使用codeigniter
In the previous part of this series we learned what Aspect Oriented Programming (AOP) is and the meaning of important AOP terminology. In this part I’ll explain why we need AOP using practical examples and how to add AOP behavior to your PHP application using the CodeIgniter framework.
在本系列的前一部分中,我们了解了什么是面向方面的编程(AOP)以及重要的AOP术语的含义。 在这一部分中,我将使用实际示例解释为什么我们需要AOP,以及如何使用CodeIgniter框架向您PHP应用程序添加AOP行为。
实用的跨领域关注 (Practical Cross-Cutting Concerns)
First, let’s take a look at some of the cross-cutting concerns that can be found in almost every web application.
首先,让我们看一下几乎每个Web应用程序中都可以找到的一些横切关注点。
记录中 (Logging)
Logging is one of the most essential functions in a web application since it allows us to trace the code execution flow. When a bug occurs in a live application, we cannot change any code to identify the bugs. Log files are often the only available resource for finding these bugs.
日志记录是Web应用程序中最重要的功能之一,因为它使我们能够跟踪代码执行流程。 当实时应用程序中发生错误时,我们无法更改任何代码以识别错误。 日志文件通常是查找这些错误的唯一可用资源。
Writing log messages to a file or to the database is the most commonly used method. The information you write to the log may vary depending on the type of application and the logic you’re logging. Some generic points worth capturing are entering into functions, returning from functions, and returning with errors.
将日志消息写入文件或数据库是最常用的方法。 您写入日志的信息可能会有所不同,具体取决于应用程序的类型和所记录的逻辑。 值得捕获的一些通用要点是进入函数,从函数返回以及有错误返回。
<?php
function addPost() {
$log->writeToLog("Entering addPost");
// Business Logic
$log->writeToLog("Leaving addPost");
}
If we think from AOP perspective, the above situation is equal to both before and after advices. Hence around advice would be most suitable for logging.
如果从AOP的角度来看,上述情况与之前和之后的建议相同。 因此,周围的建议将最适合记录日志。
认证与授权 (Authentication and Authorization)
Authentication is the process of identifying the validity of a user inside the system. We often use a username and password at a basic level for authentication. Authorization is the procedure of validating access to specific sections in the system. We need to apply both authentication and authorization in many applications.
身份验证是识别系统内部用户有效性的过程。 我们通常在基本级别上使用用户名和密码进行身份验证。 授权是验证对系统中特定部分的访问的过程。 我们需要在许多应用程序中同时应用身份验证和授权。
<?php
function addPost() {
$authentication->validateAuthentication($user);
$authorization->validateAccess($user);
// business logic
}
Authentication and authorization are generally required at the beginning of the business logic. First the user should be logged into the system and then he should have the necessary permission to add posts. These are cross-cutting concerns since they have to be applied to certain set of methods. We would require before advice in the AOP context for Authentication and Authorization.
通常在业务逻辑开始时需要认证和授权。 首先,用户应该登录到系统,然后他应该具有添加帖子的必要权限。 这些是跨领域的问题,因为必须将它们应用于某些方法。 我们将需要在AOP上下文中获得有关身份验证和授权的建议。
交易管理 (Transaction Management)
Transaction management ensures the consistency and integrity of the data in your database. Consider a situation where you have multiple dependent functions that saves the data to the database inside a single process. If one function fails and the others execute successfully, your database will be corrupted. Hence it’s important use transaction handling in every application.
事务管理可确保数据库中数据的一致性和完整性。 考虑一种情况,在这种情况下,您需要多个相关函数,这些函数会将数据保存到单个进程中。 如果一个函数失败而其他函数成功执行,则数据库将被破坏。 因此,在每个应用程序中使用事务处理很重要。
<?php
function addPost() {
$tx->beginTransaction();
try{
// Business logic
$tx->commit();
}
catch (Exception $e) {
$tx->rollback();
}
}
We commit the data when all of the function calls are successfully completed, and have a rollback operation if something fails. In the AOP context, it will be before advice, after advice and after throwing advice respectively. Before and after advices are common in most AOP frameworks. After throwing advice can be found in advanced AOP frameworks.
当所有函数调用成功完成后,我们将提交数据,如果失败则执行回滚操作。 在AOP上下文中,分别是在建议之前,建议之后和抛出建议之后。 在大多数AOP框架中,前后建议很常见。 提出建议后,可以在高级AOP框架中找到建议。
AOP的工作原理 (How AOP Works)
AOP is still not popular in the PHP community, but full-featured AOP frameworks are available for languages like JAVA. In AOP we have the core business logic class on one side and aspects on the other side. These aspects can be applied to core classes at compile time or run time. Frameworks like AspectJ applies AOP at compile time. Since PHP is an interpreted language, we don’t have to worry about applying AOP at compile time, and so it makes more sense to apply AOP at runtime.
AOP在PHP社区中仍然不流行,但是功能齐全的AOP框架可用于JAVA之类的语言。 在AOP中,我们一方面具有核心业务逻辑类,另一方面具有方面。 这些方面可以在编译时或运行时应用于核心类。 诸如AspectJ之类的框架在编译时会应用AOP。 由于PHP是一种解释型语言,因此我们不必担心在编译时应用AOP,因此在运行时应用AOP更有意义。
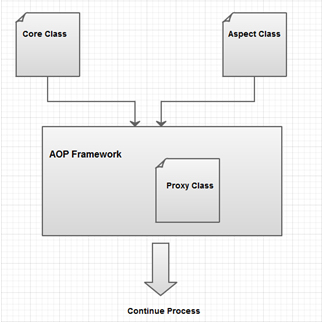
As you can see, the core logic and cross-cutting concerns are separated using two PHP classes. Then the AOP framework combines both core and aspect classes at runtime and creates a dynamic proxy class. Dynamic classes contain the advice function calls before and after the actual method class.
如您所见,使用两个PHP类将核心逻辑和跨领域关注点分开。 然后,AOP框架在运行时将核心类和方面类结合在一起,并创建一个动态代理类。 动态类包含在实际方法类之前和之后的advice函数调用。
There are two types of techniques commonly used in combining aspects with core logic: XML-based configuration and annotation-based configuration.
将方面与核心逻辑结合在一起时通常使用两种技术:基于XML的配置和基于注释的配置。
In XML-based configurations, all the AOP rules for applying cross-cutting concerns are documented in a XML file.
在基于XML的配置中,所有用于应用跨领域关注点的AOP规则都记录在XML文件中。
In annotation-based configurations, the rules are defined in-line with the actual methods using annotations. We can use a predefined document comment structure to replace annotations in PHP.
在基于注释的配置中,规则是使用注释与实际方法一致地定义的。 我们可以使用预定义的文档注释结构来替换PHP中的注释。
为什么选择CodeIgniter (Why CodeIgniter )
You might be wondering why I chose CodeIgniter to explain AOP since it doesn’t provide any support for AOP functionality. I did so on purpose to create basic AOP functionality from scratch to help you better understand the concepts and process. If I choose a framework with AOP support, then I would only be able to explain the rule structures and how it works. You wouldn’t be able to apply your knowledge to new AOP frameworks since you don’t understand the basics. Since we are creating everything from scratch here, you’ll be able to adapt to any new framework without much hassle, or even create your own AOP framework someday.
您可能想知道为什么我选择CodeIgniter来解释AOP,因为它不提供对AOP功能的任何支持。 我这样做的目的是从头开始创建基本的AOP功能,以帮助您更好地理解概念和过程。 如果选择支持AOP的框架,那么我将只能解释规则结构及其工作方式。 由于您不了解基础知识,因此您将无法将其知识应用于新的AOP框架。 由于我们在这里从头开始创建所有内容,因此您可以轻松适应任何新框架,甚至有一天可以创建自己的AOP框架。
CodeIgniter hooks is another reason which prompted me to choose this particular framework. When a method is called you have to create the proxy class. In AOP frameworks this process is not visible and very complex and hard to explain. CodeIgniter hooks allow us to trigger custom code at certain predefined hook points. Using this, we can trigger custom code on each method call and thus execute AOP functionality. I won’t create proxy classes since creating such functionality requires a lots of coding and is beyond the scope of this tutorial.
CodeIgniter挂钩是促使我选择此特定框架的另一个原因。 调用方法时,您必须创建代理类。 在AOP框架中,此过程不可见,非常复杂且难以解释。 CodeIgniter挂钩允许我们在某些预定义的挂钩点触发自定义代码。 使用此方法,我们可以在每个方法调用上触发自定义代码,从而执行AOP功能。 我不会创建代理类,因为创建此类功能需要大量的编码,并且超出了本教程的范围。
使用CodeIgniter挂钩 (Using CodeIgniter Hooks)
The CodeIgniter website explains that the hooks feature “provides a means to tap into and modify the inner workings of the framework without hacking the core files.” You can enable hooks by setting the enable_hooks
parameter in the config file to true.
CodeIgniter网站解释说,钩子功能“提供了一种在不破坏核心文件的情况下利用并修改框架的内部工作方式的方法。” 您可以通过将配置文件中的enable_hooks
参数设置为true来启用挂钩。
There are list of predefined hook points available, but I am just going to explain the hooks necessary for our application. They are:
有可用的预定义挂钩点列表,但是我将解释我们的应用程序必需的挂钩。 他们是:
pre_controller
– called immediately prior to any of your controllers being calledpre_controller
–在调用任何控制器之前立即调用post_controller
– called immediately after your controller is fully executedpost_controller
–控制器完全执行后立即调用
Let’s setup a hook to call custom code.
让我们设置一个挂钩来调用自定义代码。
<?php
$hook["pre_controller"] = array(
"class" => "AOPCodeigniter",
"function" => "applyBeforeAspects",
"filename" => "AOPCodeigniter.php",
"filepath" => "hooks"
);
Place the above code in the hooks.php
file in the config
directory. Then create the AOPCodeigniter
class in the application/hooks
folder. Now each time a request is made, the class’ applyBeforeAspects()
method will be called before the actual controller method. This is will enable us to combine before advices with core logic (in an actual AOP framework, this will be done using the proxy class).
将上面的代码放在config
目录的hooks.php
文件中。 然后在application/hooks
文件夹中创建AOPCodeigniter
类。 现在,每次发出请求时,将在实际的控制器方法之前调用类的applyBeforeAspects()
方法。 这将使我们能够将建议与核心逻辑结合起来(在实际的AOP框架中,这将使用代理类来完成)。
We can use the following code to apply after advices in the same manner as the above code:
我们可以使用以下代码以与上述代码相同的方式根据建议进行应用:
<?php
$hook["post_controller"] = array(
"class" => "AOPCodeigniter",
"function" => "applyAfterAspects",
"filename" => "AOPCodeigniter.php",
"filepath" => "hooks"
);
摘要 (Summary)
In this part of the series we’ve learned how to identify where AOP can be used considering practical scenarios of real projects, and I have introduced CodeIgniter as a context for creating basic AOP functionality. Now that we have completed the theoretical sections, in the next part I’ll show you how to use both XML and annotation-based methods to create your own rules structures and how to process those rules to apply advices.
在本系列的这一部分中,我们学习了如何考虑实际项目的实际情况来确定可以在哪里使用AOP,并且我介绍了CodeIgniter作为创建基本AOP功能的上下文。 既然我们已经完成了理论部分,那么在下一部分中,我将向您展示如何同时使用XML和基于注释的方法来创建自己的规则结构,以及如何处理这些规则以应用建议。
Image via Fotolia
图片来自Fotolia
翻译自: https://www.sitepoint.com/explore-aspect-oriented-programming-with-codeigniter-2/
使用codeigniter