gulp压缩css、js
In this article, we look at how you can use Gulp.js to automate a range of repetitive CSS development tasks to speed up your workflow.
在本文中,我们将研究如何使用Gulp.js自动化一系列重复CSS开发任务,以加快工作流程。
Web development requires little more than a text editor. However, you’ll quickly become frustrated with repetitive tasks that are essential for a modern website and fast performance, such as:
Web开发只需要一个文本编辑器即可。 但是,对于现代网站和快速的性能必不可少的重复性任务,您会很快感到沮丧,例如:
- converting or transpiling 转换或转译
- concatenating files 串联文件
- minifying production code 减少生产代码
- deploying updates to development, staging and live production servers. 将更新部署到开发,登台和实时生产服务器。
Some tasks must be repeated every time you make a change. The most infallible developer will forget to optimize an image or two and pre-production tasks become increasingly arduous.
每次进行更改时,都必须重复执行某些任务。 最可靠的开发人员会忘记优化一两个图像,并且预生产任务变得越来越繁重。
Fortunately, computers never complain about mind-numbing work. This article demonstrates how use Gulp.js to automate CSS tasks, including:
幸运的是,计算机永远不会抱怨麻木。 本文演示了如何使用Gulp.js自动执行CSS任务,包括:
- optimizing images 优化图像
compiling Sass
.scss
files编译Sass
.scss
文件- handling and inlining assets 处理和内联资产
- automatically appending vendor prefixes 自动附加供应商前缀
- removing unused CSS selectors 删除未使用CSS选择器
- minifying CSS 缩小CSS
- reporting file sizes 报告文件大小
- outputing sourcemaps for use in browser devtools 输出供在浏览器devtools中使用的源映射
- live-reloading in a browser when source files change. 当源文件更改时,在浏览器中实时重新加载。
为什么要使用Gulp? (Why Use Gulp?)
A variety of task runners are available for web projects including Gulp, Grunt, webpack, Parcel and even npm scripts. Ultimately, the choice is yours and it doesn’t matter what you use, as your site/app visitors will ever know or care.
各种任务运行程序可用于Web项目,包括Gulp , Grunt , webpack , Parcel甚至npm脚本。 最终,选择是您的选择,使用什么都无所谓,因为您的网站/应用访问者将永远知道或关心 。
Gulp is a few years old, but it’s stable, fast, supports many plugins, and is configured using JavaScript code. Writing tasks in code has several advantages, and you can modify output according to conditions — such as removing CSS sourcemaps when building the final files for live deployment.
Gulp 已有数年历史,但是它稳定,快速,支持许多插件 ,并且使用JavaScript代码进行配置。 用代码编写任务有许多优点,您可以根据条件修改输出-例如,在构建最终文件进行实时部署时删除CSS源映射。
示范代码 (Demonstration Code)
This tutorial’s code is available from GitHub. Ensure Git and Node.js are installed, then enter the following commands in your terminal to install and run the demonstration:
可从GitHub获得本教程的代码。 确保已安装Git和Node.js ,然后在终端中输入以下命令来安装和运行演示:
git clone https://github.com/craigbuckler/gulp4-css
cd gulp4-css
npm i gulp-cli -g
npm i
gulp
In your browser, navigate to http://localhost:8000/
or the External
URL shown.
在浏览器中,导航到http://localhost:8000/
或显示的External
URL。
Alternatively, you can create your own project following the steps below.
或者,您可以按照以下步骤创建自己的项目。
示例项目概述 (Example Project Overview)
This tutorial uses Gulp 4.0. This is the most recent stable version and the current default on npm.
本教程使用Gulp 4.0。 这是最新的稳定版本,当前默认为npm 。
Image file sizes will be minimized with gulp-imagemin, which optimizes JPG, GIF and PNG bitmaps as well as SVG vector graphics.
图像文件的大小将通过gulp-imagemin最小化,该文件可优化JPG,GIF和PNG位图以及SVG矢量图形。
A CSS file is built using:
CSS文件使用以下命令构建:
the Sass preprocessor, which compiles
.scss
syntax and partials into a singlemain.css
file, andSass预处理程序 ,它将
.scss
语法和部分编译为单个main.css
文件,并且the PostCSS postprocessor, which supplements
main.css
to provide asset management, vendor-prefixing, minification and more via its own plugins.PostCSS后处理器 ,它对
main.css
进行了补充,以通过其自己的插件提供资产管理,供应商前缀,缩小等功能。
Using both a preprocessor and a postprocessor provides coding flexibility.
同时使用预处理器和后处理器可提供编码灵活性。
Sass may not be as essential as it once was, but it remains a practical option for file splitting, organization, (static) variables, mixins and nesting. Alternative preprocessors include gulp-less for Less and gulp-stylus for Stylus.
Sass可能不再像以前那样重要,但是它仍然是文件拆分,组织,(静态)变量,mixins和嵌套的实用选项。 替代的预处理器包括一饮而尽少的少 ,并一饮而尽,手写笔的手写笔 。
The gulp-sass plugin is used in the example code below. This uses node-sass to call the LibSass C/C++ engine and is currently the fastest option. However, you could consider Dart Sass, which has become the primary implementation and receives language updates first. To use Dart Sass, change all npm installation and require
references from gulp-sass
to gulp-dart-sass
accordingly.
gulp-sass插件在以下示例代码中使用。 这使用node-sass来调用LibSass C / C ++引擎,目前是最快的选择。 但是,您可以考虑使用Dart Sass ,它已成为主要实现并首先接收语言更新。 要使用Dart Sass,请更改所有npm安装,并require
从gulp-sass
引用到gulp-dart-sass
。
Finally, you could forego a preprocessor and use PostCSS for all CSS transformations. It offers a range of plugins including those which replicate many (but not all) Sass syntax options.
最后,您可以放弃预处理器, 并对所有CSS转换使用PostCSS 。 它提供了一系列插件,包括那些可以复制许多(但不是全部)Sass语法选项 的插件 。
Gulp入门 (Getting Started with Gulp)
If you’ve never used Gulp before, please read “An Introduction to Gulp.js”. These are basic steps from your terminal:
如果您以前从未使用过Gulp,请阅读“ Gulp.js简介 ”。 这些是您终端的基本步骤:
Ensure a recent edition of Node.js is installed.
确保已安装最新版本的Node.js。
Install the Gulp command-line interface globally with
npm i gulp-cli -g
.使用
npm i gulp-cli -g
全局安装Gulp命令行界面。Create a new project folder — for example,
mkdir gulpcss
— and enter it (cd gulpcss
).创建一个新的项目文件夹-例如,
mkdir gulpcss
并输入它(cd gulpcss
)。Run
npm init
and answer each question (the defaults are fine). This will create apackage.json
project configuration file.运行
npm init
并回答每个问题(默认设置很好)。 这将创建一个package.json
项目配置文件。Create a
src
subfolder for source files:mkdir src
.为源文件创建一个
src
子文件夹:mkdir src
。
The example project uses the following subfolders:
示例项目使用以下子文件夹:
src/images
— image filessrc/images
—图像文件src/scss
— source Sass filessrc/scss
源Sass文件build
— the folder where compiled files are generatedbuild
—生成编译文件的文件夹
测试HTML页面 (Test HTML Page)
This tutorial concentrates on CSS-related tasks, but an index.html
file in the root folder is useful for testing. Add your own page code with a <link>
to the built stylesheet. For example:
本教程专注于与CSS相关的任务,但是根文件夹中的index.html
文件对于测试非常有用。 使用<link>
将您自己的页面代码添加到构建的样式表中。 例如:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<title>Using Gulp.js for CSS tasks</title>
<link rel="stylesheet" media="all" href="build/css/main.css">
</head>
<body>
<h1>My example page</h1>
</body>
</html>
模块安装 (Module Installation)
For this tutorial, most Node.js modules are installed as project dependencies so the CSS can be built on local development or live production servers. Alternatively, you could install them as development dependencies using the npm --save-dev
option so they must be built locally prior to deployment.
在本教程中,大多数Node.js模块都是作为项目依赖项安装的,因此CSS可以在本地开发或实时生产服务器上构建。 或者,您可以使用npm --save-dev
选项将它们安装为开发依赖项,因此必须在部署之前在本地构建它们。
To install Gulp and all plugins, run the following npm
command in your terminal from the project folder:
要安装Gulp和所有插件,请在终端中从项目文件夹运行以下npm
命令:
npm i gulp gulp-imagemin gulp-newer gulp-noop gulp-postcss gulp-sass gulp-size gulp-sourcemaps postcss-assets autoprefixer cssnano usedcss
All modules will be installed and listed in the "dependencies"
section of package.json
.
所有模块将被安装并列在package.json
的"dependencies"
部分中。
The browser-sync test server can now be installed — as a development dependency, since it should never be required on a live production device:
现在可以安装浏览器同步测试服务器-作为开发依赖项,因为永远不要在实时生产设备上使用它:
npm i browser-sync --save-dev
The module will be listed in the "devDependencies"
section of package.json
.
该模块将在package.json
的"devDependencies"
部分中列出。
创建一个Gulp任务文件 (Create a Gulp Task File)
Gulp tasks are defined in a JavaScript file named gulpfile.js
in your project root. Create it, then open the file in your editor (VS Code is a great option). Add the following code:
Gulp任务是在项目根目录中名为gulpfile.js
JavaScript文件中定义的。 创建它,然后在编辑器中打开文件( VS Code是一个不错的选择)。 添加以下代码:
(() => {
'use strict';
/**************** gulpfile.js configuration ****************/
const
// development or production
devBuild = ((process.env.NODE_ENV || 'development').trim().toLowerCase() === 'development'),
// directory locations
dir = {
src : 'src/',
build : 'build/'
},
// modules
gulp = require('gulp'),
noop = require('gulp-noop'),
newer = require('gulp-newer'),
size = require('gulp-size'),
imagemin = require('gulp-imagemin'),
sass = require('gulp-sass'),
postcss = require('gulp-postcss'),
sourcemaps = devBuild ? require('gulp-sourcemaps') : null,
browsersync = devBuild ? require('browser-sync').create() : null;
console.log('Gulp', devBuild ? 'development' : 'production', 'build');
})();
This defines a self-executing function and constants for:
这为以下项定义了一个自执行函数和常量:
devBuild
— settrue
whenNODE_ENV
is blank or set todevelopment
devBuild
—当NODE_ENV
为空白或设置为development
时设置为true
dir.src
— thesrc/
source file folderdir.src
—src/
源文件文件夹dir.build
— thebuild/
build folderdir.build
—build/
构建文件夹- Gulp and all plugin modules Gulp和所有插件模块
Note that sourcemaps
and browsersync
are only configured for development builds.
请注意,仅针对开发版本配置了sourcemaps
和browsersync
。
Gulp图像任务 (Gulp Image Task)
Create a src/images
folder and copy some image files into that or any of its subfolders.
创建一个src/images
文件夹并将一些图像文件复制到该文件夹或其任何子文件夹中。
Insert the following code below the console.log
in gulpfile.js
to define an images
processing task:
在gulpfile.js
中的console.log
下面插入以下代码,以定义images
处理任务:
/**************** images task ****************/
const imgConfig = {
src : dir.src + 'images/**/*',
build : dir.build + 'images/',
minOpts: {
optimizationLevel: 5
}
};
function images() {
return gulp.src(imgConfig.src)
.pipe(newer(imgConfig.build))
.pipe(imagemin(imgConfig.minOpts))
.pipe(size({ showFiles:true }))
.pipe(gulp.dest(imgConfig.build));
}
exports.images = images;
Configuration parameters are defined in imgConfig
, which sets:
配置参数在imgConfig
中定义,该参数设置:
the
.src
to any image insidesrc/images
or a subfolder.src
到src/images
或子文件夹中的任何图像the
.build
folder tobuild/images
, and.build
文件夹来build/images
,以及gulp-imagemin optimization options.
gulp-imagemin优化选项。
An images
function returns a Gulp stream, which pipes data through a series of plugins:
images
函数返回一个Gulp流,该流通过一系列插件传递数据:
gulp.src
is passed a source folder glob to examinegulp.src
传递给源文件夹glob进行检查the gulp-newer plugin removes any newer images already present in the build folder
gulp-newer插件将删除build文件夹中已经存在的所有较新图像
the gulp-imagemin plugin optimizes the remaining files
gulp-imagemin插件优化其余文件
the gulp-size plugin reports the resulting size of all processed files
gulp-size插件报告所有已处理文件的结果大小
the files are saved to the
gulp.dest
build folder.这些文件将保存到
gulp.dest
构建文件夹。
Finally, a public Gulp images
task is exported that calls the images
function.
最后,导出一个公共Gulp images
任务,该任务调用images
函数。
Save gulpfile.js
then run the images
task from the command line:
保存gulpfile.js
然后从命令行运行images
任务:
gulp images
The terminal will show a log such as:
终端将显示如下日志:
Gulp development build
[18:03:38] Using gulpfile /gulp4-css/gulpfile.js
[18:03:38] Starting 'images'...
[18:03:38] cave-0600.jpg 48.6 kB
[18:03:38] icons/alert.svg 308 B
[18:03:38] icons/fast.svg 240 B
[18:03:38] icons/reload.svg 303 B
[18:03:38] cave-1200.jpg 116 kB
[18:03:38] cave-1800.jpg 162 kB
[18:03:38] gulp-imagemin: Minified 3 images (saved 203 B - 19.3%)
[18:03:38] all files 328 kB
[18:03:38] Finished 'images' after 507 ms
Examine the created build/images
folder to find optimized versions of your images. If you run gulp images
again, nothing will occur because only newer files are processed.
检查创建的build/images
文件夹以查找build/images
优化版本。 如果再次运行gulp images
,则不会发生任何事情,因为仅处理较新的文件。
Gulp CSS任务 (Gulp CSS Task)
Create a src/scss
folder with a file named main.scss
. This is the root Sass file which imports other partials. You can organize these files as you like, but to get started, add:
使用名为main.scss
的文件创建一个src/scss
文件夹。 这是导入其他部分的Sass根文件。 您可以根据需要组织这些文件,但要开始使用,请添加:
// main.scss
@import 'base/_base';
Create a src/scss/base
folder and add a _base.scss
file with the following code:
创建一个src/scss/base
文件夹,并使用以下代码添加_base.scss
文件:
// base/_base.scss partial
$font-main: sans-serif;
$font-size: 100%;
body {
font-family: $font-main;
font-size: $font-size;
color: #444;
background-color: #fff;
}
Insert the following code below the images
task in gulpfile.js
to define a css
processing task:
将以下代码插入gulpfile.js
中的images
任务下方,以定义css
处理任务:
/**************** CSS task ****************/
const cssConfig = {
src : dir.src + 'scss/main.scss',
watch : dir.src + 'scss/**/*',
build : dir.build + 'css/',
sassOpts: {
sourceMap : devBuild,
imagePath : '/images/',
precision : 3,
errLogToConsole : true
},
postCSS: [
require('usedcss')({
html: ['index.html']
}),
require('postcss-assets')({
loadPaths: ['images/'],
basePath: dir.build
}),
require('autoprefixer')({
browsers: ['> 1%']
}),
require('cssnano')
]
};
function css() {
return gulp.src(cssConfig.src)
.pipe(sourcemaps ? sourcemaps.init() : noop())
.pipe(sass(cssConfig.sassOpts).on('error', sass.logError))
.pipe(postcss(cssConfig.postCSS))
.pipe(sourcemaps ? sourcemaps.write() : noop())
.pipe(size({ showFiles: true }))
.pipe(gulp.dest(cssConfig.build))
.pipe(browsersync ? browsersync.reload({ stream: true }) : noop());
}
exports.css = gulp.series(images, css);
Configuration parameters are defined in cssConfig
which sets:
配置参数在cssConfig
中定义,该参数设置:
the
.src
file tosrc/scss/main.scss
.src
文件到src/scss/main.scss
a
.watch
folder to any file withinsrc/scss
or its subfolders.watch
文件夹到src/scss
或其子文件夹中的任何文件the
.build
folder tobuild/css
, and.build
文件夹来build/css
,以及node-sass options passed by gulp-sass in
.sassOpts
..sassOpts
-sass在.sassOpts
传递的node-sass选项 。
cssConfig.postCSS
defines an array of PostCSS plugins and configuration options. The first is usedcss, which removes unused selectors by examining the example index.html
file.
cssConfig.postCSS
定义了PostCSS插件和配置选项的数组。 第一个是usedcss ,它通过检查示例index.html
文件来删除未使用的选择器。
This is followed by postcss-assets, which can resolve image URL paths and information in the CSS files. For example, if myimage.png
is a 400×300 PNG bitmap, the following code:
其次是postcss-assets ,它可以解析图像URL路径和CSS文件中的信息。 例如,如果myimage.png
是400×300 PNG位图,则以下代码:
.myimage {
background-image: resolve('myimage.jpg');
width: width('myimage.png');
height: height('myimage.png');
background-size: size('myimage.png');
}
is translated to:
转换为:
.myimage {
background-image: url('/images/myimage.png');
width: 400px;
height: 300px;
background-size: 400px 300px;
}
It’s also possible to inline bitmap and SVG images. For example:
还可以内嵌位图和SVG图像。 例如:
.mysvg {
background-image: inline('mysvg.svg');
/* url('data:image/svg+xml;charset=utf-8,... */
}
autoprefixer is the famous PostCSS plugin which adds vendor prefixes according to information from caniuse.com. In the configuration above, any browser with a global market share of 1% or more will have vendor prefixes added. For example:
autoprefixer是著名的PostCSS插件,它根据caniuse.com的信息添加供应商前缀。 在上述配置中,任何具有1%或更高的全球市场份额的浏览器都将添加供应商前缀。 例如:
user-select: none;
becomes:
变成:
-webkit-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
Finally, cssnano minifies the resulting CSS file by rearranging properties, removing unnecessary units, deleting whitespace, and so on. Several alternatives are available, such as post-clean, but cssnano generates a slightly smaller file in the demonstration code.
最后, cssnano通过重新排列属性,删除不必要的单元,删除空格等来最小化生成CSS文件。 可以使用几种替代方法,例如post-clean ,但是cssnano在演示代码中生成一个稍小的文件。
A css
function returns a Gulp stream which pipes data through a series of plugins:
一个css
函数返回一个Gulp流,该流通过一系列插件通过管道传递数据:
gulp.src
is passed a sourcesrc/scss/main.scss
file to examine.gulp.src
传递了源src/scss/main.scss
文件进行检查。If
devBuild
istrue
, the gulp-sourcemaps plugin is initialized. Otherwise, the gulp-noop does nothing.如果
devBuild
为true
,则将初始化gulp-sourcemaps插件。 否则, gulp-noop将不执行任何操作。The gulp-sass plugin preprocesses
main.scss
to CSS using thecssConfig.sassOpts
configuration options. Note theon('error')
event handler prevents Gulp terminating when a Sass syntax error is encountered.main.scss
-sass插件使用cssConfig.sassOpts
配置选项将cssConfig.sassOpts
预处理为CSS。 注意on('error')
事件处理程序可防止Gulp在遇到Sass语法错误时终止。The resulting CSS is piped into gulp-postcss, which applies the plugins described above.
生成CSS通过管道传递到gulp-postcss ,后者应用了上述插件。
- If the sourcemap is enabled, it’s appended as data to the end of the CSS file. 如果启用了源映射,则将其作为数据附加到CSS文件的末尾。
The gulp-size plugin displays the final size of the CSS file.
gulp-size插件显示CSS文件的最终大小。
The files are saved to the
gulp.dest
build folder.这些文件将保存到
gulp.dest
构建文件夹。If
browsersync
is enabled (devBuild
must betrue
), an instruction is sent to browser-sync to refresh the CSS in all connected browsers (see below).如果
browsersync
启用(devBuild
必须是true
),指令被发送到browsersync以刷新所有连接的浏览器CSS(见下文)。
Finally, a public css
task is exported which calls the images
function followed by the css
function in turn using gulp.series()
. This is necessary because the CSS relies on images being available in the build/images
folder.
最后,导出一个公共css
任务,该任务先使用gulp.series()
依次调用images
函数和css
函数。 这是必需的,因为CSS依赖于build/images
文件夹中可用的build/images
。
Save gulpfile.js
, then run the task from the command line:
保存gulpfile.js
,然后从命令行运行任务:
gulp css
The terminal will show a log such as:
终端将显示如下日志:
Gulp development build
[14:16:25] Using gulpfile /gulp4-css/gulpfile.js
[14:16:25] Starting 'css'...
[14:16:25] Starting 'images'...
[14:16:25] gulp-imagemin: Minified 0 images
[14:16:25] Finished 'images' after 61 ms
[14:16:25] Starting 'css'...
[14:16:26] main.css 9.78 kB
[14:16:26] Finished 'css' after 900 ms
[14:16:26] Finished 'css' after 967 ms
Examine the created build/css
folder to find a development version of the resulting main.css
file containing a sourcemap data:
检查创建的build/css
文件夹,以找到包含sourcemap数据的所得main.css
文件的开发版本:
body {
font-family: sans-serif;
font-size: 100%;
color: #444;
background-color: #fff; }
/*# sourceMappingURL=data:application/json;charset=utf8;base64,...
自动化工作流程 (Automating Your Workflow)
Running one task at a time and manually refreshing all browsers is no fun. Fortunately, Browsersync provides a seemingly magical solution:
一次运行一个任务并手动刷新所有浏览器并不有趣。 幸运的是, Browsersync提供了一个看似神奇的解决方案:
- It implements a development web server or proxies an existing server. 它实现开发Web服务器或代理现有服务器。
- Code changes are dynamically applied and CSS can refresh without a full page reload 代码更改是动态应用的,CSS可以刷新而无需重新加载整个页面
- Connected browsers can mirror scrolling and form input. For example, you complete a form on your desktop PC and see it happening on a mobile device. 连接的浏览器可以镜像滚动和表单输入。 例如,您在台式机上填写表格,然后在移动设备上看到该表格。
- It’s fully compatible with Gulp and other build tools. 它与Gulp和其他构建工具完全兼容。
Insert the following code below the css
task in gulpfile.js
to define a server
function to launch Browsersync and a watch
function to monitor file changes:
将以下代码插入gulpfile.js
中的css
任务下方,以定义用于启动Browsersync的server
功能和用于监视文件更改的watch
功能:
/**************** server task (private) ****************/
const syncConfig = {
server: {
baseDir : './',
index : 'index.html'
},
port : 8000,
open : false
};
// browser-sync
function server(done) {
if (browsersync) browsersync.init(syncConfig);
done();
}
/**************** watch task ****************/
function watch(done) {
// image changes
gulp.watch(imgConfig.src, images);
// CSS changes
gulp.watch(cssConfig.watch, css);
done();
}
/**************** default task ****************/
exports.default = gulp.series(exports.css, watch, server);
The browser-sync configuration parameters are defined in syncConfig
, which sets options such as the port and default file.
浏览器同步配置参数在syncConfig
中定义,该参数设置诸如端口和默认文件之类的选项 。
The server
function initiates Browsersync and a done()
callback is executed so Gulp knows it has completed.
server
功能将启动Browsersync并执行done()
回调,以便Gulp知道已完成。
Browsersync is able to watch for file changes itself but, in this case, we want to control it via Gulp to ensure refreshes only occur when a Sass change causes main.css
to be rebuilt.
Browsersync本身可以监视文件更改,但是在这种情况下,我们希望通过Gulp对其进行控制,以确保仅在Sass更改导致main.css
重建时才进行刷新。
The watch
function uses gulp.watch()
to monitor files and trigger the appropriate function (optionally within gulp.series()
or gulp.parallel()
methods). Again, a done()
callback is executed once the function has completed.
watch
函数使用gulp.watch()
监视文件并触发适当的函数(可选在gulp.series()
或gulp.parallel()
方法中)。 同样,函数完成后,将执行done()
回调。
Finally, a default task is created which can be executed by running gulp
without arguments. It calls the css
task (which also runs images()
) to build all files, runs the watch()
function to monitor updates, and launches the Browsersync server.
最后,创建一个默认任务,可以通过运行不带参数的gulp
来执行该任务。 它调用css
任务(还将运行images()
)来构建所有文件,运行watch()
函数以监视更新,并启动Browsersync服务器。
Save gulpfile.js
and run the default task from the command line:
保存gulpfile.js
并从命令行运行默认任务:
gulp
The terminal will show a log but, unlike before, it will not terminate and remain running:
终端将显示日志,但与以前不同,它将不会终止并保持运行:
Gulp development build
[14:32:05] Using gulpfile /gulp4-css/gulpfile.js
[14:32:05] Starting 'default'...
[14:32:05] Starting 'images'...
[14:32:05] gulp-imagemin: Minified 0 images
[14:32:05] Finished 'images' after 64 ms
[14:32:05] Starting 'css'...
[14:32:05] main.css 9.78 kB
[14:32:05] Finished 'css' after 843 ms
[14:32:05] Starting 'watch'...
[14:32:05] Finished 'watch' after 33 ms
[14:32:05] Starting 'server'...
[14:32:06] Finished 'server' after 19 ms
[14:32:06] Finished 'default' after 967 ms
[Browsersync] Access URLs:
-------------------------------------
Local: http://localhost:8000
External: http://192.168.1.234:8000
-------------------------------------
UI: http://localhost:3001
UI External: http://localhost:3001
-------------------------------------
[Browsersync] Serving files from: ./
Your PC is now running a web server from http://localhost:8000
. Other devices on the network can connect to the External URL. Open the URL in a browser or two, then make changes to any .scss
file. The results are immediately refreshed.
您的PC现在正在从http://localhost:8000
运行Web服务器。 网络上的其他设备可以连接到外部 URL。 在一两个浏览器中打开URL,然后对任何.scss
文件进行更改。 结果将立即刷新。
Examine any element in the devtools and the Styles panel will show the location of the pre-compiled Sass code. You can click the filename to view the full source:
检查devtools中的任何元素,“ 样式”面板将显示预编译的Sass代码的位置。 您可以单击文件名以查看完整源代码:
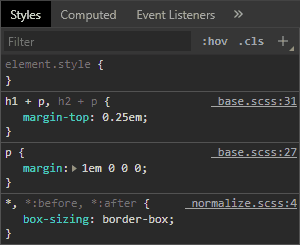
Finally, press Ctrl + C to stop the Gulp task running in your terminal.
最后,按Ctrl + C停止在终端中运行Gulp任务。
现场制作代码 (Live Production Code)
The NODE_ENV
environment variable must be set to production
so Gulp tasks know when to produce final code and disable sourcemap generation. On Linux and macOS terminals:
必须将NODE_ENV
环境变量设置为production
以便Gulp任务知道何时生成最终代码并禁用sourcemap生成。 在Linux和macOS终端上:
NODE_ENV=production
Windows Powershell:
Windows Powershell:
$env:NODE_ENV="production"
Windows legacy command line:
Windows旧版命令行:
set NODE_ENV=production
You can either:
您可以:
Install Gulp and run tasks directly on the live server. Ideally,
NODE_ENV
should be permanently set on production machines by modifying the startup script. For example, addexport NODE_ENV=production
to the end of a Linux~/.bashrc
file.安装Gulp并直接在实时服务器上运行任务。 理想情况下,应通过修改启动脚本在生产机器上永久设置
NODE_ENV
。 例如,将export NODE_ENV=production
添加到export NODE_ENV=production
~/.bashrc
文件的末尾。- Create production code locally, then upload to live servers. 在本地创建生产代码,然后上传到实时服务器。
Run gulp css
to generate the final code.
运行gulp css
生成最终代码。
To return to development mode, change NODE_ENV
to development
or an empty string.
要返回开发模式, NODE_ENV
更改为development
或一个空字符串。
下一步 (Next Steps)
This article demonstrates a possible Gulp CSS workflow, but it can be adapted for any project:
本文演示了可能的Gulp CSS工作流程,但可以将其应用于任何项目:
There are more than 3,700 Gulp plugins. Many help with CSS, but you’ll find others for HTML, templating, image handling, JavaScript, server-side languages, linting and more.
有3,700多个Gulp插件 。 许多关于CSS的帮助,但是您会发现其他有关HTML,模板,图像处理,JavaScript,服务器端语言,linting等的信息。
There are hundreds of PostCSS plugins and it’s simple to write your own.
Whichever tools you choose, I recommend you:
无论您选择哪种工具,我都建议您:
- Automate the most frustrating, time-consuming or performance-improving tasks first. For example, optimizing images could shave hundreds of Kilobytes from your total page weight. 首先自动化最令人沮丧,耗时或性能提高的任务。 例如,优化图像可以使页面总重量减少数百千字节。
- Don’t over-complicate your build process. A few hours should be adequate to get started. 不要使您的构建过程过于复杂。 几个小时就足以开始使用。
- Try other task runners but don’t switch on a whim! 尝试其他任务赛跑者,但不要一时兴起!
The code above is available from GitHub and you can view the whole gulpfile.js
configuration. Please use it as you wish.
上面的代码可从GitHub获得 ,您可以查看整个gulpfile.js
配置 。 请根据需要使用它。
gulp压缩css、js