One requirement that never changes whether you are working on your projects alone or in a team, on small projects or big, is Code Quality. The bigger the project and the team, the harder it gets to maintain it.
无论是单独还是团队工作,无论是小型项目还是大型项目,一个不变的要求就是代码质量。 项目和团队越大,维护它就越困难。
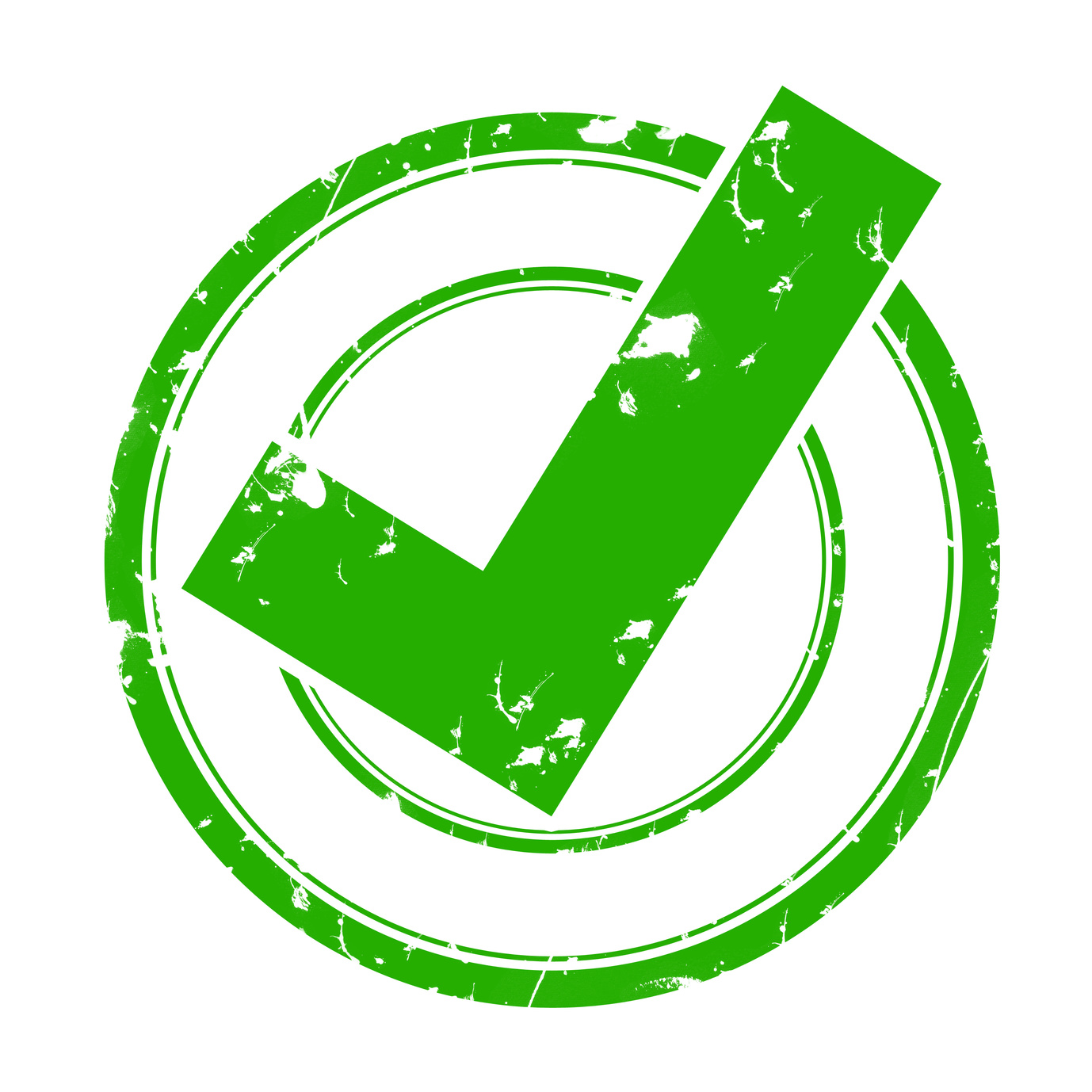
A good way of slowing this increase in difficulty down is to use static analysis tools. Static analysis is the process of analyzing software without actually executing the program – a sort of automatic code review. Static analysis tools will detect common errors, enforce coding standards, and even clean up code blocks. The days of php -l filename
are not over, but we now have a number of great tools that go the extra mile in helping us create and maintain high quality code.
降低难度增加的一个好方法是使用静态分析工具。 静态分析是在没有实际执行程序的情况下分析软件的过程-一种自动代码审查。 静态分析工具将检测常见错误,执行编码标准,甚至清理代码块。 php -l filename
的日子还没有结束,但是我们现在有了许多出色的工具,可以帮助我们创建和维护高质量的代码。
Speaking of php -l filename
, the good old PHP lint, it’s what will execute a syntax analysis on the target file and output any errors it finds. I used to have this little piece of code that I used on and off to send emails with PHP. It is a good starting point for our analysis.
说到php -l filename
,这是一个很好的旧PHP lint,它将对目标文件执行语法分析并输出发现的任何错误。 我曾经有过这样的小段代码,可以用来通过PHP发送电子邮件。 这是我们分析的良好起点。
<?php
class Email{
//Constructor
function Email( $subject, $message, $senderName, $senderEmail, $toList, $ccList=0, $bccList=0, $replyTo=0 ){
$this->sender = $senderName . " <$senderEmail>";
$this->replyTo = $replyTo;
$this->subject = $subject;
$this->message = $message;
// Set the To recipients
if( is_array($toList)){
$this->to = join( $toList, "," );
}else{
$this->to = $toList;
}
// Set the cc list
if( is_array($ccList) && sizeof($ccList)){
$this->cc = join( $ccList, "," );
}else{
$this->cc = $ccList;
}
// Set the bcc list
if( is_array($bccList) && sizeof($bccList)){
$this->bcc = join( $bccList, "," );
}else{
$this->bcc = $bccList;
}
}
function sendMail(){
//create the headers for PHP mail() function
$this->headers = "From: " . $this->sender . "\n";
if( $this->replyTo ){
$this->headers .= "Reply-To: " . $this->replyTo . "\n";
}
if( $this->cc ){
$this->headers .= "Cc: " . $this->cc . "\n";
}
if( $this->bcc ){
$this->headers .= "Bcc: " . $this->bcc . "\n";
}
print "To: " . $this->to ."<br>Subject: " . $this->subject . "<br>Message: " . $this->message . "<br>Headers: " . $this->headers;
return mail( $this->to, $this->subject, $this->message, $this->headers );
}
}
As you can see, this is a simple email sending class. If we run our PHP lint on this code, we will see that everything is good.
如您所见,这是一个简单的电子邮件发送类。 如果在此代码上运行我们PHP皮棉,我们将看到一切都很好。
php -l Email.php
The result is the following:
结果如下:
No syntax errors detected in Email.php
No syntax errors detected in Email.php
In 2016, this result is not enough, because we also need to consider code quality and programming standards.
在2016年,这个结果还不够,因为我们还需要考虑代码质量和编程标准。
输入PHP Smart Analyzer (Enter PHP Smart Analyzer)
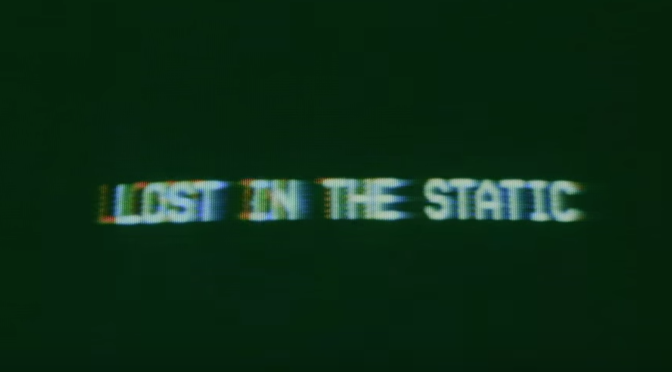
PHPSA is a static analysis tool for PHP.
PHPSA是用于PHP的静态分析工具。
PHPSA
can be installed as a .phar
, or through Composer, like so:
PHPSA
可以安装为.phar
或通过Composer安装 ,如下所示:
composer require ovr/phpsa
This will create a command line utility that will be symlinked to the vendor/bin
folder of our project.
这将创建一个命令行实用程序,该实用程序将符号链接到我们项目的vendor/bin
文件夹。
使用PHPSA (Using PHPSA)
After the installation is done, we can run ./vendor/bin/phpsa
.
安装完成后,我们可以运行./vendor/bin/phpsa
。
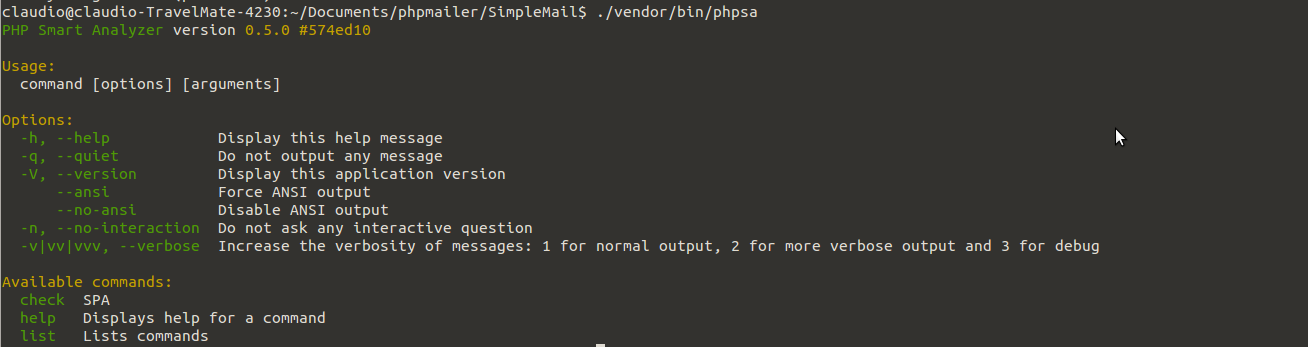
The result we get after the above executes is the same result as running the list
command. The help
command will list the instructions for running help on PHPSA. The check
command will execute the static analysis on a designated file or folder.
执行上述操作后得到的结果与运行list
命令的结果相同。 help
命令将列出在PHPSA上运行帮助的说明。 check
命令将在指定的文件或文件夹上执行静态分析。
Because we ran the PHP lint earlier, it is expected that PHPSA will not find any syntax errors on our code. But what happens if we insert an error on purpose? Will PHPSA be able to find it?
因为我们之前运行过PHP lint,所以预期PHPSA在我们的代码中不会发现任何语法错误。 但是,如果我们故意插入错误会怎样? PHPSA能够找到它吗?
Let’s make a slight change in our Email class.
让我们在Email类中稍作更改。
<?php
class Email{
//Constructor
function Email( $subject, $message, $senderName, $senderEmail, $toList, $ccList=0, $bccList=0, $replyTo=0 ){
$this->sender = $senderName . " <$senderEmail>";
$this->replyTo = $replyTo;
$this->subject = $subject;
$this->message = $message;
// Set the To recipients
if( is_array($toList)){
$this->to = join( $toList, "," );
}else{
$this->to = $toList;
}
// Set the cc list
if( is_array($ccList) && sizeof($ccList)){
$this->cc = join( $ccList, "," )
}else{
$this->cc = $ccList;
}
// Set the bcc list
if( is_array($bccList) && sizeof($bccList)){
$this->bcc = join( $bccList, "," );
}else{
$this->bcc = $bccList;
}
}
function sendMail(){
//create the headers for PHP mail() function
$this->headers = "From: " . $this->sender . "\n";
if( $this->replyTo ){
$this->headers .= "Reply-To: " . $this->replyTo . "\n";
}
if( $this->cc ){
$this->headers .= "Cc: " . $this->cc . "\n";
}
if( $this->bcc ){
$this->headers .= "Bcc: " . $this->bcc . "\n";
}
print "To: " . $this->to ."<br>Subject: " . $this->subject . "<br>Message: " . $this->message . "<br>Headers: " . $this->headers;
return mail( $this->to, $this->subject, $this->message, $this->headers );
}
}
This time around we have a clear syntax error in our code. Let’s run PHPSA and check the results.
这次,我们的代码中出现了明显的语法错误。 让我们运行PHPSA并检查结果。

As we can see, PHPSA is quick to detect a syntax error. But none of this is actually new, our simple PHP lint can detect this error, too. So let’s correct it and check what else PHPSA has in store for us.
如我们所见,PHPSA可以快速检测到语法错误。 但是,这些都不是真正的新东西,我们简单PHP皮棉也可以检测到此错误。 因此,让我们对其进行更正并检查PHPSA还为我们提供了什么。
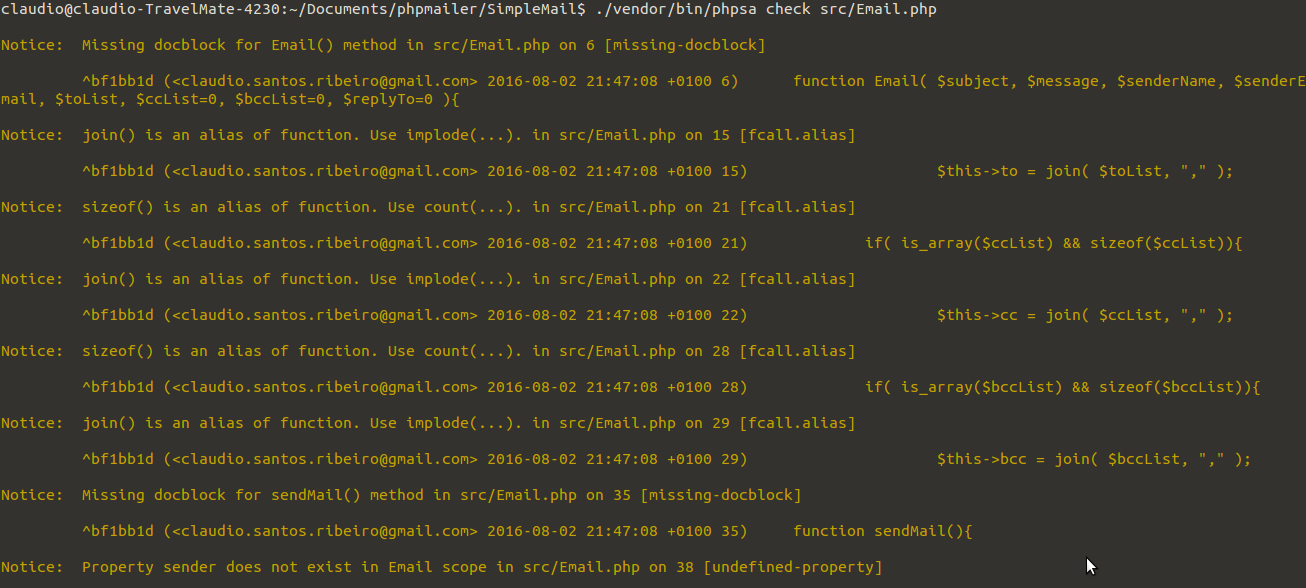
A lot of things to look at now!
现在有很多事情要看!
Notice: Missing docblock for Email() method in src/Email.php on 6 [missing-docblock]
Notice: join() is an alias of function. Use implode(...). in src/Email.php on 15 [fcall.alias]
Notice: sizeof() is an alias of function. Use count(...). in src/Email.php on 21 [fcall.alias]
Notice: Property sender does not exist in Email scope in src/Email.php on 38 [undefined-property]
PHPSA warns us about a lot of different things, many more than the ones listed above. From functions that are just aliases of others, undefined properties and missing docblocks, PHPSA does a great job suggesting the use of better coding principles.
PHPSA警告我们很多不同的事情,而不仅仅是上面列出的事情。 通过仅是其他函数的别名,未定义的属性和缺少的文档块的功能,PHPSA很好地建议使用更好的编码原理。
Let’s fix our code to correct all the above.
让我们修复代码以更正以上所有内容。
<?php
/**
* Simple email sender class
*
*/
class Email
{
/**
* The email headers
*/
var $headers;
/**
* The email sender
*/
var $sender;
/**
* The email recipients
*/
var $to;
/**
* Reply To
*/
var $replyTo;
/**
* Email cc list
*/
var $cc;
/**
* Email bcc list
*/
var $bcc;
/**
* Email content
*/
var $message;
/**
* The subject of the email
*/
var $subject;
/**
* This is the constructor for the Email class
*/
function Email(
$subject,
$message,
$senderName,
$senderEmail,
$toList,
$ccList = 0,
$bccList = 0,
$replyTo = 0
) {
$this->sender = $senderName . " <$senderEmail>";
$this->replyTo = $replyTo;
$this->subject = $subject;
$this->message = $message;
// Set the To recipients
if (is_array($toList)) {
$this->to = implode($toList, ",");
} else {
$this->to = $toList;
}
// Set the cc list
if (is_array($ccList) && count($ccList)) {
$this->cc = implode($ccList, ",");
} else {
$this->cc = $ccList;
}
// Set the bcc list
if (is_array($bccList) && count($bccList)) {
$this->bcc = implode($bccList, ",");
} else {
$this->bcc = $bccList;
}
}
/**
* The function that actually sends the email
*
* @return boolean Returns TRUE if the mail was successfully accepted for delivery, FALSE otherwise.
*/
function sendMail()
{
//create the headers for PHP mail() function
$this->headers = "From: " . $this->sender . "\n";
if ($this->replyTo) {
$this->headers .= "Reply-To: " . $this->replyTo . "\n";
}
if ($this->cc) {
$this->headers .= "Cc: " . $this->cc . "\n";
}
if ($this->bcc) {
$this->headers .= "Bcc: " . $this->bcc . "\n";
}
print "To: " . $this->to . "<br>Subject: " . $this->subject . "<br>Message: " . $this->message . "<br>Headers: " . $this->headers;
return mail($this->to, $this->subject, $this->message, $this->headers);
}
}
Not a lot of changes but enough for us to understand how useful a tool like PHPSA
can be. We went from undocumented, kind of sloppy code, to fully documented and clear code. It is now easier to understand what every property and function in our code does. Running PHPSA now, we will not see any errors or warnings, which means we’ve just added another layer of quality to our code.
变化不多,但足以让我们了解像PHPSA
这样的工具有多有用。 我们从没有记载的,草率的代码变成了完全记载和清晰的代码。 现在,更容易理解代码中每个属性和功能的作用。 现在运行PHPSA,我们将看不到任何错误或警告,这意味着我们刚刚在代码中增加了另一层质量。
结论 (Conclusion)
PHPSA is open source, which means that we can actually follow its development, request functionality and contribute for it, and since it is a focused tool, PHPSA is fast and lightweight. At this point it is still in early alpha stage, which means that it can behave strangely sometimes, mainly giving different results on different operating systems. Also, a lot of functionality is still missing.
PHPSA是开源的,这意味着我们实际上可以关注其开发,请求功能并为此做出贡献,并且由于它是一种有针对性的工具,因此PHPSA既快速又轻巧。 此时,它仍处于Alpha早期阶段,这意味着有时它的行为可能会很奇怪,主要是在不同的操作系统上产生不同的结果。 此外,仍然缺少许多功能。
Static analysis is a valuable tool if we want to enforce quality standards in our code bases. It becomes even more valuable when working in a team, as it forces everybody to use the same standards. Even though it is still a little behind some other tools like Code Sniffer or Mess Detector, PHPSA is a very useful tool that shows a lot of promise. Since one of the better ways to cover a wider range of errors is to combine various analysis tools, consider using PHPSA in your QA stack. Be sure to take it for a spin, and maybe contribute to the project on github where it lists a variety of todos and planned features.
如果我们要在代码库中强制执行质量标准,则静态分析是一种有价值的工具。 在团队中工作时,它变得更加有价值,因为它迫使每个人都使用相同的标准。 尽管PHPSA仍然落后于诸如Code Sniffer或Mess Detector之类的其他工具,但PHPSA是一个非常有用的工具,它显示了很多希望。 由于涵盖多种错误的更好方法之一是组合各种分析工具,因此请考虑在质量检查堆栈中使用PHPSA。 一定要试一试,并可能对github上的项目有所贡献,其中列出了各种待办事项和计划中的功能。
Have you tried PHPSA yet? Let us know how you feel it compares to the rest!
您是否尝试过PHPSA? 让我们知道您与其他人相比感觉如何!
翻译自: https://www.sitepoint.com/static-analysis-with-phpsa-php-smart-analyzer/