laravel5.6 邮件
This article was peer reviewed by Viraj Khatavkar. Thanks to all of SitePoint’s peer reviewers for making SitePoint content the best it can be!
本文由Viraj Khatavkar进行了同行评审。 感谢所有SitePoint的同行评审人员使SitePoint内容达到最佳状态!
One of the many goodies Laravel offers is mailing. You can easily configure and send emails through multiple popular services, and it even includes a logging helper for development.
Laravel提供的许多好处之一就是邮寄。 您可以轻松地通过多种流行的服务配置和发送电子邮件,甚至还包括用于开发的日志记录帮助程序。
Mail::send('emails.welcome', ['user' => $user], function ($m) use ($user) {
$m->to($user->email, $user->name)->subject('Welcome to the website');
});
This will send an email to a new registered user on the website using the emails.welcome
view. It got even simpler with Laravel 5.3 using mailables (but the old syntax is still valid).
这将使用emails.welcome
视图将电子邮件发送给网站上的新注册用户。 它采用了更简单的使用Laravel 5.3 mailables (但旧的语法仍然有效)。
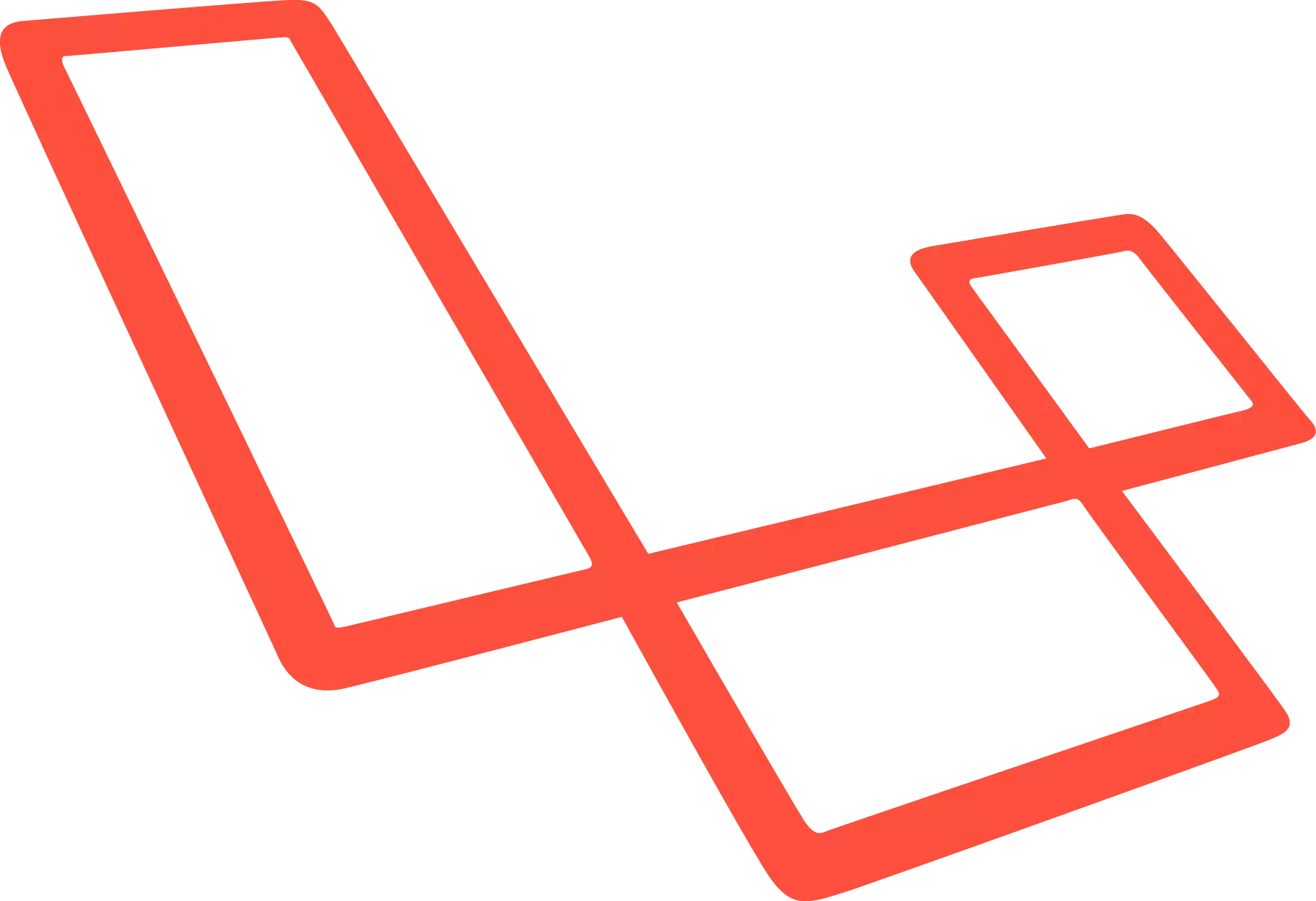
Here’s an example:
这是一个例子:
# Generate a new mailable class
php artisan make:mail WelcomeMail
// app/Mail/WelcomeMail.php
class WelcomeUser extends Mailable
{
use Queueable, SerializesModels;
public $user;
public function __construct(User $user)
{
$this->user = $user;
}
public function build()
{
return $this->view('emails.welcome');
}
}
// routes/web.php
Route::get('/', function () {
$user = User::find(2);
\Mail::to($user->email)->send(new WelcomeUser($user));
return "done";
});
Laravel also provides a good starting point for sending mails during the development phase using the log
driver, and in production using smtp
, sparkpost
, mailgun
, etc. This seems fine in most cases, but it can’t cover all the available services! In this tutorial, we’re going to learn how to extend the existing mail driver system to add our own.
Laravel还为在开发阶段使用log
驱动程序以及在生产中使用smtp
, sparkpost
, mailgun
等发送邮件提供了一个很好的起点。在大多数情况下,这看起来不错,但它无法涵盖所有可用的服务! 在本教程中,我们将学习如何扩展现有的邮件驱动程序系统以添加我们自己的邮件驱动程序。
To keep our example simple and straightforward, we’re going to log our emails to a DB table.
为了使示例简单明了,我们将把电子邮件记录到数据库表中。
创建服务提供商 (Creating a Service Provider)
The preferred way to accomplish this is by creating a service provider to interact with our application at boot time and register our services. Let’s start by generating a new service provider using the artisan command line helper.
实现此目的的首选方法是创建一个服务提供程序,以便在启动时与我们的应用程序进行交互并注册我们的服务。 让我们从使用artisan命令行助手生成一个新的服务提供者开始。
php artisan make:provider DBMailProvider
This will create a new class inside our app/Providers
folder. If you’re familiar with Laravel service providers, you’ll know that we extend the ServiceProvider
class and define the boot
and register
methods. You can read more about providers in the documentation.
这将在我们的app/Providers
文件夹中创建一个新类。 如果您熟悉Laravel服务提供者,就会知道我们扩展了ServiceProvider
类并定义了boot
和register
方法。 您可以在文档中阅读有关提供程序的更多信息。
使用邮件提供者 (Using the Mail Provider)
Instead of using the parent service provider class, we can take a shortcut and extend the existing Illuminate\Mail\MailServiceProvider
one. This means that the register
method is already implemented.
可以使用一种快捷方式并扩展现有的Illuminate\Mail\MailServiceProvider
,而不是使用父服务提供程序类。 这意味着该register
方法已经实现。
// vendor/Illuminate/Mail/MailServiceProvider.php
public function register()
{
$this->registerSwiftMailer();
// ...
}
The registerSwiftMailer
method will return the appropriate transport driver based on the mail.driver
config value. What we can do here is perform the check before calling the registerSwiftMailer
parent method and return our own transport manager.
registerSwiftMailer
方法将基于mail.driver
配置值返回适当的传输驱动程序。 我们在这里可以做的是在调用registerSwiftMailer
父方法并返回我们自己的传输管理器之前执行检查。
// app/Providers/DBMailProvider.php
function registerSwiftMailer()
{
if ($this->app['config']['mail.driver'] == 'db') {
$this->registerDBSwiftMailer();
} else {
parent::registerSwiftMailer();
}
}
使用运输管理器 (Using the Transport Manager)
Laravel resolves the swift.mailer
instance from the IOC, which should return a Swift_Mailer
instance of SwiftMailer. We need to bind our Swift mailer instance to the container.
Laravel从IOC解析swift.mailer
实例,该实例应返回SwiftMailer的Swift_Mailer
实例。 我们需要将Swift邮件程序实例绑定到容器。
private function registerDBSwiftMailer()
{
$this->app['swift.mailer'] = $this->app->share(function ($app) {
return new \Swift_Mailer(new DBTransport());
});
}
You can think of the transport object as the actual driver. If you check the Illuminate\Mail\Transport
namespace, you’ll find the different transport classes for every driver (like: LogTransport
, SparkPostTransport
, etc.).
您可以将运输对象视为实际驱动程序。 如果检查Illuminate\Mail\Transport
命名空间,则会为每个驱动程序找到不同的传输类(例如: LogTransport
, SparkPostTransport
等)。
The Swift_Mailer
class requires a Swift_Transport
instance, which we can satisfy by extending the Illuminate\Mail\Transport\Transport
class. It should look something like this.
Swift_Mailer
类需要一个Swift_Transport
实例,我们可以通过扩展Illuminate\Mail\Transport\Transport
类来满足该实例。 它应该看起来像这样。
namespace App\Mail\Transport;
use Illuminate\Mail\Transport\Transport;
use Illuminate\Support\Facades\Log;
use Swift_Mime_Message;
use App\Emails;
class DBTransport extends Transport
{
public function __construct()
{
}
public function send(Swift_Mime_Message $message, &$failedRecipients = null)
{
Emails::create([
'body' => $message->getBody(),
'to' => implode(',', array_keys($message->getTo())),
'subject' => $message->getSubject()
]);
}
}
The only method we should implement here is the send
one. It’s responsible for the mail-sending logic, and in this case, it should log our emails to the database. As for our constructor, we can leave it empty for the moment because we don’t need any external dependencies.
我们应该在这里实现的唯一方法是send
。 它负责邮件发送逻辑,在这种情况下,它应该将我们的电子邮件记录到数据库中。 至于我们的构造函数,我们暂时可以将其保留为空,因为我们不需要任何外部依赖关系。
The $message->getTo()
method always returns an associative array of recipients’ emails and names. We get the list of emails using the array_keys
function and then implode them to get a string.
$message->getTo()
方法始终返回收件人电子邮件和姓名的关联数组。 我们使用array_keys
函数获取电子邮件列表,然后将其内爆以获得字符串。
将电子邮件记录到数据库 (Log Email to DB)
The next step is to create the necessary migration for our database table.
下一步是为我们的数据库表创建必要的迁移。
php artisan make:migration create_emails_table --create="emails"
class CreateEmailsTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('emails', function (Blueprint $table) {
$table->increments('id');
$table->text('body');
$table->string('to');
$table->string('subject');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('emails');
}
}
Our migration only contains the email body, subject and recipient email, but you can add as many details as you want. Check the Swift_Mime_Message
class definition to see the list of available fields.
我们的迁移仅包含电子邮件正文,主题和收件人电子邮件,但是您可以根据需要添加任意数量的详细信息。 检查Swift_Mime_Message
类定义以查看可用字段的列表。
Now, we need to create a new model to interact with our table, and add the necessary fields to the fillable array.
现在,我们需要创建一个新模型来与我们的表进行交互,并将必要的字段添加到可填充数组中。
php artisan make:model Emails
class Emails extends Model
{
protected $fillable = [
'body',
'to',
'subject'
];
}
发送邮件 (Sending Emails)
Ok, it’s time to test what we’ve got so far. We start by adding our provider to the list of providers inside the config/app.php
file.
好的,现在该测试我们到目前为止所获得的。 我们首先将提供程序添加到config/app.php
文件内的提供程序列表中。
return [
// ...
'providers' => [
// ...
App\Providers\DBMailProvider::class,
// ...
],
// ...
];
Then we register our mail driver to db
inside the config/mail.php
file.
然后,我们将邮件驱动程序注册到config/mail.php
文件中的db
。
return [
'driver' => 'db',
// ...
];
The only remaining part is to send a test email and check if it gets logged to the database. I’m going to send an email when the home URL is hit. Here’s the code.
剩下的唯一部分是发送测试电子邮件,并检查是否将其记录到数据库中。 当我点击家庭网址时,我将发送一封电子邮件。 这是代码。
// routes/web.php
Route::get('/', function () {
$user = User::find(2);
Mail::send('emails.welcome', ['user' => $user], function ($m) use ($user) {
$m->to($user->email, $user->name)->subject('Welcome to the website');
});
return "done";
});
After hitting the home route, we can run the php artisan tinker
command to check the emails table records.
到达本地路线后,我们可以运行php artisan tinker
命令来检查电子邮件表记录。
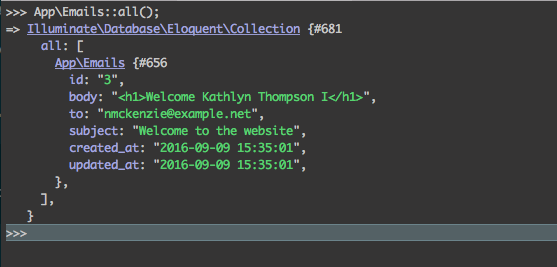
结论 (Conclusion)
In this article, we saw how to extend the mail driver system to intercept emails for debugging purposes. One of the things I admire in Laravel is its unparalleled extensibility: you can alter or extend almost any functionality from router and IOC, to Mail and beyond.
在本文中,我们看到了如何扩展邮件驱动程序系统以拦截电子邮件以进行调试。 我在Laravel中欣赏的一件事是它无与伦比的可扩展性:您可以更改或扩展几乎所有功能,从路由器和IOC,到邮件,甚至更多。
If you have any questions or comments, be sure to post them below and I’ll do my best to answer them!
如果您有任何疑问或意见,请务必将其张贴在下面,我会尽力回答!
翻译自: https://www.sitepoint.com/mail-logging-in-laravel-5-3-extending-the-mail-driver/
laravel5.6 邮件