ava美学
Most development teams want to get their codebase into a better, more maintainable state. But what definition of better should be chosen? In many cases, it is not necessary to dig deep into Domain Driven Design (DDD) to achieve this goal. Sometimes, it’s even counter productive. But one of the most basic collections of principles can help each team a lot already: Clean Code.
大多数开发团队希望使他们的代码库处于更好,更可维护的状态。 但是应该选择更好的定义是什么? 在许多情况下,没有必要深入研究域驱动设计(DDD)来实现此目标。 有时,它甚至适得其反。 但是,最基本的原则集合之一可以为每个团队提供很多帮助:Clean Code。
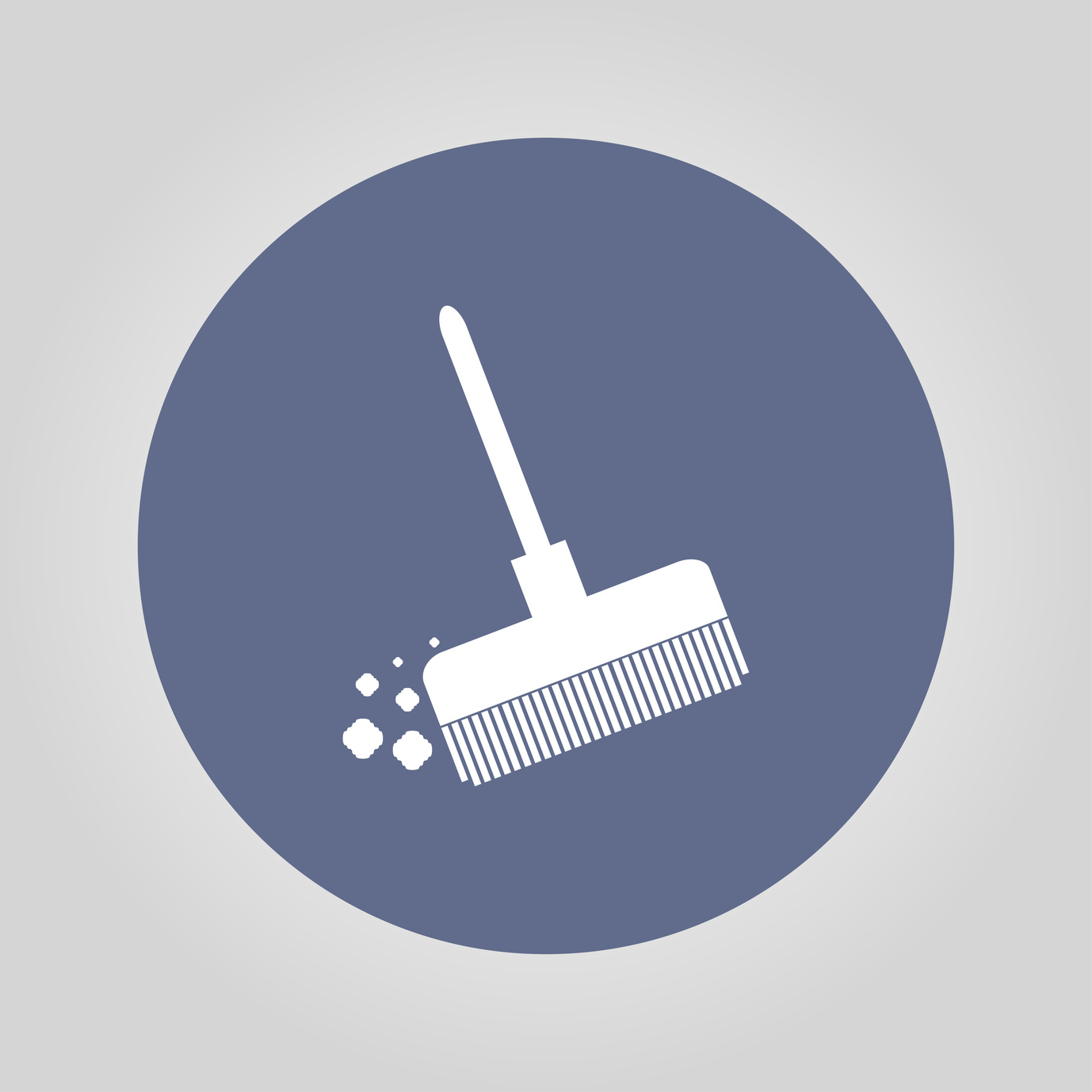
目标 (The Goal)
The Clean Code book by Robert C. Martin summarizes many simple and advanced improvements to get better, understandable, and therefore more maintainable code. Let’s take the following code snippet as an example:
罗伯特·C·马丁(Robert C. Martin)编写的“ 干净代码 ”( Clean Code)总结了许多简单和高级的改进,以使代码更好,更易于理解,从而更易于维护。 让我们以以下代码段为例:
public function getJobs($date)
{
$ret = array();
$res = $this->db->query(
'SELECT * FROM jobs WHERE year=' . date('Y', $date) . ' AND month='
. date('m', $date)
);
$res = $res->fetchAll();
foreach ($res as $data) {
$j = new Job(/* $data['...'] */);
$j->rev = $j->time * $j->euro;
$ret[] = $j;
}
return $ret;
}
The purpose of this already simple method seems to be to return jobs for a certain date. While this intention is clear, reading through the method and understanding how it performs its job is not that easy. Once done with it the algorithm appears pretty easy: first it fetches some data from a database, then it prepares job objects, calculates some additional data and returns the objects.
这种已经很简单的方法的目的似乎是在特定日期返回作业。 尽管这个意图很明确,但通读方法并理解其工作方式并不容易。 完成后,该算法看起来非常简单:首先,它从数据库中获取一些数据,然后准备作业对象,计算一些其他数据,然后返回对象。
But the time spent to understand this simple code snippet is wasted. As you, a developer, analyze your and other people’s everyday work, you will notice that you spend much more time reading and understanding code than actually writing it.
但是,浪费在理解这个简单代码段上的时间却浪费了。 当您作为开发人员分析您和他人的日常工作时,您会发现与实际编写代码相比,您花费更多的时间阅读和理解代码。
A low hanging fruit would be to just rename the local variables to improve readability:
一个低挂的结果就是重命名局部变量以提高可读性:
public function getJobs($date)
{
$jobs = array();
$statement = $this->db->query(
'SELECT * FROM jobs WHERE year=' . date('Y', $date) . ' AND month='
. date('m', $date)
);
$rows = $statement->fetchAll();
foreach ($rows as $row) {
$job = new Job(/* $row['...'] */);
$job->rev = $job->time * $job->euro;
$jobs[] = $job;
}
return $jobs;
}
The variable previously known as $ret
was renamed to $jobs
. This name now clearly indicates what we can expect from it: a list ob jobs. Furthermore, this cannot be easily confused with $ret
which was renamed into $statement
and $rows
. We now understand what’s going on much better.
以前称为$ret
的变量被重命名为$jobs
。 现在,该名称清楚地表明了我们可以期望的:作业列表。 此外,不能轻易将其与$ret
混淆,后者被重命名为$statement
和$rows
。 现在,我们了解发生了什么事情。
Another step towards improving readability would be to break the code up into logical pieces that belong together and make it read more like a book, or newspaper, where paragraphs are information groups:
改善可读性的另一步是将代码分解成逻辑部分,使它们在一起,使阅读起来更像书或报纸,其中段落是信息组:
public function getJobs($date)
{
$statement = $this->db->query(
'SELECT * FROM jobs WHERE year=' . date('Y', $date) . ' AND month='
. date('m', $date)
);
$rows = $res->fetchAll();
$jobs = array();
foreach ($rows as $row) {
$job = new Job(/* $row['...'] */);
$job->rev = $job->time * $job->euro;
$jobs[] = $job;
}
return $jobs;
}
There are two paragraphs now. In the first one, there is a number of rows fetched from the database. The second paragraph converts these rows into objects. This minor relocation of the declaration of $jobs
and the delimiting blank line did not only ease the reading flow of the method, but also prepared a possible subsequent refactoring step of splitting the tasks into private methods with the intention of revealing names.
现在有两段。 在第一个中,从数据库中获取了许多行。 第二段将这些行转换为对象。 $jobs
声明和定界空白行的微小重定位不仅简化了该方法的阅读流程,而且还准备了一个可能的后续重构步骤,该任务将任务拆分为专用方法以显示名称。
This is just the tip of the iceberg that Clean Code offers.
这只是Clean Code提供的冰山一角。
方法论 (The Methodology)
As can be seen above, the refactoring steps are really minimalistic – baby steps. This is not just for the purpose of presentation but should be the way whenever you refactor. The recommended approach is to commit after each and every step:
从上面可以看出,重构步骤实际上非常简单- 婴儿步骤 。 这不仅是出于演示的目的,而且应该是重构时的一种方式。 推荐的方法是在每个步骤之后进行提交:
Choose 1 single refactoring step
选择1个重构步骤
- Execute the selected step 执行所选步骤
- Verify the code still works 验证代码是否仍然有效
- Commit current state and goto 1 提交当前状态并转到1
Most importantly, this procedure gives you a safety line so you can easily jump one step back. You should do this whenever you feel like you might run in the wrong direction. If you need to start debugging – don’t. It is way too much effort to try to fix a refactoring instead of reverting it. Chances are good that you’ll lose your way in debugging and it will eat up more time than starting over would. If you go with baby steps, a reversion of the latest step would take 5 minutes instead.
最重要的是,此过程为您提供了一条安全线,因此您可以轻松地向后退一步。 只要您觉得自己可能走错了方向,就应该这样做。 如果您需要开始调试–不需要。 尝试修复重构而不是还原它是一种过多的工作。 很有可能您会迷路于调试,并且比重新开始要花更多的时间。 如果您使用婴儿步,则恢复最新步骤将需要5分钟。
Furthermore, this approach helps you stay focused and open minded about what the eventual goal of your refactoring is. Code is complex and our brain often has a biased idea of what the optimal outcome of this complex structure is. By keeping your mind open to adjusting the goal during the process, you allow it to find better solutions along the way.
此外,这种方法可以帮助您集中精力,对重构的最终目标持开放态度。 代码是复杂的,我们的大脑通常对这种复杂结构的最佳结果有偏见。 通过在过程中保持开放的态度来调整目标,您可以让其在整个过程中找到更好的解决方案。
The process also allows you to stop refactoring at any point in time without losing progress. An urgent bug to fix? A colleague asks for sparring? Lunch? No problem, you can either just merge the working state you have or leave it in a branch to pick it up later again.
该过程还使您可以随时停止重构,而不会失去进度。 紧急漏洞修复? 一位同事要求陪练? 午餐? 没问题,您可以合并现有的工作状态,也可以将其留在分支中以便以后再次使用。
Still, there is one draw-back with frequent commits during refactoring: so many small commits might pollute your version control history. If you are using Git, you can mitigate this by squashing the commits of a refactoring into a single one before pushing upstream.
尽管如此,在重构过程中仍存在频繁提交的缺点:这么多小的提交可能会污染您的版本控制历史记录。 如果您使用的是Git,则可以通过在向上游推送重构之前将重构的提交压缩为单个来减轻这种情况。
前提条件 (The Preconditions)
Taking Clean Code as a goal and applying baby steps are good building blocks for sustainable refactoring, but there is more to take into account.
将“ 清洁代码”作为目标并采取一些必要的步骤是实现可持续重构的良好基础,但还有更多需要考虑的因素。
First of all, it is not a good idea to perform a refactoring just because you can or because you think the current code looks ugly. Changing the code is always a risk and you should take no risk without good reason. One good reason would e.g. be that you often need to work on a single piece of code which is hard to grasp and where changes cascade throughout your system. But there are many other reasons like frequent bugs, unforeseen business requirements, etc. It is important that you analyze the reasons carefully, and not just blindly run into refactoring.
首先,仅仅因为您可以或者因为您认为当前代码看起来丑陋而执行重构并不是一个好主意。 更改代码总是有风险的,没有正当理由,您应该不要冒险。 一个很好的理由是,例如,您经常需要处理一段难以理解的代码,并且整个系统级联的更改都在其中。 但是还有许多其他原因,例如频繁出现的错误,不可预见的业务需求等。重要的是,仔细分析原因,而不仅仅是盲目地进行重构。
Secondly, it’s important to have at least some degree of automated tests that cover the most important business cases where the code being refactored is used. Legacy systems are typically hard to test with unit or integration test methods. Therefore, it’s recommended not to try to apply these methods through a brute force approach. Instead, we found it quite useful to apply tests through the front-end – in our case using Mink and PHPUnit.
其次,至少要有一定程度的自动化测试来覆盖使用重构代码的最重要的业务案例,这一点很重要。 传统系统通常很难用单元或集成测试方法进行测试。 因此,建议不要尝试通过蛮力方法应用这些方法。 相反,我们发现通过前端应用测试非常有用–在我们的示例中,使用Mink和PHPUnit。
But, as with so many decisions in software development, these are only rules of thumb and every project has its own requirements and priorities. Join me in my Clean Code workshop at the WebSummerCamp 2016 to learn more. Bring a companion, too – there’s a dedicated program for significant others, too, so bring company and make it a work vacation!
但是,与软件开发中的许多决定一样,这些只是经验法则,每个项目都有其自己的要求和优先级。 加入我的WebSummerCamp 2016 清洁代码研讨会中,了解更多信息。 也要带同伴 -也有针对重要他人的专门计划,因此带公司陪伴并使其成为工作假期!
翻译自: https://www.sitepoint.com/cleaning-up-code-is-refactoring-for-aesthetics-worth-it/
ava美学