javascript 模板
Templating in web applications is crucial for separating your business logic from your presentation. There are two types of templates: server-side templates, which are used through a server-side templating engine during the initial page load, and client-side templates, which are used for working with JavaScript-based applications or Ajax requests.
Web应用程序中的模板对于将业务逻辑与演示文稿分开至关重要。 模板有两种类型:服务器端模板(用于在初始页面加载期间通过服务器端模板引擎使用)和客户端端模板(用于处理基于JavaScript的应用程序或Ajax请求)。
In scenarios where your web application depends heavily on Ajax, it can be difficult to maintain both server-side and client-side templates without duplication. Hence it can be useful to choose a templating engine which provides both client- and server-side support which allows maximum reusability.
在您的Web应用程序严重依赖Ajax的情况下,很难在没有重复的情况下维护服务器端和客户端模板。 因此,选择提供客户端和服务器端支持以实现最大可重用性的模板引擎可能会很有用。
Mustache is quickly becoming one of the more popular templating engines among those available, and provides implementations for different programming languages and platforms to cater to our needs.
在可使用的模板引擎中,MustacheSwift成为最受欢迎的模板引擎之一,并提供了针对不同编程语言和平台的实现方案,以满足我们的需求。
In my article Creating HTML Templates with Mustache.js on JSPro, I showed you how to work with Mustache templates in client-side code. If you don’t have any previous experience working with Mustache, I recommend reading it. In this article I’ll focus on creating server-side Mustache templates and sharing the same set of templates with client-side JavaScript.
在我的文章JSPro上使用Mustache.js创建HTML模板中 ,我向您展示了如何在客户端代码中使用Mustache模板。 如果您以前没有使用过Moustache的经验,建议您阅读。 在本文中,我将重点介绍创建服务器端Mustache模板,以及与客户端JavaScript共享同一组模板。
Mustache.php简介 (Introduction to Mustache.php)
The Mustache implementation in PHP is named Mustache.php, and you can grab a copy of the library using from the official GitHub project page. However, if you are familiar with using Composer, I recommend you to install Mustache using that for better dependency management (and if you’re not familiar with Composer, I recommend you read PHP Dependency Management with Composer).
PHP中的Mustache实现被命名为Mustache.php,您可以使用GitHub项目官方页面上的库副本。 但是,如果您熟悉使用Composer,建议您使用它来安装Mustache,以更好地进行依赖关系管理(如果您不熟悉Composer,则建议您阅读使用ComposerPHP Dependency Management )。
Add the following to your composer.json
file and run either composer install
or composer update
(whichever is appropriate):
将以下内容添加到您的composer.json
文件中,然后运行composer install
或composer update
(以适当者为准):
{
"require": {
"mustache/mustache": "2.0.*"
}
}
Let’s start by looking at a simple example for doing templating with Mustache.php.
让我们先来看一个使用Mustache.php进行模板制作的简单示例。
<?php
require 'vendor/autoload.php';
$tpl = new Mustache_Engine();
echo $tpl->render('Hello, {{planet}}!', array('planet' => 'World'));
First the Composer autoloader is included (alternatively you can use Mustache’s autoloader if you are working with a cloned or downloaded copy of the library). Then the render()
method of the Mustache_Engine
class is called to generate the view by passing it the template data and some replacement values.
首先包括了Composer自动加载器(或者,如果您正在使用库的克隆或下载副本,则可以使用Mustache的自动加载器)。 然后,调用Mustache_Engine
类的render()
方法,以通过将模板数据和一些替换值传递给视图来生成视图。
Ideally you wouldn’t provide the template contents inline like the example does. Templates should be kept in their own files in a dedicated directory. You can configure the path to the template files when creating an instance of the Mustache_Engine
class.
理想情况下,您不会像示例一样内联地提供模板内容。 模板应保存在自己的文件中的专用目录中。 创建Mustache_Engine
类的实例时,可以配置模板文件的路径。
<?php
$mustache = new Mustache_Engine(array(
'loader' => new Mustache_Loader_FilesystemLoader('../templates')
));
$tpl = $mustache->loadTemplate('greeting');
echo $tpl->render(array('planet' => 'World'));
loader in the configuration array defines the path to the template files (more details on the configuration parameters can be found in the Mustache.php Wiki). In this scenario, template files are located inside the templates directory. The loadTemplate()
method loads the file templates/greeting.mustache
.
配置数组中的loader定义了模板文件的路径(有关配置参数的更多详细信息,请参见Mustache.php Wiki )。 在这种情况下,模板文件位于模板目录内。 loadTemplate()
方法加载文件templates/greeting.mustache
。
Having briefly covered the necessary theory for working with Mustache, we can now look at how to share the templates.
简要介绍了使用Moustache的必要理论之后,我们现在来看一下如何共享模板。
在PHP和JavaScript之间共享模板 (Sharing Templates between PHP and JavaScript)
Our main goal is to share the templates between PHP (server-side) and JavaScript (client-side). For the basis of our example, let’s assume we have an e-commerce site with two product categories: Books and Movies. When the user first visits, all of the products will be displayed in a single list as shown below.
我们的主要目标是在PHP(服务器端)和JavaScript(客户端)之间共享模板。 以我们的示例为基础,假设我们有一个电子商务网站,其中包含两个产品类别:书籍和电影。 用户首次访问时,所有产品将显示在一个列表中,如下所示。
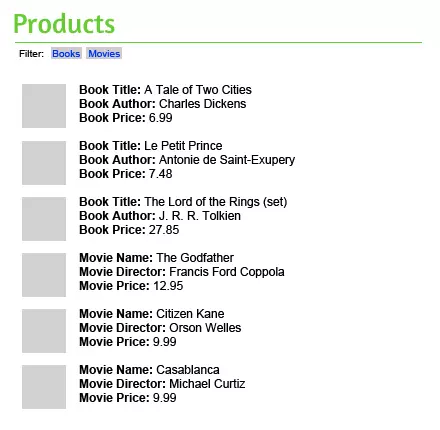
You can see that the details of each product type are different; hence we need separate templates for the two categories.
您会看到每种产品类型的详细信息都不同; 因此,我们需要针对这两种类别的单独模板。
Create two templates, one called books.mustache
and another movies.mustache
. This is how the books.mustache
file will look like:
创建两个模板,一个模板名为books.mustache
,另一个movies.mustache
。 这是books.mustache
文件的样子:
{{#products}}
{{#book}}
<div>
<p>Book Title: {{title}}</p>
<p>Book Author: {{author}}</p>
<p>Book Price: {{price}}</p>
</div>
{{/book}}
{{/products}}
The above is a simple template with a section for looping purposes and some template-specific variables. {{#products}}
is used to loop through all of the elements inside the products section of the array we will provide. {{#book}}
is used to check if the item is actually a book. If the book key doesn’t exist, then nothing will be displayed to the user.
上面是一个简单的模板,其中包含用于循环目的的部分和一些模板特定的变量。 {{#products}}
用于遍历我们将提供的数组的products部分内的所有元素。 {{#book}}
用于检查项目是否实际上是一本书。 如果书键不存在,则不会向用户显示任何内容。
On the initial page load, all of the products need to be retrieved and displayed to the user, so we use PHP to generate the initial display using both the template and the data set.
在初始页面加载时,需要检索所有产品并将其显示给用户,因此我们使用PHP使用模板和数据集生成初始显示。
<?php
$books = array();
$result = $db->query('SELECT title, author, price FROM books');
while ($row = $result->fetch(PDO::FETCH_ASSOC)) {
$row['book'] = true;
$books[] = $row;
}
$result->closeCursor();
$movies = array();
$result = $db->query('SELECT name, price, cast FROM movies');
while ($row = $result->fetch(PDO::FETCH_ASSOC)) {
$row['movie'] = true;
$movies[] = $row;
}
$booksTmpl = $this->mustache->loadTemplate('books');
$moviesTmpl = $this->mustache->loadTemplate('movies');
$data = array(
'products' => array_merge($books, $movies)
);
$html = $booksTmpl->render($data);
$html .= $moviesTmpl->render($data);
echo $html;
We use two database queries to pull the book and movie data from the database and save it into an associative array for passing into the templates . I’ve used basic PDO methods for pulling the data; you can use your own database abstraction layer or ORM if you like.
我们使用两个数据库查询从数据库中提取书籍和电影数据,并将其保存到关联数组中以传递到模板中。 我使用了基本的PDO方法提取数据。 您可以根据需要使用自己的数据库抽象层或ORM。
The templates for books and movies are loaded separately into two variables using the loadTemplate()
method, and I pass the products array to each template’s render()
method. It applies the template to the product data and returns the HTML output.
使用loadTemplate()
方法将书籍和电影的模板分别加载到两个变量中,然后将product数组传递给每个模板的render()
方法。 它将模板应用于产品数据并返回HTML输出。
To filter the results based on product type, we could get all the filtered books (or movies) as rendered HTML from the server, but all of the markup would incur additional overhead for each request. We really don’t want to have to send the same markup over and over again. This is where the client-side templates come into play – we can keep the necessary templates loaded into memory on the client and only load the data from the server using Ajax.
为了根据产品类型过滤结果,我们可以从服务器获取所有过滤后的书籍(或电影)作为HTML呈现,但是所有标记都会为每个请求带来额外的开销。 我们真的不想一次又一次地发送相同的标记。 这是客户端模板发挥作用的地方–我们可以将必要的模板加载到客户端的内存中,而仅使用Ajax从服务器加载数据。
To make the templates available to the client, they can be injected in the initial page request in <script>
tags for future use by JavaScript. The code inside these tags will not be displayed, nor will they be executed by JavaScript if we set the type to text/mustache
.
为了使模板对客户端可用,可以将它们插入<script>
标记中的初始页面请求中,以供JavaScript将来使用。 如果将类型设置为text/mustache
,则这些标记内的代码将不会显示,也不会由JavaScript执行。
<script id="booksTmpl" type="text/mustache">
<?php
echo file_get_contents(dirname(__FILE__) . '/templates/books.mustache');
?>
</script>
<script id="moviesTmpl" type="text/mustache">
<?php
echo file_get_contents(dirname(__FILE__) . '/templates/movies.mustache');?>
</script>
In order to use these templates in JavaScript, we just need to include the Mustache.js library in the document. You can grab a copy of mustache.js
file from its GitHub page and include it in your HTML as following:
为了在JavaScript中使用这些模板,我们只需要在文档中包括Mustache.js库即可。 您可以从其GitHub页面上获取mustache.js
文件的副本,并将其包含在HTML中,如下所示:
<script type="text/javascript" src="mustache.js"></script>
Once the user selects the filter option, we can get the data using an Ajax request. But unlike the initial page load, we won’t apply the templates to the data before sending them to the client since we have the templates available client-side already.
一旦用户选择了过滤器选项,我们就可以使用Ajax请求获取数据。 但是与初始页面加载不同,由于模板已经在客户端可用,因此在将模板发送到客户端之前,我们不会将模板应用于数据。
<script>
$("#booksFilter").click(function () {
$.ajax({
type: "GET",
url: "ajax.php?type=books"
}).done(function (msg) {
var template = $("#booksTmpl").html();
var output = Mustache.render(template, msg);
$("#products").html(output);
});
});
</script>
The book list is retrieved from the database and returned in JSON format to the client, and then we fetch the book template from within its script tag using $("#booksTmpl").html()
and pass both the template and data to the client-side Mustache rendering function. The generated output is then applied back to a products section on the page.
从数据库中检索出图书清单,并以JSON格式返回给客户端,然后我们使用$("#booksTmpl").html()
从其脚本标签中获取图书模板,并将模板和数据都传递给客户端小胡子渲染功能。 然后将生成的输出应用回页面上的产品部分。
摘要 (Summary)
Working with separate template engines both client-side and server-side can be a difficult task and can lead to template duplication. Luckily, various implementations of Mustache allow us to share the same templates between both sides. This increases the maintainability of your code. If you’d like to play around with some example code for this article, you can find it on the PHPMaster GitHub account.
在客户端和服务器端使用单独的模板引擎可能是一项艰巨的任务,并且可能导致模板重复。 幸运的是,Mustache的各种实现允许我们在双方之间共享相同的模板。 这样可以提高代码的可维护性。 如果您想使用本文的一些示例代码,可以在PHPMaster GitHub帐户上找到它。
Feel free to share your suggestions and previous experiences with Mustache templates in the comments below.
欢迎在下面的评论中分享与Mustache模板有关的建议和以前的经验。
Image via Fotolia
图片来自Fotolia
翻译自: https://www.sitepoint.com/sharing-templates-between-php-and-javascript/
javascript 模板