vue 父组件视图传递数据
In MVC based architectures, working with template engines is an inevitable part of the development routine. It usually goes like this: we prepare and pass the data to the view. In the view, we print them based on our layout design.
在基于MVC的体系结构中,使用模板引擎是开发例程中不可避免的一部分。 通常是这样的:我们准备数据并将其传递给视图。 在视图中,我们根据布局设计打印它们。
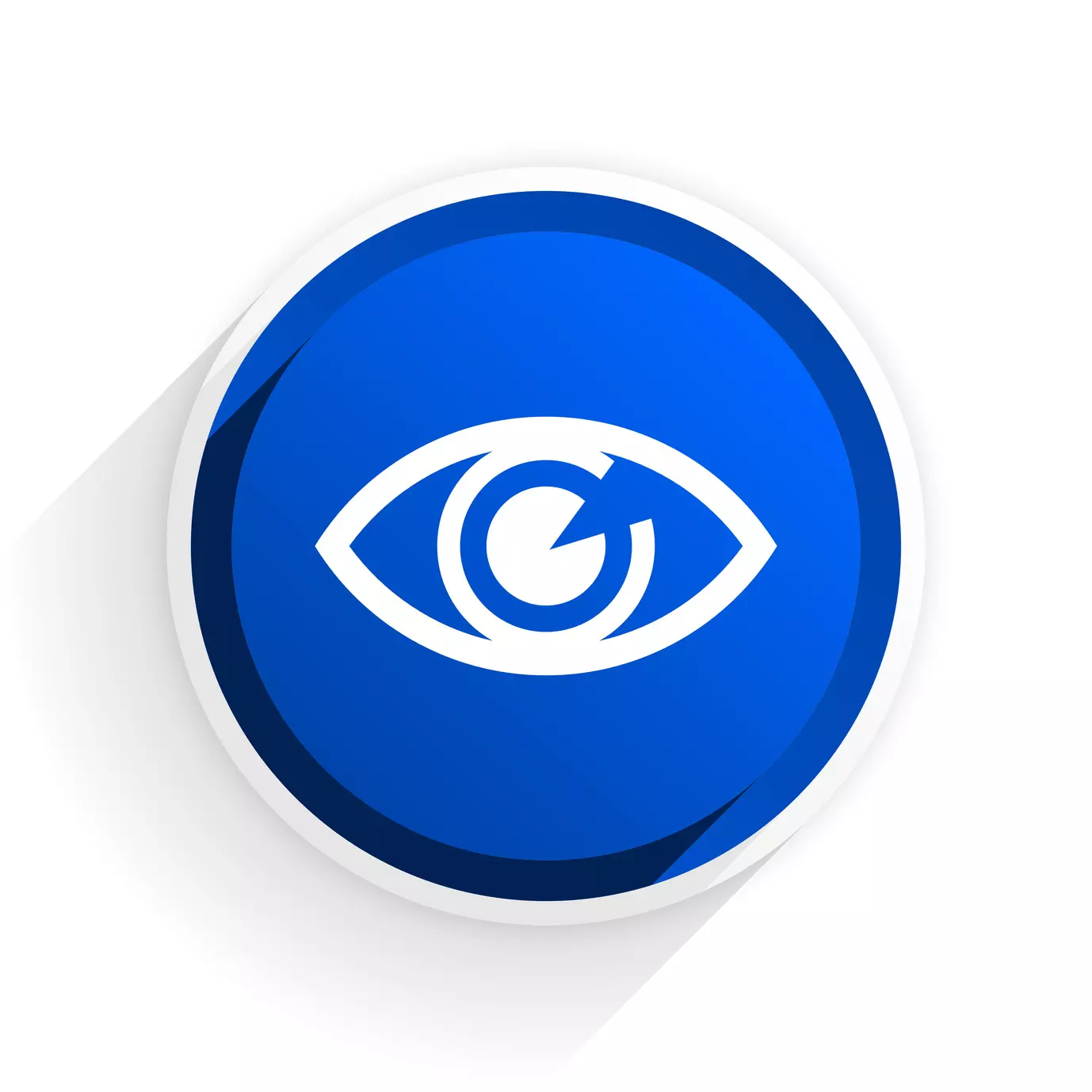
Here is a basic example of how it works using Twig.
这是使用Twig的基本示例。
<?php
// Twig initialization
// Preparing data
$user = 'user data';
$posts = 'posts';
$comments = 'comments';
$twig->render('author.page', [
'user' => $user,
'posts' => $posts,
'comments' => $comments,
]);
// ...
There are times, however, when the number of variables might be much higher than this: ten or more. In that case, we’ll have a tall list of variables (as an associative array), being passed to the respective template. It gets messy and unreadable quickly. If only there was a way to just list what we need by name, and have PHP take care of the rest for us. Well… there is!
但是,有时变量的数量可能远远大于此:十个或更多。 在这种情况下,我们将有大量的变量(作为关联数组)被传递给相应的模板。 它变得凌乱且无法快速读取。 如果只有一种方法可以按名称列出我们需要的东西,然后让PHP为我们处理其余的工作。 好吧...有!
In this post, we’re going to talk about a trick for passing the defined variables to the view in a somewhat more convenient way: we can use the PHP native compact() function to handpick the data we need, by referring to the variables by name:
在本文中,我们将讨论一种以更方便的方式将定义的变量传递给视图的技巧:我们可以使用PHP本机的compact()函数通过引用变量来手动选择所需的数据。按名字:
<?php
// Twig initialization
// Preparing data
$user = 'user data';
$posts = 'posts';
$comments = 'comments';
$twig->render('author.page', compact('user', 'posts'));
// ...
compact()
accepts a list of names, looks for variables with those names (within the current scope) and returns them all as one associative array.
compact()
接受一个名称列表,查找具有这些名称的变量(在当前作用域内),并将它们全部作为一个关联数组返回。
Sometimes it’s even more convenient to specify the variables we don’t need instead of those we do. Since there’s no built-in solution in PHP to compact all the variables (within the current scope) with a few of them excluded, we have to do this as a three-step process.
有时,指定我们不需要的变量甚至更方便些。 由于PHP中没有内置的解决方案来压缩所有变量(在当前范围内),而其中的一些变量被排除在外,因此我们必须分三步进行。
First we need to get all the variables within the controller’s scope with get_defined_vars()
:
首先,我们需要使用get_defined_vars()
获取控制器范围内的所有变量:
<?php
// ...
$variables = get_defined_vars();
// ...
Then, we filter out the unwanted variable names from wanted ones, by using PHP’s array_diff function.
然后,通过使用PHP的array_diff函数,从需要的变量名中过滤掉不需要的变量名。
array_diff
compares an array against one or more arrays, and returns the entries in the first array, which are not present in any of the other arrays.
array_diff
将一个数组与一个或多个数组进行比较,并返回第一个数组中的条目,这些条目在任何其他数组中都不存在 。
<?php
// ...
$variables = get_defined_vars();
$including = array_diff(array_keys($variables), ['user', 'posts', 'comments']);
// ...
Finally, we can extract our desired variables (listed in $including
) out of $variables
by using array_intersect_key()
.
最后,我们可以使用array_intersect_key()
从$variables
提取所需变量(列在$including
array_intersect_key()
。
array_intersect_key()
accepts a number of arrays and returns the entries of the first array the keys of which exist in all the other arrays.
array_intersect_key()
接受多个数组,并返回第一个数组的项,其键存在于所有其他数组中。
Please note since array_intersect_key()
compares keys to get the intersection of the arrays, we need to switch the keys with their associated values in $including
by using array_flip():
请注意,由于array_intersect_key()
比较键以获取数组的交集,因此我们需要使用array_flip()来切换键及其在$including
的关联值, $including
:
<?php
// ...
$variables = get_defined_vars();
$including = array_diff(array_keys($variables), ['user', 'posts', 'comments']);
$vars = array_intersect_key($variables, array_flip($including));
// ...
Now, to make the procedure reusable, we can abstract the complexity into a helper function:
现在,为了使该过程可重用,我们可以将复杂性抽象为一个辅助函数:
<?php
//Helpers.php
// ...
if (!function_exists('only_compact')) {
function only_compact($values, $keys) {
$keys = array_diff(array_keys($values), $keys);
return array_intersect_key($values, array_flip($keys));
}
And this is how it will make things easier:
这就是使事情变得容易的方法:
<?php
// Twig initialization...
// Preparing data
$user = 'user data';
$posts = 'posts';
$comments = 'comments';
$anotherOne = 'some value';
$yetAnotherOne = 0;
$andAnotherOne = 0;
$counter = 0;
$test_1 = 1;
$test_2 = 2;
$test_3 = 3;
$test_4 = 4;
$test_5 = 5;
$test_6 = 6;
// Even more variables ...
$twig->render('author.page', only_compact(get_defined_vars(), ['counter', 'twig']));
);
// ...
As a result, we get an array of variables with all the unwanted variables excluded.
结果,我们得到了一个变量数组,其中排除了所有不需要的变量。
To use the helper file, we can add it under files
(under autoload
) in composer.json
:
要使用帮助程序文件,我们可以将其添加到composer.json
files
下(在autoload
下):
// ...
"autoload":{
"files": [
"helpers.php"
]
}
// ...
Since we’re using PHP’s built-in functions, the performance impact isn’t noticeable. Below is the result of a quick profile test done with Blackfire, before and after using the above techniques:
由于我们使用的是PHP的内置函数,因此对性能的影响并不明显。 以下是在使用上述技术之前和之后使用Blackfire进行的快速配置文件测试的结果:
Without using the functions:
不使用功能:

With using the functions:
使用功能:

As you can see, the results of both profile runs are almost the same.
如您所见,两个概要文件运行的结果几乎相同。
Hopefully, this trick will save you some typing!
希望这个技巧可以节省您一些打字的时间!
Have any other tips to share in regards to templating and view efficiency? Let us know!
还有其他关于模板化和查看效率的技巧吗? 让我们知道!
翻译自: https://www.sitepoint.com/quick-tip-convenience-hacks-for-passing-data-to-views/
vue 父组件视图传递数据