web soap服务
As application developers, the ability to develop software and services for a wide range of platforms is a necessary skill, but not everyone uses the same language or platform and writing code to support them all is not feasible. If only there was a standard that allowed us to write code once and allow others to interact with it from their own software with ease. Well luckily there is… and it’s name is SOAP. (SOAP used to be an acronym which stood for Simple Object Access Protocol, but as of version 1.2 the protocol goes simply by the name SOAP.)
作为应用程序开发人员,具有为广泛的平台开发软件和服务的能力是一项必需的技能,但是并不是每个人都使用相同的语言或平台,并且编写代码来支持它们都是不可行的。 如果有一个标准允许我们编写一次代码,并允许其他人轻松地从自己的软件与之交互。 好吧,幸运的是……它的名字叫SOAP。 (SOAP曾经是“简单对象访问协议”的缩写,但是从1.2版开始,该协议仅以SOAP名称命名。)
SOAP allows you to build interoperable software and allows others to take advantage of your software over a network. It defines rules for sending and receiving Remote Procedure Calls (RPC) such as the structure of the request and responses. Therefore, SOAP is not tied to any specific operating system or programming language. As that matters is someone can formulate and parse a SOAP message in their chosen language
SOAP允许您构建可互操作的软件,并允许其他人通过网络利用您的软件。 它定义了发送和接收远程过程调用(RPC)的规则,例如请求和响应的结构。 因此,SOAP不受任何特定的操作系统或编程语言的束缚。 至关重要的是,有人可以用他们选择的语言来制定和解析SOAP消息。
In this first of a two part series on web services I’ll talk about the SOAP specification and what is involved in creating SOAP messages. I’ll also demonstrate how to create a SOAP server and client using the excellent NuSOAP library to illustrate the flow of SOAP. In the second part I’ll talk about the importance of WSDL files, how you can easily generate them with NuSOAP as well, and how a client may use a WSDL file to better understand your web service.
在关于Web服务的两部分系列的第一个系列中,我将讨论SOAP规范以及创建SOAP消息所涉及的内容。 我还将演示如何使用出色的NuSOAP库创建SOAP服务器和客户端,以说明SOAP的流程。 在第二部分中,我将讨论WSDL文件的重要性,如何通过NuSOAP轻松生成它们,以及客户端如何使用WSDL文件来更好地理解您的Web服务。
SOAP消息的结构 (The Structure of a SOAP Message)
SOAP is based on XML so it is considered human read, but there is a specific schema that must be adhered to. Let’s first break down a SOAP message, stripping out all of its data, and just look at the specific elements that make up a SOAP message.
SOAP基于XML,因此被认为是人类可读的,但是必须遵守特定的架构。 首先,让我们分解SOAP消息,剥离所有数据,然后查看构成SOAP消息的特定元素。
<?xml version="1.0"?>
<soap:Envelope
xmlns:soap="https://www.w3.org/2001/12/soap-envelope"
soap:encodingStyle="https://www.w3.org/2001/12/soap-encoding">
<soap:Header>
...
</soap:Header>
<soap:Body>
...
<soap:Fault>
...
</soap:Fault>
</soap:Body>
</soap:Envelope>
This might look like just an ordinary XML file, but what makes it a SOAP message is the root element Envelope with the namespace soap
as https://www.w3.org/2001/12/soap-envelope
. The soap:encodingStyle
attribute determines the data types used in the file, but SOAP itself does not have a default encoding.
这可能看起来像只是一个普通的XML文件,但使它成为SOAP消息的是根元素Envelope,其名称空间为soap
如https://www.w3.org/2001/12/soap-envelope
。 soap:encodingStyle
属性确定文件中使用的数据类型,但是SOAP本身没有默认编码。
soap:Envelope
is mandatory, but the next element, soap:Header
, is optional and usually contains information relevant to authentication and session handling. The SOAP protocol doesn’t offer any built-in authentication, but allows developers to include it in this header tag.
soap:Envelope
是必需的,但下一个元素soap:Header
是可选的,通常包含与身份验证和会话处理有关的信息。 SOAP协议不提供任何内置身份验证,但允许开发人员将其包含在此标头标记中。
Next there’s the required soap:Body
element which contains the actual RPC message, including method names and, in the case of a response, the return values of the method. The soap:Fault
element is optional; if present, it holds any error messages or status information for the SOAP message and must be a child element of soap:Body
.
接下来是必需的soap:Body
元素,其中包含实际的RPC消息,包括方法名称以及(在响应的情况下)方法的返回值。 soap:Fault
元素是可选的; 如果存在,则它包含SOAP消息的任何错误消息或状态信息,并且必须是soap:Body
的子元素。
Now that you understand the basics of what makes up a SOAP message, let’s look at what SOAP request and response messages might look like. Let’s start with a request.
现在您已经了解了构成SOAP消息的基础知识,让我们看一下SOAP请求和响应消息的外观。 让我们从一个请求开始。
<?xml version="1.0"?>
<soap:Envelope
xmlns:soap="https://www.w3.org/2001/12/soap-envelope"
soap:encodingStyle="https://www.w3.org/2001/12/soap-encoding">
<soap:Body xmlns:m="http://www.yourwebroot.com/stock">
<m:GetStockPrice>
<m:StockName>IBM</m:StockName>
</m:GetStockPrice>
</soap:Body>
</soap:Envelope>
Above is an example SOAP request message to obtain the stock price of a particular company. Inside soap:Body
you’ll notice the GetStockPrice
element which is specific to the application. It’s not a SOAP element, and it takes its name from the function on the server that will be called for this request. StockName
is also specific to the application and is an argument for the function.
上面是获取特定公司的股价的示例SOAP请求消息。 在soap:Body
您会注意到特定于该应用程序的GetStockPrice
元素。 它不是SOAP元素,它的名称来自将为此请求调用的服务器上的函数。 StockName
也特定于应用程序,并且是该函数的参数。
The response message is similar to the request:
响应消息类似于请求:
<?xml version="1.0"?>
<soap:Envelope
xmlns:soap="https://www.w3.org/2001/12/soap-envelope"
soap:encodingStyle="https://www.w3.org/2001/12/soap-encoding">
<soap:Body xmlns:m="http://www.yourwebroot.com/stock">
<m:GetStockPriceResponse>
<m:Price>183.08</m:Price>
</m:GetStockPriceResponse>
</soap:Body>
</soap:Envelope>
Inside the soap:Body element there is a GetStockPriceResponse
element with a Price
child that contains the return data. As you would guess, both GetStockPriceResponse
and Price
are specific to this application.
soap:Body元素内部有一个GetStockPriceResponse
元素,其中有一个Price
子元素,其中包含返回数据。 您可能会猜到, GetStockPriceResponse
和Price
都特定于此应用程序。
Now that you’ve seen an example request and response and understand the structure of a SOAP message, let’s install NuSOAP and build a SOAP client and server to demonstrate generating such messages.
现在,您已经看到了示例请求和响应并了解了SOAP消息的结构,让我们安装NuSOAP并构建SOAP客户端和服务器以演示如何生成此类消息。
构建一个SOAP服务器 (Building a SOAP Server)
It couldn’t be easier to get NuSOAP up and running on your server; just visit sourceforge.net/projects/nusoap, download and unzip the package in your web root direoctry, and you’re done. To use the library just include the nusoap.php
file in your code.
在服务器上启动和运行NuSOAP并不容易。 只需访问sourceforge.net/projects/nusoap ,下载该软件包并将其解压缩到您的Web根目录中,即可完成。 要使用该库,只需在nusoap.php
中包含nusoap.php
文件。
For the server, let’s say we’ve been given the task of building a service to provide a listing of products given a product category. The server should read in the category from a request, look up any products that match the category, and return the list to the user in a CSV format.
对于服务器,假设我们已经承担了构建服务的任务,以提供给定产品类别的产品列表。 服务器应从请求中读取类别,查找与该类别匹配的所有产品,并将列表以CSV格式返回给用户。
Create a file in your web root named productlist.php
with the following code:
使用以下代码在您的Web根目录中创建一个名为productlist.php
的文件:
<?php
require_once "nusoap.php";
function getProd($category) {
if ($category == "books") {
return join(",", array(
"The WordPress Anthology",
"PHP Master: Write Cutting Edge Code",
"Build Your Own Website the Right Way"));
}
else {
return "No products listed under that category";
}
}
$server = new soap_server();
$server->register("getProd");
$server->service($HTTP_RAW_POST_DATA);
First, the nusoap.php file is included to take advantage of the NuSOAP library. Then, the getProd()
function is defined. Afterward, a new instance of the soap_server class is instantiated, the getProd()
function is registered with its register()
method.
首先,包含nusoap.php文件以利用NuSOAP库。 然后,定义了getProd()
函数。 然后,实例化soap_server类的新实例,并使用其register()
方法注册getProd()
函数。
This is really all that’s needed to create your own SOAP server – simple, isn’t it? In a real-world scenario you would probably look up the list of books from a database, but since I want to focus on SOAP, I’ve mocked getProd()
to return a hard-coded list of titles.
这实际上是创建您自己的SOAP服务器所需的全部–是,不是吗? 在现实世界中,您可能会从数据库中查找书籍列表,但是由于我想专注于SOAP,因此我getProd()
以返回硬编码的书名列表。
If you want to include more functionality in the sever you only need to define the additional functions (or even methods in classes) and register each one as you did above.
如果要在服务器中包含更多功能,则只需定义其他功能(甚至是类中的方法)并像上面一样注册每个功能。
Now that we have a working server, let’s build a client to take advantage of it.
现在我们有了一个可以正常工作的服务器,让我们构建一个客户端来利用它。
构建一个SOAP客户端 (Building a SOAP Client)
Create a file named productlistclient.php
and use the code below:
创建一个名为productlistclient.php
的文件,并使用以下代码:
<?php
require_once "nusoap.php";
$client = new nusoap_client("http://localhost/nusoap/productlist.php");
$error = $client->getError();
if ($error) {
echo "<h2>Constructor error</h2><pre>" . $error . "</pre>";
}
$result = $client->call("getProd", array("category" => "books"));
if ($client->fault) {
echo "<h2>Fault</h2><pre>";
print_r($result);
echo "</pre>";
}
else {
$error = $client->getError();
if ($error) {
echo "<h2>Error</h2><pre>" . $error . "</pre>";
}
else {
echo "<h2>Books</h2><pre>";
echo $result;
echo "</pre>";
}
}
Once again we include nusoap.php
with require_once
and then create a new instance of nusoap_client
. The constructor takes the location of the newly created SOAP server to connect to. The getError()
method checks to see if the client was created correctly and the code displays an error message if it wasn’t.
我们再次将nusoap.php
包含在require_once
,然后创建nusoap_client
的新实例。 构造函数获取新创建的SOAP服务器要连接的位置。 getError()
方法检查是否正确创建了客户端,如果不是,则代码显示错误消息。
The call()
method generates and sends the SOAP request to call the method or function defined by the first argument. The second argument to call()
is an associate array of arguments for the RPC. The fault property and getError()
method are used to check for and display any errors. If no there are no errors, then the result of the function is outputted.
call()
方法生成并发送SOAP请求,以调用第一个参数定义的方法或函数。 call()
的第二个参数是RPC参数的关联数组。 fault属性和getError()
方法用于检查和显示任何错误。 如果没有错误,则输出函数的结果。
Now with both files in your web root directory, launch the client script (in my case http://localhost/nusoap/productlistclient.php
) in your browser. You should see the following:
现在,两个文件都位于您的Web根目录中,在浏览器中启动客户端脚本(在我的情况下为http://localhost/nusoap/productlistclient.php
)。 您应该看到以下内容:
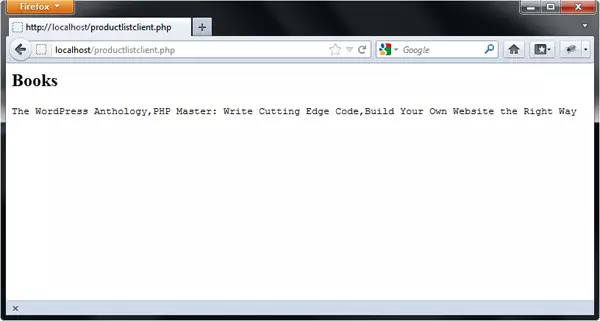
If you want to inspect the SOAP request and response messages for debug purposes, or if you just to pick them apart for fun, add these lines to the bottom of productlistclient.php
:
如果要出于调试目的检查SOAP请求和响应消息,或者只是为了娱乐而将它们分开,请将这些行添加到productlistclient.php
的底部:
echo "<h2>Request</h2>";
echo "<pre>" . htmlspecialchars($client->request, ENT_QUOTES) . "</pre>";
echo "<h2>Response</h2>";
echo "<pre>" . htmlspecialchars($client->response, ENT_QUOTES) . "</pre>";
The HTTP headers and XML content will now be appended to the output.
HTTP标头和XML内容现在将添加到输出中。
摘要 (Summary)
In this first part of the series you learned that SOAP provides the ability to build interoperable software supporting a wide range of platforms and programming languages. You also learned about the different parts of a SOAP message and built your own SOAP server and client to demonstrate how SOAP works.
在本系列的第一部分中,您了解了SOAP提供了构建可互操作的软件的能力,该软件支持广泛的平台和编程语言。 您还了解了SOAP消息的不同部分,并构建了自己的SOAP服务器和客户端来演示SOAP的工作方式。
In the next part I’ll take you deeper into the SOAP rabbit hole and explain what a WSDL file is and how it can help you with the documentation and structure of your web service.
在下一部分中,我将带您更深入地了解SOAP兔子漏洞,并解释什么是WSDL文件以及它如何帮助您提供Web服务的文档和结构。
Comments on this article are closed. Have a question about PHP? Why not ask it on our forums?
本文的评论已关闭。 对PHP有疑问吗? 为什么不在我们的论坛上提问呢?
Image via Lilyana Vynogradova / Shutterstock
图片来自Lilyana Vynogradova / Shutterstock
翻译自: https://www.sitepoint.com/web-services-with-php-and-soap-1/
web soap服务