mvc模式 php
The Model-View-Control (MVC) pattern, originally formulated in the late 1970s, is a software architecture pattern built on the basis of keeping the presentation of data separate from the methods that interact with the data. In theory, a well-developed MVC system should allow a front-end developer and a back-end developer to work on the same system without interfering, sharing, or editing files either party is working on.
最初在1970年代后期制定的Model-View-Control(MVC)模式是一种软件体系结构模式,其建立在将数据表示与与数据交互的方法分开的基础上。 从理论上讲,一个完善的MVC系统应允许前端开发人员和后端开发人员在同一系统上工作,而不会干扰,共享或编辑任何一方正在工作的文件。
Even though MVC was originally designed for personal computing, it has been adapted and is widely used by web developers due to its emphasis on separation of concerns, and thus indirectly, reusable code. The pattern encourages the development of modular systems, allowing developers to quickly update, add, or even remove functionality.
尽管MVC最初是为个人计算而设计的,但由于它强调关注点的分离以及间接地可重用的代码,因此已被改编并被Web开发人员广泛使用。 这种模式鼓励模块化系统的开发,从而使开发人员能够快速更新,添加甚至删除功能。
In this article, I will go the basic principles of MVC, a run through the definition of the pattern and a quick example of MVC in PHP. This is definitely a read for anyone who has never coding with MVC before or those wanting to brush up on previous MVC development skills.
在本文中,我将介绍MVC的基本原理,对模式的定义以及PHP中MVC的快速示例。 对于任何以前从未使用过MVC编码的人或想要重温以前的MVC开发技能的人来说,这绝对是一本读物。
了解MVC (Understanding MVC)
The pattern’s title is a collation of its three core parts: Model, View, and Controller. A visual representation of a complete and correct MVC pattern looks like the following diagram:
模式的标题是其三个核心部分的排序规则:模型,视图和控制器。 完整正确的MVC模式的直观外观如下图所示 :
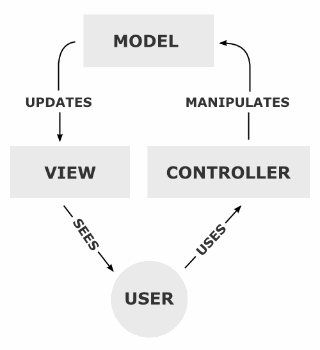
The image shows the single flow layout of data, how it’s passed between each component, and finally how the relationship between each component works.
该图显示了数据的单一流布局,如何在每个组件之间传递数据以及最后如何在每个组件之间建立关系。
模型 (Model)
The Model is the name given to the permanent storage of the data used in the overall design. It must allow access for the data to be viewed, or collected and written to, and is the bridge between the View component and the Controller component in the overall pattern.
模型是永久性存储在整个设计中使用的数据的名称。 它必须允许访问数据以进行查看,收集或写入,并且是整个模式中View组件和Controller组件之间的桥梁。
One important aspect of the Model is that it’s technically “blind” – by this I mean the model has no connection or knowledge of what happens to the data when it is passed to the View or Controller components. It neither calls nor seeks a response from the other parts; its sole purpose is to process data into its permanent storage or seek and prepare data to be passed along to the other parts.
该模型的一个重要方面是它在技术上是“盲目的”的–我的意思是该模型与数据传递给View或Controller组件时没有任何联系或知识。 它既不要求也不寻求其他部分的回应。 它的唯一目的是将数据处理到其永久存储器中,或者寻找并准备要传递给其他部分的数据。
The Model, however, cannot simply be summed up as a database, or a gateway to another system which handles the data process. The Model must act as a gatekeeper to the data itself, asking no questions but accepting all requests which comes its way. Often the most complex part of the MVC system, the Model component is also the pinnacle of the whole system since without it there isn’t a connection between the Controller and the View.
但是,不能将模型简单地概括为一个数据库,或作为处理数据过程的另一个系统的网关。 该模型必须充当数据本身的看门人,不问任何问题,而是接受随之而来的所有请求。 Model组件通常是MVC系统中最复杂的部分,也是整个系统的巅峰之作,因为没有它,Controller和View之间就没有联系。
视图 (View)
The View is where data, requested from the Model, is viewed and its final output is determined. Traditionally in web apps built using MVC, the View is the part of the system where the HTML is generated and displayed. The View also ignites reactions from the user, who then goes on to interact with the Controller. The basic example of this is a button generated by a View, which a user clicks and triggers an action in the Controller.
视图是查看从模型请求的数据并确定其最终输出的位置。 传统上,在使用MVC构建的Web应用程序中,视图是生成和显示HTML的系统的一部分。 视图还引发用户的React,然后用户继续与控制器进行交互。 一个基本的例子是一个由View生成的按钮,用户单击该按钮并在Controller中触发一个动作。
There are some misconceptions held about View components, particularly by web developers using the MVC pattern to build their application. For example, many mistake the View as having no connection whatsoever to the Model and that all of the data displayed by the View is passed from the Controller. In reality, this flow disregards the theory behind the MVC pattern completely. Fabio Cevasco’s article The CakePHP Framework: Your First Bite demonstrates this confused approach to MVC in the CakePHP framework, an example of the many non-traditional MVC PHP frameworks available:
对于View组件存在一些误解,尤其是使用MVC模式构建其应用程序的Web开发人员。 例如,许多人错误地认为View与模型没有任何关系,并且View显示的所有数据都是从Controller传递的。 实际上,此流程完全无视MVC模式背后的理论。 Fabio Cevasco的文章CakePHP框架:您的第一口证明了CakePHP框架中这种对MVC的混淆方法,这是可用的许多非传统MVC PHP框架的一个示例:
“It is important to note that in order to correctly apply the MVC architecture, there must be no interaction between models and views: all the logic is handled by controllers“
“重要的是要注意,为了正确地应用MVC架构,模型和视图之间必须没有交互:所有逻辑都由控制器处理”
Furthermore, the description of Views as a template file is inaccurate. However, as Tom Butler points out, this is not one person’s fault but a multitude of errors by a multitude of developers which result in developers learning MVC incorrectly. They then go on to educate others incorrectly. The View is really much more than just a template, however modern MVC inspired frameworks have bastardised the view almost to the point that no one really cares whether or not a framework actually adheres to the correct MVC pattern or not.
此外,将Views作为模板文件的描述是不准确的。 但是,正如汤姆·巴特勒(Tom Butler)指出的那样,这不是一个人的错,而是众多开发人员的众多错误,这些错误导致开发人员错误地学习MVC。 然后他们继续错误地教育他人。 该视图实际上不仅仅是一个模板,但是受现代MVC启发的框架几乎破坏了这种观点,以至于没有人真正在乎框架是否确实遵循正确的MVC模式。
It’s also important to remember that the View part is never given data by the Controller. As I mentioned when discussing the Model, there is no direct relationship between the View and the Controller without the Model in between them.
同样重要的是要记住,控制器从未向视图部分提供数据。 正如我在讨论模型时提到的,视图与控制器之间没有模型之间就没有直接关系。
控制者 (Controller)
The final component of the triad is the Controller. Its job is to handle data that the user inputs or submits, and update the Model accordingly. The Controller’s life blood is the user; without user interactions, the Controller has no purpose. It is the only part of the pattern the user should be interacting with.
三合会的最后一个组件是控制器。 它的工作是处理用户输入或提交的数据,并相应地更新模型。 控制器的生命是使用者; 没有用户交互,控制器就没有任何目的。 这是用户应该与之交互的模式的唯一部分。
The Controller can be summed up simply as a collector of information, which then passes it on to the Model to be organized for storage, and does not contain any logic other than that needed to collect the input. The Controller is also only connected to a single View and to a single Model, making it a one way data flow system, with handshakes and signoffs at each point of data exchange.
可以简单地将Controller概括为一个信息收集器,然后将其传递到要组织存储的Model上,除了收集输入所需的逻辑外,不包含任何逻辑。 Controller也仅连接到单个View和单个Model,从而使其成为一种单向数据流系统,并且在数据交换的每个点都具有握手和签名。
It’s important to remember the Controller is only given tasks to perform when the user interacts with the View first, and that each Controller function is a trigger, set off by the user’s interaction with the View. The most common mistake made by developers is confusing the Controller for a gateway, and ultimately assigning it functions and responsibilities that the View should have (this is normally a result of the same developer confusing the View component simply as a template). Additionally, it’s a common mistake to give the Controller functions that give it the sole responsibility of crunching, passing, and processing data from the Model to the View, whereas in the MVC pattern this relationship should be kept between the Model and the View.
重要的是要记住,只有当用户首先与View进行交互时,Controller才被赋予要执行的任务,并且每个Controller功能都是触发器,由用户与View的交互引起。 开发人员最常见的错误是混淆了Controller的网关,并最终为其分配了View应该具有的功能和职责(这通常是同一开发人员将View组件简单地作为模板混淆的结果)。 此外,赋予Controller函数以处理,从模型到视图的数据传递,传递和处理的唯一责任是一个常见的错误,而在MVC模式中,应在模型和视图之间保持这种关系。
PHP中的MVC (MVC in PHP)
It is possible to write a web application in PHP whose architecture is based on the MVC pattern. Let’s start with a bare bones example:
可以用PHP编写基于MVC模式的Web应用程序。 让我们从一个简单的例子开始:
<?php
class Model
{
public $string;
public function __construct(){
$this->string = "MVC + PHP = Awesome!";
}
}
<?php
class View
{
private $model;
private $controller;
public function __construct($controller,$model) {
$this->controller = $controller;
$this->model = $model;
}
public function output(){
return "<p>" . $this->model->string . "</p>";
}
}
<?php
class Controller
{
private $model;
public function __construct($model) {
$this->model = $model;
}
}
We have our project started with some very basic classes for each part of the pattern. Now we need to set up the relationships between them:
我们的项目从模式的每个部分的一些非常基础的类开始。 现在我们需要建立它们之间的关系:
<?php
$model = new Model();
$controller = new Controller($model);
$view = new View($controller, $model);
echo $view->output();
As you can see in the example above, we don’t have any Controller-specific functionality because we don’t have any user interactions defined with our application. The View holds all of the functionality as the example is purely for display purposes.
如您在上面的示例中看到的,我们没有任何特定于Controller的功能,因为我们没有与应用程序定义任何用户交互。 该视图拥有所有功能,因为该示例仅用于显示目的。
Let’s now expand the example to show how we would add functionality to the controller, thereby adding interactivity to the application:
现在让我们扩展该示例,以展示如何向控制器添加功能,从而为应用程序增加交互性:
<?php
class Model
{
public $string;
public function __construct(){
$this->string = “MVC + PHP = Awesome, click here!”;
}
}
<?php
class View
{
private $model;
private $controller;
public function __construct($controller,$model) {
$this->controller = $controller;
$this->model = $model;
}
public function output() {
return '<p><a href="mvc.php?action=clicked"' . $this->model->string . "</a></p>";
}
}
<?php
class Controller
{
private $model;
public function __construct($model){
$this->model = $model;
}
public function clicked() {
$this->model->string = “Updated Data, thanks to MVC and PHP!”
}
}
We’ve enhanced the application with some basic functionality. Setting up the relationship between our components now looks like this:
我们通过一些基本功能增强了该应用程序。 现在,建立组件之间的关系如下所示:
<?php
$model = new Model();
$controller = new Controller($model);
$view = new View($controller, $model);
if (isset($_GET['action']) && !empty($_GET['action'])) {
$controller->{$_GET['action']}();
}
echo $view->output();
Run the code and when you click on the link you’ll be able to see the string change its data.
运行代码,然后单击链接,您将看到字符串更改其数据。
结论 (Conclusion)
We’ve covered the basic theory behind the MVC pattern and have produced a very basic MVC application, but we still have a long way to go before we get into any nitty-gritty functionality.
我们已经介绍了MVC模式背后的基本理论,并且已经开发了一个非常基本的MVC应用程序,但是在进入任何实质性功能之前,我们还有很长的路要走。
Next up in the series we’ll cover some of the choices you face when trying to create a true MVC application on the web in PHP. Stay tuned!
在本系列的下一篇文章中,我们将介绍尝试在Web上使用PHP创建真正的MVC应用程序时遇到的一些选择。 敬请关注!
Image via Fotolia
图片来自Fotolia
Comments on this article are closed. Have a question about MVC Patterns and PHP? Why not ask it on our forums?
本文的评论已关闭。 对MVC模式和PHP有疑问吗? 为什么不在我们的论坛上提问呢?
mvc模式 php