symfony连接数据库
Testing code that interacts with a database can be a massive pain. Some developers mock database abstractions, and thus do not test the actual query. Others create a test database for the development environment, but this can also be a pain when it comes to continuous integration and maintaining the state of this database.
测试与数据库交互的代码可能会非常麻烦。 一些开发人员模拟数据库抽象,因此不测试实际查询。 其他人为开发环境创建了一个测试数据库,但是在持续集成和维护该数据库的状态时,这也可能会很痛苦。
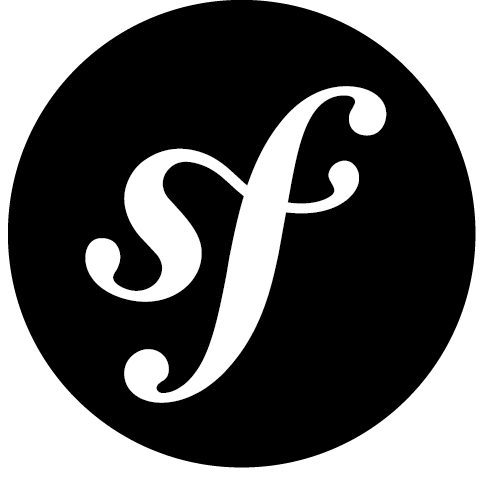
In-memory databases are an alternative to these options. As they exist only in the memory of the application, they are truly disposable and great for testing. Thankfully, these are very easy to set up with Symfony applications that use Doctrine. Try reading our guide to functional testing with Symfony after this to learn about testing the end-to-end behaviour of an application.
内存数据库是这些选项的替代方法。 由于它们仅存在于应用程序的内存中,因此它们确实是一次性的,非常适合测试。 幸运的是,使用使用Doctrine的Symfony应用程序可以很容易地设置它们。 之后,请尝试阅读我们的Symfony功能测试指南,以了解有关测试应用程序端到端行为的信息。
Symfony环境配置 (The Symfony Environment Configuration)
One of the most powerful features of the Symfony framework is the ability to create different environments with their own unique configuration. This feature can be overlooked by Symfony developers, especially when it comes to the lesser known test environment that is investigated here.
Symfony框架最强大的功能之一就是能够使用自己独特的配置创建不同的环境。 Symfony开发人员可能会忽略此功能,特别是涉及此处研究的鲜为人知的测试环境时。
The Symfony guide to mastering and creating new environments explains how the framework handles the configuration for different environments and shows some useful examples. The configuration file that needs to be edited to set up disposable test databases is app/config/config_test.php
. When the application is accessed in the test suite, the kernel will be loaded using the test environment and this configuration file will be processed.
Symfony 掌握和创建新环境的指南说明了框架如何处理不同环境的配置,并显示了一些有用的示例。 需要编辑以设置一次性测试数据库的配置文件是app/config/config_test.php
。 在测试套件中访问应用程序时,将使用测试环境加载内核,并将处理此配置文件。
具有原则的内存数据库 (In-Memory Databases with Doctrine)
SQLite3 supports in-memory databases which are great for testing. Using these, an application can be tested by actually sending SQL queries to a functioning database, removing the need to tediously mock repository classes with predefined behaviour. The database will be fresh at the start of the test and cleanly destroyed at the end.
SQLite3支持非常适合测试的内存数据库 。 使用这些,可以通过将SQL查询实际发送到正常运行的数据库来测试应用程序,而无需繁琐地模拟具有预定义行为的存储库类。 该数据库将在测试开始时是新鲜的,并在测试结束时被彻底销毁。
To override the default doctrine connection configuration, the lines below need to be added to the test environment configuration file. If there are multiple doctrine connections configured in the application, it may need to be adapted slightly to match.
要覆盖默认的学说连接配置,需要将以下各行添加到测试环境配置文件中。 如果在应用程序中配置了多个教义连接,则可能需要稍加调整以使其匹配。
# app/config/config_test.yml
doctrine:
dbal:
driver: pdo_sqlite
memory: true
charset: UTF8
在测试类中使用数据库 (Using the Database in Test Classes)
When using this shiny new in-memory database in test classes, the schema must first be constructed. This means creating the tables from the entities and loading any fixtures that are required for the test suite.
在测试类中使用此崭新的内存数据库时,必须首先构建架构。 这意味着从实体创建表并加载测试套件所需的所有固定装置。
The class below can be used as a database primer that does most of this work. It has the same effect as forcefully running the doctrine schema update console command.
下面的类可用作完成大多数工作的数据库入门。 它具有与强制运行doctrine schema update console命令相同的效果。
<?php
namespace Tests\AppBundle;
use Doctrine\ORM\EntityManager;
use Doctrine\ORM\Tools\SchemaTool;
use Symfony\Component\HttpKernel\KernelInterface;
class DatabasePrimer
{
public static function prime(KernelInterface $kernel)
{
// Make sure we are in the test environment
if ('test' !== $kernel->getEnvironment()) {
throw new \LogicException('Primer must be executed in the test environment');
}
// Get the entity manager from the service container
$entityManager = $kernel->getContainer()->get('doctrine.orm.entity_manager');
// Run the schema update tool using our entity metadata
$metadatas = $entityManager->getMetadataFactory()->getAllMetadata();
$schemaTool = new SchemaTool($entityManager);
$schemaTool->updateSchema($metadatas);
// If you are using the Doctrine Fixtures Bundle you could load these here
}
}
If the entity manager is required to test a class, the primer must be applied:
如果要求实体管理员测试一门课程,则必须应用入门知识:
<?php
namespace Tests\AppBundle;
use Symfony\Bundle\FrameworkBundle\Test\KernelTestCase;
use Tests\AppBundle\DatabasePrimer;
class FooTest extends KernelTestCase
{
public function setUp()
{
self::bootKernel();
DatabasePrimer::prime(self::$kernel);
}
public function testFoo()
{
$fooService = self::$kernel->getContainer()->get('app.foo_service');
// ...
}
}
In the example above the container is used to get the service that is being tested. If this service depends on the entity manager, it will be constructed with the same entity manager that is primed in the setUp
method. If more control is required, maybe to mock another dependency, the entity manager can always be retrieved from the container and used to manually instantiate the class that needs testing.
在上面的示例中,容器用于获取正在测试的服务。 如果此服务依赖于实体管理器,则将使用在setUp
方法中启动的同一实体管理器来构造它。 如果需要更多控制权(可能是为了模拟另一个依赖关系),则始终可以从容器中检索实体管理器,并使用它手动实例化需要测试的类。
It may also be a good idea to use the Doctrine Fixtures Bundle to populate the database with test data, but this will depend on your use case.
使用Doctrine Fixtures捆绑包将测试数据填充到数据库中也是一个好主意,但这取决于您的用例。
翻译自: https://www.sitepoint.com/quick-tip-testing-symfony-apps-with-a-disposable-database/
symfony连接数据库