looking at single.view.php
in the views folder. Here is the logic of single.php
:
single.php
:当您查看单个帖子页面(单击帖子的更多内容)时,您正在查看views文件夹中的single.view.php
。 这是single.php
的逻辑:
<?php
require 'app.php';
// Fetch the entire post
$post = $db->getById($_GET['id'], 'posts');
if ($post) {
$layout->view('single', array(
'article' => $post
));
}
This file receives the _id
of the article as an HTTP GET parameter. We invoke the findOne()
method on the articles collection, sending the _id
value as a parameter to the method. The findOne()
method is used to retrieve a single document. See function getById()
in file db.php
该文件接收文章的_id
作为HTTP GET参数。 我们在articles集合上调用findOne()
方法,将_id
值作为参数发送给该方法。 findOne()
方法用于检索单个文档。 请参见文件db.php
函数getById()
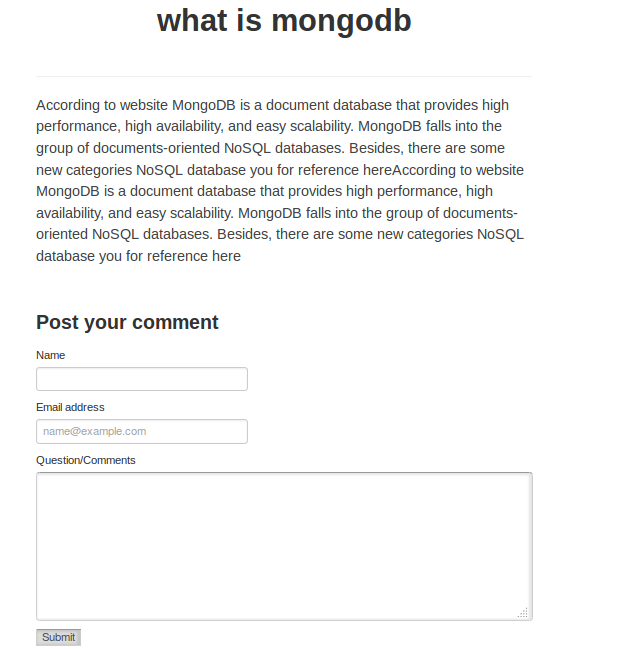
Enter an arbitrary Name and Email in the input boxes under the comments section, put some text in the textarea as well. Then click on the Save button and the page will reload with the comment you just posted. This is what comment.php
looks like:
在评论部分下的输入框中输入任意名称和电子邮件,并在文本区域中也输入一些文本。 然后单击“保存”按钮,页面将重新加载您刚刚发布的评论。 这是comment.php
样子:
<?php
require 'app.php';
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$id = $_POST['article_id'];
$comment = array(
'name' => $_POST['fName'],
'email' => $_POST['fEmail'],
'comment' => $_POST['fComment'],
'posted_at' => new MongoDate()
);
$status = $db->commentId($id, 'posts', $comment);
if ($status == TRUE) {
header('Location: single.php?id=' . $id);
}
}
Comments for an article are stored in an array field of the document name comments
. Each element of a comment is an embedded document that contains several fields.
文章的注释存储在文档名称comments
的数组字段中。 注释的每个元素都是一个嵌入式文档,其中包含多个字段。
结论 (Conclusion)
In this article, we covered a basic CRUD introduction into PhP with MongoDB. We’ve even created a sort of very primitive MVC in the process (see full app on Github). It’s up to you to use a proper framework, implement authentication beyond the simple HTTP auth used here, and add more functionality, but the basics are in place and you can hack away at this demo application to your heart’s content.
在本文中,我们介绍了MongoDB对PhP的基本CRUD简介。 我们甚至在此过程中创建了一种非常原始的MVC(请参阅Github上的完整应用程序)。 您可以使用适当的框架,在此处使用的简单HTTP身份验证之外实现身份验证并添加更多功能,但是基础知识已经就位,您可以根据自己的实际情况来使用此演示应用程序。
For more information on MongoDB check out the online documentation. Did you enjoy this article? Let us know your thoughts!
有关MongoDB的更多信息,请查看在线文档 。 你喜欢这篇文章吗? 让我们知道您的想法!
翻译自: https://www.sitepoint.com/building-simple-blog-app-mongodb-php/