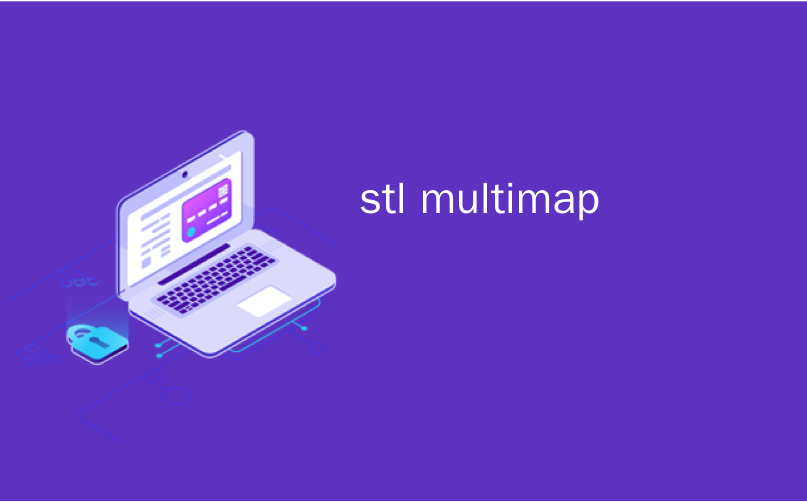
stl multimap
In this tutorial you will learn about stl multimap i.e., std::multimap and all functions applicable on it with some example code.
在本教程中,您将通过一些示例代码学习stl multimap,即std :: multimap及其适用的所有函数。
In previous articles we already learned about std::map container. It is an associative container which give priority to key values. But problem with map is it won’t allow duplicate values. But multimap allows duplicate values. Here in multimap both key and value pair becomes unique. And we already know that one of the great property of map and multimap is always elements automatically inserted into sorted order on fly. Keys can’t be modified once inserted. Only possible way is we have to delete and update with a new value.
在先前的文章中,我们已经了解了std :: map容器 。 它是一个关联容器,它优先考虑键值。 但是map的问题是它不允许重复的值。 但是多图允许重复值。 在多图中,键和值对都变得唯一。 我们已经知道map和multimap的一大特性就是总是将元素自动动态插入到排序顺序中。 密钥一旦插入便无法修改。 唯一可能的方法是我们必须删除并使用新值进行更新。
Implementation of multimaps follows a binary search tree type of implementation.
多图的实现遵循二进制搜索树类型的实现。
C ++ STL多图容器– std :: multimap (C++ STL Multimap Container – std::multimap)
To work with multimap we need to include map header file.
要使用多地图,我们需要包含地图头文件。
#include <map>
#include <map>
Iterators that can be applicable on multimap:
可以应用于多图的迭代器:
begin(): returns iterator to the beginning.
begin():将迭代器返回到开头。
end(): returns iterator to the end of the map.
end():将迭代器返回到地图的末尾。
rbegin(): returns reverse iterator to reverse beginning.
rbegin():将反向迭代器返回为反向开始。
rend(): returns reverse iterator to reverse end.
rend():将反向迭代器返回到反向端点。
cbegin(): Returns constant iterator to the beginning.
cbegin():将常数迭代器返回到开头。
cend(): Returns constant iterator to the end.
cend():将常量迭代器返回到末尾。
Declaring a multimap:
声明多图:
multimap < datatype, datatype > multiMapName;
multimap <数据类型,数据类型> multiMapName;
This is for key and value pair.
这是用于键和值对的。
Now see some basic operations on multimap:
现在看一下多图上的一些基本操作:
Insert operation: Insert operations effects size of multimap.
插入操作 :插入操作会影响多图的大小。
multiMapName.insert (pair (datatype, datatype ) ( keyt, value ))
multiMapName.insert(对(数据类型,数据类型)(keyt,value))
Another type of insertion is, we can directly assign one multimap elements to other multimap elements directly by giving range using iterators.
另一种插入类型是,我们可以使用迭代器指定范围,从而直接将一个多图元素直接分配给其他多图元素。
multimap <datatype, datatype> newMmap (oldMmap.begin(), oldMmap.end())
多图<数据类型,数据类型> newMmap(oldMmap.begin(),oldMmap.end())
size(): This will return the number of key value pairs in the multimap.
size():这将返回多重映射中的键值对的数量。
max_size(): This will return the what is the maximum capacity of the multimap
max_size() :这将返回多图的最大容量
clear(): This will truncate all elements from multimap
clear():这将截断multimap中的所有元素
empty(): This is a Boolean operation. Returns true if multimap is empty. Returns false if multimap is not empty.
empty() :这是布尔运算。 如果multimap为空,则返回true。 如果multimap不为空,则返回false。
Example program to explain all above functions is:
解释上述所有功能的示例程序是:
#include <iostream>
#include <map>
#include <iterator>
using namespace std;
int main()
{
multimap <int, int> mmp; // declaring an empty multimap
multimap <int, int> :: iterator it;
// inserting elements
for (int i=1; i<=5; i++ ) {
mmp.insert (pair <int, int> (i, 10*i));
}
// printing elements in multimap
cout << "Elements in multimap (key and value) pair wise" << endl;
for (it = mmp.begin(); it != mmp.end(); it++ ) {
cout << it->first << '\t' << it->second << endl;
}
cout << endl;
// assigning all elements of multimap1 to multimap2
multimap <int, int> mmp2(mmp.begin(),mmp.end());
//printing elements of multimap2
cout << "Elements in multimap2 (key and value) pair wise" << endl;
for (it = mmp2.begin(); it != mmp2.end(); it++ ) {
cout << it->first << '\t' << it->second << endl;
}
cout << endl;
cout << "Size of the multimap is " ;
cout << mmp.size() << endl;
cout << endl << "Maximum size of the multimap is " ;
cout << mmp.max_size() << endl;
cout << endl << "Applying clear operation on multimap2..." <<endl;
mmp2.clear();
cout << endl << "Checking wheter multimap2 is empty or not using empty() operation" << endl;
if (mmp2.empty()) {
cout << "Multimap2 is empty " << endl;
}
else {
cout << "Multimap2 is not empty " << endl;
}
return 0;
}
Output
输出量
Elements in multimap (key and value) pair wise 1 10 2 20 3 30 4 40 5 50
多图中的元素(键和值)成对配对 1 10 2 20 3 30 4 40 5 50
Elements in multimap2 (key and value) pair wise 1 10 2 20 3 30 4 40 5 50
multimap2中的元素(键和值)成对配对 1 10 2 20 3 30 4 40 5 50
Size of the multimap is 5
多图的大小为5
Maximum size of the multimap is 461168601842738790
多重地图的最大大小为461168601842738790
Applying clear operation on multimap2…
在multimap2上应用清除操作…
Checking wheter multimap2 is empty or not using empty() operation Multimap2 is empty
检查哪个multimap2为空或不使用empty()操作 Multimap2为空
Some other operations are:
其他一些操作是:
find(key): This will find all elements with specified key value.
find(key) :这将查找具有指定键值的所有元素。
erase(key): This will delete the value with specified key value.
delete(key):这将删除具有指定键值的值。
swap(): This operations swaps all key value pairs of multimap1 to multimap2 and same way multimap2 to multimap1.
swap():此操作将multimap1的所有键值对交换为multimap2,并以相同的方式将multimap2交换为multimap1。
upper_bound(): This will return the iterator to upper bound.
upper_bound():这将使迭代器返回上限。
lower_bound(): This will return the iterator to lower bound.
lower_bound():这将使迭代器返回下限。
Example program to show above operations:
显示上述操作的示例程序:
#include <iostream>
#include <map>
#include <iterator>
using namespace std;
int main()
{
multimap <int, int> mmp;
multimap <int, int> mmp2;
multimap <int, int> :: iterator it;
multimap <int, int> :: iterator it1;
multimap <int, int> :: iterator it2;
for (int i=1; i<=5; i++ ) {
mmp.insert (pair <int, int> (i, 10*i));
}
for (int i=1; i<=5; i++ ) {
mmp2.insert (pair <int, int> (i*3, i*i*i));
}
cout << "Elements in multimap1 before swapping are" << endl;
for (it = mmp.begin(); it != mmp.end(); it++ ) {
cout << it->first << '\t' << it->second << endl;
}
cout << endl;
cout << "Elements in multimap2 before swapping are" << endl;
for (it = mmp2.begin(); it != mmp2.end(); it++ ) {
cout << it->first << '\t' << it->second << endl;
}
cout << endl;
cout << "Performing swapping operation......" << endl;
mmp.swap(mmp2);
cout << "Elements in multimap1 after swapping are" << endl;
for (it = mmp.begin(); it != mmp.end(); it++ ) {
cout << it->first << '\t' << it->second << endl;
}
cout << endl;
cout << "Elements in multimap2 after swapping are" << endl;
for (it = mmp2.begin(); it != mmp2.end(); it++ ) {
cout << it->first << '\t' << it->second << endl;
}
cout << endl;
it= mmp.begin();
mmp.erase (it); // erasing first element of the mmp
cout << "After erasing first element in multimap1" << endl;
for (it = mmp.begin(); it != mmp.end(); it++ ) {
cout << it->first << '\t' << it->second << endl;
}
cout << "\nUsing lower bound and upper bound for printing" << endl;
it1= mmp2.lower_bound(2);
it2= mmp2.upper_bound(4);
for (it = it1; it != it2; it++ ) {
cout << it->first << '\t' << it->second << endl;
}
return 0;
}
Output
输出量
Elements in multimap1 before swapping are 1 10 2 20 3 30 4 40 5 50
交换之前multimap1中的元素是 1 10 2 20 3 30 4 40 5 50
Elements in multimap2 before swapping are 3 1 6 8 9 27 12 64 15 125
交换之前multimap2中的元素是 3 1 6 8 9 27 12 64 15 125
Performing swapping operation…… Elements in multimap1 after swapping are 3 1 6 8 9 27 12 64 15 125
执行交换操作……交换 后multimap1中的元素为 3 1 6 8 9 27 12 64 15 125
Elements in multimap2 after swapping are 1 10 2 20 3 30 4 40 5 50
交换后multimap2中的元素是 1 10 2 20 3 30 4 40 5 50
After erasing first element in multimap1 6 8 9 27 12 64 15 125
删除multimap中的第一个元素后1 6 8 9 27 12 64 15 125
Using lower bound and upper bound for printing 2 20 3 30 4 40
使用下限和上限进行打印 2 20 3 30 4 40
Comment below if you have any queries related to above stl multimap or std::multimap tutorial.
如果您对上述stl multimap或std :: multimap教程有任何疑问,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2017/10/stl-multimap.html
stl multimap