When creating WordPress plugins and themes, which will be used across thousands of websites, you need to be cautious about how to handle both the data coming into WordPress, and the data that is being presented to the user.
创建将在数以千计的网站中使用的WordPress插件和主题时,您需要谨慎对待如何处理进入WordPress的数据以及呈现给用户的数据。
In this tutorial, we are going to look at the native functions that can secure, clean and check data that is coming in or going out of WordPress. This is necessary when creating a settings page, HTML form, manipulating shortcodes, and so on.
在本教程中,我们将研究可以保护,清理和检查来自WordPress的数据的本机功能。 在创建设置页面,HTML表单,操作短代码等时,这是必需的。
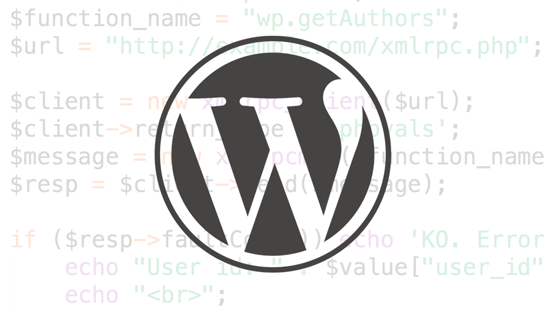
什么是消毒? (What is Sanitizing?)
In a nutshell, sanitizing is cleaning user input. It is the process of removing text, characters or code from input that is not allowed.
简而言之, 消毒就是清洁用户输入 。 这是不允许从输入中删除文本,字符或代码的过程。
Gmail Example: Gmail removes <style>
tags and their contents from HTML email messages before they are displayed on the Gmail browser client. This is done to prevent email CSS from overwriting Gmail styles.
Gmail示例: Gmail会从HTML电子邮件中删除<style>
标记及其内容,然后再在Gmail浏览器客户端上显示它们。 这样做是为了防止电子邮件CSS覆盖Gmail样式。
WordPress Example: Widget titles cannot have HTML tags in them. If you put HTML tags in them, then they are automatically removed before the title is saved.
WordPress示例:窗口小部件标题中不能包含HTML标签。 如果将HTML标记放入其中,则在保存标题之前会自动将其删除。
There are various functions provided by WordPress to sanitize different data into different forms. Here are some of them:
WordPress提供了各种功能,可以将不同的数据清除为不同的形式。 这里是其中的一些:
sanitize_email() (sanitize_email())
This function strips out all characters that are not allowed in an email address. Code example:
此功能会删除电子邮件地址中不允许的所有字符。 代码示例:
<?php
echo sanitize_email("narayan prusty@sitepoint.com"); //Output "narayanprusty@sitepoint.com"
Email address don’t allow whitespace characters. Therefore, the whitespace characters were removed from my email address.
电子邮件地址不允许使用空格字符。 因此,空格字符已从我的电子邮件地址中删除。
sanitize_file_name() (sanitize_file_name())
This function strips characters from a filename that can cause issues while referencing the file in the command line. This function is used by WordPress Media Uploader to sanitize media file names. Code example:
此函数从文件名中剥离字符,这些字符在命令行中引用文件时可能导致问题。 WordPress Media Uploader使用此功能来清理媒体文件名。 代码示例:
<?php
echo sanitize_file_name("_profile pic--1_.png"); //Output "profile-pic-1_.png"
Here, the underscore at the beginning of the name was removed and double dashes were replaced by one single dash. And, finally, whitespace was replaced by a single dash.
此处,名称开头的下划线被删除,双破折号替换为一个破折号。 最后,空白由一个破折号代替。
sanitize_key() (sanitize_key())
Options, Meta Data and Transients Keys can only have lowercase alphanumeric characters, dashes and underscores. This function is used to sanitize the keys. Code example:
选项,元数据和瞬态键只能包含小写字母数字字符,破折号和下划线。 此功能用于清洁按键。 代码示例:
<?php
echo sanitize_key("http://SitePoint.com"); //Output "httpsitepointcom"
Here, uppercase characters were converted to lowercase characters and other invalid characters were removed.
在这里,大写字符转换为小写字符,其他无效字符也被删除。
sanitize_text_field() (sanitize_text_field())
This function removes invalid UTF-8 characters, converts HTML specific characters to entities, strips all tags, and removes line breaks, tabs and extra whitespace, strip octets.
此函数删除无效的UTF-8字符,将特定于HTML的字符转换为实体,去除所有标签,并去除换行符,制表符和多余的空格,去除八位字节。
WordPress uses this to sanitize widget titles.
WordPress使用它来清理小部件标题。
<?php
echo sanitize_text_field("<b>Bold<</b>"); //Output "Bold<"
sanitize_title() (sanitize_title())
This function removes PHP and HTML tags from a string, as well as removing accents. Whitespace characters are converted to dashes.
此函数从字符串中删除PHP和HTML标记,以及删除重音符号。 空格字符将转换为破折号。
Note: This function is not used to sanitize titles. For sanitizing titles, you need to use sanitize_text_field
. This function is used by WordPress to generate the slug for the posts/pages from the post/page title. Code example:
注意:此功能不用于清理标题。 为了sanitize_text_field
标题,您需要使用sanitize_text_field
。 WordPress使用此功能从帖子/页面标题生成帖子/页面的信息。 代码示例:
<?php
echo sanitize_title("Sanítizing, Escaping and Validating Data in WordPress"); //Output "sanitizing-escaping-and-validating-data-in-wordpress"
Here the í
character was converted to i
and whitespaces were replaced with the -
character. And, finally, uppercase characters were converted to lowercase characters.
此处í
字符被转换为i
,空白被-
字符替换。 最后,将大写字符转换为小写字符。
什么是转义? (What is Escaping?)
In a nutshell, escaping is securing output. This is done to prevent XSS attack and also to make sure that the data is displayed the way the user expects it to be.
简而言之, 逃避就是确保输出 。 这样做是为了防止XSS攻击,并确保以用户期望的方式显示数据。
Escaping converts the special HTML characters to HTML entities so that they are displayed, instead of being executed.
转义将特殊HTML字符转换为HTML实体,以便显示它们,而不是执行它们。
Example: Facebook escapes the chat messages while displaying them. To make sure that users don’t run code on each other’s computer.
示例: Facebook在显示聊天消息时将其转义。 为了确保用户不会在彼此的计算机上运行代码。
WordPress provides some functions to escape different varieties of data.
WordPress提供了一些功能来转义不同种类的数据。
esc_html() (esc_html())
This functions escapes HTML specific characters. Example code:
此函数转义HTML特定字符。 示例代码:
<?php
echo esc_html("<html>HTML</html>"); //Output "<html>HTML</html>"
esc_textarea() (esc_textarea())
Use esc_textarea()
instead of esc_html()
while displays text in textarea. Because esc_textarea()
can double encode entities.
在textarea中显示文本时,请使用esc_textarea()
而不是esc_html()
。 因为esc_textarea()
可以对实体进行双重编码。
esc_attr() (esc_attr())
This function encodes the <
,>
, &
, "
and '
characters. It will never double encode entities. This function is used to escape the value of HTML tags attributes.
此函数对<
, >
, &
, "
和'
字符进行编码。它将永远不会对实体进行双重编码。此函数用于转义HTML标签属性的值。
<?php
echo esc_html("<html>HTML</html>"); //Output "<html>HTML</html>"
esc_url() (esc_url())
URLs can also contain JavaScript code in them. So, if you want to display a URL or a complete <a>
tag, then you should escape the href
attribute or else it can cause an XSS attack.
网址中也可以包含JavaScript代码。 因此,如果要显示URL或完整的<a>
标记,则应转义href
属性,否则它将导致XSS攻击。
<?php
$url = "javascript:alert('Hello')";
?>
<a href="<?php echo esc_url($url);?>">Text</a>
esc_url_raw() (esc_url_raw())
This is used if you want to store a URL in a database or use in URL redirecting. The difference between esc_url
and esc_url_raw
is that esc_url_raw
doesn’t replace ampersands and single quotes.
如果要将URL存储在数据库中或用于URL重定向,则使用此方法。 之间的区别esc_url
和esc_url_raw
是esc_url_raw
不会替换&符号和单引号。
antispambot() (antispambot())
There are lots of email bots, which are constantly looking for email addresses. We may want to display the email address to the users, but not want it to be recognised by email bots. antispambot
allows us to do that exactly.
有很多电子邮件机器人,它们一直在寻找电子邮件地址。 我们可能希望向用户显示电子邮件地址,但不希望它被电子邮件机器人识别。 antispambot
允许我们精确地做到这一点。
antispambot
converts email address characters to HTML entities to block spam bots. Example code:
antispambot
将电子邮件地址字符转换为HTML实体,以阻止垃圾邮件机器人。 示例代码:
<?php
echo antispambot("narayanprusty@sitepoint.com"); //Output "narayanprusty@sitepoint.com"
什么是验证? (What is Validating?)
In a nutshell, validating is checking user input. This is done to check if the user has entered a valid value.
简而言之, 验证就是检查用户输入 。 这样做是为了检查用户是否输入了有效值。
If data is not valid, then it is not processed or stored. The user is asked to enter the value again.
如果数据无效,则不进行处理或存储。 要求用户再次输入该值。
Example: While creating an account on a site, we are asked to enter the password twice. Both the passwords are validated; they are checked to confirm whether they both are same or not.
示例:在网站上创建帐户时,要求我们输入两次密码。 这两个密码均已通过验证; 检查它们以确认它们是否相同。
You shouldn’t rely on HTML5 validation as it can be easily bypassed. Server side validation is required before processing or storing specific data.
您不应该依赖HTML5验证,因为可以轻松地绕过它。 在处理或存储特定数据之前,需要服务器端验证。
WordPress provides a couple of functions to validate only some types of data. Developers usually define their own functions for validate data. Let’s see some WordPress provided validation functions:
WordPress提供了一些功能来仅验证某些类型的数据。 开发人员通常定义自己的功能来验证数据。 让我们看看WordPress提供的一些验证功能:
is_email() (is_email())
Email validation is required while submitting comments, contact forms, and creating an account. is_email()
function is provided by WordPress to check if a given is an email address or not. Code example:
提交评论,联系表格和创建帐户时,需要电子邮件验证。 WordPress提供了is_email()
函数来检查给定的电子邮件地址。 代码示例:
<?php
if(is_email("narayanprusty@sitepoint.com"))
{
echo "Valid Email";
}
else
{
echo "Invalid Email";
}
is_serialized() (is_serialized())
is_serialized()
checks if the passed data is string or not. WordPress uses this function while storing options, meta data and transients. If value associated with a key is not a string then WordPress serializes it before storing in database.
is_serialized()
检查所传递的数据是否为字符串。 WordPress在存储选项,元数据和瞬态时使用此功能。 如果与键关联的值不是字符串,则WordPress在存储到数据库之前对其进行序列化。
Here is example code on how you can use it:
这是有关如何使用它的示例代码:
<?php
$data = array("a", "b", "c");
//while storing
if(!is_serialized($data))
{
//serialize it
$data = maybe_serialize($data);
//or else ask user to re-input the data
}
//while displaying
echo maybe_unserialize($data);
结论 (Conclusion)
We saw what sanitizing, validating and escaping are, and why it is important for every developer to know the functions associated with them. You can find more reading on the topic at the Data Validation Codex page on WordPress.org. It is always a good idea to include these functions when developing a WordPress theme or plugin. Unfortunately, quite a lot of plugins are poorly developed, and do not escape the output. The result is that they make the website open to potential XSS attacks. Please feel free to include any comments or helpful tips in the section below.
我们看到了什么是消毒,验证和转义,以及为什么每个开发人员都知道与其相关的功能很重要。 您可以在WordPress.org上的Data Validation Codex页面上找到有关该主题的更多信息。 开发WordPress主题或插件时,最好包括这些功能。 不幸的是,很多插件开发欠佳,并且无法逃避输出。 结果是它们使网站容易受到潜在的XSS攻击。 请随时在以下部分中添加任何评论或有用的提示。
翻译自: https://www.sitepoint.com/sanitizing-escaping-validating-data-in-wordpress/