twilio
With the advent of cloud-based telephony services such as Twilio, writing an application that works with SMS messages and telephone calls has never been easier. In addition to allowing you to send SMS messages via their simple API, Twilio also allows you to process incoming messages which opens up a whole world of possible applications.
随着基于云的电话服务(例如Twilio)的出现 ,编写可处理SMS消息和电话的应用程序从未如此简单。 除了允许您通过其简单的API发送SMS消息外,Twilio还允许您处理传入的消息,从而打开了可能的应用程序的整个世界。
In this article, I’ll show you how you can use Twilio to build a simple SMS-based stock quote service which handles both incoming SMS messages and sending responses. The application will allow the user to request a stock price for a given company via SMS and receive it a few moments later via the same method.
在本文中,我将向您展示如何使用Twilio构建一个简单的基于SMS的股票报价服务,该服务可以处理传入的SMS消息和发送响应。 该应用程序将允许用户通过SMS请求给定公司的股票价格,并在稍后通过相同方法接收股票价格。
在你开始前 (Before You Start)
First – if you haven’t done so already – you’ll need to create an account with Twilio.
首先-如果您尚未这样做,则需要使用Twilio 创建一个帐户 。
Once you’re signed up, you need to requisition (hire, in essence) a phone number which is what people will send messages to in order to use the service. Go to twilio.com/user/account/phone-numbers/incoming and click “Buy a number” to pick an available number. You may wish to pick one specific to your region, but you’re free to pick any of the available numbers – the only variable is cost.
注册后,您需要索取(实质上是租用)电话号码,人们将使用该电话号码向其发送消息以使用该服务。 转到twilio.com/user/account/phone-numbers/incoming ,然后单击“购买号码”以选择可用的号码。 您可能希望选择一个特定于您所在地区的数字,但您可以自由选择任何可用数字-唯一的变量是成本。
Then go to the number you’ve selected, check the SMS box, and enter the URL you’re going to use for your application (it has to be somewhere publicly accessible) followed by /callback
. For example:
然后转到您选择的号码,选中SMS框,然后输入要用于您的应用程序的URL(该URL必须是可公共访问的位置),然后是/callback
。 例如:
http://example.com/callback
Take note of your account SID and the corresponding auth token (they appear at the top of your account page) – you’ll need them later.
记下您的帐户SID和相应的身份验证令牌(它们显示在帐户页面的顶部)–以后将需要它们。
应用程序 (The Application)
The user will request a stock price via SMS and the application will process the incoming message, extract the relevant data, use an external web service to grab the requested information, and send back the response to the user in a second SMS.
用户将通过SMS请求股票价格,应用程序将处理传入的消息,提取相关数据,使用外部Web服务获取请求的信息,然后在第二条SMS中将响应发送回用户。
The process will be as follows:
该过程将如下所示:
- the user sends a command via SMS to a specified number 用户通过短信将命令发送到指定号码
- the SMS is handled by Twilio, which “pings” the configured end-point (our application) SMS由Twilio处理,它“ ping”配置的端点(我们的应用程序)
- our application parses the command and extracts the company code 我们的应用程序解析命令并提取公司代码
- the application pings an external web service, passing the company code as a parameter 应用程序ping外部Web服务,并将公司代码作为参数传递
- that web service returns a price 该网络服务返回价格
- the application pings the Twilio API with a response and the number to send the SMS to 应用程序使用响应和发送ping短信的号码ping通Twilio API。
- the user receives an SMS with the requested stock price 用户收到带有要求的股价的短信
This process is illustrated in the following diagram:
下图说明了此过程:
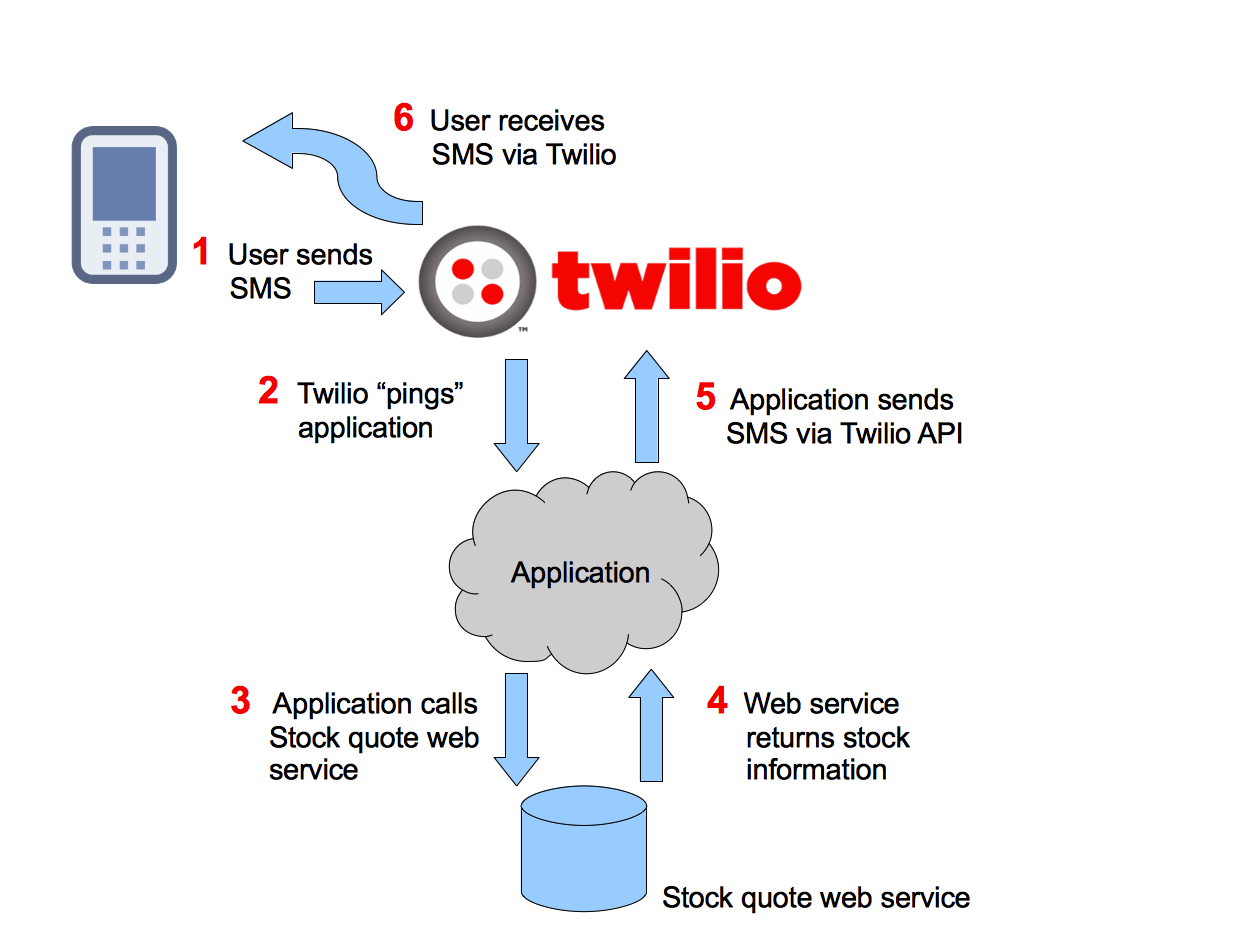
Sound complicated? Don’t worry, it’s really not! Let’s get started.
听起来复杂吗? 不用担心,实际上不是! 让我们开始吧。
建立基础 (Building the Foundation)
The easiest way to get started is to use composer. Create a composer.json
file in your project root with the following:
最简单的入门方法是使用作曲家。 使用以下命令在项目根目录中创建composer.json
文件:
{
"require": {
"slim/slim": "2.3.0",
"slim/extras": "dev-develop",
"twilio/sdk": "dev-master"
}
}
I’m using the Slim framework; however, much of the code will be re-usable across whatever framework you favor (if indeed you wish to use a framework at all).
我正在使用Slim框架; 但是,许多代码都可以在您喜欢的任何框架中重用(如果确实希望使用框架)。
Now run:
现在运行:
php composer.phar install
You’ll find that the Slim framework now resides in the newly-created vendor
folder, along with the official Twilio SDK, and some extra goodies (we’re going to use some logging functionality from Slim Extras later).
您会发现Slim框架现在连同新的官方Twilio SDK一起位于新创建的vendor
文件夹中,还有一些其他功能(稍后我们将使用Slim Extras的某些日志记录功能)。
Create an includes
folder, and in that a file called config.php
:
创建一个includes
文件夹,然后在其中创建一个名为config.php
的文件:
return array(
'twilio' => array(
'account_sid' => 'YOUR-ACCOUNT-SID',
'auth_token' => 'YOUR-AUTH-TOKEN',
'phone_number' => 'YOUR-PHONE-NUMBER',
),
);
Of course you’ll need to replace these values with the ones you got from Twilio earlier.
当然,您需要将这些值替换为您先前从Twilio获得的值。
Create a public
directory in the project root and point your Apache configuration to serve files from this folder.
在项目根目录中创建一个public
目录,并指向您的Apache配置以提供该文件夹中的文件。
Now create an .htaccess
file in the public folder:
现在在公用文件夹中创建一个.htaccess
文件:
RewriteEngine On
RewriteCond %{REQUEST_FILENAME} !-f
RewriteRule ^ index.php [QSA,L]
This will route all requests for non-existent files to a central controller file, index.php
.
这会将所有对不存在文件的请求路由到中央控制器文件index.php
。
Now for the index.php
file:
现在为index.php
文件:
<?php
require '../vendor/autoload.php';
$config = require '../includes/config.php';
$app = new SlimSlim();
$app->get('/', function () use ($app) {
print 'Installation successful';
});
$app->run();
Try browsing to the application – you should see the “Installation successful” message.
尝试浏览到该应用程序–您应该看到“安装成功”消息。
Because we’re building an application that’s not going to render content for a browser, it will be very helpful to implement some logging. Slim logs to STDERR by default, but the Slim Extras library referred to in the composer.json
file contains a file-based log writer.
因为我们正在构建的应用程序不会为浏览器呈现内容,所以实现一些日志记录将非常有帮助。 Slim默认情况下会记录到STDERR,但是composer.json
文件中引用的Slim Extras库包含基于文件的日志编写器。
Change the instantiation of the new Slim
object to:
将新的Slim
对象的实例化更改为:
$app = new SlimSlim(array(
'log.writer' => new SlimExtrasLogDateTimeFileWriter(array(
'path' => '../logs',
'name_format' => 'Y-m-d',
'message_format' => '%label% - %date% - %message%'
))
));
Then, create the logs
folder in the project root and make it writable by the web server.
然后,在项目根目录中创建logs
文件夹,并使其可被Web服务器写入。
Now you can access the logger as follows:
现在,您可以按以下方式访问记录器:
$log = $app->getLog();
And log messages like this:
并记录以下消息:
$log->info('This is a message');
处理传入的短信 (Handling the Incoming SMS)
We’ve specified our callback which gets pinged when an incoming SMS is received. Specifically, by “pinged” I mean that a POST request is made to the specified URL with two key pieces of information:
我们已经指定了回调,当收到传入的SMS时会对其执行ping操作。 具体来说,“ ping”是指对带有两个关键信息的指定URL进行POST请求:
- the sender’s number 发件人的号码
- the content of the SMS 短信内容
The sender’s number and message are sent as POST variables – From
and Body
respectively.
-发送者的电话号码和邮件发送为POST变量From
与Body
分别。
So, let’s create the callback – I’ll keep it simple for now and just log the information:
因此,让我们创建回调-现在让我保持简单,只记录信息:
$app->post('/callback', function () use ($app) {
$log = $app->getLog();
$sender = $app->request()->post('From');
$message = $app->request()->post('Body');
$log->info(sprintf('Message received from %s: %s', $sender, $message));
});
Try sending an SMS to your configured number and then check the logs – the log entry should show up within a few minutes. If you don’t see anything, Twilio keeps a record of activity so you may be able to debug the process from there.
尝试将SMS发送到您配置的号码,然后检查日志-日志条目应在几分钟内显示出来。 如果您什么都没看到,Twilio会保留活动记录,以便您可以从那里调试过程。
The format of the message the application will accept is: COMMAND ARGUMENT
. It will only accept one command – PRICE
– as in, “give me the share price for..”, and a single argument, the company code.
应用程序将接受的消息格式为: COMMAND ARGUMENT
。 它只会接受一个命令PRICE
例如“给我股票价格...”,以及一个参数,即公司代码。
Here’s an example:
这是一个例子:
PRICE AAPL
What the command means is “Send me Apple, Inc.’s current share price”.
该命令的意思是“向我发送Apple,Inc.的当前股价”。
By incorporating a command, we’re leaving open the option of adding additional functionality later. For example, perhaps you may want to offer the facility to SUBSCRIBE
to regular updates.
通过合并命令,我们将在以后保留添加其他功能的选项。 例如,也许您可能想提供该功能来SUBSCRIBE
定期更新。
To parse the incoming message we simply need to extract the command and the argument – we can use a simple regular expression for this:
要解析传入的消息,我们只需要提取命令和参数–我们可以为此使用一个简单的正则表达式:
$pattern = '/^([A-Z]+) ([A-Z]+)/';
preg_match($pattern, $subject, $matches);
print_r($matches);
If you try this with the example earlier, you should see this:
如果您在前面的示例中尝试过此操作,则应该看到以下内容:
Array
(
[0] => PRICE AAPL
[1] => PRICE
[2] => AAPL
)
The relevant parts are in positions 1 and 2.
相关部分位于位置1和2。
$command = $matches[1];
$argument = $matches[2];
Although there’s only one valid command, there’s no harm in thinking ahead and using a switch to determine what action to take based on the command.
尽管只有一个有效的命令,但预先考虑并使用开关来确定基于该命令采取的操作并没有什么害处。
switch ($command) {
case 'PRICE':
// perform action
break;
default:
print 'Invalid command!';
}
获取股票价格 (Getting the Stock Price)
I’m going to use a web service to get the latest share price for a given company. Free stock services are few and far between – and even then there are often delays, but there’s one here.
我将使用网络服务来获取给定公司的最新股价。 免费股票服务是少之又少-即使这样经常有延迟,但有一个在这里 。
Create a directory called src
in the project root, and in that a directory called SitePoint
, and in that a file called StockService.php
:
在项目根目录中创建一个名为src
的目录,在该目录中创建一个名为SitePoint
的目录,并在其中创建一个名为StockService.php
的文件:
<?php
namespace SitePoint;
class StockService
{
}
In order for the autoloader to pick up the location of this class, add an autoloader declaration in your composer.json
:
为了使自动加载器选择此类的位置,请在composer.json
添加一个自动加载器声明:
"autoload": {
"psr-0": {"SitePoint\": "src/"}
}
Now run:
现在运行:
php composer.phar update
To implement a method for grabbing the stock quote for a given company, create a public method in the StockService
class like this:
要实现获取给定公司股票报价的方法,请在StockService
类中创建一个公共方法,如下所示:
public static function GetQuote($symbol) {
$client = new SoapClient('http://www.webservicex.net/stockquote.asmx?WSDL');
$params = array(
'symbol' => $symbol,
);
$response = $client->__soapCall('GetQuote', array($params));
$quotes = simplexml_load_string($response->GetQuoteResult);
return $quotes->Stock[0];
}
All I’ve done is create an instance of SoapClient
and passed it the URL to the service’s WSDL file, and then call the GetQuote
method, process the XML, and return the first (and only) Stock
element.
我要做的就是创建一个SoapClient
实例,并将URL传递给服务的WSDL文件,然后调用GetQuote
方法,处理XML,并返回第一个(也是唯一一个) Stock
元素。
You can add a new route to test it:
您可以添加新的路线进行测试:
$app->get('/quote', function () use ($app) {
$symbol = $app->request()->get('symbol');
$quote = SitePointStockService::GetQuote($symbol);
var_dump($quote);
});
If you call http://localhost/quote?symbol=AAPL, you should see something like this:
如果调用http:// localhost / quote?symbol = AAPL ,则应该看到类似以下内容的内容:
object(SimpleXMLElement)#39 (16) {
["Symbol"]=>
string(4) "AAPL"
["Last"]=>
string(6) "431.31"
["Date"]=>
string(9) "7/18/2013"
["Time"]=>
string(6) "1:03pm"
["Change"]=>
string(5) "+1.00"
["Open"]=>
string(6) "433.55"
["High"]=>
string(6) "434.87"
["Low"]=>
string(6) "430.61"
["Volume"]=>
string(7) "4878034"
["MktCap"]=>
string(6) "404.8B"
["PreviousClose"]=>
string(6) "430.31"
["PercentageChange"]=>
string(6) "+0.23%"
["AnnRange"]=>
string(15) "385.10 - 705.07"
["Earns"]=>
string(6) "41.896"
["P-E"]=>
string(5) "10.27"
["Name"]=>
string(10) "Apple Inc."
}
In the next section, we’ll finish off by collating this information into an SMS message and send it to the requesting user’s mobile phone.
在下一部分中,我们将把这些信息整理成一条SMS消息并将其发送到发出请求的用户的手机中,以完成此操作。
通过短信回复 (Responding via SMS)
The first thing to do here is construct the message the application is going to reply with:
这里要做的第一件事是构造应用程序将要回复的消息:
// construct the response
$response = sprintf('%s: %s (%s) [Hi: %s Lo: %s]',
$quote->Name,
$quote->Last,
$quote->Change,
$quote->High,
$quote->Low
);
Based on the information earlier, you’d end up with a message like this:
根据前面的信息,您最终会得到如下消息:
Apple Inc.: 431.31 (+1.000) [Hi: 434.87 Lo: 430.61]
To send it as an SMS, instantiate the Twilio
class and call the method which corresponds to the required REST endpoint:
要将其作为SMS发送,请实例化Twilio
类并调用与所需REST端点相对应的方法:
// instantiate the Twilio client
$twilio = new Services_Twilio(
$config['twilio']['account_sid'],
$config['twilio']['auth_token']
);
// send the SMS
$sms = $twilio->account->sms_messages->create(
$config['twilio']['phone_number'], // the number to send from
$sender,
$response
);
… and that’s it!
…就是这样!
There’s still some work to be done, such as performing proper error handling, and the command parsing is a little unforgiving. Feel free to download the code from GitHub and play around!
仍然需要完成一些工作,例如执行正确的错误处理,并且命令解析有些不容忍。 可以从GitHub免费下载代码并随意玩!
摘要 (Summary)
In this article I’ve demonstrated how you can use Twilio to both receive and send SMS messages and how you can build a simple application from scratch to deliver a piece of requested information directly to someone’s mobile phone. In practice our example isn’t incredibly useful; stock prices fluctuate far quicker than you can send an SMS – but perhaps you can think of other uses?
在本文中,我演示了如何使用Twilio接收和发送SMS消息,以及如何从头开始构建简单的应用程序以将所需的信息直接传递到某人的手机。 实际上,我们的示例并不是非常有用。 股票价格的波动远快于您发送短信的速度-但是也许您还能想到其他用途?
You may also wish to play around with adding new commands. Perhaps instead of a service with an instant response, you subscribe to regular updates? Or even set some thresholds, for example “send me an SMS if the share price drops below x”). Feel free to pop any other ideas in the comments below!
您可能还希望尝试添加新命令。 也许您订阅了定期更新,而不是提供即时响应的服务? 甚至设置一些阈值,例如“如果股价跌至x以下,请发送短信给我”)。 随时在下面的评论中弹出其他任何想法!
Image via Fotolia
图片来自Fotolia
翻译自: https://www.sitepoint.com/create-an-sms-based-stock-quote-service-with-twilio/
twilio