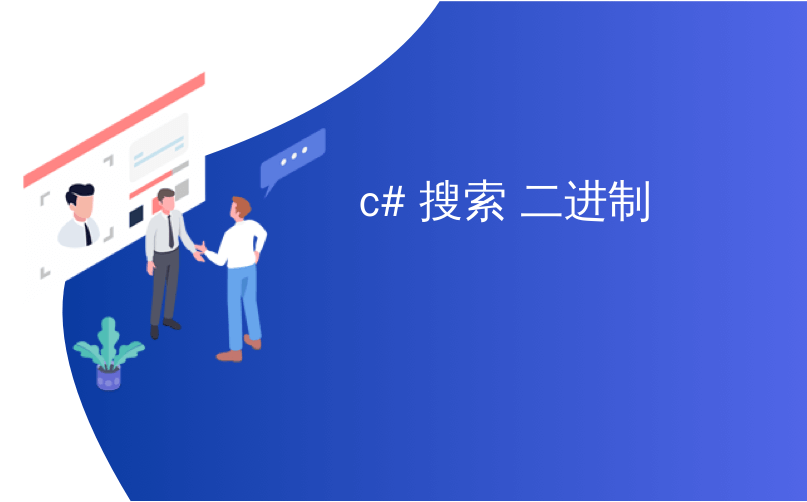
c# 搜索 二进制
Here you will get program for binary search in C.
在这里,您将获得用C进行二进制搜索的程序。
Binary search algorithm can be applied on a sorted array to search an element. Search begins with comparing middle element of array to target element. If both are equal then position of element is returned. If target element is less than middle element of array then upper half of array is discarded and again search continued by dividing the lower half. If target element is greater than middle element then lower half is discarded and search is continued in upper half.
二进制搜索算法可以应用于排序后的数组以搜索元素。 搜索开始于将数组的中间元素与目标元素进行比较。 如果两者相等,则返回元素的位置。 如果目标元素小于数组的中间元素,则丢弃数组的上半部分,并通过划分下半部分再次继续搜索。 如果目标元素大于中间元素,则将下半部分丢弃,并在上半部分继续搜索。
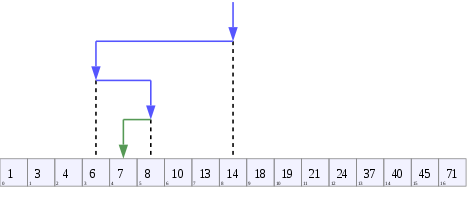
Worst Case Time Complexity: O(log n)
最坏情况下的时间复杂度: O(log n)
Best Case Time Complexity: O(1)
最佳情况下时间复杂度: O(1)
Also Read: Linear Search in C
另请阅读: C语言中的线性搜索
C语言二进制搜索程序 (Program for Binary Search in C)
Below program shows the implementation of binary search algorithm in C.
下面的程序显示了C语言中二进制搜索算法的实现。
#include<stdio.h>
int main()
{
int arr[50],i,n,x,flag=0,first,last,mid;
printf("Enter size of array:");
scanf("%d",&n);
printf("\nEnter array element(ascending order)\n");
for(i=0;i<n;++i)
scanf("%d",&arr[i]);
printf("\nEnter the element to search:");
scanf("%d",&x);
first=0;
last=n-1;
while(first<=last)
{
mid=(first+last)/2;
if(x==arr[mid]){
flag=1;
break;
}
else
if(x>arr[mid])
first=mid+1;
else
last=mid-1;
}
if(flag==1)
printf("\nElement found at position %d",mid+1);
else
printf("\nElement not found");
return 0;
}
Output
输出量
Enter size of array:6
输入数组大小:6
Enter array element(ascending order) 20 27 40 50 58 99
输入数组元素(升序) 20 27 40 50 58 99
Enter the element to search:27
输入要搜索的元素:27
Element found at position 2
在位置2找到元素
影片教学 (Video Tutorial)
翻译自: https://www.thecrazyprogrammer.com/2017/11/binary-search-c.html
c# 搜索 二进制